Today we'll talk about how to use one of Laravel's lesser-known features to quickly read data from our Laravel applications. We can use Laravel artisan's built-in php artisan tinker
to mess around with your application and things in the database.
今天,我们将讨论如何使用Laravel鲜为人知的功能之一从我们的Laravel应用程序快速读取数据。 我们可以使用Laravel artisan内置的php artisan tinker
来摆弄您的应用程序和数据库中的内容。
Laravel artisan's tinker is a repl (read-eval-print loop). A repl translates to read-eval-print-loop
, and it is an interactive language shell. It takes in a single user input, evaluates it, and returns the result to the user.
Laravel工匠的修补匠是repl(读取-评估-打印循环) 。 一个repl转换为read-eval-print-loop
,它是一个交互式语言外壳。 它接受单个用户输入,对其进行评估,然后将结果返回给用户。
A quick and easy way to see the data in your database.
一种快速简便的方法来查看数据库中的数据。
Wouldn't it be nice to see the immediate output of commands like:
看到以下命令的立即输出不是很好:
// see the count of all users
App\User::count();
// find a specific user and see their attributes
App\User::where('username', 'samuel')->first();
// find the relationships of a user
$user = App\User::with('posts')->first();
$user->posts;
With php artisan tinker
, we can do the above pretty quickly. Tinker is Laravel's own repl, based on PsySH. It allows us to interact with our applications and stop dd()
ing and die()
ing all the time. A lot of us know the insanity that ensues when there are print_r()
s and dd()
s all over our code.
使用php artisan tinker
,我们可以很快完成上述操作。 Tinker是Laravel自己的代表,基于PsySH 。 它允许我们与应用程序进行交互,并始终停止dd()
和die()
。 我们很多人都知道,在我们的代码中到处都有print_r()
和dd()
时,会发生疯狂。
Before we tinker with our application, let us create a demo project. Let's call it ScotchTest. If you have the laravel installer installed on your computer, run this command.
在修改应用程序之前,让我们创建一个演示项目。 我们称之为ScotchTest 。 如果您的计算机上安装了laravel安装程序,请运行此命令。
laravel new ScotchTest
For those without the Laravel installer on their computer, you can still use composer
to create a new Laravel project.
对于计算机上没有Laravel安装程序的用户,您仍然可以使用composer
创建一个新的Laravel项目。
composer create-project laravel/laravel ScotchTest --prefer-dist
数据库设置:运行迁移 ( Database Setup: Running Migrations )
After installing our demo Laravel project, we need to create a database and setup migrations. For this article we will be using the default Laravel migrations. So we configure our .env
file to point to the database you created for this test. The default migrations include creating a users
table and a password_resets
table.
在安装演示Laravel项目之后,我们需要创建一个数据库并设置迁移。 对于本文,我们将使用默认的Laravel迁移。 因此,我们将.env
文件配置为指向您为此测试创建的数据库。 默认迁移包括创建users
表和password_resets
表。
From the root of the project, run
从项目的根目录运行
php artisan migrate
After migrating our database, we should see something similar to
迁移数据库后,我们应该看到类似于
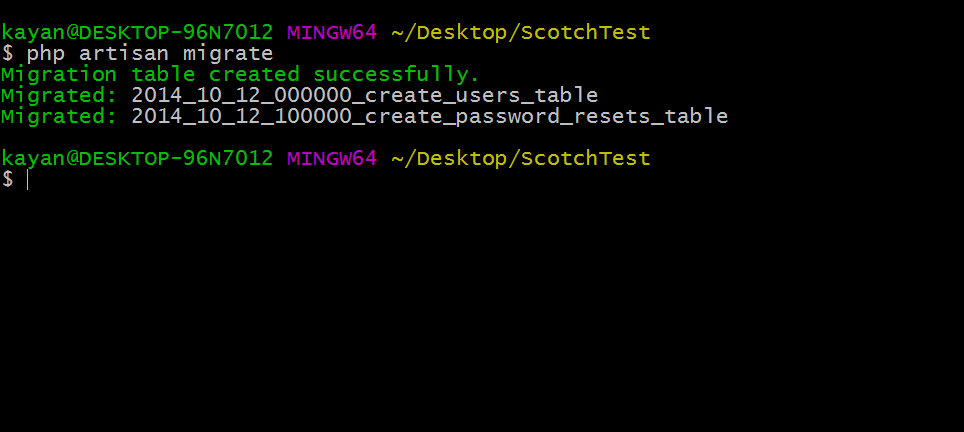
播种我们的数据库 ( Seeding our Database )
By default, Laravel provides a model factory that we can use to seed our database. Now lets begin to tinker with our application.
默认情况下,Laravel提供了一个模型工厂 ,我们可以使用它来播种数据库。 现在开始修改我们的应用程序。
From the root of the Laravel project, run the following command.
从Laravel项目的根目录,运行以下命令。
php artisan tinker
This command opens a repl
for interacting with your Laravel application. First let's migrate our database. While in the repl
, we can run our model factory and seed our database.
此命令打开一个repl
与您Laravel应用程序进行交互。 首先,让我们迁移数据库。 在repl
,我们可以运行模型工厂并为数据库添加种子。
factory(App\User::class, 10)->create();
A collection of ten new users should show up on your terminal. We can then check the database to see if the users were actually created.
十个新用户的集合应显示在您的终端上。 然后,我们可以检查数据库以查看是否实际创建了用户。
App\User::all();
To get the total number of users in our database, we can just call count
on the User
model.
要获取数据库中的用户总数,我们只需调用User
模型上的count
即可。
App\User::count();
After running App\User::all()
and App\User::count()
, mine looks like this. You should get something similar to mine only difference being the data generated.
运行App\User::all()
和App\User::count()
,我的看起来像这样。 您应该得到类似于我的东西,只是所生成的数据有所不同。
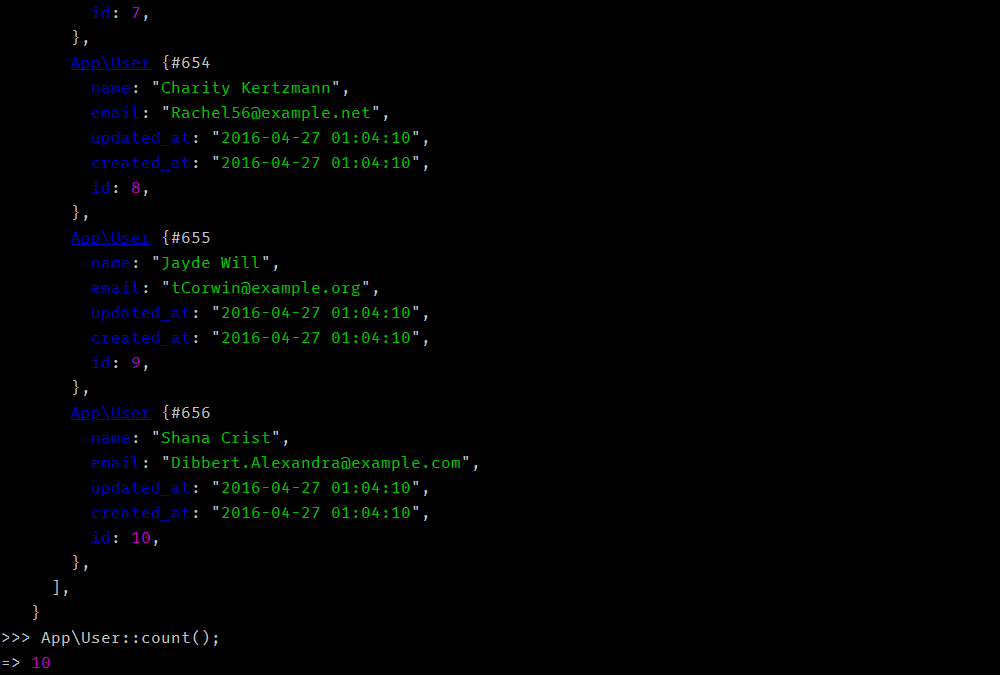
创建一个新用户 ( Creating a New User )
From the repl, we can create a new user. You should note that we interact with this repl
just like you would write code in your laravel application. So to create a new user, we would do
从REPL,我们可以创建一个新用户。 您应该注意,我们与此repl
进行交互,就像您在laravel应用程序中编写代码一样。 因此,要创建一个新用户,我们将
$user = new App\User;
$user->name = "Wruce Bayne";
$user->email = "iambatman@savegotham.com";
$user->save();
Now we can type $user
to the repl
and get something like this.
现在我们可以在repl
键入$user
并得到类似的内容。
删除用户 ( Deleting a User )
To delete a user, we can just do
要删除用户,我们可以做
$user = App\User::find(1);
$user->delete();
阅读功能/类文档 ( Reading a Function/Class Documentation )
With tinker, you can checkout a class or function documentation right from the repl
. But it depends on the class or function having DocBlocks
.
使用修补程序,您可以直接从repl
检出类或函数文档。 但这取决于具有DocBlocks
的类或函数。
doc<functionName> # replace <functionName> with function name or class FQN
Calling doc
on dd
gives us this.
在dd
上调用doc
可以使我们doc
这一点。
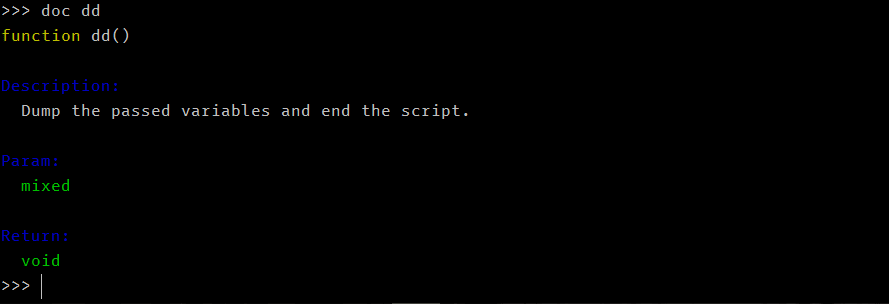
检查来源 ( Checking Source )
We can also check out a function or class source code while in the repl
using
我们还可以在repl
使用以下命令签出函数或类源代码
show<functionName>
For example, calling show
on dd
give us this.
例如,在dd
上调用show
可以达到这个目的。
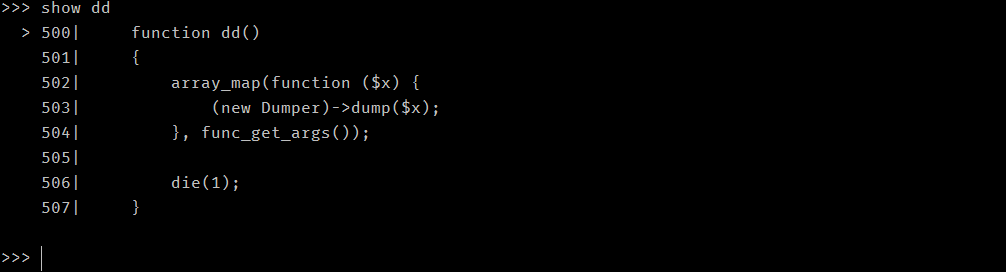
结论 ( Conclusion )
Laravel Tinker is a tool that can help us easily interact with our application without having to spin up a local server. Think of a simple feature you want to test in a couple of lines you'd delete from your project, use tinker instead.
Laravel Tinker是一个工具,可以帮助我们轻松地与应用程序进行交互,而不必启动本地服务器。 考虑一下要在要从项目中删除的几行中进行测试的简单功能,请改用tinker。
翻译自: https://scotch.io/tutorials/tinker-with-the-data-in-your-laravel-apps-with-php-artisan-tinker