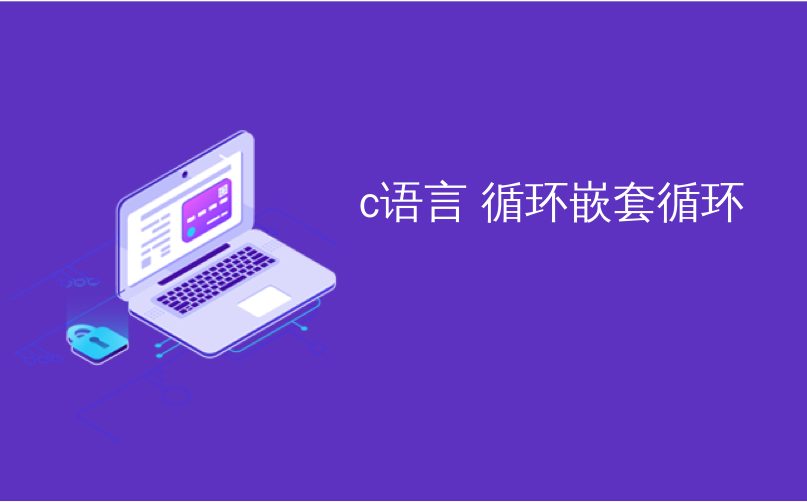
c语言 循环嵌套循环
Here you will learn about nested loops in C.
在这里,您将了解C中的嵌套循环。
Nesting is one of the most complex topics of C programming. Just like decision control instructions, a loop control instruction can also be nested easily. But sometimes understanding the execution of such programs could be a bit tricky. So it is important to make the basics clear.
嵌套是C编程中最复杂的主题之一。 就像决策控制指令一样,循环控制指令也可以轻松嵌套。 但是有时了解此类程序的执行可能会有些棘手。 因此,弄清楚基础知识很重要。
C中的嵌套循环 (Nested loops in C)
As I said in my earlier tutorials, nesting means defining statement under the scope of another similar statement. In case of loops, when we nest two loops then it generally multiplies the execution frequency of loops.
正如我在之前的教程中所说的,嵌套意味着在另一个类似语句的范围内定义语句。 在循环的情况下,当我们嵌套两个循环时,它通常会乘以循环的执行频率。
We can nest for loop inside while loop and vice versa is also true. Generally programmer nest up to 3 loops. But there is no limit of nesting in C.
我们可以在循环内嵌套for循环,反之亦然。 通常,程序员最多嵌套3个循环。 但是在C中嵌套没有限制。
Now let’s try a small program with nested loops.
现在让我们尝试一个带有嵌套循环的小程序。
#include<stdio.h>
void main()
{
int row,col;
for(row=1;row<4;row++)
{
for(col=1;col<4;col++)
{
printf("%d\t%d\n",row,col);
}
}
}
Output
输出量
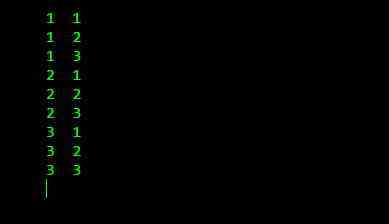
Explanation
说明
- Every row executes a column at least 3 times. Therefore you are seeing all three values with every row. 每行执行一列至少3次。 因此,您将在每一行看到所有三个值。
- Once the control reaches inside the inner loop, it came out only when the loop is fully executed. 一旦控件到达内部循环内部,则仅在循环完全执行后才出现。
- We cannot alter the control once it reaches inside the inner loop. 一旦控件进入内部循环,我们将无法对其进行更改。
Note: I have deliberately shown a simple program to show nesting of loops. Execution of nested loops can’t be summarized in words. So it will be better if you try your hands on the nesting of loops to understand them better.
注意:我故意显示了一个简单的程序来显示循环嵌套。 嵌套循环的执行不能用文字概括。 因此,如果您尝试循环嵌套以更好地理解它们,那就更好了。
奇数循环 (Odd Loop)
There are situations when we need to execute a set of instructions until the user deny it. So we need to check if the user wants to repeat the set of instructions or not.
在某些情况下,我们需要执行一组指令,直到用户拒绝为止。 因此,我们需要检查用户是否要重复这组指令。
In that case programmer has no idea about the executing time and frequency of the program. This is called odd loop in C programming. So basically we have to make a program which will ask to the user about the re-execution of program.
在那种情况下,程序员不知道程序的执行时间和频率。 这在C编程中称为奇数循环。 因此,基本上我们必须制作一个程序,该程序将询问用户有关程序的重新执行。
#include<stdio.h>
void main()
{
char yes='Y';
int x,y;
while(yes=='Y')
{
printf("Enter two values to perform additionn");
scanf("%d%d",&x,&y);
printf("Sum is %d n",x+y);
printf("Do you want to continue ? Press Y for Yes and N for Non");
scanf("%c", &yes);
}
}
Output
输出量
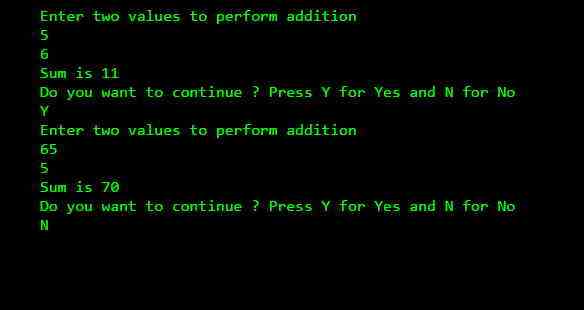
Explanation
说明
- In the beginning I have character variable yes with character initial value ‘Y’. After that I have declared two more integer variables. 一开始,我有字符变量yes,字符初始值为“ Y”。 之后,我又声明了两个整数变量。
- Now I have started while loop with condition yes==’Y’. It means the loop will be executed until it receives value ‘Y’. 现在,我以条件yes =='Y'开始了while循环。 这意味着循环将一直执行,直到收到值“ Y”为止。
- After that I have displayed the message to enter two values. By using scanf() function I have stored data inside the variables. 之后,我显示了输入两个值的消息。 通过使用scanf()函数,我将数据存储在变量中。
- Now instead of using a third variable to calculate sum. I have used a simple argument to calculate sum inside printf() function. 现在,而不是使用第三个变量来计算总和。 我使用了一个简单的参数来计算printf()函数中的总和。
- In the end the program will display the message for user. If he wants to continue then he will press “Y”. Otherwise he will press “N” and the program will be terminated. 最后,程序将为用户显示消息。 如果他想继续,那么他将按“ Y”。 否则,他将按“ N”,程序将终止。
Comment below if you have doubts or found any information incorrect in above tutorial for nested loops in C.
如果您有疑问或在上面的教程中对C中的嵌套循环有任何不正确的信息,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2015/01/nested-loops-in-c.html
c语言 循环嵌套循环