We have completed the basic introduction to C++ and the language features. Hoping that you have set the environment necessary to run the C++ programs in your machine. Let us jump to write first C++ program to print hello world. Before that we need to know some basics of the language.
我们已经完成了对C ++和语言功能的基本介绍 。 希望您已经设置了在计算机中运行C ++程序所需的环境。 让我们跳到编写第一个C ++程序来打印问候世界。 在此之前,我们需要了解该语言的一些基础知识。
The ISO C++ standard defines two kinds of entities: 1. Core language features, such as built-in types and loops. 2. Standard-library components, such as containers and I/O operations.
ISO C ++标准定义了两种实体: 1.核心语言功能,例如内置类型和循环。 2.标准库组件,例如容器和I / O操作。
The standard-library components are perfectly ordinary C++ code provided by every C++ version implementation. Actually the C++ standard library is implemented in C++ itself which makes us know that the language is expressive enough and powerful. We’ll discuss more about them in detail when we move on to further tutorials.
标准库组件是每个C ++版本实现提供的完美的普通C ++代码。 实际上,C ++标准库是用C ++本身实现的,这使我们知道该语言具有足够的表达能力和强大的功能。 当我们继续学习进一步的教程时,我们将详细讨论它们。
第一个C ++程序– Hello World (First C++ Program – Hello World)
#include<iostream> //header file
using namespace std;
int main ()
{
cout<<"Hello Wolrd";
return 0;
}
Output
输出量
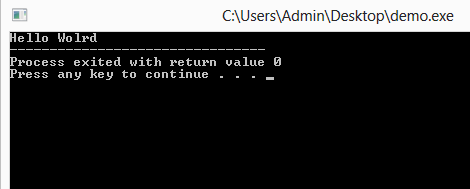
int main() (int main())
Like C, C++ is also a collection of several functions. In the above program we have only one function named main(). This is the necessary default function that every program must contain. Its return type may vary according to the user requirement. Execution of C program begins from the main function. The compiler ignores the white spaces, and carriage returns. You can indent the tokens with any number of white spaces. Just like C, every program statement must end with a semicolon in C++. The whole code comes inside curly braces {}. It shows the body of the main() function
像C一样,C ++也是多个函数的集合。 在上面的程序中,我们只有一个名为main()的函数。 这是每个程序必须包含的必需的默认功能。 其返回类型可能会根据用户要求而有所不同。 C程序的执行从主要功能开始。 编译器将忽略空格,并返回回车符。 您可以使用任意数量的空格缩进标记。 与C一样,每个程序语句在C ++中都必须以分号结尾。 整个代码放在花括号{}中。 它显示了main()函数的主体
注释 (Comments)
If you are familiar with C, you might remember that it allows us multiple line comments enclosed in /* and */ comment symbols. These are still valid in C++. Comments increases the readability and makes the code easy to understand.
如果您熟悉C,您可能还记得它允许我们在/ *和* /注释符号中包含多行注释。 这些在C ++中仍然有效。 注释增加了可读性并使代码易于理解。
/* this is a multiple line comment here you can comment about the program author name, inputs, outputs, control flow etc. */
/ *这是多行注释 ,您可以在此处注释程序 作者的名称,输入,输出,控制流等。 * /
Apart from this multiple line comments, C++ introduced single line comments with a // symbol preceding the comment. You can see the first line of the program, to see such type of comments. They allow only single line comments and these are ignored by the compiler.
除了这些多行注释之外,C ++还引入了单行注释,并在注释之前加上了//符号。 您可以看到程序的第一行,以查看此类注释。 它们仅允许单行注释,编译器将忽略它们。
// this is a single line comments // you can give a short description here of the line or about of the following code block
//这是一行注释 // //您可以在此处对该行或以下代码块进行简短描述
#include <iostream> (#include<iostream>)
The first line of the program tells the preprocessor to include contents of iostream header file to the program. We use this header file as it contains the declarations of identifier cout and the operator <<.
程序的第一行告诉预处理器将iostream头文件的内容包括到程序中。 我们使用此头文件,因为它包含标识符cout和运算符<<的声明。
ANSI C++ introduced new changes to the header files. Usually we used to write the header files in old versions of C++ as “iostream.h”. If your compiler doesn’t support ANSI C++ then make sure that you change the header file as “iostream.h”. Note that some implementations also use as “iostream.hpp”. We must include the proper header files according to our compiler implementation.
ANSI C ++对头文件进行了新的更改。 通常,我们以前在C ++的旧版本中将头文件编写为“ iostream.h”。 如果您的编译器不支持ANSI C ++,请确保将头文件更改为“ iostream.h”。 请注意,某些实现也用作“ iostream.hpp”。 根据我们的编译器实现,我们必须包括适当的头文件。
Old version #include<iostream.h>
旧版本 #include <iostream.h>
New version #include<iostream>
新版本 #include <iostream>
使用命名空间标准 (using namespace std)
Namespace is a new concept in ISO C++. This defines the scope of identifiers that we use in our program. For using the identifiers defined in namespace scope we need to include it in the program. In above program std is the namespace where the C++ standard libraries are found. As we discussed at the start, we have a standard library and core language features.
命名空间是ISO C ++中的一个新概念。 这定义了我们在程序中使用的标识符的范围。 为了使用在命名空间范围内定义的标识符,我们需要将其包括在程序中。 在上面的程序中std是可以找到C ++标准库的名称空间。 正如我们在一开始所讨论的,我们具有标准的库和核心语言功能。
cout&<< (cout & <<)
See the above program, we have written a single statement in the main() function that prints the message to the standard output screen. This statements helps us to know about two new features of C++. First is the object and the second is the operator overloading. cout is a predefined object that represents the standard output in C++. The operator << sends the given message in quotes to the standard output screen using the object cout.
参见上面的程序,我们在main()函数中编写了一条语句,该语句将消息打印到标准输出屏幕。 此语句有助于我们了解C ++的两个新功能。 首先是对象,其次是运算符重载。 cout是一个预定义的对象,代表C ++中的标准输出。 操作员<<使用对象cout将给定消息以引号发送到标准输出屏幕。
You may get a doubt that the operator << is used as bit wise left shift operator in C. Yes it also serves the same purpose in C++. We already are familiar that C++ exhibits polymorphism which means existing in many forms depending on the context. Here we use the operator << as put to operator. And in some cases, we many use it as bit wise left shift operator. This concept is called Operator Overloading.
您可能会怀疑运算符<<在C中用作按位左移运算符。是的,它在C ++中也具有相同的作用。 我们已经很熟悉C ++表现出多态性,这意味着根据上下文以多种形式存在。 这里我们使用运算符<<放在运算符上。 在某些情况下,我们很多人将其用作按位左移运算符。 这个概念称为运算符重载。
So this was our first C++ program to print hello world. You have seen so many strange terms in above tutorial. Don’t worry about them, we will discuss them in upcoming tutorials. If you have any doubts then feel free to ask it by commenting below.
因此,这是我们第一个打印问候世界的C ++程序。 在上面的教程中,您已经看到了很多奇怪的术语。 不用担心,我们将在以后的教程中讨论它们。 如果您有任何疑问,请随时在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2015/07/first-cpp-program-hello-world.html