In the previous tutorial, we have looked over the Introduction to Python Programming. Now lets jump to actual programming. In this tutorial we will write our first Python program.
在上一教程中,我们研究了Python编程简介 。 现在让我们跳到实际编程。 在本教程中,我们将编写第一个Python程序。
To start Python in Windows Environment Click Start > Python > Python IDLE
要在Windows环境中启动Python,请 单击开始> Python> Python IDLE。
As soon as you start Python IDLE, you get a Python Shell which lets you use an Interactive Mode in Python Programming Environment.
一旦启动Python IDLE,您将获得一个Python Shell,可让您在Python编程环境中使用交互模式。
Python Hello World程序 (Python Hello World Program)
print("Hello World");
Press Enter after typing this statement. You will get the output as shown below.
键入此语句后,按Enter键。 您将获得如下所示的输出。
Output
输出量
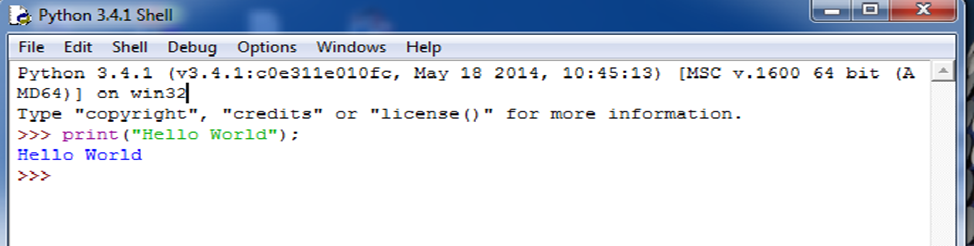
Note: Before Python 3, print statement was used. After Python 3 version print statement is modified to print() function. Still if you use print statement in newer version then you will not get an error. One more thing to note here is, semicolon (;) is optional in Python. If you don’t add semicolon at the end of a statement then you will not get an error.
注意:在Python 3之前,使用了print语句。 在Python 3版本中,将print语句修改为print()函数。 不过,如果您在较新的版本中使用print语句,则不会收到错误。 这里要注意的另一件事是,分号(;)在Python中是可选的。 如果不在语句末尾添加分号,则不会出现错误。
Python is very clear and concise. It has excellent easy to use interface and a very easy learning environment.
Python非常清晰简洁。 它具有出色的易于使用的界面和非常容易的学习环境。
Python in Windows provides us with a Graphical User Interface Integrated into the IDLE. Python IDLE provides us with two modes of environment:
Windows中的Python为我们提供了集成在IDLE中的图形用户界面。 Python IDLE为我们提供了两种环境模式:
1. Script Mode Environment 2. Interactive Mode Environment
1.脚本模式环境 2.交互模式环境
Interactive Mode comes in by default that lets you use the Python programming environment in Python Shell. There, you can directly write commands and execute it to see the output. It is best for evaluation purposes. Interactive Mode is probably the quickest way to try out a small idea and check its results.
交互模式默认情况下进入,可让您在Python Shell中使用Python编程环境。 在这里,您可以直接编写命令并执行命令以查看输出。 最好是出于评估目的。 交互模式可能是尝试一个小主意并检查其结果的最快方法。
As told in the previous tutorial, Python is a scripting language rather than just a programming language. The Interactive Mode is perfect if you plan to save programs and run them later. To start the Script Mode in Python IDLE, select File at the top left corner of Python Shell and click on New File.
如上一教程所述,Python是一种脚本语言,而不仅仅是一种编程语言。 如果您计划保存程序并在以后运行它们,则“交互模式”是理想的选择。 要启动脚本模式在Python IDLE,在顶部选择文件离开Python Shell中的一角,然后单击新建文件 。
You can think of Python Interactive Mode as a scratchpad wherein you can try new ideas quickly so as to verify its usage whereas Python’s Script Mode is where you can craft the final product.
您可以将Python交互模式视为便笺簿,您可以在其中快速尝试新想法以验证其用法,而Python的脚本模式是您制作最终产品的地方。
Here, you can write your programs in a file and save it to create an executable file.
在这里,您可以将程序写入文件并保存以创建可执行文件。
Python Code is case-sensitive. So, print, Print and PRINT are different. Python doesn’t accept Print or print statements. It generally accepts only lowercase characters. It has been found that Python code length is around 40% of what other languages such as C, Java, C++ and others consume.
Python代码区分大小写。 因此,打印,打印和打印不同。 Python不接受Print或print语句。 它通常只接受小写字符。 已经发现,Python代码长度约为其他语言(如C,Java,C ++和其他语言)消耗的40%。
修改了Python Hello World程序 (Python Hello World Program Modified)
#Hello World Program
print("Hello World");
input("\nPress Enter to Exit");
Note: If you’re using Python 2.7, you may have to use raw_input instead of input.
注意:如果使用的是Python 2.7,则可能必须使用raw_input而不是input。
Explanation
说明
1. The first line of this program is a Comment. The Python interpreter completely ignores these comment lines and is totally for the programmer. Comments are invaluable feature for any programmer. It lets us to understand the program in a better way as it can be easily interpreted by humans in any language so as to understand the code in a better way.
1.该程序的第一行是注释。 Python解释器完全忽略了这些注释行,完全供程序员使用。 注释对于任何程序员来说都是无价之宝。 它使我们能够以更好的方式理解程序,因为人类可以轻松地以任何语言解释它,从而以更好的方式理解代码。
Syntax
句法
#comment comes here
2. The Second line is the print command which lets us to display a statement over the console screen for the End User of the program. This command tells the computer to display text on the screen. You can use either quotation marks (double) or apostrophes in the print command.
2.第二行是print命令,它使我们可以在控制台屏幕上为程序的最终用户显示一条语句。 此命令告诉计算机在屏幕上显示文本。 您可以在打印命令中使用双引号或双引号。
Syntax
句法
print("Expression");
OR
要么
print('Expression');
An Expression can be a statement like Hello World or something like 2+5 which is evaluate to 7 on hitting Enter.
表达式可以是诸如Hello World之类的语句,也可以是2 + 5之类的语句,在按Enter键时其求值为7。
3. The Third statement is probably more useful in Script Mode Environment in Python. This statement helps the output screen to wait for the user to press Enter and then Exit the program otherwise it will just execute within seconds and the program terminates. You can relate it to getch() function in C Programming Environment.
3. Third语句在Python的脚本模式环境中可能更有用。 该语句有助于输出屏幕等待用户按下Enter键,然后退出程序,否则它将在几秒钟内执行并终止程序。 您可以将其与C编程环境中的getch()函数关联。
Syntax
句法
input("Statements");
This was all about our Python hello world program. If you have any doubts regarding above tutorial then feel free to ask it by commenting below.
这一切都与我们的Python hello world程序有关。 如果您对以上教程有任何疑问,请随时在下面评论以提出疑问。
翻译自: https://www.thecrazyprogrammer.com/2015/08/python-hello-world-program.html