You may have heard of Python before, especially if you have been coding for a while.
您可能以前听说过Python,特别是如果您编码已有一段时间。
If not, Python is a high level, general purpose programming language. What this means is that you can use it to code up anything from a simple game to a website supporting millions of users per month.
如果不是这样,Python是一种高级通用编程语言。 这意味着您可以使用它来编写代码,从简单的游戏到每月可支持数百万用户的网站。
In fact, several high profile sites with millions of visitors per month rely on Python for some of their services. Examples include YouTube and Dropbox
实际上,每个月有数百万访问者的知名网站都依赖Python提供某些服务。 例子包括YouTube和Dropbox

That being said, why should you use Python in the first place? Why not one of the many other popular languages out in the wild like Ruby or PHP? Well, with Python you get the following awesome benefits:
话虽这么说,为什么首先要使用Python? 为什么不像Ruby或PHP那样狂热地使用其他众多流行语言之一? 好吧,有了Python,您将获得以下令人敬畏的好处:
- Easily readable syntax. 语法易读。
- Awesome community around the language. 很棒的语言社区。
- Easy to learn. 简单易学。
- Python is useful for a myriad of tasks from basic shell sripting to advanced web development. Python适用于从基本Shell转换到高级Web开发的众多任务。
什么时候不使用Python ( When Not to Use Python )
While you can easily write a Desktop app with Python using tools like wxPython, you generally would do better to use the specialized tools offered by the platform you are targeting for example .NET on Windows.
尽管您可以使用wxPython之类的工具轻松地使用Python编写桌面应用程序,但通常最好使用目标平台所提供的专用工具,例如Windows上的.NET。
You should also not use Python when your particular use case has very specialized requirements which are better met by other languages. An example is when you are building an embedded system, a domain in which languages like C, C++ and Java dominate.
当您的特定用例具有非常特殊的要求,而其他语言可以更好地满足这些要求时,您也不应使用Python。 一个示例是在构建嵌入式系统时,该系统是C,C ++和Java等语言占主导地位的域。
Python 2和Python 3 ( Python 2 vs Python 3 )
Python 2.7.x and 3.x are both being used extensively in the wild. Python 3 introduced changes into the language which required applications written in Python 2 to be rewritten in order to work with the Python 3.x branch. However, most libraries you will require to use have now been ported to Python 3.
Python 2.7.x和3.x都在野外广泛使用。 Python 3引入了对语言的更改,该语言需要重写用Python 2编写的应用程序才能与Python 3.x分支一起使用。 但是,您将需要使用的大多数库现在已移植到Python 3。
This tutorial will use Python 3 which is at version 3.5.1 at the time of writing. The principles remain the same though and only minor syntax modifications will be required to get the code running under Python 2.7.x.
在编写本文时,本教程将使用版本3.5.1的Python 3。 尽管原理保持不变,并且仅需对语法进行少量修改即可使代码在Python 2.7.x下运行。
一些Python代码样本 ( Some Python Code Samples )
你好,世界 (Hello World)
As I said before, one of Python's main benefits is its' very easily readable syntax. How easy? Check out Python's version of the ubiquitous Hello World
.
就像我之前说的,Python的主要好处之一是它的语法易于阅读。 有多容易? 查看无所不在的Hello World
Python版本。
# This line of code will print "Hello, World!" to your terminal
print("Hello, World!")
This code prints out Hello, World!
to the console. You can easily try out this code by visiting this site, pasting the code samples in the editor on the right side of the page, and clicking the run
button above the page to see the output.
此代码将打印出“ Hello, World!
到控制台。 您可以通过访问此站点 ,将代码示例粘贴到页面右侧的编辑器中,然后单击页面上方的run
按钮以查看输出,轻松地尝试这些代码。
条件逻辑 (Conditional Logic)
Conditional logic is just as easy. Here is some code to check if a user's age is above 18, and if it is, to print Access allowed
or Access not allowed
otherwise.
条件逻辑也很容易。 这是一些代码,用于检查用户的年龄是否大于18岁,如果是,则打印Access allowed
Access not allowed
或否则Access allowed
Access not allowed
。
# read in age
age = int(input("What's your age?"))
if age >= 18:
print("Access allowed")
elif age < 18 and age > 0:
print("Access not allowed")
else:
print("Invalid age")
The input()
function is used to read in keyboard input. You will therefore need to type something in the terminal prompt after running the script for rest of the script to execute. Note that the input()
function is wrapped in the int()
function.
input()
函数用于读取键盘输入。 因此,您需要在运行脚本后在终端提示符下键入一些内容,才能执行其余脚本。 注意, input()
函数包装在int()
函数中。
This is because input()
reads in values as strings
and yet we need age to be an integer
. We therefore have to cast the keyboard input into a string or else we will get an error for example when checking if the string is greater than 18.
这是因为input()
以strings
读取值,但我们需要age为integer
。 因此,我们必须将键盘输入转换为字符串,否则例如在检查字符串是否大于18时会出现错误。
Finally, note the else
statement which executes for any other input which doesn't fit the criteria being checked for in the if statements.
最后,请注意else
语句,该语句针对不符合if语句中要检查的条件的任何其他输入执行。
抽象数据类型 (Abstract Data Types)
Python also has some excellent built in abstract data types for holding collections of items. An example is a list which can be used to hold variables of any type. The following code shows how to create a list and iterate through it to print each item to the terminal.
Python还具有一些出色的内置抽象数据类型,用于保存项目集合。 一个示例是一个列表,可用于保存任何类型的变量。 以下代码显示了如何创建列表并遍历列表以将每个项目打印到终端。
# create a list called my_list
my_list = [1, 2, 3, "python", 67, [4, 5]]
# go through my_list and print every item
for item in my_list:
print item
The code above creates a list with numbers, a string and a list (yes, lists can contain other lists!). To iterate through lists, a for-in
loop comes in handy. Remember, lists are zero-indexed so we can also access list items using indexes. For example, to output the string python
, you can write:
上面的代码创建一个包含数字,字符串和列表的列表(是的,列表可以包含其他列表!)。 要遍历列表,可以使用for-in
循环。 请记住,列表是零索引的,因此我们也可以使用索引访问列表项。 例如,要输出字符串python
,您可以编写:
# create a list called my_list
my_list = [1, 2, 3, "python", 67, [4, 5]]
print(my_list[3])
辞典 (Dictionaries)
Another excellent data type Python offers out of the box is dictionaries. Dictionaries store key-value pairs, kind of like JSON objects. Creating a dictionary is quite simple as well.
Python提供的另一种出色的数据类型是字典。 字典存储键值对,类似于JSON对象。 创建字典也非常简单。
# create a dictionary
person = {
"name": "Amos",
"age": 23,
"hobbies": ["Travelling", "Swimming", "Coding", "Music"]
}
# iterate through the dict and print the keys
for key in person:
print(key)
# iterate through the dict's keys and print their values
for key in person:
print(person[key])
Now that you know a little bit of Python, let's talk about Django.
现在您已经了解Python了,让我们谈谈Django。
Django的 ( Django )

Django is a Python web framework. It is free and open source and has been around since 2005. It is very mature and comes with excellent documentation and awesome features included by default. Some excellent tools it provides are:
Django是Python网络框架。 它是免费和开源的,自2005年以来一直存在。它已经非常成熟,并具有出色的文档和默认包含的强大功能。 它提供了一些出色的工具:
- Excellent lightweight server for development and testing. 出色的轻量级服务器,用于开发和测试。
- Good templating language. 良好的模板语言。
- Security features like CSRF included right out of the box. 开箱即用的安全特性包括CSRF。
There are a myriad of other useful things included in Django but you shall probably discover them as you go along. We are going to be using Django to build our website in this tutorial.
Django中还包含许多其他有用的东西,但是您可能会在继续学习时发现它们。 在本教程中,我们将使用Django构建我们的网站。
配置 ( Setting Up )
In this tutorial, I will show you how to get a Django website up and running. Before we get there though, first grab a copy of the latest Python from the Python website.
在本教程中,我将向您展示如何启动和运行Django网站。 在到达那里之前,首先从Python网站上获取最新的Python副本。

Note that if you are on OSX and you have Homebrew installed you can do
请注意,如果您使用的是OSX并且安装了Homebrew,则可以
brewinstall python3
brew install python3
After that go straight to the
Getting started with Django
section之后,直接转到
Getting started with Django
部分
After installing the correct version for your OS, you will need to make sure it is set up correctly. Open a terminal and type:
在为您的操作系统安装了正确的版本之后,您需要确保正确设置了它。 打开一个终端并输入:
python3
You should see something resembling the following:
您应该看到类似于以下内容:
Python 3.5.1 ( v3.5.1:37a07cee5969, Dec 5 2015, 21:12:44 )
[ GCC 4.2.1 ( Apple Inc. build 5666 ) ( dot 3 ) ] on darwin
Type "help" , "copyright" , "credits" or "license" for more information.
>> >
This is the interactive Python shell. Hit CTRL + D
to exit it for now
这是交互式Python shell。 按CTRL + D
退出它
搭建环境 ( Setting Up the Environment )
To avoid polluting our global scope with unecessary packages, we are going to use a virtual environment to store our packages. One excellent virtual environment manager available for free is virtualenv
. We will be using Python's package manager pip
to install this and other packages like Django which we will require later on. First, let's get virtualenv
installed.
为了避免不必要的软件包污染我们的全球范围,我们将使用虚拟环境来存储我们的软件包。 免费提供的一款出色的虚拟环境管理器是virtualenv
。 我们将使用Python的软件包管理器pip
来安装此软件包以及其他类似Django的软件包,稍后我们将需要它们。 首先,让我们安装virtualenv
。
pip install virtualenv
Once that is done, create a folder called projects anywhere you like then cd
into it.
完成后,在任意位置创建一个名为projects的文件夹,然后将其插入cd
。
mkdir projects
cd projects
Once inside the projects folder, create another folder called hello. This folder will hold our app.
进入projects文件夹后,创建另一个名为hello的文件夹。 此文件夹将保存我们的应用程序。
mkdir hello
At this point, we need to create the environment to hold our requirements. We will do this inside the hello
folder.
在这一点上,我们需要创建一个环境来满足我们的需求。 我们将在hello
文件夹中执行此操作。
virtualenv -p /usr/local/bin/python3 env
The -p
switch tells virtualenv the path to the python version you want to use. Feel free to switch out the path after it with your own Python installation path. The name env
is the environment name. You can also change it to something else which fits the name of your project.
-p
开关告诉virtualenv您要使用的python版本的路径。 您可以使用自己的Python安装路径随意切换路径。 名称env
是环境名称。 您也可以将其更改为适合项目名称的其他名称。
Once that is done, you should have a folder called env
inside your hello
folder. Your structure should now look something like this.
完成此操作后,您将在hello
文件夹中有一个名为env
的文件夹。 您的结构现在应该看起来像这样。
projects
├─hello
│ ├── env
You are now ready to activate the environment and start coding!
现在您可以激活环境并开始编码了!
source env/bin/activate
You will see a prompt with the environment name. That means the environment is active.
您将看到带有环境名称的提示。 这意味着环境处于活动状态。
( env )
安装Django ( Installing Django )
This is a simple pip install. The latest Django version at the time of writing is Django 1.9.6
这是一个简单的pip安装。 撰写本文时,最新的Django版本是Django 1.9.6。
pip install django
创建一个应用 ( Creating an App )
Now that Django is installed, we can use its start script to create a skeleton project. This is as simple as using its admin script in the following way.
现在已经安装了Django,我们可以使用其启动脚本来创建框架项目。 这就像通过以下方式使用其管理脚本一样简单。
django-admin startproject helloapp
Running this command creates a skeleton django app with the following structure:
运行此命令将创建具有以下结构的django骨架应用程序:
helloapp
├─helloapp
│ ├── __ init __ .py
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
└── manage.py
When you look into the helloapp
folder that was created, you will find a file called manage.py
and another folder called helloapp
. This is your main project folder and contains the project's settings in a file called settings.py
and the routes in your project in the file called urls.py
. Feel free to open up the settings.py
file to familiarize yourself with its contents.
当您查看创建的helloapp
文件夹时,将找到一个名为manage.py
的文件和另一个名为helloapp
文件夹。 这是您的主项目文件夹,并且在名为settings.py
的文件中包含项目的设置,而在名为urls.py
的文件中包含项目中的路由。 随时打开settings.py
文件以熟悉其内容。
Ready to move on? Excellent.
准备继续前进吗? 优秀的。
更改应用程序设置 ( Changing App Settings )
Let's change a few settings. Open up the settings.py
file in your favorite editor. Find a section called Installed Apps which looks something like this.
让我们更改一些设置。 在您喜欢的编辑器中打开settings.py
文件。 找到一个类似于“已安装的应用程序”的部分。
# helloapp/settings.py
INSTALLED_APPS = [
'django.contrib.admin' ,
'django.contrib.auth' ,
'django.contrib.contenttypes' ,
'django.contrib.sessions' ,
'django.contrib.messages' ,
'django.contrib.staticfiles' ,
]
Django operates on the concept of apps. An app is a self contained unit of code which can be executed on its own. An app can do many things such as serve a webpage on the browser or handle user authentication or anything else you can think of. Django comes with some default apps preinstalled such as the authentication and session manager apps. Any apps we will create or third-party apps we will need will be added at the bottom of the Installed Apps
list after the default apps installed.
Django致力于应用程序的概念。 应用程序是一个可独立执行的代码单元。 应用程序可以做很多事情,例如在浏览器上提供网页或处理用户身份验证或您可以想到的其他任何事情。 Django预先安装了一些默认应用程序,例如身份验证和会话管理器应用程序。 在Installed Apps
默认应用程序之后,我们将创建的任何应用程序或所需的第三方应用程序都将添加到“ Installed Apps
列表的底部。
Before we create a custom app, let's change the application timezone. Django uses the tz database
timezones, a list of which can be found here.
在创建自定义应用程序之前,让我们更改应用程序时区。 Django使用tz database
时区,可在此处找到其时区列表。
The timezone setting looks like this.
时区设置如下所示。
# helloapp/settings.py
TIME_ZONE = 'UTC'
Change it to something resembling this as appropriate for your timezone.
将其更改为类似于您所在时区的名称。
# helloapp/settings.py
TIME_ZONE = 'America/Los_Angeles'
创建自己的应用 ( Creating your own app )
It is important to note that Django apps follow the Model, View, Template paradigm. In a nutshell, the app gets data from a model, the view does something to the data and then renders a template containing the processed information. As such, Django templates correspond to views in traditional MVC and Django views can be likened to the controllers found in traditional MVC.
请务必注意,Django应用遵循模型,视图,模板范式。 简而言之,应用程序从模型获取数据,视图对数据进行处理,然后呈现包含已处理信息的模板。 这样,Django模板对应于传统MVC中的视图,并且Django视图可以比作传统MVC中的控制器。
That being said, let's create an app. cd
into the first helloapp
folder and type;
话虽如此,让我们创建一个应用程序。 cd
进入第一个helloapp
文件夹并输入;
python manage.py startapp howdy
Running this command will create an app called howdy. Your file structure should now look something like this.
运行此命令将创建一个名为howdy的应用程序。 您的文件结构现在应该看起来像这样。
helloapp
├── helloapp
│ ├── __ init __ .py
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
├── howdy
│ ├── __ init __ .py
│ ├── admin.py
│ ├── apps.py
│ ├── migrations
│ ├── models.py
│ ├── tests.py
│ └── views.py
└── manage.py
To get Django to recognize our brand new app, we need to add the app name to the Installed Apps
list in our settings.py
file.
为了使Django能够识别我们全新的应用程序,我们需要将应用程序名称添加到settings.py
文件中的Installed Apps
列表中。
# helloapp/settings.py
INSTALLED_APPS = [
'django.contrib.admin' ,
'django.contrib.auth' ,
'django.contrib.contenttypes' ,
'django.contrib.sessions' ,
'django.contrib.messages' ,
'django.contrib.staticfiles' ,
'howdy'
]
Once that is done, let's run our server and see what will be output. We mentioned that Django comes with a built in lightweight web server which, while useful during development, should never be used in production. Run the server as follows:
完成后,让我们运行服务器,看看将输出什么。 我们提到Django带有一个内置的轻量级Web服务器,该服务器虽然在开发过程中很有用,但切勿在生产中使用。 如下运行服务器:
python manage.py runserver
Your output should resemble the following:
您的输出应类似于以下内容:
Performing system checks .. .
System check identified no issues ( 0 silenced ) .
You have unapplied migrations ; your app may not work properly until they are applied.
Run 'python manage.py migrate' to apply them.
June 04, 2016 - 07:42:08
Django version 1.9.6, using settings 'helloapp.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
If you look carefully, you will see a warning that you have unapplied migrations. Ignore that for now. Go to your browser and access http://127.0.0.1:8000/
. If all is running smoothly, you should see the Django welcome page.
如果仔细查看,将会看到一条警告,提示您尚未应用迁移。 现在忽略它。 转到浏览器并访问http://127.0.0.1:8000/
。 如果一切运行顺利,则应该看到Django欢迎页面。

We are going to replace this page with our own template. But first, let's talk migrations.
我们将用我们自己的模板替换此页面。 但是首先,让我们谈谈迁移。
移居 ( Migrations )
Migrations make it easy for you to change your database schema (model) without having to lose any data. Any time you create a new database model, running migrations will update your database tables to use the new schema without you having to lose any data or go through the tedious process of dropping and recreating the database yourself.
迁移使您轻松更改数据库架构(模型)而不必丢失任何数据。 每当您创建新的数据库模型时,运行迁移都会更新您的数据库表以使用新的架构,而不必丢失任何数据或经历繁琐的删除和重新创建数据库的过程。
Django comes with some migrations already created for its default apps. If your server is still running, stop it by hitting CTRL + C
. Apply the migrations by typing:
Django随附了一些为其默认应用创建的迁移。 如果服务器仍在运行,请通过按CTRL + C
停止它。 通过键入以下内容来应用迁移:
python manage.py migrate
If successful, you will see an output resembling this one.
如果成功,您将看到类似于此的输出。
Operations to perform:
Apply all migrations: sessions, auth, contenttypes, admin
Running migrations:
Rendering model states .. . DONE
Applying contenttypes.0001_initial .. . OK
Applying auth.0001_initial .. . OK
Applying admin.0001_initial .. . OK
Applying admin.0002_logentry_remove_auto_add .. . OK
Applying contenttypes.0002_remove_content_type_name .. . OK
Applying auth.0002_alter_permission_name_max_length .. . OK
Applying auth.0003_alter_user_email_max_length .. . OK
Applying auth.0004_alter_user_username_opts .. . OK
Applying auth.0005_alter_user_last_login_null .. . OK
Applying auth.0006_require_contenttypes_0002 .. . OK
Applying auth.0007_alter_validators_add_error_messages .. . OK
Applying sessions.0001_initial .. . OK
Running the server now will not show any warnings.
现在运行服务器将不会显示任何警告。
网址和模板 ( Urls & Templates )
When we ran the server, the default Django page was shown. We need Django to access our howdy
app when someone goes to the home page URL which is /
. For that, we need to define a URL which will tell Django where to look for the homepage template.
当我们运行服务器时,将显示默认的Django页面。 当有人转到/
主页URL时,我们需要Django访问我们的howdy
应用程序。 为此,我们需要定义一个URL,该URL会告诉Django在哪里寻找主页模板。
Open up the urls.py
file inside the inner helloapp
folder. It should look like this.
打开内部helloapp
文件夹中的urls.py
文件。 它应该看起来像这样。
# helloapp/urls.py
"""helloapp URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/1.9/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: url(r'^$', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: url(r'^$', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.conf.urls import url, include
2. Add a URL to urlpatterns: url(r'^blog/', include('blog.urls'))
"""
from django . conf . urls import url
from django . contrib import admin
urlpatterns = [
url ( r '^admin/' , admin . site . urls ) ,
]
As you can see, there is an existing URL pattern for the Django admin site which comes by default with Django. Let's add our own url to point to our howdy app. Edit the file to look like this.
如您所见,Django管理站点有一个现有的URL模式,默认情况下是Django附带的。 让我们添加自己的网址以指向我们的howdy应用程序。 编辑文件,如下所示。
# helloapp/urls.py
from django . conf . urls import url , include
from django . contrib import admin
urlpatterns = [
url ( r '^admin/' , admin . site . urls ) ,
url ( r '^' , include ( 'howdy.urls' ) ) ,
]
Note that we have added an import for include
from django.conf.urls and added a url pattern for an empty route. When someone accesses the homepage, (in our case http://localhost:8000), Django will look for more url definitions in the howdy
app. Since there are none, running the app will produce a huge stack trace due to an ImportError
.
请注意,我们从django.conf.urls添加了include
的导入,并为空路由添加了url模式。 当有人访问主页(在我们的示例中为http:// localhost:8000 )时,Django将在howdy
应用程序中查找更多url定义。 由于没有任何内容,由于ImportError
,运行该应用程序将产生巨大的堆栈跟踪。
.
.
ImportError: No module named 'howdy.urls'
Let's fix that. Go to the howdy
app folder and create a file called urls.py
. The howdy
app folder should now look like this.
让我们修复它。 转到howdy
应用程序文件夹并创建一个名为urls.py
的文件。 howdy
应用程序文件夹现在应如下所示。
├── howdy
│ ├── __ init __ .py
│ ├── admin.py
│ ├── apps.py
│ ├── migrations
│ │ ├── __ init __ .py
│ ├── models.py
│ ├── tests.py
│ ├── urls.py
│ └── views.py
Inside the new urls.py
file, write this.
在新的urls.py
文件中,编写此代码。
# howdy/urls.py
from django . conf . urls import url
from howdy import views
urlpatterns = [
url ( r '^$' , views . HomePageView . as_view ( ) ) ,
]
This code imports the views from our howdy
app and expects a view called HomePageView
to be defined. Since we don't have one, open the views.py
file in the howdy
app and write this code.
此代码从我们的howdy
应用程序导入视图,并期望定义一个称为HomePageView
的视图。 由于我们没有人,所以在howdy
应用程序中打开views.py
文件并编写此代码。
# howdy/views.py
from django . shortcuts import render
from django . views . generic import TemplateView
# Create your views here.
class HomePageView ( TemplateView ) :
def get ( self , request , ** kwargs ) :
return render ( request , 'index.html' , context = None )
This file defines a view called HomePageView
. Django views take in a request
and return a response
. In our case, the method get
expects a HTTP GET request to the url defined in our urls.py
file. On a side note, we could rename our method to post
to handle HTTP POST requests.
该文件定义了一个称为HomePageView
的视图。 Django视图接受request
并返回response
。 在我们的例子中,方法get
期望对我们的urls.py
文件中定义的url发出HTTP GET请求。 附带说明一下,我们可以重命名方法以post
以处理HTTP POST请求。
Once a HTTP GET request has been received, the method renders a template called index.html
which is just a normal HTML file which could have special Django template tags written alongside normal HTML tags. If you run the server now, you will see the following error page:
收到HTTP GET请求后,该方法将呈现一个名为index.html
的模板,该模板只是一个普通HTML文件,可以在普通HTML标签的旁边写入特殊的Django模板标签。 如果现在运行服务器,将看到以下错误页面:

This is because we do not have any templates at all! Django looks for templates in a templates
folder inside your app so go ahead and create one in your howdy
app folder.
这是因为我们根本没有任何模板! Django在您的应用程序内的templates
文件夹中查找模板,因此请继续在您howdy
app文件夹中创建一个templates
。
mkdir templates
Go into the templates folder you just created and create a file called index.html
进入刚刚创建的模板文件夹,并创建一个名为index.html
的文件
( env ) hello/helloapp/howdy/templates
> touch index.html
Inside the index.html
file, paste this code.
在index.html
文件中,粘贴此代码。
<!-- howdy/templates/index.html -->
<!DOCTYPE html>
< html >
< head >
< meta charset = " utf-8 " >
< title > Howdy! </ title >
</ head >
< body >
< h1 > Howdy! I am Learning Django! </ h1 >
</ body >
</ html >
Now run your server.
现在运行您的服务器。
python manage.py runserver
You should see your template rendered.
您应该看到呈现的模板。
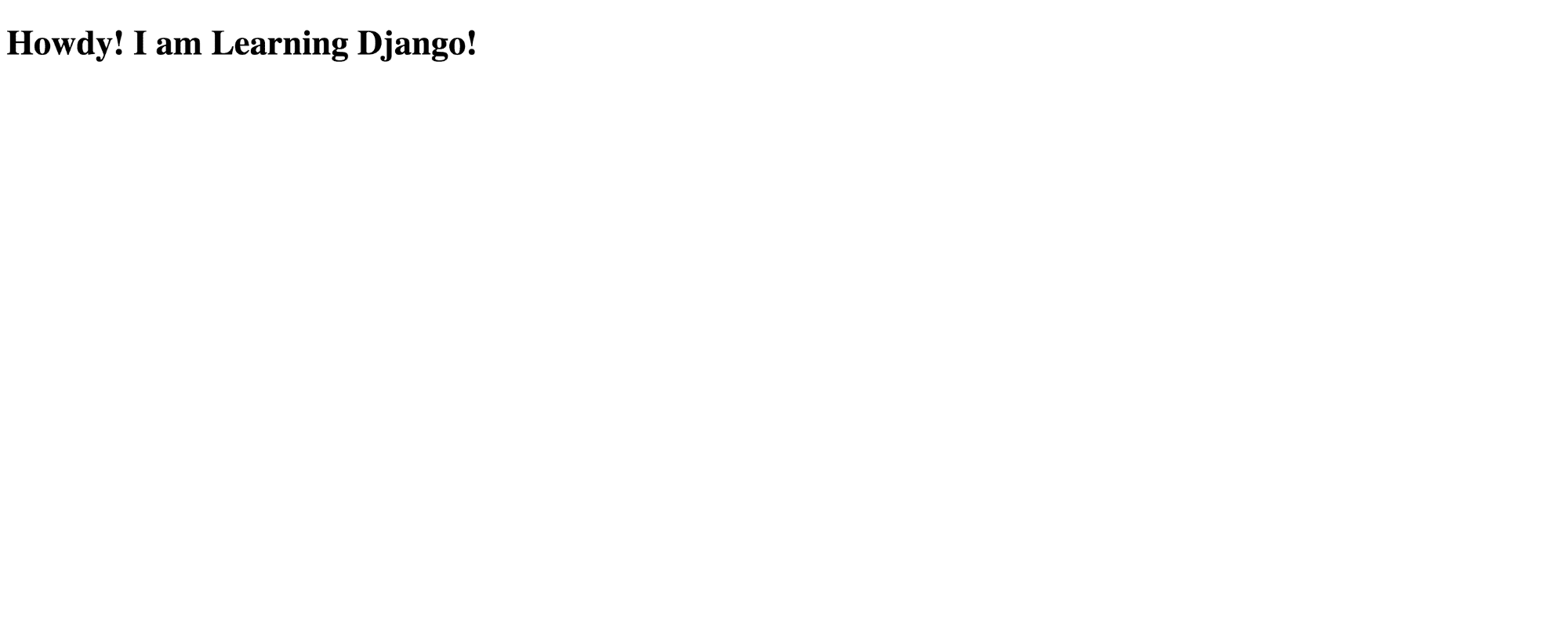
链接页面 ( Linking pages )
Let's add another page. In your howdy/templates
folder, add a file called about.html
. Inside it, write this HTML code:
让我们添加另一个页面。 在您的howdy/templates
文件夹中,添加一个名为about.html
的文件。 在其中编写以下HTML代码:
<!-- howdy/templates/about.html -->
<!DOCTYPE html>
< html >
< head >
< meta charset = " utf-8 " >
< title > Howdy! </ title >
</ head >
< body >
< h1 > Welcome to the about page </ h1 >
< p >
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc quis neque ex. Donec feugiat egestas dictum. In eget erat sit amet elit pellentesque convallis nec vitae turpis. Vivamus et molestie nisl. Aenean non suscipit velit. Nunc eleifend convallis consectetur. Phasellus ornare dolor eu mi vestibulum, ornare tempus lacus imperdiet. Interdum et malesuada fames ac ante ipsum primis in faucibus. Pellentesque ut sem ligula. Mauris volutpat vestibulum dui in cursus. Donec aliquam orci pellentesque, interdum neque sit amet, vulputate purus. Quisque volutpat cursus nisl, in volutpat velit lacinia id. Maecenas id felis diam.
</ p >
< a href = " / " > Go back home </ a >
</ body >
</ html >
Once done, edit the original index.html
page to look like this.
完成后,编辑原始的index.html
页面,如下所示。
<!-- howdy/templates/index.html -->
<!DOCTYPE html>
< html >
< head >
< meta charset = " utf-8 " >
< title > Howdy! </ title >
</ head >
< body >
< h1 > Howdy! I am Learning Django! </ h1 >
< a href = " /about/ " > About Me </ a >
</ body >
</ html >
Clicking on the About me
link won't work quite yet because our app doesn't have a /about/
url defined. Let's edit the urls.py
file in our howdy
app to add it.
由于我们的应用程序未定义/about/
网址,因此点击About me
链接尚无法使用。 让我们在howdy
应用程序中编辑urls.py
文件以添加它。
# howdy/urls.py
from django . conf . urls import url
from howdy import views
urlpatterns = [
url ( r '^$' , views . HomePageView . as_view ( ) ) ,
url ( r '^about/$' , views . AboutPageView . as_view ( ) ) , # Add this /about/ route
]
Once we have added the route, we need to add a view to render the about.html
template when we access the /about/
url. Let's edit the views.py
file in the howdy
app.
添加路线后,当我们访问/about/
url时,需要添加一个视图以呈现about.html
模板。 让我们在howdy
应用程序中编辑views.py
文件。
# howdy/views.py
from django . shortcuts import render
from django . views . generic import TemplateView
class HomePageView ( TemplateView ) :
def get ( self , request , ** kwargs ) :
return render ( request , 'index.html' , context = None )
# Add this view
class AboutPageView ( TemplateView ) :
template_name = "about.html"
Notice that in the second view, I did not define a get
method. This is just another way of using the TemplateView
class. If you set the template_name
attribute, a get request to that view will automatically use the defined template. Try changing the HomePageView
to use the format used in AboutPageView
.
请注意,在第二个视图中,我没有定义get
方法。 这只是使用TemplateView
类的另一种方式。 如果设置template_name
属性,则对该视图的get请求将自动使用定义的模板。 尝试更改HomePageView
以使用AboutPageView
使用的格式。
Running the server now and accessing the home page should display our original template with the newly added link to the about page.
现在运行服务器并访问主页应显示我们的原始模板,并带有新添加的关于页面的链接。
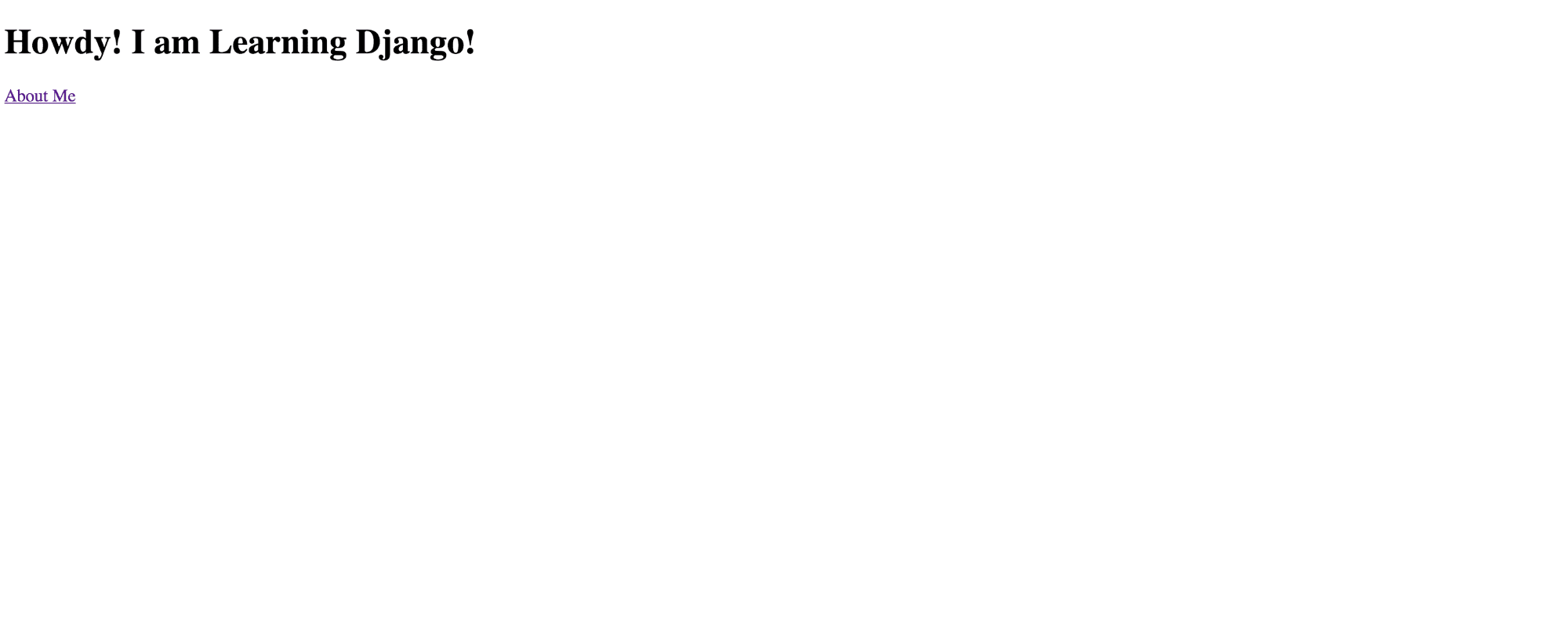
Clicking on the About me
link should direct you to the About
page.
单击“ About me
链接应将您定向到“ About
页面。
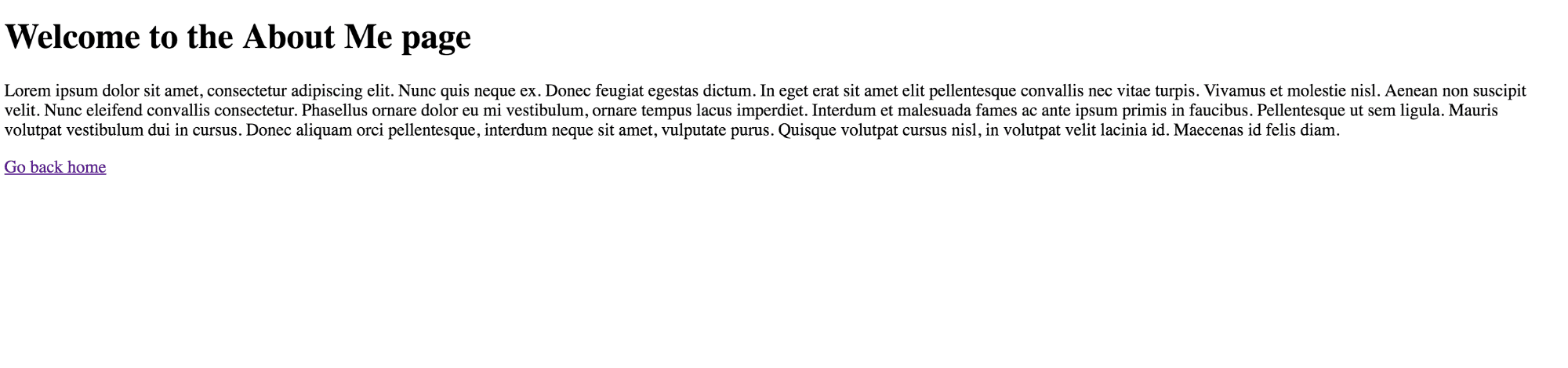
On the About me
page, clicking on the Go back home
link should redirect you back to our original index page. Try editing both these templates to add more information about you.
在“ About me
页面上,单击Go back home
链接应将您重定向到我们的原始索引页面。 尝试编辑这两个模板以添加有关您的更多信息。
结论 ( Conclusion )
That was just a brief look at how to get a Django website up and running. You can read more about Django on the official Django docs. The full code for this tutorial can also be found on Github.
这只是有关如何启动和运行Django网站的简要介绍。 您可以在官方Django文档上阅读有关Django的更多信息。 本教程的完整代码也可以在Github上找到。
翻译自: https://scotch.io/tutorials/build-your-first-python-and-django-application