ios pusher使用
When building web applications, it is not uncommon to find some sort of in-app notification system that will notify you instantly when someone carries out an action related to you or your account. On Facebook, you will be notified when someone likes your status, or when someone comments on your profile. We will replicate this feature by creating a web notifications system using Laravel and Pusher.
在构建Web应用程序时,通常会找到某种应用程序内通知系统,当有人执行与您或您的帐户相关的操作时,该系统会立即通知您。 在Facebook上,当有人喜欢您的身份或有人在您的个人资料中发表评论时,您会收到通知。 我们将通过使用Laravel和Pusher创建一个Web通知系统来复制此功能。
To follow this tutorial, you need to have PHP and Laravel installed on your machine.
要遵循本教程,您需要在计算机上安装PHP和Laravel。
我们将要建立的 ( What we would be building )
After this tutorial we would demonstrate how we can have a small web application show notifications using Laravel and Pusher. It would be similar to how websites like Facebook show notifications. Here is a preview of what we would be building:
在学习完本教程之后,我们将演示如何使用Laravel和Pusher使小型Web应用程序显示通知。 这类似于Facebook之类的网站显示通知的方式。 这是我们将要构建的预览:
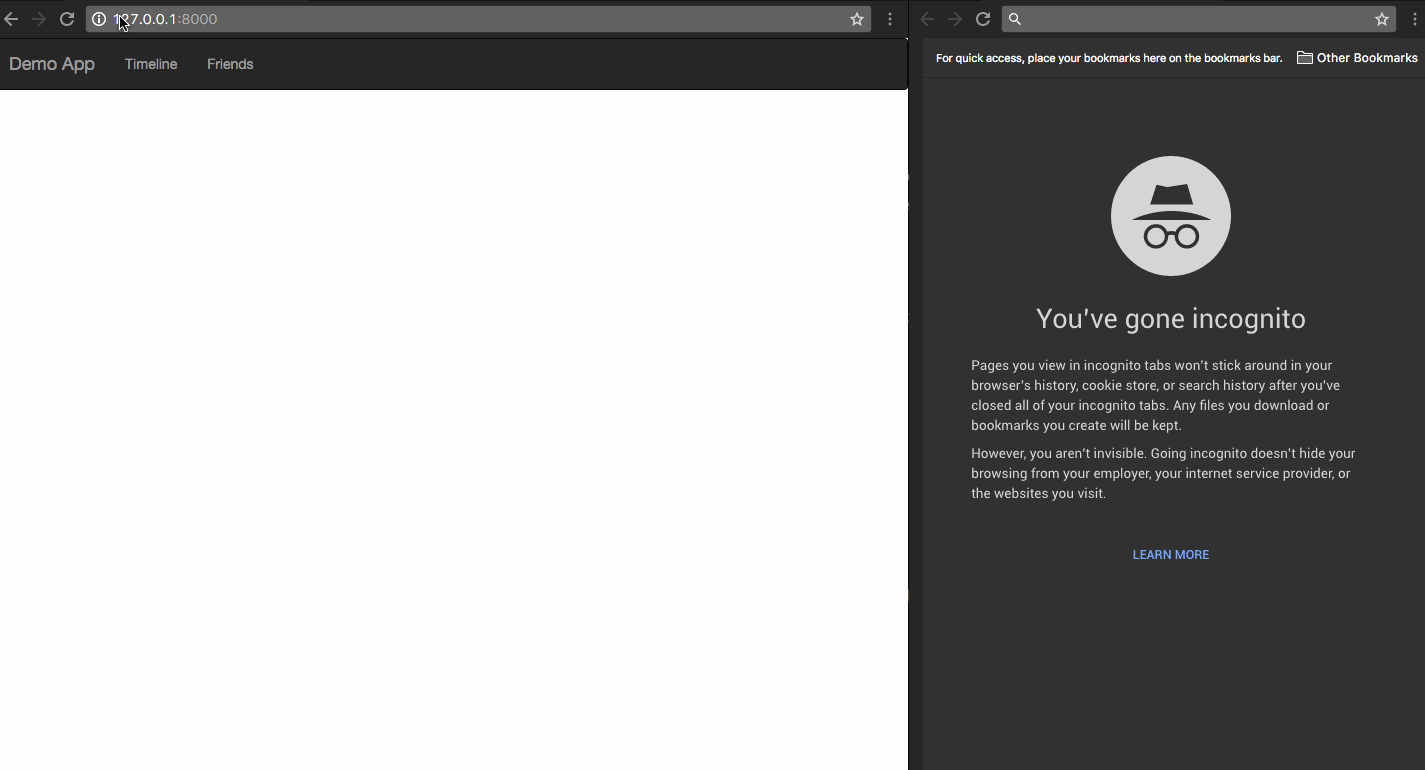
设置您的Pusher应用程序 ( Setting up your Pusher application )
Create a Pusher account, if you have not already, and then set up your application as seen in the screenshot below.
创建Pusher帐户 (如果尚未创建),然后按照下面的屏幕快照所示设置应用程序。
设置您的Laravel应用程序 ( Setting up your Laravel application )
You can create a new Laravel application by running the command below in your terminal:
您可以通过在终端中运行以下命令来创建新的Laravel应用程序:
laravel new laravel-web-notifications
After that, we will need to install the Pusher PHP SDK, you can do this using Composer by running the command below:
之后,我们将需要安装Pusher PHP SDK,您可以通过运行以下命令使用Composer进行此操作:
composer require pusher/pusher-php-server
When Composer is done, we will need to configure Laravel to use Pusher as its broadcast driver, to do this, open the .env
file that is in the root directory of your Laravel installation. Update the values to correspond with the configuration below:
完成Composer后,我们需要将Laravel配置为使用Pusher作为其广播驱动程序,为此,请打开Laravel安装根目录中的.env
文件。 更新值以符合以下配置:
PUSHER_APP_ID=322700
BROADCAST_DRIVER=pusher
// Get the credentials from your pusher dashboard
PUSHER_APP_ID=XXXXX
PUSHER_APP_KEY=XXXXXXX
PUSHER_APP_SECRET=XXXXXXX
Important Note: If you’re using the EU or AP Cluster, make sure to update the options array in your
config/broadcasting.php
config since Laravel defaults to using the US Server. You can use all the options the Pusher PHP Library supports.重要说明:如果您使用的是EU或AP群集,请确保更新
config/broadcasting.php
配置中的options数组,因为Laravel默认使用US Server。 您可以使用Pusher PHP库支持的所有选项。
Open config/app.php
and uncomment the App\Providers\BroadcastServiceProvider::class
.
打开config/app.php
并取消注释App\Providers\BroadcastServiceProvider::class
。
创建我们的Laravel和Pusher应用程序 ( Creating our Laravel and Pusher application )
Now that we are done with configuration, let us create our application. First we would create an Event
class that would broadcast to Pusher from our Laravel application. Events can be fired from anywhere in the application.
现在我们已经完成了配置,让我们创建应用程序。 首先,我们将创建一个Event
类,该类将从Laravel应用程序广播到Pusher。 可以从应用程序中的任何位置触发事件。
php artisan make:event StatusLiked
This will create a new StatusLiked
class in the app/Events
directory. Open the contents of the file and update to the following below:
这将在app/Events
目录中创建一个新的StatusLiked
类。 打开文件的内容并更新为以下内容:
<?php
namespace App\Events;
use Illuminate\Queue\SerializesModels;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class StatusLiked implements ShouldBroadcast
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $username;
public $message;
/**
* Create a new event instance.
*
* @return void
*/
public function __construct($username)
{
$this->username = $username;
$this->message = "{$username} liked your status";
}
/**
* Get the channels the event should broadcast on.
*
* @return Channel|array
*/
public function broadcastOn()
{
return ['status-liked'];
}
}
Above, we have implemented the ShouldBroadcast
interface and this tells Laravel that this event should be broadcasted using whatever driver we have set in the configuration file.
上面,我们实现了ShouldBroadcast
接口,这告诉Laravel该事件应该使用我们在配置文件中设置的任何驱动程序进行广播。
We also have a constructor that accepts two parameters, username and verb. We will get back to this later on. We assigned these variables to class properties named the same way. It is important to set the visibility of the properties to public; if you don't, the property will be ignored.
我们还有一个接受两个参数的构造函数,用户名和动词。 稍后我们将回到这一点。 我们以相同的方式将这些变量分配给类属性。 重要的是将属性公开可见; 如果您不这样做,该属性将被忽略。
Lastly, we set the channel name to broadcast on.
最后,我们设置要播放的频道名称。
创建应用程序视图 ( Creating the application views )
We will keep it simple and create a single view where you can see a navigation bar with a notification icon. The icon will be updated when new notifications are available without the need to refresh the page. The notifications are ephemeral in this tutorial by design; you can extend the functionality and make it last longer after the page reloads if you so desire.
我们将使其保持简单,并创建一个视图,您可以在其中查看带有通知图标的导航栏。 当有新的通知可用而无需刷新页面时,图标将更新。 在本教程中,通知是短暂的,是设计使然的; 您可以根据需要扩展功能并使其在页面重新加载后持续更长的时间。
Open the welcome.blade.php
file and replace it with the HTML below.
打开welcome.blade.php
文件,并将其替换为下面HTML。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Demo Application</title>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous">
<link rel="stylesheet" type="text/css" href="/css/bootstrap-notifications.min.css">
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-9" aria-expanded="false">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="#">Demo App</a>
</div>
<div class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li class="dropdown dropdown-notifications">
<a href="#notifications-panel" class="dropdown-toggle" data-toggle="dropdown">
<i data-count="0" class="glyphicon glyphicon-bell notification-icon"></i>
</a>
<div class="dropdown-container">
<div class="dropdown-toolbar">
<div class="dropdown-toolbar-actions">
<a href="#">Mark all as read</a>
</div>
<h3 class="dropdown-toolbar-title">Notifications (<span class="notif-count">0</span>)</h3>
</div>
<ul class="dropdown-menu">
</ul>
<div class="dropdown-footer text-center">
<a href="#">View All</a>
</div>
</div>
</li>
<li><a href="#">Timeline</a></li>
<li><a href="#">Friends</a></li>
</ul>
</div>
</div>
</nav>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.4/jquery.min.js"></script>
<script src="//js.pusher.com/3.1/pusher.min.js"></script>
<script src="//maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="sha384-Tc5IQib027qvyjSMfHjOMaLkfuWVxZxUPnCJA7l2mCWNIpG9mGCD8wGNIcPD7Txa" crossorigin="anonymous"></script>
<script type="text/javascript">
var notificationsWrapper = $('.dropdown-notifications');
var notificationsToggle = notificationsWrapper.find('a[data-toggle]');
var notificationsCountElem = notificationsToggle.find('i[data-count]');
var notificationsCount = parseInt(notificationsCountElem.data('count'));
var notifications = notificationsWrapper.find('ul.dropdown-menu');
if (notificationsCount <= 0) {
notificationsWrapper.hide();
}
// Enable pusher logging - don't include this in production
// Pusher.logToConsole = true;
var pusher = new Pusher('API_KEY_HERE', {
encrypted: true
});
// Subscribe to the channel we specified in our Laravel Event
var channel = pusher.subscribe('status-liked');
// Bind a function to a Event (the full Laravel class)
channel.bind('App\\Events\\StatusLiked', function(data) {
var existingNotifications = notifications.html();
var avatar = Math.floor(Math.random() * (71 - 20 + 1)) + 20;
var newNotificationHtml = `
<li class="notification active">
<div class="media">
<div class="media-left">
<div class="media-object">
<img src="https://api.adorable.io/avatars/71/`+avatar+`.png" class="img-circle" alt="50x50" style="width: 50px; height: 50px;">
</div>
</div>
<div class="media-body">
<strong class="notification-title">`+data.message+`</strong>
<!--p class="notification-desc">Extra description can go here</p-->
<div class="notification-meta">
<small class="timestamp">about a minute ago</small>
</div>
</div>
</div>
</li>
`;
notifications.html(newNotificationHtml + existingNotifications);
notificationsCount += 1;
notificationsCountElem.attr('data-count', notificationsCount);
notificationsWrapper.find('.notif-count').text(notificationsCount);
notificationsWrapper.show();
});
</script>
</body>
</html>
This is mostly a lot of Bootstrap noise so we will isolate the important parts, mostly Javascript. We include the Pusher javascript library, and then we added the javascript block that does the magic. Let us look at some snippets of the javascript block:
这主要是很多Bootstrap噪音,因此我们将隔离重要部分,主要是Javascript。 我们包括Pusher javascript库 ,然后添加了执行魔术的javascript块。 让我们看一下javascript块的一些片段:
// Enable pusher logging - don't include this in production
// Pusher.logToConsole = true;
// Initiate the Pusher JS library
var pusher = new Pusher('API_KEY_HERE', {
encrypted: true
});
// Subscribe to the channel we specified in our Laravel Event
var channel = pusher.subscribe('status-liked');
// Bind a function to a Event (the full Laravel class)
channel.bind('App\\Events\\StatusLiked', function(data) {
// this is called when the event notification is received...
});
PROTIP: By default, Laravel will broadcast the event using the event's class name. However, you may customize the broadcast name by defining a broadcast as method on the event: public function broadcastAs() { return 'event-name'; }
提示 :默认情况下,Laravel将使用事件的类名广播事件。 但是,您可以通过将广播定义为事件的方法来自定义广播名称:public function broadcastAs(){return'event-name'; }
The code above just initializes the Pusher JS library and subscribes to a channel. It then sets a callback to call when the event broadcasted is received on that channel.
上面的代码只是初始化Pusher JS库并订阅频道。 然后,当在该频道上接收到广播的事件时,它将设置一个回调以进行调用。
测试我们的设置 ( Testing our set up )
Finally to test our set up, we will create a route that manually calls the event. If everything works as expected, we will get a new notification anytime we hit the route. Let's add the new route:
最后,为了测试设置,我们将创建一条手动调用该事件的路由。 如果一切正常,我们在上路线时会收到新的通知。 让我们添加新路线:
Route::get('test', function () {
event(new App\Events\StatusLiked('Someone'));
return "Event has been sent!";
});
Now we can start a PHP server using Laravel so we can test our code to see if it works.
现在,我们可以使用Laravel启动PHP服务器,以便我们测试代码以查看其是否有效。
$ php artisan serve
结论 ( Conclusion )
In this article we have been able to leverage the power of Pusher to create a modern web notifications system and it was very easy. This is just scratching the surface of what can be really done using Pusher. The example was just to show you the possibilities.
在本文中,我们已经能够利用Pusher的功能来创建现代的Web通知系统,这非常容易。 这只是使用Pusher可以真正完成的工作的开始。 该示例只是为了向您展示可能性。
The code is available on GitHub, you can star, fork and play around with it.
该代码可在GitHub上获得 ,您可以对其进行加注 ,标记和操作。
翻译自: https://scotch.io/tutorials/create-web-notifications-using-laravel-and-pusher-channels
ios pusher使用