phantomjs 使用
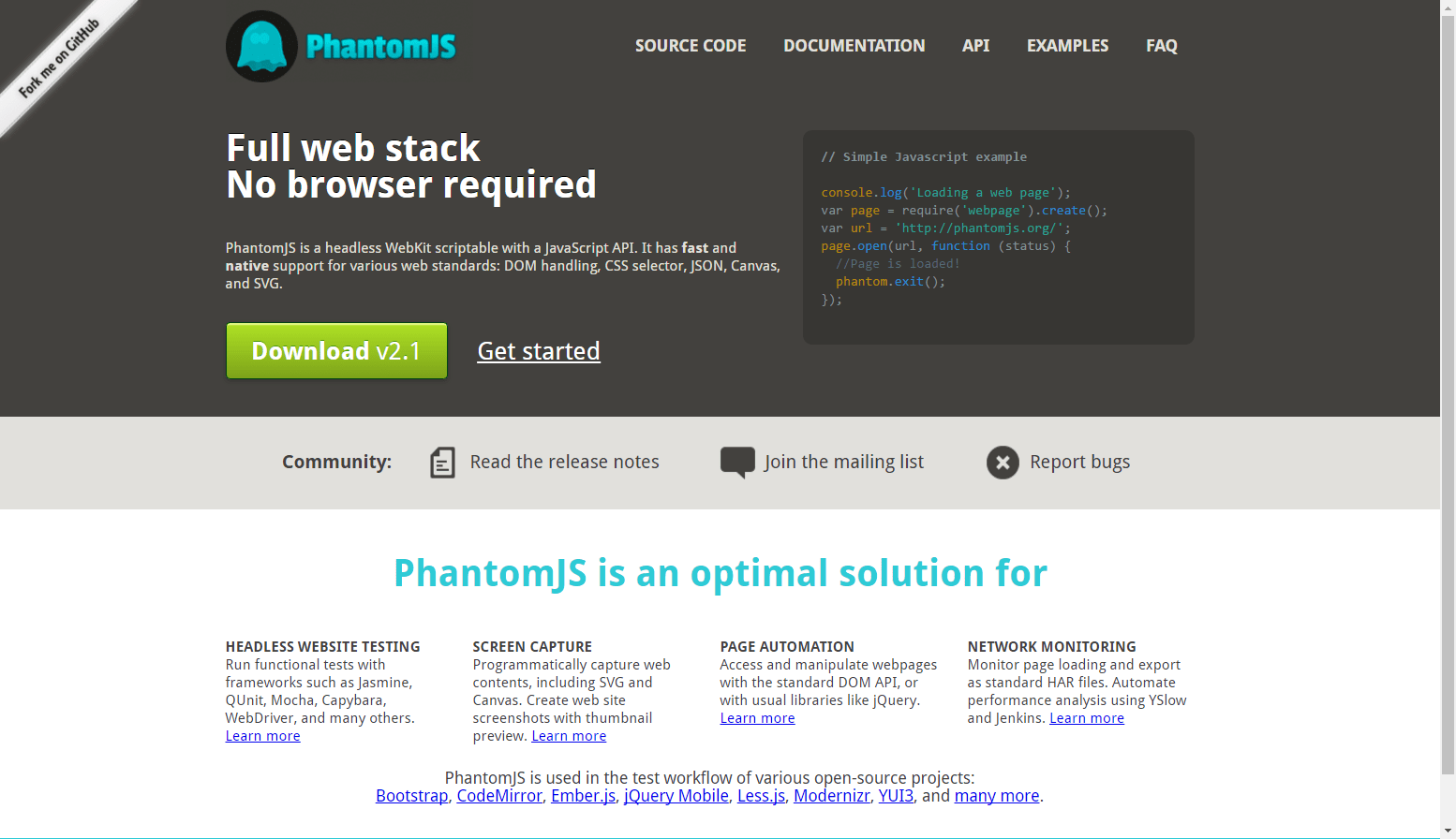
PhantomJS is a headless WebKit scriptable with a JavaScript API. It has fast and native support for various web standards: DOM handling, CSS selector, JSON, Canvas, and SVG.
PhantomJS是可使用JavaScript API编写脚本的无头WebKit。 它具有对各种Web标准的快速本机支持:DOM处理,CSS选择器,JSON,Canvas和SVG。
The above definition may be ambiguous, in simple terms, PhantomJS is a web browser without a graphical user interface.
上面的定义可能是模棱两可的,简单来说,PhantomJS是没有图形用户界面的Web浏览器。
In simple terms, PhantomJS is a web browser without a graphical user interface
简单来说,PhantomJS是一种没有图形用户界面的网络浏览器
This then begs the question, What use is a browser without a GUI? A web browser without a graphical user interface is pretty much useless. But because PhantomJS provides a Javascript API, this makes the browser useful. Hence the phrase "WebKit scriptable".
这就引出了一个问题, 没有GUI的浏览器有什么用? 没有图形用户界面的Web浏览器几乎没有用。 但是因为PhantomJS提供了Javascript API,所以这使浏览器很有用。 因此,短语“ WebKit可编写脚本”。
安装PhantomJS ( Installing PhantomJS )
Before we learn more about PhantomJS, first you need to install it on your computer. To install PhantomJS, head over to the official website and download the binary suitable for your device.
在我们了解有关PhantomJS的更多信息之前,首先需要在计算机上安装它。 要安装PhantomJS,请访问官方网站并下载适合您设备的二进制文件。
After downloading the binary, you need to add the executable to PATH
environment variable. For Linux users (Mac included), you can place the downloaded binary in usr/bin
directory. While Windows users can place the executable in C:\Windows
. After doing that, you should be able to open a command prompt or terminal and type phantomjs --help
and see a screen like this.
下载二进制文件后,需要将可执行文件添加到PATH
环境变量中 。 对于Linux用户(包括Mac),您可以将下载的二进制文件放在usr/bin
目录中。 Windows用户可以将可执行文件放置在C:\Windows
。 之后,您应该能够打开命令提示符或终端,然后键入phantomjs --help
并看到类似这样的屏幕。
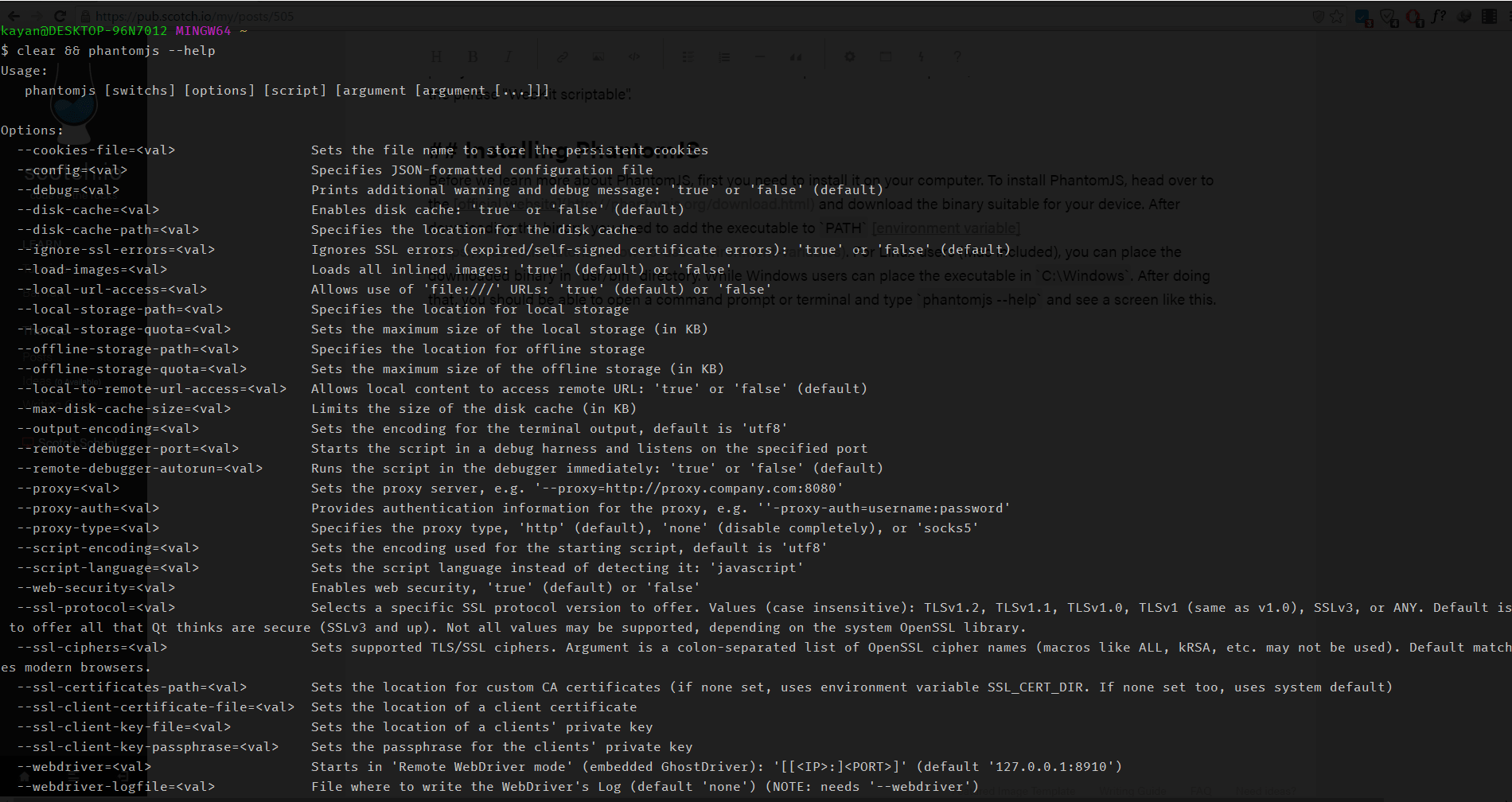
PhantomJS核心概念 ( PhantomJS Core Concepts )
Since PhantomJS is not usable when it comes to surfing the web, it has a whole set of features that developers love and use for many purposes.
由于PhantomJS在浏览网络时不可用,因此它具有一整套功能,开发人员喜欢并将其用于许多目的。
- Screen capture 屏幕截图
- Page automation 页面自动化
- Network monitoring 网络监控
- Testing 测试中
- And more... 和更多...
屏幕截图 (Screen Capture)
PhantomJS can be used to take screenshots of websites, those screenshots can be rendered in different formats also. Let's spin up a basic javascript script that takes screenshots of a website.
PhantomJS可用于拍摄网站的屏幕快照,这些屏幕快照也可以以不同的格式呈现。 让我们启动一个基本JavaScript脚本,该脚本获取网站的屏幕截图。
var webpage = require('webpage').create();
webpage.open('https://scotch.io/', function() {
webpage.render('scotch.png');
phantom.exit();
});
Running this snippet from a web-browser won't work, we need to load this script using PhantomJS. So we save this snippet in a file screenshot.js
, can be anything you want to name it. Then from the command line, run.
无法从网络浏览器运行此代码段,我们需要使用PhantomJS加载此脚本。 因此,我们将此片段保存在文件screenshot.js
,可以是您要命名的任何内容。 然后从命令行运行。
phantomjs screenshot.js
Give it a few seconds to run and you should see a file in the same path as screenshot.js
named scotch.png
, open it and you should see a full page screenshot of scotch.io.
给它几秒钟的运行时间,您应该看到一个与screenshot.js
相同的路径名为scotch.png
,打开它,您应该看到scotch.io的整个屏幕截图。
页面自动化 (Page Automation)
Because we can use PhantomJS to load and manipulate a web page, it is perfect for carrying out page automation. This helps developers run a bunch of tests without ever having to open a web browser.
因为我们可以使用PhantomJS加载和操作网页,所以它是执行页面自动化的理想选择。 这可以帮助开发人员无需打开Web浏览器即可进行大量测试。
Although this may not seem important, this allows us to automate any sort of interactions with a web page without having to open a browser (an operation that will save you a tremendous amount of time).
尽管这似乎并不重要,但是这使我们能够自动进行与网页的任何形式的交互,而无需打开浏览器(该操作将为您节省大量时间)。
var webpage = require('webpage').create();
// open scotch.io
webpage.open('https://scotch.io', function(status) {
if (status !== 'success') {
console.log('Unable to access network');
} else {
var title = webpage.evaluate(function() {
return document.title;
});
// log the title
console.log(title === 'Scotch | Developers bringing fire to the people.');
}
phantom.exit();
});
In the evaluate()
method, that's where we write the javascript we want to run on the loaded page. We can save the snippet above in a file and run phantomjs <filename>.js
.
在evaluate()
方法中,我们在此处编写了要在加载的页面上运行的javascript。 我们可以将上面的代码片段保存在文件中,然后运行phantomjs <filename>.js
。
网络监控 (Network Monitoring)
Because PhantomJS permits the inspection of network traffic, it is suitable to build various analysis on the network behavior and performance.
由于PhantomJS允许检查网络流量,因此适合对网络行为和性能进行各种分析。
We can hook into PhantomJS during a request-response cycle and collect data about the website. This data can be reformatted and allows us to check the performance of a web page.
我们可以在请求-响应周期中加入PhantomJS并收集有关网站的数据。 可以重新格式化此数据,并允许我们检查网页的性能。
var page = require('webpage').create();
// hook into initial request
page.onResourceRequested = function(request) {
console.log('Request ' + JSON.stringify(request, undefined, 4));
};
// hook to response
page.onResourceReceived = function(response) {
console.log('Receive ' + JSON.stringify(response, undefined, 4));
};
page.open(url);
We can use tools like confess.js (a PhantomJS script) and YSlow for a more in-depth network analysis. This is useful in the sense that we can detect regression in the performance of our website before pushing the code.
我们可以使用诸如confess.js (PhantomJS脚本)和YSlow之类的工具来进行更深入的网络分析。 从某种意义上说,这很有用,因为我们可以在推送代码之前检测到网站性能的下降。
测试中 (Testing)
This is an important aspect of software development, but developers rarely talk about. PhantomJS has been made popular as a tool for running unit tests. It can run a lot of tests and show the user the result in the command line. Testing tools like Mocha, Casper, just to mention but a few are good examples of testing with PhantomJS as these tools are based on it.
这是软件开发的重要方面,但是开发人员很少谈论。 PhantomJS已作为运行单元测试的工具而流行。 它可以运行许多测试,并在命令行中向用户显示结果。 仅需提及Mocha , Casper等测试工具,但其中一些是使用PhantomJS进行测试的很好的示例,因为这些工具都是基于PhantomJS的。
PhantomJS在野外 ( PhantomJS in the Wild )
PhantomJS is used by many companies, you may have used the product and wondered how it was built. For example, Media Queries (source of inspiration for responsive websites) takes a link, checks if the website is responsive, and shows a preview of the website using different screen sizes. The site was made possible thanks to PhantomJS.
PhantomJS已被许多公司使用,您可能已经使用过该产品,并且想知道它是如何构建的。 例如,“ 媒体查询” (响应式网站的灵感来源)获取一个链接,检查网站是否响应式,并使用不同的屏幕尺寸显示网站的预览。 多亏了PhantomJS,该网站才得以实现。
A Spanish Life uses PhantomJS to create ads based on user content. Check Out more examples of PhantomJS.
西班牙人生活使用PhantomJS根据用户内容创建广告。 查看更多PhantomJS示例。
Twitter uses PhantomJS to run QUnit tests on their website.
Twitter使用PhantomJS在其网站上运行QUnit测试。
结论 ( Conclusion )
This article is just an introduction to PhantomJS, in follow-up articles, we will build a screenshot taking application with PhantomJS etc. Stay tuned.
本文只是对PhantomJS的介绍,在后续文章中,我们将使用PhantomJS等构建截屏应用程序。敬请期待。
翻译自: https://scotch.io/tutorials/what-is-phantomjs-and-how-is-it-used
phantomjs 使用