In this tutorial you will learn about Java StringBuffer class and its important methods with examples.
在本教程中,您将通过示例了解Java StringBuffer类及其重要方法。
StringBuffer in Java is used to create mutable strings. Here mutable means, we can modify or change the strings.
Java中的StringBuffer用于创建可变字符串。 这里是可变的,我们可以修改或更改字符串。
StringBuffer class is thread safe which means multiple threads can’t access the same object simultaneously.
StringBuffer类是线程安全的,这意味着多个线程不能同时访问同一对象。
Apart from StringBuffer, we can also make mutable strings using StringBuilder class. We will discuss about it in upcoming tutorials.
除了StringBuffer之外,我们还可以使用StringBuilder类制作可变的字符串。 我们将在以后的教程中对此进行讨论。
Java StringBuffer构造函数 (Java StringBuffer Constructors)
StringBuffer class in Java provides following four constructors.
Java中的StringBuffer类提供以下四个构造函数。
StringBuffer() – Creates an empty string with the default capacity of 16 characters.
StringBuffer() –创建一个空字符串,默认容量为16个字符。
StringBuffer(int size) – Creates an empty string with the specified capacity.
StringBuffer(int size) –创建具有指定容量的空字符串。
StringBuffer(String str) – Creates a string with an initial value contained by str. The total capacity of created string is the sum of length of initial value and 16 more characters.
StringBuffer(String str) –创建一个包含str初始值的字符串。 创建的字符串的总容量是初始值的长度和16个其他字符的总和。
StringBuffer(CharSequence chars) – Creates a string with an initial value contained by chars. It also reserves extra space for 16 more characters.
StringBuffer(CharSequence chars) –创建一个字符串,其初始值包含在chars中。 它还为额外的16个字符保留了额外的空间。
Note: When we do modification in a given string and if the length of new string exceeds the current capacity then the capacity is increased by (oldcapacity*2)+2.
注意:当我们在给定的字符串中进行修改时,如果新字符串的长度超过当前容量,则容量将增加(oldcapacity * 2)+2。
Below I have discussed few important methods of StringBuffer class which are different from methods of String in Java.
下面,我讨论了StringBuffer类的一些重要方法,这些方法与Java中的String方法不同。
Java StringBuffer方法 (Java StringBuffer Methods)
StringBuffer length()和Capacity()方法 (StringBuffer length() and capacity() Methods)
The length of string value present in StringBuffer object is calculated by length() method. The total capacity of an object is calculated by capacity() method. See below example code to understand it more clearly.
StringBuffer对象中存在的字符串值的长度是通过length()方法计算的。 对象的总容量是通过Capacity()方法计算的。 请参阅下面的示例代码,以更清楚地了解它。
Syntax
句法
int length()
int capacity()
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("Hello");
System.out.println("length = "+str.length());
System.out.println("capacity = "+str.capacity());
}
}
Output
输出量
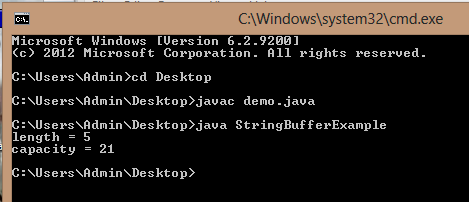
In above example str.length() gives output 5 because length of Hello is 5. While str.capacity() gives output 21 because space for 16 more characters is automatically added.
在上面的示例中,因为Hello的长度是5,str.length()给出了输出5,而str.capacity()给出了输出21,因为自动添加了16个字符的空格。
StringBuffer sureCapacity()方法 (StringBuffer ensureCapacity() Method)
This method ensures that the given capacity is the minimum to the current capacity. If the given capacity is more than the current capacity then the current capacity is increased by (oldcapacity*2)+2. For example if the current capacity is 20 then it will become (20*2)+2 = 42.
此方法可确保给定容量是当前容量的最小值。 如果给定容量大于当前容量,那么当前容量将增加(oldcapacity * 2)+2。 例如,如果当前容量为20,则它将变为(20 * 2)+2 = 42。
Syntax
句法
void ensureCapacity(int minCapacity)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("Hello");
System.out.println("capacity = "+str.capacity());
str.ensureCapacity(30);
System.out.println("capacity = "+str.capacity());
}
}
Output
输出量
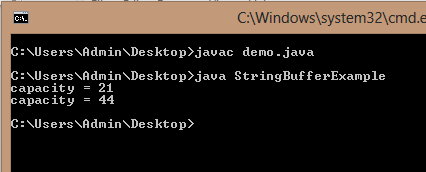
StringBuffer setLength()方法 (StringBuffer setLength() Method)
As its name shows, it is used to set the length of string within an object of StringBuffer class. If the new length is less than the current length then the characters beyond the new length are lost.
顾名思义,它用于设置StringBuffer类的对象内字符串的长度。 如果新长度小于当前长度,则超出新长度的字符将丢失。
Syntax
句法
void setLength(int len)
StringBuffer charAt()和setCharAt()方法 (StringBuffer charAt() and setCharAt() Methods)
The charAt() method is used to access a particular character in given string. It is same as we use for String class object. We can replace a particular character in a string with a new character using setCharAt() method.
charAt()方法用于访问给定字符串中的特定字符。 与用于String类对象的方法相同。 我们可以使用setCharAt()方法用新字符替换字符串中的特定字符。
Syntax
句法
char charAt(int where)
void setCharAt(int where, char ch)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("Hello");
System.out.println("before = "+str);
str.setCharAt(1,'a');
System.out.println("after = "+str);
}
}
Output
输出量
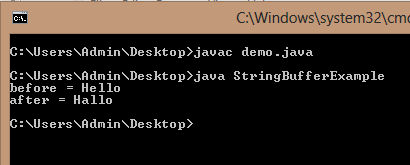
StringBuffer append()方法 (StringBuffer append() Method)
The append() method is used to add a string at the end of given string. It has several overloaded versions as given below.
append()方法用于在给定字符串的末尾添加字符串。 它具有几个重载的版本,如下所示。
Syntax
句法
StringBuffer append(String str)
StringBuffer append(int num)
StringBuffer append(Object obj)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("Hello");
System.out.println("before = "+str);
str.append("World");
System.out.println("after = "+str);
}
}
Output
输出量
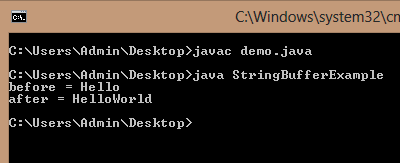
StringBuffer insert()方法 (StringBuffer insert() Method)
The insert() method is used to insert a string in given string. Same as like append() method it also has various overloaded versions. We have to specify the index where we want to insert and the string to be inserted as arguments.
insert()方法用于在给定的字符串中插入一个字符串。 与like append()方法相同,它也具有各种重载版本。 我们必须指定要插入的索引和要插入的字符串作为参数。
Syntax
句法
StringBuffer insert(int index, String str)
StringBuffer insert(int index, char ch)
StringBuffer insert(int index, Object obj)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("TheProgrammer");
System.out.println("before = "+str);
str.insert(3,"Crazy");
System.out.println("after = "+str);
}
}
Output
输出量
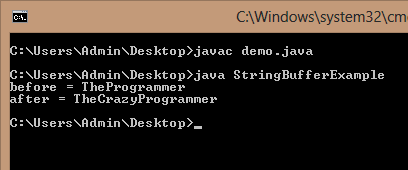
StringBuffer reverse()方法 (StringBuffer reverse() Method)
The characters within a StringBuffer object can be reversed using reverse() method.
可以使用reverse()方法反转StringBuffer对象中的字符。
Syntax
句法
StringBuffer reverse()
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("TheCrazyProgrammer");
System.out.println("before = "+str);
str.reverse();
System.out.println("after = "+str);
}
}
Output
输出量
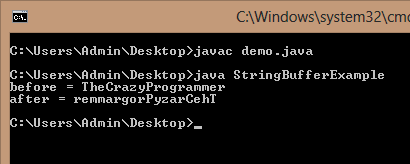
StringBuffer replace()方法 (StringBuffer replace() Method)
The replace() method is used to replace a part of given string with another string. We have to just specify the startindex, endindex and the string with which we want to replace as arguments.
replace()方法用于将给定字符串的一部分替换为另一个字符串。 我们只需要指定startindex,endindex和我们要替换的字符串作为参数即可。
Note: Here the end index is one less than what we have specified in the argument i.e. endIndex – 1.
注意:此处的结束索引比我们在参数中指定的索引endIndex –小1。
Syntax
句法
StringBuffer replace(int startIndex, int endIndex, String str)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("TheCrazyProgrammer");
System.out.println("before = "+str);
str.replace(8,18,"Coder");
System.out.println("after = "+str);
}
}
Output
输出量
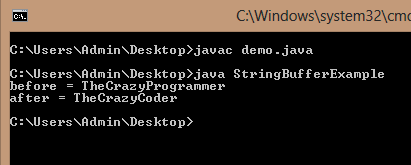
StringBuffer delete()和deleteCharAt()方法 (StringBuffer delete() and deleteCharAt() Method)
The delete() method is used to delete a sequence of characters from start to end index. The deleteCharAt() method is used to delete single character at specific index.
delete()方法用于删除从开始到结束索引的一系列字符。 deleteCharAt()方法用于删除特定索引处的单个字符。
Note: Here the end index is one less than what we have specified in the argument i.e. endIndex – 1.
注意:此处的结束索引比我们在参数中指定的索引endIndex –小1。
Syntax
句法
StringBuffer delete(int startIndex, int endIndex)
StringBuffer deleteCharAt(int where)
Example
例
class StringBufferExample
{
public static void main(String...s)
{
StringBuffer str=new StringBuffer("HelloWorld");
System.out.println("before = "+str);
str.delete(0,2);
System.out.println("delete(0,2) = "+str);
str.deleteCharAt(1);
System.out.println("deleteCharAt(1) = "+str);
}
}
Output
输出量
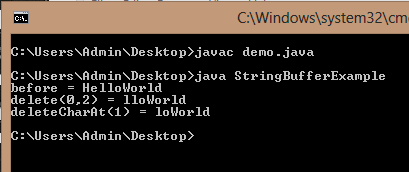
There are so many other methods of Java StringBuffer class. If you found anything incorrect or have any doubts regarding above tutorial then please comment below.
Java StringBuffer类还有许多其他方法。 如果您发现任何不正确的地方或对以上教程有任何疑问,请在下面发表评论。
翻译自: https://www.thecrazyprogrammer.com/2015/09/java-stringbuffer-class.html