grpc调用
RPC简介 ( Introduction To RPC )
Remote procedure call (RPC) architecture is popular in building scalable distributed client/server model based applications.
远程过程调用(RPC)体系结构在构建可扩展的基于分布式客户端/服务器模型的应用程序中很流行。
RPC allows a client to a make procedure call (also referred to as subroutine call or function call ) to a server on a different address space without understanding the network configuration as if the server was in the same network (making a local procedure call).
RPC允许客户端在不同的地址空间上对服务器进行过程调用(也称为子例程调用或函数调用 ),而无需像服务器在同一网络中那样理解网络配置(进行本地过程调用 )。
In this tutorial, we will implement an RPC client/server model using Google's gRPC and Protocol Buffers.
在本教程中,我们将使用Google的gRPC和协议缓冲区来实现RPC客户端/服务器模型。
RPC如何运作 (How RPC Works)
Before we dive into the heart of RPC, let's take some time to understand how it works.
在深入研究RPC的核心之前,让我们花一些时间来了解它是如何工作的。
- A client application makes a local procedure call to the client stub containing the parameters to be passed on to the server. 客户端应用程序对客户端存根进行本地过程调用,其中包含要传递给服务器的参数。
- The client stub serializes the parameters through a process called marshalling forwards the request to the local client-time library in the local computer which forwards the request to the server stub. 客户端存根通过称为封送处理的过程对参数进行序列化,该过程将请求转发到本地计算机中的本地客户端时间库,本地计算机将请求转发到服务器存根。
- The server run-time library receives the request and calls the server stub procedure which unmarshalls (unpacks) the passed parameters and calls the actual procedure. 服务器运行时库接收该请求并调用服务器存根过程,该过程将解组(解包)传递的参数并调用实际过程。
- The server stub sends back a response to the client-stub in the same fashion, a point at which the client resumes normal execution. 服务器存根以相同的方式将响应发送回客户端存根,此时客户端将恢复正常执行。
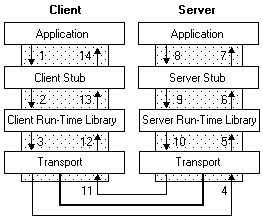
何时以及为何使用RPC ( When and Why to use RPC )
While in the process of building out a scalable microservice architecture and Andela, we are implementing REST to expose API endpoints, through and API gateway, to external applications (mostly web apps in AngularJS and ReactJS). Inter service communication is then handled using RPC.
在构建可伸缩的微服务架构和Andela的过程中 ,我们正在实现REST,以通过API网关将API端点公开给外部应用程序(主要是AngularJS和ReactJS中的Web应用程序)。 然后使用RPC处理服务间通信。
Remote procedure call's data serialization into binary format for inter-process (server to server) communication makes it ideal for building out scalable microservice architecture by improving performance.
远程过程调用将数据序列化为二进制格式以进行进程间(服务器到服务器)通信,使其成为通过提高性能来构建可伸缩微服务体系结构的理想选择。
RPC client and server run-time stubs take care of the network protocol and communication so that you can focus on your application.
RPC客户端和服务器运行时存根负责网络协议和通信,因此您可以专注于应用程序。
RPC与REST。 斗争! ( RPC vs REST. Fight! )
While RPC and REST use the request/response HTTP protocol to send and receive data respectively, REST is the most popular and preferred client-server communication approach.
尽管RPC和REST使用请求/响应HTTP协议分别发送和接收数据,但是REST是最受欢迎的客户端-服务器通信方法。
Before we dive into RPC. Let's make a comparison of the two.
在我们深入到RPC之前。 让我们对两者进行比较。
- REST as an architectural style implements HTTP protocol between a client and a server through a set of constraints, typically a method (GET) and a resource or endpoint (/users/1). REST作为一种体系结构样式,通过一组约束(通常是方法( GET )和资源或端点( / users / 1 ))在客户端和服务器之间实现HTTP协议。
- RPC implements client and server stubs that essentially make it possible to make calls to procedures over a network address as if the procedure was local. Simply put, RPC exposes methods (say, getUsers()) in the server to the client. RPC实现了客户端和服务器存根,从本质上来说,可以通过网络地址对过程进行调用,就像过程是本地的一样。 简而言之,RPC将服务器中的方法(例如getUsers() )公开给客户端。
The choice of architecture to use entirely remains at the discretion of the development teams and the nature of the system. Some developers have argued against comparing the two, and rightfully so because they can work well together.
完全使用体系结构的选择取决于开发团队和系统的性质。 一些开发人员反对将两者进行比较,这是正确的,因为它们可以很好地协同工作。
Read more about how the two compare on this article by Joshua Hartman.
在Joshua Hartman的这篇文章中阅读更多有关两者比较的信息。
使用gRPC和协议缓冲区实现RPC ( Implementing RPC with gRPC and Protocol Buffers )
Google managed gRPC is a very popular open source RPC framework with support of languages such as C++, Java, Python, Go, Ruby, Node.js, C#, Objective-C and PHP.
Google托管的gRPC是一个非常流行的开源RPC框架,支持诸如C ++,Java,Python,Go,Ruby,Node.js,C#,Objective-C和PHP等语言。
gRPC is a modern open source high performance RPC framework that can run in any environment. It can efficiently connect services in and across data centers with pluggable support for load balancing, tracing, health checking and authentication. It is also applicable in last mile of distributed computing to connect devices, mobile applications and browsers to backend services.
gRPC是可以在任何环境中运行的现代开源高性能RPC框架。 它可以通过可插拔的支持来有效地连接数据中心内和跨数据中心的服务,以实现负载平衡,跟踪,健康检查和身份验证。 它也适用于分布式计算的最后一英里,以将设备,移动应用程序和浏览器连接到后端服务。
As we mentioned, with gRPC, a client application can directly call methods on a server application on a different network as if the method was local. The beauty about RPC is that it is language agnostic. This means you could have a grpc server written in Java handling client calls from node.js, PHP and Go.
如前所述,使用gRPC,客户端应用程序可以直接在其他网络上的服务器应用程序上调用方法,就像该方法是本地方法一样。 RPC的优点在于它与语言无关。 这意味着您可以使用Java编写的grpc服务器来处理来自node.js,PHP和Go的客户端调用。