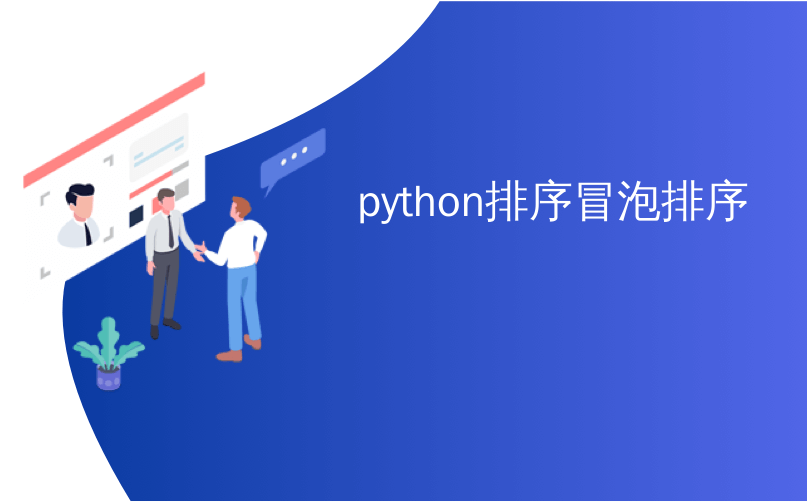
python排序冒泡排序
Here you will learn about Python bubble sort.
在这里,您将了解Python冒泡排序。
In Bubble Sort, all we have to do is pick the first two elements of the array and compare them if first one is greater than the second one then swap them. After it, pick next two elements and compare them and so on.
在冒泡排序中,我们要做的就是选择数组的前两个元素,如果第一个大于第二个,则比较它们,然后交换它们。 之后,选择下两个元素并进行比较,依此类推。
After travelling the array once, the greatest number will be placed on the last index.
在对数组进行一次遍历之后,最大的数字将放在最后一个索引上。
To sort entire array we have to travel the array (n-1) times where n is the length of the array.
为了对整个数组进行排序,我们必须遍历数组(n-1)次,其中n是数组的长度。
Python气泡排序 (Python Bubble Sort)
例 (Example)
Let’s consider we have an array:
考虑一下我们有一个数组:
21,14,18,25,9
21,14,18,25,9
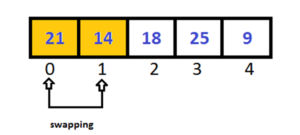
Pass-1:
通过1:
As we know 14 is less than 21 so swap them and pick next two elements.
我们知道14小于21,所以交换它们并选择下两个元素。
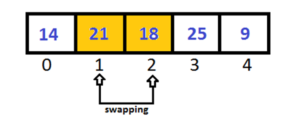
Again 18 is less than 21, so we have to swap them, and pick next two elements.
同样,18小于21,因此我们必须交换它们,并选择下两个元素。
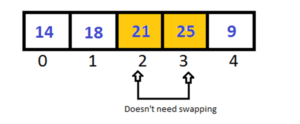
25 is greater than 21 so we doesn’t need to swap them, now pick next two elements
25大于21,所以我们不需要交换它们,现在选择下两个元素
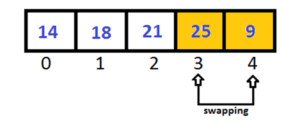
9 is less than 25, swap them.
9小于25,交换它们。
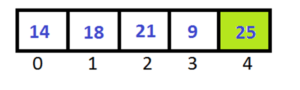
So here we have been traveling array once and the largest number is placed at last index.
因此,在这里,我们已经进行了一次遍历数组,并且将最大数目放置在最后一个索引处。
As we mentioned above that to sort the entire array we have to travel array (n-1) times, where n is the length of array.
正如我们上面提到的,要对整个数组进行排序,我们必须经过数组(n-1)次,其中n是数组的长度。
So we name it Pass-1.
因此,我们将其命名为Pass-1。
Let’s start Pass-2.
让我们开始Pass-2。
Pass-2:
第二阶段:
This time we doesn’t need to check the last index, because it is sorted after Pass-1.
这次我们不需要检查最后一个索引,因为它在Pass-1之后排序。
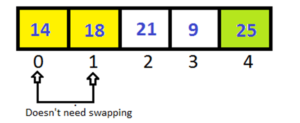
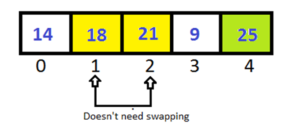
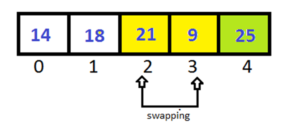
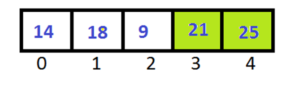
After completing Pass-2 the last two elements have been sorted. So in Pass-3 we don’t have to check them.
完成Pass-2之后,已对最后两个元素进行了排序。 因此,在Pass-3中,我们不必检查它们。
Pass-3:
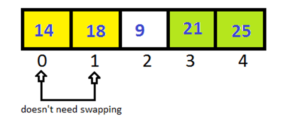
第三阶段:
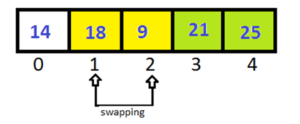
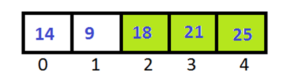
After completing Pass-3 , the last 3 elements of array are in sorted order.
完成Pass-3之后,数组的最后3个元素按排序顺序。
Pass-4:
第四阶段:
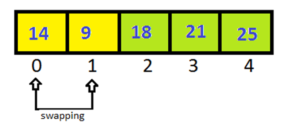
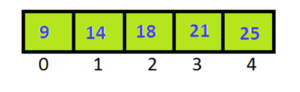
We have been traveled array (n-1) times. Array has been sorted.
我们已经旅行过阵列(n-1)次。 数组已排序。
“As after every step the largest element tend to move up into the correct order like bubbles rising to the surface, that’s why it is called as BUBBLE SORT.”
“ 随着每一步之后,最大的元素倾向于向上移动到正确的顺序,例如气泡上升到表面,这就是为什么它被称为气泡排序的原因。”
算法 (Algorithm)
we have an array Arr[n], where n is number of elements.
我们有一个数组Arr [n],其中n是元素数。
Step:1- set pass =1
步骤:1-设置通过次数= 1
Step:2- set index = 0
步骤:2-设置索引= 0
Step:3- if Arr[index]>Arr[index+1]
步骤3:如果Arr [index]> Arr [index + 1]
Then swap Arr[index] with Arr[index+1]
然后将Arr [index]与Arr [index + 1]交换
Increase index by 1.
将索引增加1。
Step:4- Go to Step:3 till index<=n-pass-1
步骤:4-转到步骤:3,直到index <= n-pass-1
[pass will prevent checking of the sorted element again]
[通过将阻止再次检查排序的元素]
Step:5- increase pass by 1 and go to Step:2 till pass<=n-1
步骤5:将通行证增加1,然后转到步骤2:直到通行证<= n-1
Python中的冒泡排序程序 (Program for Bubble Sort in Python)
Arr = [21,14,18,25,9]
n = len(Arr) #length
Pass = 1
while(Pass<=n-1):
index = 0
while(index<=n-Pass-1):
if Arr[index]>Arr[index+1]:
Arr[index],Arr[index+1] = Arr[index+1],Arr[index] #swapping
index = index+1
Pass =Pass + 1
for item in Arr:
print item,
Output
输出量
9 14 18 21 25
9 14 18 21 25
翻译自: https://www.thecrazyprogrammer.com/2017/12/python-bubble-sort.html
python排序冒泡排序