python中的变量
In the previous tutorial on Basic Data Types in Python, you saw how values of various Python data types can be created. But so far, all the values shown have been literal or constant values:
在上一篇有关Python中的基本数据类型的教程中 ,您了解了如何创建各种Python数据类型的值。 但是到目前为止,所有显示的值都是文字或常量值:
>>> >>> printprint (( 5.35.3 )
)
5.3
5.3
If you’re writing more complex code, your program will need data that can change as program execution proceeds.
如果您要编写更复杂的代码,则程序将需要随着程序执行的进行而变化的数据。
Here’s what you’ll learn in this tutorial: You will learn how every item of data in a Python program can be described by the abstract term object, and you’ll learn how to manipulate objects using symbolic names called variables.
这是在本教程中您将学到的内容:您将学习如何用抽象术语object来描述Python程序中的每一项数据,并且还将学习如何使用称为变量的符号名来操作对象。
Get Notified: Don’t miss the follow up to this tutorial—Click here to join the Real Python Newsletter and you’ll know when the next installment comes out.
通知您:不要错过本教程的后续内容- 单击此处加入Real Python Newslet ,您将知道下一期的发行时间。
变量分配 (Variable Assignment)
Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign (=
):
将变量视为附加到特定对象的名称。 在Python中,不需要像其他许多编程语言一样预先声明或定义变量。 要创建变量,只需为它分配一个值,然后开始使用它。 分配使用单个等号( =
)完成:
This is read or interpreted as “n
is assigned the value 300
.” Once this is done, n
can be used in a statement or expression, and its value will be substituted:
可以将其读取或解释为“为n
分配了值300
。 完成此操作后,可以在语句或表达式中使用n
,其值将被替换:
>>> >>> printprint (( nn )
)
300
300
Just as a literal value can be displayed directly from the interpreter prompt in a REPL session without the need for print()
, so can a variable:
就像可以在REPL会话中直接从解释器提示中显示文字值而不需要print()
,变量也可以:
Later, if you change the value of n
and use it again, the new value will be substituted instead:
以后,如果您更改n
的值并再次使用,则将替换为新值:
>>> >>> n n = = 1000
1000
>>> >>> printprint (( nn )
)
1000
1000
>>> >>> n
n
1000
1000
Python also allows chained assignment, which makes it possible to assign the same value to several variables simultaneously:
Python还允许使用链式分配,这使得可以将相同的值同时分配给多个变量:
The chained assignment above assigns 300
to the variables a
, b
, and c
simultaneously.
上面的链接分配将300
同时分配给变量a
, b
和c
。
Python中的变量类型 (Variable Types in Python)
In many programming languages, variables are statically typed. That means a variable is initially declared to have a specific data type, and any value assigned to it during its lifetime must always have that type.
在许多编程语言中,变量是静态类型的。 这意味着变量最初被声明为具有特定的数据类型,并且在其生命周期内分配给它的任何值都必须始终具有该类型。
Variables in Python are not subject to this restriction. In Python, a variable may be assigned a value of one type and then later re-assigned a value of a different type:
Python中的变量不受此限制。 在Python中,可以为变量分配一种类型的值,然后在以后重新分配另一种类型的值:
>>> >>> var var = = 23.5
23.5
>>> >>> printprint (( varvar )
)
23.5
23.5
>>> >>> var var = = "Now I'm a string"
"Now I'm a string"
>>> >>> printprint (( varvar )
)
Now I'm a string
Now I'm a string
对象引用 (Object References)
What is actually happening when you make a variable assignment? This is an important question in Python, because the answer differs somewhat from what you’d find in many other programming languages.
进行变量分配时实际上发生了什么? 这是Python中的一个重要问题,因为答案与您在许多其他编程语言中所发现的有所不同。
Python is a highly object-oriented language. In fact, virtually every item of data in a Python program is an object of a specific type or class. (This point will be reiterated many times over the course of these tutorials.)
Python是一种高度面向对象的语言 。 实际上,Python程序中几乎每一项数据都是特定类型或类的对象。 (这一点将在本教程的过程中多次重申。)
Consider this code:
考虑以下代码:
When presented with the statement print(300)
, the interpreter does the following:
当出现语句print(300)
,解释器将执行以下操作:
- Creates an integer object
- Gives it the value
300
- Displays it to the console
- 创建一个整数对象
- 给它值
300
- 显示到控制台
You can see that an integer object is created using the built-in type()
function:
您可以看到使用内置的type()
函数创建了一个整数对象:
>>> >>> typetype (( 300300 )
)
<class 'int'>
<class 'int'>
A Python variable is a symbolic name that is a reference or pointer to an object. Once an object is assigned to a variable, you can refer to the object by that name. But the data itself is still contained within the object.
Python变量是一个符号名称,它是对对象的引用或指针。 将对象分配给变量后,即可使用该名称引用该对象。 但是数据本身仍然包含在对象中。
For example:
例如:
This assignment creates an integer object with the value 300
and assigns the variable n
to point to that object.
此分配创建一个值为300
的整数对象,并将变量n
分配为指向该对象。
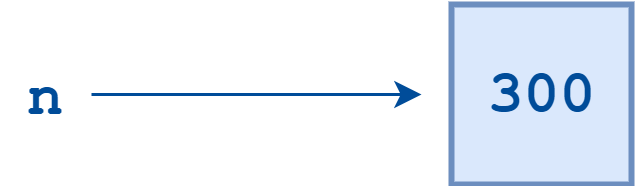
The following code verifies that n
points to an integer object:
以下代码验证n
指向一个整数对象:
>>> >>> printprint (( nn )
)
300
300
>>> >>> typetype (( nn )
)
<class 'int'>
<class 'int'>
Now consider the following statement:
现在考虑以下语句:
What happens when it is executed? Python does not create another object. It simply creates a new symbolic name or reference, m
, which points to the same object that n
points to.
执行它会发生什么? Python不会创建另一个对象。 它只是创建一个新的符号名称或引用m
,它指向n
指向的同一对象。

Next, suppose you do this:
接下来,假设您执行以下操作:
>>> >>> m m = = 400
400
Now Python creates a new integer object with the value 400
, and m
becomes a reference to it.
现在,Python创建了一个新的整数对象,其值是400
,而m
成为对它的引用。
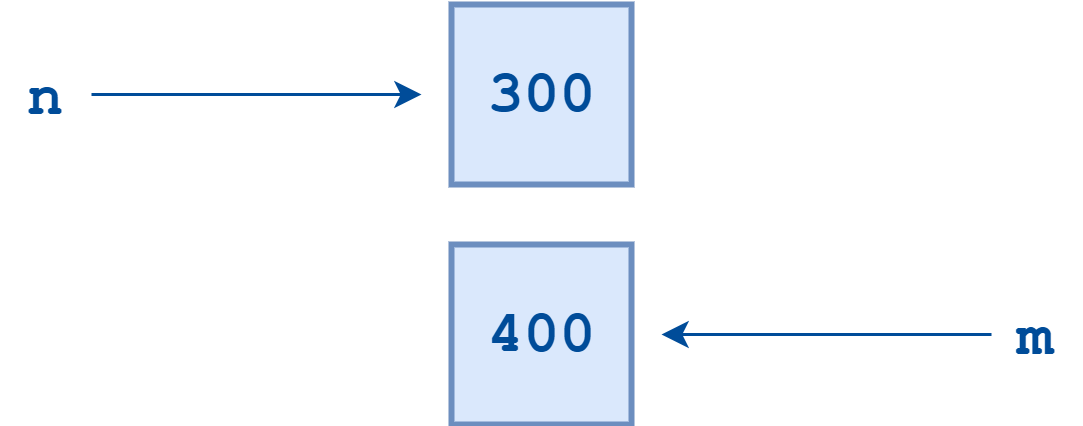
Lastly, suppose this statement is executed next:
最后,假设此语句接下来执行:
Now Python creates a string object with the value "foo"
and makes n
reference that.
现在,Python创建一个值为"foo"
的字符串对象,并使n
引用该对象。
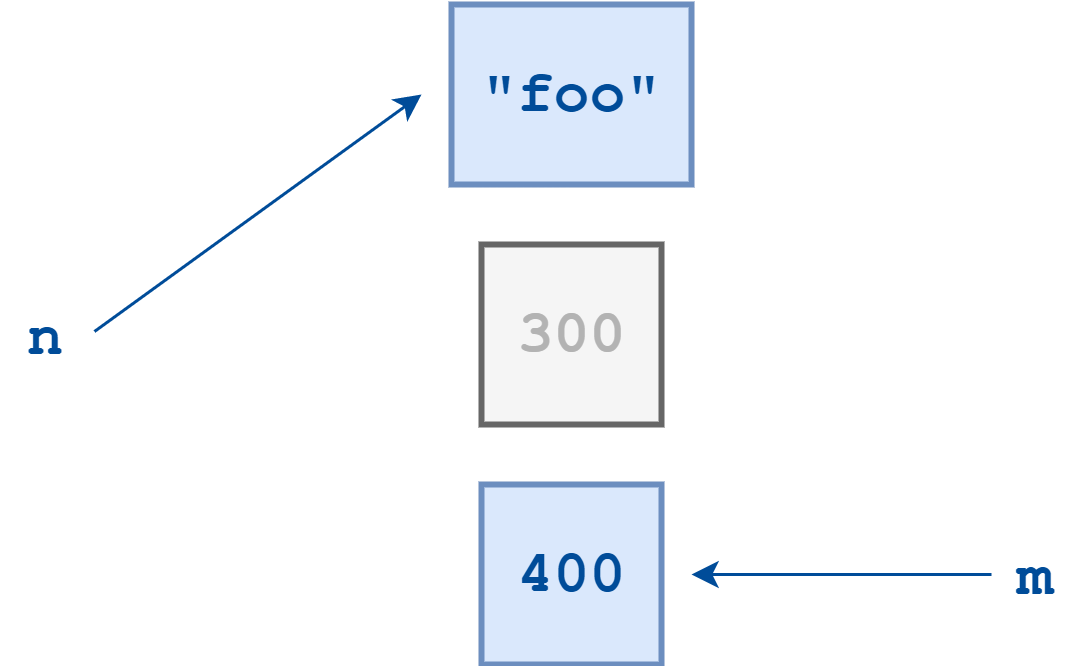
There is no longer any reference to the integer object 300
. It is orphaned, and there is no way to access it.
不再有对整数对象300
引用。 它是孤立的,无法访问它。
Tutorials in this series will occasionally refer to the lifetime of an object. An object’s life begins when it is created, at which time at least one reference to it is created. During an object’s lifetime, additional references to it may be created, as you saw above, and references to it may be deleted as well. An object stays alive, as it were, so long as there is at least one reference to it.
本系列中的教程有时会涉及对象的生存期。 对象的生命始于创建之时,这时至少创建了对其的一个引用。 如上所见,在对象的生命周期内,可能会创建对该对象的其他引用,并且对它的引用也可能会被删除。 只要有至少一个引用,一个对象就可以保持原样。
When the number of references to an object drops to zero, it is no longer accessible. At that point, its lifetime is over. Python will eventually notice that it is inaccessible and reclaim the allocated memory so it can be used for something else. In computer lingo, this process is referred to as garbage collection.
当对一个对象的引用数降至零时,将无法再访问该对象。 到那时,它的寿命结束了。 Python最终将注意到它不可访问,并回收分配的内存,以便将其用于其他用途。 在计算机术语中,此过程称为垃圾收集 。
对象身份 (Object Identity)
In Python, every object that is created is given a number that uniquely identifies it. It is guaranteed that no two objects will have the same identifier during any period in which their lifetimes overlap. Once an object’s reference count drops to zero and it is garbage collected, as happened to the 300
object above, then its identifying number becomes available and may be used again.
在Python中,为每个创建的对象赋予一个唯一标识它的数字。 确保在生命周期重叠的任何时间段内,没有两个对象具有相同的标识符。 一旦对象的引用计数降为零并被垃圾回收(如上面的300
对象一样),则其标识号可用,并且可以再次使用。
The built-in Python function id()
returns an object’s integer identifier. Using the id()
function, you can verify that two variables indeed point to the same object:
内置的Python函数id()
返回对象的整数标识符。 使用id()
函数,您可以验证两个变量确实指向同一对象:
>>> >>> n n = = 300
300
>>> >>> m m = = n
n
>>> >>> idid (( nn )
)
60127840
60127840
>>> >>> idid (( mm )
)
60127840
60127840
>>> >>> m m = = 400
400
>>> >>> idid (( mm )
)
60127872
60127872
After the assignment m = n
, m
and n
both point to the same object, confirmed by the fact that id(m)
and id(n)
return the same number. Once m
is reassigned to 400
, m
and n
point to different objects with different identities.
在赋值m = n
, m
和n
都指向同一个对象,这由id(m)
和id(n)
返回相同数字这一事实证实。 将m
重新分配给400
, m
和n
指向具有不同标识的不同对象。
深入研究:缓存较小的整数值 (Deep Dive: Caching Small Integer Values)
From what you now know about variable assignment and object references in Python, the following probably won’t surprise you:
从您现在对Python中的变量赋值和对象引用的了解中,以下内容可能不会令您感到惊讶:
>>> >>> m m = = 300 300 >>> >>> n n = = 300 300 >>> >>> idid (( mm ) ) 60062304 60062304 >>> >>> idid (( nn ) ) 60062896 60062896
With the statement
m = 300
, Python creates an integer object with the value300
and setsm
as a reference to it.n
is then similarly assigned to an integer object with value300
—but not the same object. Thus, they have different identities, which you can verify from the values returned byid()
.使用语句
m = 300
,Python创建一个值为300
的整数对象,并将m
设置为其引用。 然后类似地将n
分配给值为300
的整数对象,但不是同一对象。 因此,它们具有不同的身份,您可以从id()
返回的值中进行验证。But consider this:
但是考虑一下:
>>> >>> m m = = 30 30 >>> >>> n n = = 30 30 >>> >>> idid (( mm ) ) 1405569120 1405569120 >>> >>> idid (( nn ) ) 1405569120 1405569120
Here,
m
andn
are separately assigned to integer objects having value30
. But in this case,id(m)
andid(n)
are identical!在此,将
m
和n
分别分配给值为30
整数对象。 但是在这种情况下,id(m)
和id(n)
是相同的!For purposes of optimization, the interpreter creates objects for the integers in the range
[-5, 256]
at startup, and then reuses them during program execution. Thus, when you assign separate variables to an integer value in this range, they will actually reference the same object.为了优化起见,解释器在启动时为范围为
[-5, 256]
的整数创建对象,然后在程序执行期间重新使用它们。 因此,当您为该范围内的整数值分配单独的变量时,它们实际上将引用同一对象。
变量名 (Variable Names)
The examples you have seen so far have used short, terse variable names like m
and n
. But variable names can be more verbose. In fact, it is usually beneficial if they are because it makes the purpose of the variable more evident at first glance.
到目前为止,您所看到的示例都使用了简短而简洁的变量名,例如m
和n
。 但是变量名可能更冗长。 实际上,如果这样做通常是有益的,因为乍一看会使变量的目的更加明显。
Officially, variable names in Python can be any length and can consist of uppercase and lowercase letters (A-Z
, a-z
), digits (0-9
), and the underscore character (_
). An additional restriction is that, although a variable name can contain digits, the first character of a variable name cannot be a digit.
正式地,Python中的变量名称可以是任何长度,并且可以由大写和小写字母( AZ
, az
),数字( 0-9
)和下划线字符( _
)组成。 另一个限制是,尽管变量名可以包含数字,但是变量名的第一个字符不能是数字。
Note: One of the additions to Python 3 was full Unicode support, which allows for Unicode characters in a variable name as well. You will learn about Unicode in greater depth in a future tutorial.
注意: Python 3的新增功能之一是对Unicode的完全支持,该支持还允许在变量名中使用Unicode字符。 在以后的教程中,您将更深入地了解Unicode。
For example, all of the following are valid variable names:
例如,以下所有都是有效的变量名:
But this one is not, because a variable name can’t begin with a digit:
但这不是,因为变量名不能以数字开头:
>>> >>> 10991099 _filed _filed = = False
False
SyntaxError: invalid token
SyntaxError: invalid token
Note that case is significant. Lowercase and uppercase letters are not the same. Use of the underscore character is significant as well. Each of the following defines a different variable:
请注意,这种情况很重要。 小写字母和大写字母不同。 下划线字符的使用也很重要。 以下各项定义了一个不同的变量:
There is nothing stopping you from creating two different variables in the same program called age
and Age
, or for that matter agE
. But it is probably ill-advised. It would certainly be likely to confuse anyone trying to read your code, and even you yourself, after you’d been away from it awhile.
没有什么可以阻止您在同一个程序中创建两个不同的变量,分别称为age
和Age
,或者说是agE
。 但这可能是不明智的。 在您离开代码一段时间之后,肯定会使任何试图读取您的代码的人,甚至您自己都感到困惑。
It is worthwhile to give a variable a name that is descriptive enough to make clear what it is being used for. For example, suppose you are tallying the number of people who have graduated college. You could conceivably choose any of the following:
值得给变量一个足以描述性的名称,以弄清楚它的用途。 例如,假设您要计算大学毕业的人数。 可以想象,您可以选择以下任何一种:
>>> >>> numberofcollegegraduates numberofcollegegraduates = = 2500
2500
>>> >>> NUMBEROFCOLLEGEGRADUATES NUMBEROFCOLLEGEGRADUATES = = 2500
2500
>>> >>> numberOfCollegeGraduates numberOfCollegeGraduates = = 2500
2500
>>> >>> NumberOfCollegeGraduates NumberOfCollegeGraduates = = 2500
2500
>>> >>> number_of_college_graduates number_of_college_graduates = = 2500
2500
>>> >>> printprint (( numberofcollegegraduatesnumberofcollegegraduates , , NUMBEROFCOLLEGEGRADUATESNUMBEROFCOLLEGEGRADUATES ,
,
... ... numberOfCollegeGraduatesnumberOfCollegeGraduates , , NumberOfCollegeGraduatesNumberOfCollegeGraduates ,
,
... ... number_of_college_graduatesnumber_of_college_graduates )
)
2500 2500 2500 2500 2500
2500 2500 2500 2500 2500
All of them are probably better choices than n
, or ncg
, or the like. At least you can tell from the name what the value of the variable is supposed to represent.
与n
或ncg
等类似,它们都是更好的选择。 至少您可以从名称中看出变量的值应该表示什么。
On the other hand, they aren’t all necessarily equally legible. As with many things, it is a matter of personal preference, but most people would find the first two examples, where the letters are all shoved together, to be harder to read, particularly the one in all capital letters. The most commonly used methods of constructing a multi-word variable name are the last three examples:
另一方面,它们不一定都一样易读。 与许多事情一样,这是个人喜好问题,但是大多数人会发现前两个示例比较难读,尤其是所有大写字母中的两个字母都挤在一起。 最后三个示例是构造多字变量名的最常用方法:
- Camel Case: Second and subsequent words are capitalized, to make word boundaries easier to see. (Presumably, it struck someone at some point that the capital letters strewn throughout the variable name vaguely resemble camel humps.)
- Example:
numberOfCollegeGraduates
- Example:
- Pascal Case: Identical to Camel Case, except the first word is also capitalized.
- Example:
NumberOfCollegeGraduates
- Example:
- Snake Case: Words are separated by underscores.
- Example:
number_of_college_graduates
- Example:
- 骆驼案:第二个及以后的单词都大写,以使单词边界更易于查看。 (大概是在某个时候让某人感到震惊,因为整个变量名中的大写字母模糊地类似于驼峰。)
- 示例:
numberOfCollegeGraduates
- 示例:
- Pascal Case:与Camel Case相同,除了第一个单词也大写。
- 示例:
NumberOfCollegeGraduates
- 示例:
- 蛇形案例:单词之间用下划线分隔。
- 示例:
number_of_college_graduates
- 示例:
Programmers debate hotly, with surprising fervor, which of these is preferable. Decent arguments can be made for all of them. Use whichever of the three is most visually appealing to you. Pick one and use it consistently.
程序员以惊人的热情进行了激烈的辩论,其中哪一个更可取。 可以为所有这些提出合理的论据。 使用这三个在视觉上最吸引您的东西。 选择一个并持续使用它。
You will see later that variables aren’t the only things that can be given names. You can also name functions, classes, modules, and so on. The rules that apply to variable names also apply to identifiers, the more general term for names given to program objects.
稍后您将看到,变量并不是唯一可以命名的东西。 您还可以命名函数,类,模块等。 适用于变量名称的规则也适用于标识符,标识符是程序对象名称的更通用术语。
The Style Guide for Python Code, also known as PEP 8, contains Naming Conventions that list suggested standards for names of different object types. PEP 8 includes the following recommendations:
Python代码样式指南 (也称为PEP 8 )包含命名约定 ,该约定列出了针对不同对象类型名称的建议标准。 PEP 8包含以下建议:
- Snake Case should be used for functions and variable names.
- Pascal Case should be used for class names. (PEP 8 refers to this as the “CapWords” convention.)
- Snake Case应该用于函数和变量名。
- Pascal Case应用于类名。 (PEP 8将其称为“ CapWords”约定。)
保留字(关键字) (Reserved Words (Keywords))
There is one more restriction on identifier names. The Python language reserves a small set of keywords that designate special language functionality. No object can have the same name as a reserved word.
标识符名称还有一个限制。 Python语言保留了一小组指定特殊语言功能的关键字。 任何对象都不能与保留字具有相同的名称。
In Python 3.6, there are 33 reserved keywords:
在Python 3.6中,有33个保留关键字:
Python Keywords | Python 关键词 | ||||||
---|---|---|---|---|---|---|---|
False False | def def | if if | raise raise | ||||
None None | del del | import import | return return | ||||
True True | elif elif | in in | try try | ||||
and and | else else | is is | while while | ||||
as as | except except | lambda lambda | with with | ||||
assert assert | finally finally | nonlocal nonlocal | yield yield | ||||
break break | for for | not not | |||||
class class | from from | or or | |||||
continue continue | global global | pass pass |
You can see this list any time by typing help("keywords")
to the Python interpreter. Reserved words are case-sensitive and must be used exactly as shown. They are all entirely lowercase, except for False
, None
, and True
.
您可以随时通过在Python解释器中输入help("keywords")
来查看此列表。 保留字区分大小写,必须完全按照所示使用。 它们都是完全小写的,除了False
, None
和True
。
Trying to create a variable with the same name as any reserved word results in an error:
尝试创建与任何保留字同名的变量会导致错误:
结论 (Conclusion)
This tutorial covered the basics of Python variables, including object references and identity, and naming of Python identifiers.
本教程介绍了Python 变量的基础知识,包括对象引用和标识以及Python标识符的命名。
You now have a good understanding of some of Python’s data types and know how to create variables that reference objects of those types.
您现在对Python的某些数据类型有了很好的了解,并且知道如何创建引用这些类型的对象的变量。
Next, you will see how to combine data objects into expressions involving various operations.
接下来,您将看到如何将数据对象组合成涉及各种操作的 表达式 。
Get Notified: Don’t miss the follow up to this tutorial—Click here to join the Real Python Newsletter and you’ll know when the next installment comes out.
通知您:不要错过本教程的后续内容- 单击此处加入Real Python Newslet ,您将知道下一期的发行时间。
翻译自: https://www.pybloggers.com/2018/06/variables-in-python/
python中的变量