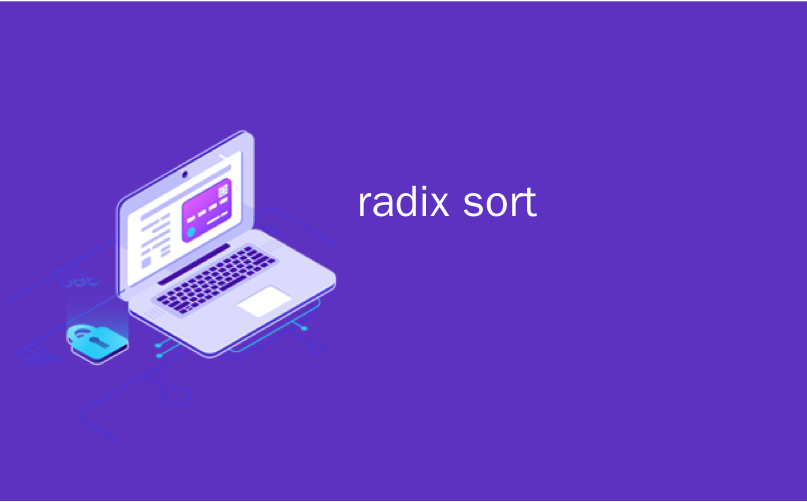
radix sort
In this tutorial you will learn about radix sort program in C.
在本教程中,您将学习C语言中的基数排序程序。
Radix sorting technique is one of the oldest techniques of sorting.
基数排序技术是最古老的排序技术之一。
Lets assume that we are given a list of some names and asked to sort them alphabetically. We would normally proceed by first dividing the names into 26 different sets (since, there are total of 26 alphabets) with each set containing names that start with the same alphabet. In the first set, we will put all the names which start with alphabet ‘A’. In the Second Set, we will put all the names that start with ‘B’ and so on.
让我们假设我们得到了一些名称的列表,并要求它们按字母顺序排序。 通常,我们通常先将名称分为26个不同的集合(因为总共有26个字母),每个集合都包含以相同字母开头的名称。 在第一组中,我们将所有以字母“ A”开头的名称。 在第二组中,我们将放置所有以“ B”开头的名称,依此类推。
Also Read: Quick Sort in C [Program & Algorithm]
另请阅读: 用C快速排序[程序和算法]
After this comparison of the first alphabets of all the names, each set is further divided into different sub-sets according to the second alphabet of the names.
在比较所有名称的第一字母之后,根据名称的第二字母将每个集合进一步划分为不同的子集。
This process continues till the list becomes completely sorted.
这个过程一直进行到列表完全排序为止。
However, the drawback of radix sorting technique is that we have to keep track of lots of sets and its sub-sets simultaneously.
但是,基数排序技术的缺点是我们必须同时跟踪许多集合及其子集合。
To ease out on this drawback, there are two methods available in radix sorting:
为了缓解此缺点,基数排序有两种方法可用:
1. Most Significant Digit Radix Sort (MSD)
1.最重要的数字基数排序(MSD)
2. Least Significant Digit Radix Sort (LSD)
2.最低有效数字基数排序(LSD)
In least significant digit radix sort, sorting is performed digit by digit starting from the least significant digit and ending at the most significant digit.
在最低有效位基数排序中,从最低有效位开始到最高有效位逐位进行排序。
The concept of most significant sorting is to divide all the digits with an equal value into their own set and then do the same thing with all the sets until all of them are sorted.
最重要的排序概念是将具有相等值的所有数字划分为自己的集合,然后对所有集合进行相同的操作,直到对所有集合进行排序。
基数排序算法分析 (Analysis of Radix Sort ALgorithm)
The number of passes p is equal to the number of digits in largest element and in every pass (iteration) we have n operations where n is the total number of elements. In this program we can see that the outer loop iterates p times and inner loop iterates n times. Hence, run time complexity of radix sort is denoted by O(p*n).
遍数p等于最大元素中的位数,并且在每遍(迭代)中,我们有n次运算,其中n是元素总数。 在此程序中,我们可以看到外循环迭代p次,内循环迭代n次。 因此,基数排序的运行时复杂度用O(p * n)表示。
If the number of digits in largest element is equal to n, then the run time becomes O(n square) which is the worst case scenario in radix sort. The best case scenario of radix sort is if the number of digits in largest element is Log n. In this case, the run time becomes O(n log n).
如果最大元素中的位数等于n,则运行时间变为O(n square),这是基数排序中最坏的情况。 基数排序的最佳情况是最大元素中的位数为Log n。 在这种情况下,运行时间变为O(n log n)。
The major disadvantage of radix sort is the need for extra memory for maintaining Queues and hence it is not an in-place sort. It is therefore a stable sort.
基数排序的主要缺点是需要额外的内存来维护队列,因此它不是就地排序。 因此,这是一种稳定的排序。
C语言中的Radix Sort程序 (Radix Sort Program in C)
#include<stdio.h>
#include<stdlib.h>
struct node
{
int data ;
struct node *next;
}*start=NULL;
void radix_sorting();
int larger_dig();
int digit(int num, int k);
main()
{
struct node *temp,*q;
int count,n,num_item;
printf("Enter the Number of Elements to be Inserted into the List : ");
scanf("%d", &n);
for(count=0;count<n;count++)
{
printf("Enter element %d : ",count+1);
scanf("%d",&num_item);
temp= malloc(sizeof(struct node));
temp->data=num_item;
temp->next=NULL;
if(start==NULL)
start=temp;
else
{
q=start;
while(q->next!=NULL)
q=q->next;
q->next=temp;
}
}
radix_sorting();
printf("Sorted List is :\n");
q=start;
while( q !=NULL)
{
printf("%d ", q->data);
q = q->next;
}
printf("\n");
}
void radix_sorting()
{
int count,k,dig,least_sig,most_sig;
struct node *p, *rear[10], *front[10];
least_sig=1;
most_sig=larger_dig(start);
for(k=least_sig; k<=most_sig; k++)
{
for(count=0; count<=9 ; count++)
{
rear[count] = NULL;
front[count] = NULL ;
}
for( p=start; p!=NULL; p=p->next )
{
dig = digit(p->data, k);
if(front[dig] == NULL)
front[dig] = p ;
else
rear[dig]->next = p;
rear[dig] = p;
}
count=0;
while(front[count] == NULL)
count++;
start = front[count];
while(count<9)
{
if(rear[count+1]!=NULL)
rear[count]->next=front[count+1];
else
rear[count+1]=rear[count];
count++;
}
rear[9]->next=NULL;
}
}
int larger_dig()
{
struct node *p=start;
int large=0,ndigit=0;
while(p != NULL)
{
if(p ->data > large)
large = p->data;
p = p->next ;
}
while(large != 0)
{
ndigit++;
large = large/10 ;
}
return(ndigit);
}
int digit(int num, int k)
{
int digit, count ;
for(count=1; count<=k; count++)
{
digit = num % 10 ;
num = num /10 ;
}
return(digit);
}
Output
输出量
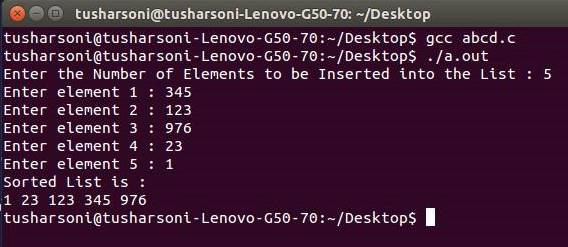
If you found anything incorrect or have doubts regarding above radix sort program in C then comment below.
如果您发现任何不正确的地方或对上面C中的基数排序程序有疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2015/09/radix-sort-program-in-c.html
radix sort