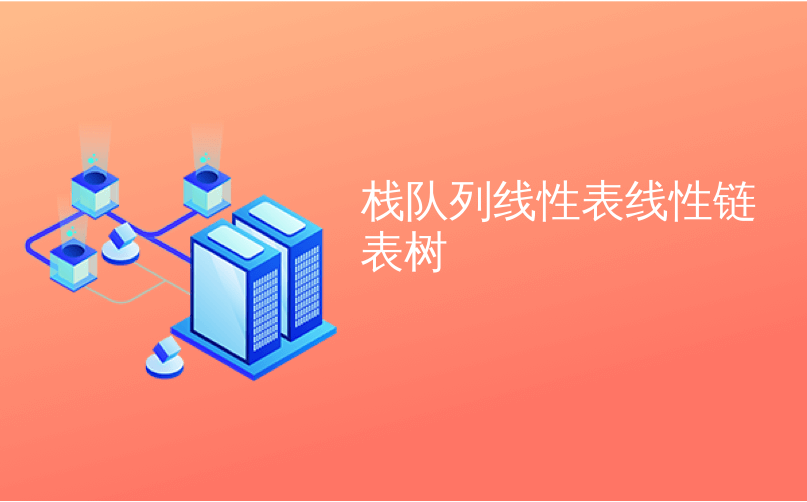
栈队列线性表线性链表树
Here you will learn about linear queue in C++.
在这里,您将了解C ++中的线性队列。
What is Queue?
什么是队列?
The queue is a linear data structure where operations of insertion and deletion are performed at separate ends that are known as front and rear. The queue follows FIFO (First in First Out) concept. First element added to the queue will be first one to be removed.
队列是线性数据结构,其中插入和删除操作在分别称为前端和后端的不同端执行。 队列遵循FIFO(先进先出)概念。 添加到队列中的第一个元素将是第一个要删除的元素。
In this program we will implement linear queue using linked list. It is a menu driven program that contains four options insert, delete, display and exit. The program will ask the user to enter the choice and then appropriate functions are invoked to perform specific operation according to the user’s choice.
在此程序中,我们将使用链表实现线性队列。 它是一个菜单驱动程序,包含四个选项插入,删除,显示和退出。 该程序将要求用户输入选择,然后根据用户的选择调用适当的功能以执行特定的操作。
C ++中的线性队列程序 (Program for Linear Queue in C++ )
#include<iostream>
#include<stdlib.h>
using namespace std;
struct node
{
int data;
struct node *next;
}*front=NULL,*rear,*temp;
void ins()
{
temp=new node;
cout<<"Enter data:";
cin>>temp->data;
temp->next=NULL;
if(front==NULL)
front=rear=temp;
else
{
rear->next=temp;
rear=temp;
}
}
void del()
{
if(front==NULL)
cout<<"Queue is empty\n";
else
{
temp=front;
front=front->next;
cout<<"Deleted node is "<<temp->data<<"\n";
delete(temp);
}
}
void dis()
{
if(front==NULL)
cout<<"Queue is empty\n";
else
{
temp=front;
while(temp!=NULL)
{
cout<<temp->data<<"->";
temp=temp->next;
}
}
}
int main()
{
int ch;
while(1)
{
cout<<"\n\n*** Menu ***"<<"\n1.Insert\n2.Delete\n3.Display\n4.Exit";
cout<<"\n\nEnter your choice(1-4):";
cin>>ch;
cout<<"\n";
switch(ch)
{
case 1: ins();
break;
case 2: del();
break;
case 3: dis();
break;
case 4: exit(0);
break;
default: cout<<"Wrong Choice!!!";
}
}
return 0;
}
Output
输出量
*** Menu ***
***菜单***
1.Insert
1.插入
2.Delete
2.删除
3.Display
3.显示
4.Exit
4.退出
Enter your choice(1-4):1
输入您的选择(1-4):1
Enter data:8
输入数据:8
*** Menu ***
***菜单***
1.Insert
1.插入
2.Delete
2.删除
3.Display
3.显示
4.Exit
4.退出
Enter your choice(1-4):1
输入您的选择(1-4):1
Enter data:12
输入数据:12
*** Menu ***
***菜单***
1.Insert
1.插入
2.Delete
2.删除
3.Display
3.显示
4.Exit
4.退出
Enter your choice(1-4):3
输入您的选择(1-4):3
8->12->
8-12->
*** Menu *** 1.Insert 2.Delete 3.Display 4.Exit
*** ***菜单 2.删除 1.Insert 3.显示 一,进入
Enter your choice(1-4):4
输入您的选择(1-4):4
Insertion
插入
In the insertion operation,
temp points to the new node. If this is first node to be inserted then front will be
NULL and now both
front and
rear points to this new node. If
front is not
NULL then insertion is similar to adding the node at the end of linked list. The
next pointer of
rear points to
temp and
rear becomes
temp.
在插入操作中,
temp指向新节点。 如果这是第一个节点将被插入前,然后将
NULL现在
前后指向该新节点。 如果
front不为
NULL,则插入类似于将节点添加到链表的末尾。
后的点到
温度和
后部的
下一个指针变为
温度 。
Deletion
删除中
For deletion purpose, it is first checked whether
front is
NULL, if it is
NULL, we display the message “Queue is empty”. In case the queue is not empty, deletion is done in such a way that
temp pointer points to
front and
front pointer points to its next node. After displaying data for the node to be deleted, node is deleted by
delete(temp) function.
出于删除目的,首先检查
front是否为
NULL ,如果为
NULL ,则显示消息“ Queue is empty”。 如果队列不为空,则以
临时指针指向
前部且
前端指针指向其下一个节点的方式进行删除。 显示要删除的节点的数据后,通过
delete(temp)函数
删除该节点。
Display
显示
front is
front是否为
NULL, if it is
NULL ,如果为
NULL, we display the message “Queue is empty”. If queue is not empty,
NULL ,则显示消息“ Queue is empty”。 如果queue不为空,则将
front pointer is assigned to
前指针分配给
temp and data for all the nodes are displayed till
temp,并显示所有节点的数据,直到
temp does not become
temp不变为
NULL.
NULL为止。
If you have any doubts related to above linear queue in C++ program then you can ask it by commenting below.
如果您对C ++程序中的上述线性队列有任何疑问,可以通过在下面的注释中提出来。
翻译自: https://www.thecrazyprogrammer.com/2013/10/c-program-for-linked-list.html
栈队列线性表线性链表树