In this Django tutorial, we’ll see how we can get the data stored in the database and display it to the template.
在本Django教程中 ,我们将看到如何获取存储在数据库中的数据并将其显示在模板上。
Prerequisites
先决条件
Have a look on the previous article, in which we’ve seen how to create an app, model and create a super user to work with admin interface.
请看上一篇文章,其中我们已经了解了如何创建应用,建模和创建超级用户以使用管理界面。
https://www.thecrazyprogrammer.com/2019/01/django-models.html
https://www.thecrazyprogrammer.com/2019/01/django-models.html
So let’s start.
因此,让我们开始吧。
从PostgreSQL获取数据并将其显示为模板 (Getting Data from PostgreSQL and Showing it to Template)
1.首先上传数据 (1. Upload Data First)
We’ve already created a model Job inside jobs app, which is having two fields ImageField and CharField, ImageField for storing a picture for a particular job and TextField to store the summary about that job.
我们已经在Jobs app中创建了一个Job模型,其中有两个字段ImageField和CharField, ImageField用于存储特定作业的图片,TextField用于存储有关该作业的摘要。
Screenshot of Model Job:
模型作业的屏幕截图:
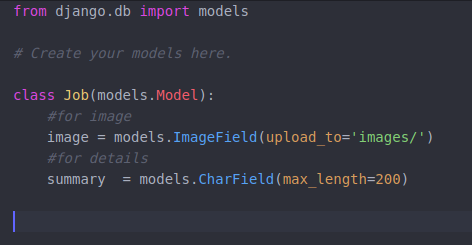
Screenshot of Admin Panel:
管理面板的屏幕截图:
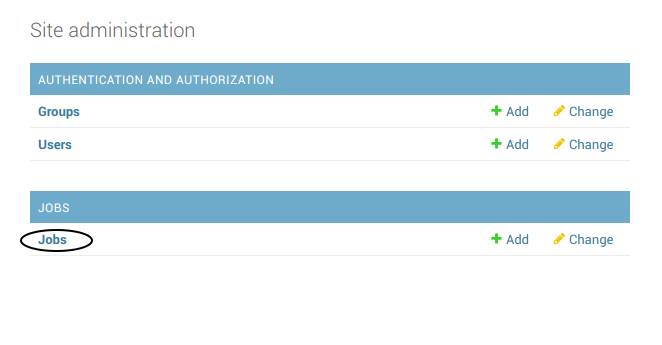
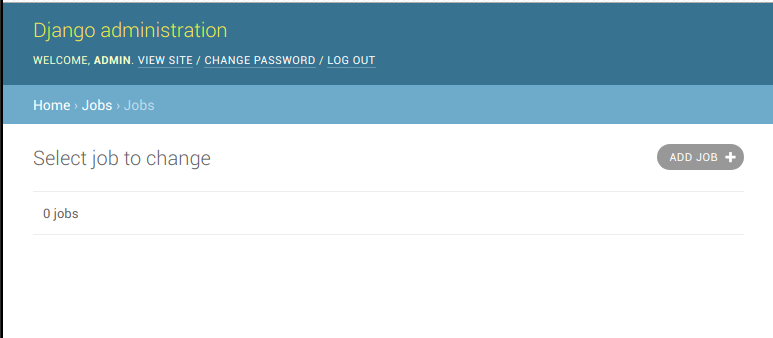
Currently we have no data inside our database. So let’s upload some data using the admin panel. (we’ll also see how to upload data using templates in later articles). So click on ADD JOB button and upload some pics and text.
目前,我们的数据库中没有数据。 因此,让我们使用管理面板上传一些数据。 (我们还将在后面的文章中看到如何使用模板上传数据)。 因此,单击“ 添加作业”按钮并上传一些图片和文字。
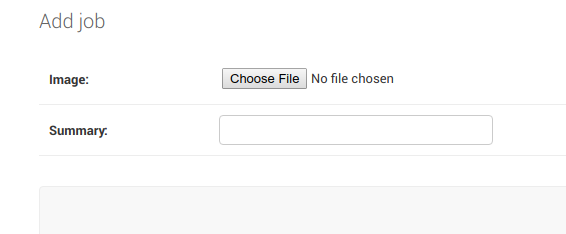
So I’ve added two Job objects. Now let’s see how to get them and print them on the template.
因此,我添加了两个Job对象。 现在,让我们看看如何获取它们并将它们打印在模板上。
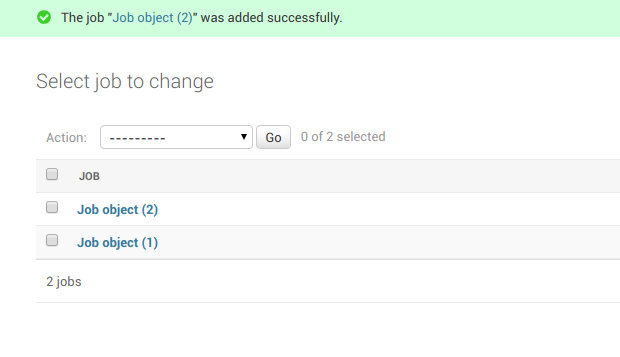
Now I am going to create a template inside the jobs App. You can also create outside the App as we have created in the previous article. But as we’re going to show jobs on that template so its a good idea to create the templates related to the jobs App inside the App.
现在,我将在Jobs App中创建一个模板。 您也可以像在上一篇文章中创建的那样在App外部创建。 但正如我们将显示该模板的作业,以便它是一个好主意,以创建与工作应用程序内的应用程序模板。
So to create a template inside app, first create a directory named as templates inside the jobs app, then create a directory with the same name as app inside templates. So our directory structure will look like this:
因此,要在应用程序内部创建模板,请首先在作业应用程序内部创建一个名为模板的目录,然后在模板内部创建与应用程序同名的目录。 因此,我们的目录结构如下所示:
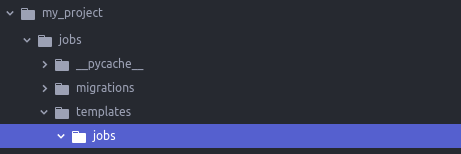
Inside this jobs folder we’ll create a HTML file named as myjobs.html.
这里面的工作文件夹,我们将创建一个名为作为myjobs.html一个HTML文件。
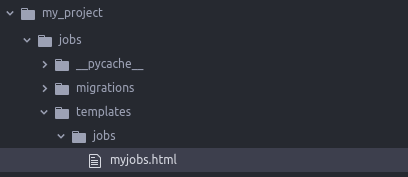
3.为模板创建URL路径 (3. Create a URL Path for Template)
To create a url path, open your urls.py file and add a path for our newly created template myjobs.html.
要创建url路径,请打开urls.py文件,并为我们新创建的模板myjobs.html添加路径。
Currently we’ve only one path in our urls.py, which we have also used to open admin panel.
目前,我们的urls.py中只有一个路径,我们也用它来打开管理面板。
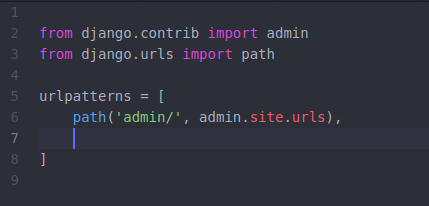
And now add this line to load images from MEDIA_ROOT:
现在添加此行以从MEDIA_ROOT加载图像:
+ static(settings.MEDIA_URL, document_root = settings.MEDIA_ROOT)
+静态(settings.MEDIA_URL,document_root = settings.MEDIA_ROOT)
To use static and settings in above line we’ve to import:
要在上面的行中使用静态和设置 ,我们必须导入:
from django.conf.urls.static import static
从django.conf.urls.static导入静态
from django.conf import settings
从django.conf导入设置
So finally our urls.py will look like:
所以最后我们的urls.py看起来像:
from django.contrib import admin
from django.urls import path
from jobs import views
from django.conf.urls.static import static
from django.conf import settings
urlpatterns = [
path('admin/', admin.site.urls),
path('myjobs/', views.showjobs),
] + static(settings.MEDIA_URL, document_root = settings.MEDIA_ROOT)
Now we’ll add one more path to navigate to myjobs template.
现在,我们将添加另一个路径以导航到myjobs模板。
urls.py:
urls.py:
from django.contrib import admin
from django.urls import path
from jobs import views
urlpatterns = [
path('admin/', admin.site.urls),
path('myjobs/', views.showjobs),
]
So I’ve added a new path for my jobs have two parameters, first is the URL to navigate to myjobs.html and second one is a functions name which will describe what to do when someone open the URL ‘domain-name.com/myjobs’, in our case it is https://localhost:8000/myjobs.
因此,我为作业添加了一个新路径,其中包含两个参数,第一个是导航至myjobs.html的URL,第二个是函数名称,该名称将描述当有人打开URL'domain-name.com/时的操作。 myjobs”,在我们的例子中是https:// localhost:8000 / myjobs 。
So now we’ve to create a function named as showjobs inside the views.py of jobs app. If you’re wondering that how we can access views.py file of jobs app in urls.py, then take a look on the third line in above code where I had imported views of jobs app.
现在,我们已经创建了一个名为作为工作的应用程序的views.py内showjobs功能。 如果您想知道我们如何访问urls.py中的jobs应用程序的views.py文件,请查看上面代码中我导入了Jobs应用程序视图的第三行。
4.创建一个函数以返回模板 (4. Create a Function to Return the Template)
Open views.py file and add a function named as showjobs.
打开views.py文件并添加一个名为showjobs的函数。
views.py:
views.py:
from django.shortcuts import render
from .models import Job
def showjobs(request):
jobs = Job.objects
return render(request, 'jobs/myjobs.html', { 'jobs':jobs } )
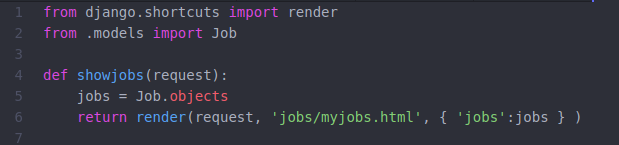
In the second line we’ve imported our model Job to get access to all the Job objects stored in our database. In Line 5, we’re getting all the objects from database and store it into the new variable called jobs. At last we’re passing that jobs variable with the same key name to the template.
在第二行中,我们导入了Job模型,以访问存储在数据库中的所有Job对象。 在第5行中,我们从数据库中获取所有对象,并将其存储到名为Jobs的新变量中。 最后,我们将具有相同键名的Jobs变量传递给模板。
5.编辑模板文件以显示作业 (5. Edit Template File to Show Jobs)
myjobs.html:
myjobs.html:
{% for job in jobs.all %}
<img src="{{ job.image.url }}">
<br>
<p > {{ job.summary }}</p>
<hr>
{% endfor %}
Now run the server and open the link below:
现在运行服务器并打开以下链接:
http://localhost:8000/myjobs/
http:// localhost:8000 / myjobs /
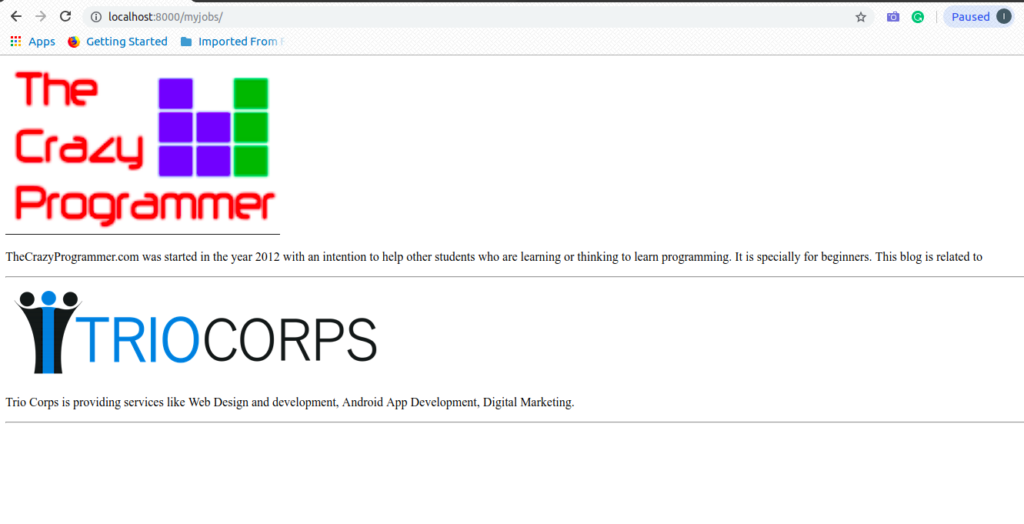
So that’s all. Finally we got our data from database and displayed it on the template.
就是这样。 最后,我们从数据库中获取数据并将其显示在模板上。
运作方式 (How it is Working)
Let’s say, a user want to access a web-page on our website, his/her request will be redirected to the urls.py. For example – user tries to access domain-name.com/myjobs/ (https://localhost:8000/myjobs) so his request will be redirected to urls.py looking for a path having ‘myjobs/’ after domain name.
假设某个用户想要访问我们网站上的网页,他/她的请求将被重定向到urls.py。 例如,–用户尝试访问domain-name.com/myjobs/(https:// localhost:8000 / myjobs),因此他的请求将被重定向到urls.py,以查找域名后具有“ myjobs /”的路径。
- In urls.py, we have a path for myjobs (line 10) 在urls.py中,我们有一个myjobs的路径(第10行)
path(‘myjobs/’, views.showjobs),
路径( 'MyJobs的/',views.showjobs),
After requested URL found, the request will be redirected to the function written along with the path. In our case it is views.showjobs.
找到请求的URL后,请求将被重定向到与路径一起编写的函数。 在我们的例子中是views.showjobs。
In views, we have a functions called showjobs
在视图中,我们有一个名为showjobs的函数

which is receiving the request and return a HTML template myjobs.html to the user along with the all the objects stored in the database.
它正在接收请求,并将HTML模板myjobs.html以及存储在数据库中的所有对象返回给用户。
So now myjobs.html will be displayed to the user.
因此,现在将myjobs.html显示给用户。
- On myjobs.html page: 在myjobs.html页面上:
on template we’ve all the objects of model Job sent from the showjobs function. So we’ll loop through all the objects using for loop and display it.
模板我们都从showjobs功能发送的模式工作的对象。 因此,我们将使用for循环遍历所有对象并显示它。
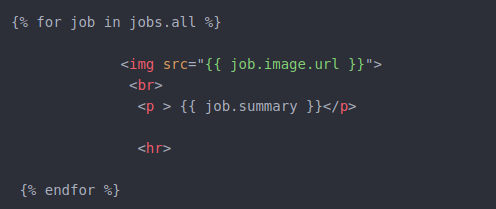
To display the image we’ve used <img> tag and to print the summary, the <p> is used.
要显示我们使用<img>标签的图像并打印摘要,请使用<p>。
So that’s all for this tutorial.
这就是本教程的全部内容。
If you’ve any confusion related with this topic, let us know in the comment box.
如果您对此主题有任何疑问,请在评论框中告诉我们。