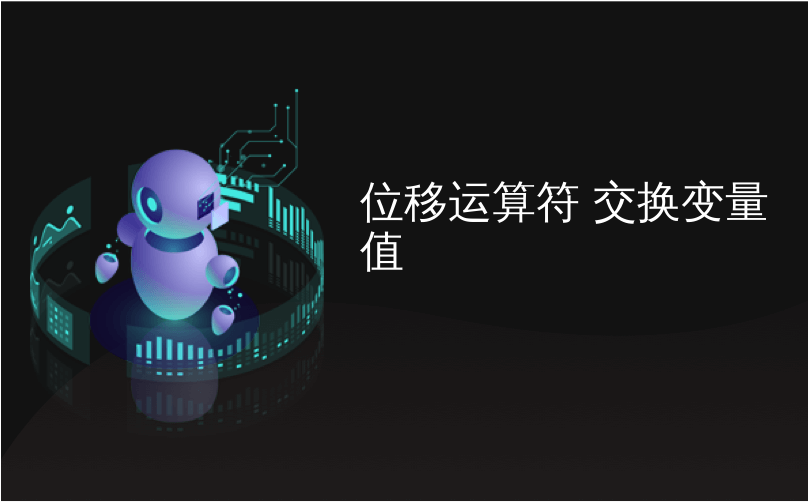
位移运算符 交换变量值
You have done swapping
of two numbers using temporary variable or by using arithmetic operators. It
can be done in following way.
of two numbers using temporary variable or by using arithmetic operators. It
can be done in following way.
Lets take two numbers
a=5 and b=7.
a=5 and b=7.
Using Temporary Variable
temp=a; //temp becomes 5
a=b; //a becomes 7
b=temp; //b becomes 5
Also Read: C++ program to swap two numbers using class
Using Arithmetic Operators
a=a+b; //a becomes 12
b=a-b; //b becomes 5
a=a-b; //a becomes 7
or
a=a*b; //a becomes 35
b=a/b; //b becomes 5
a=a/b; //a becomes 7
In this article I am
sharing another method, may be you are already familiar with it. Swapping of
two numbers can also be done by using bitwise XOR operator i.e. ^. The XOR of two numbers a and b returns a number which has all the bits as 1 wherever bits of a and b differ. If bits are same then resultant bit will be 0.
sharing another method, may be you are already familiar with it. Swapping of
two numbers can also be done by using bitwise XOR operator i.e. ^. The XOR of two numbers a and b returns a number which has all the bits as 1 wherever bits of a and b differ. If bits are same then resultant bit will be 0.
For example binary of 5
is 0101 and 7 is 0111. If you do XOR of 5 and 7 then result will be 0010. A c program
is given below that uses this concept to swap values of two numbers.
is 0101 and 7 is 0111. If you do XOR of 5 and 7 then result will be 0010. A c program
is given below that uses this concept to swap values of two numbers.
Also Read: C++ program to swap two numbers using macros
#include <stdio.h>
int main()
{
int
a=5,b=7;
a=5,b=7;
a=a^b; // a becomes 2 (0010)
b=a^b; // b becomes 5 (0101)
a=a^b; // a becomes 7 (0111)
printf(“After Swapping: a=%d,b=%d”,a,b);
return 0;
}
If you have any doubts
or know any other method to swap values of two numbers then mention it in
comment section.
or know any other method to swap values of two numbers then mention it in
comment section.
Happy Coding!! 🙂 🙂
You have done swapping
of two numbers using temporary variable or by using arithmetic operators . It
can be done in following way.
of two numbers using temporary variable or by using arithmetic operators . It
can be done in following way.
Lets take two numbers
a=5 and b=7.
a=5 and b=7.
Using Temporary Variable
temp=a; //temp becomes 5
a=b; //a becomes 7
b=temp; //b becomes 5
Also Read: C++ program to swap two numbers using class
Using Arithmetic Operators
a=a+b; //a becomes 12
b=a-b; //b becomes 5
a=a-b; //a becomes 7
or
a=a*b; //a becomes 35
b=a/b; //b becomes 5
a=a/b; //a becomes 7
In this article I am
sharing another method, may be you are already familiar with it. Swapping of
two numbers can also be done by using bitwise XOR operator i.e. ^ . The XOR of two numbers a and b returns a number which has all the bits as 1 wherever bits of a and b differ. If bits are same then resultant bit will be 0.
sharing another method, may be you are already familiar with it. Swapping of
two numbers can also be done by using bitwise XOR operator i.e. ^ . The XOR of two numbers a and b returns a number which has all the bits as 1 wherever bits of a and b differ. If bits are same then resultant bit will be 0.
For example binary of 5
is 0101 and 7 is 0111. If you do XOR of 5 and 7 then result will be 0010. A c program
is given below that uses this concept to swap values of two numbers.
is 0101 and 7 is 0111. If you do XOR of 5 and 7 then result will be 0010. A c program
is given below that uses this concept to swap values of two numbers.
Also Read: C++ program to swap two numbers using macros
#include <stdio.h>
int main()
{
int
a=5,b=7;
a=5,b=7;
a=a^b; // a becomes 2 (0010)
b=a^b; // b becomes 5 (0101)
a=a^b; // a becomes 7 (0111)
printf(“After Swapping: a=%d,b=%d”,a,b);
return 0;
}
If you have any doubts
or know any other method to swap values of two numbers then mention it in
comment section.
or know any other method to swap values of two numbers then mention it in
comment section.
Happy Coding!! 🙂 🙂
位移运算符 交换变量值