python 桌面备忘录
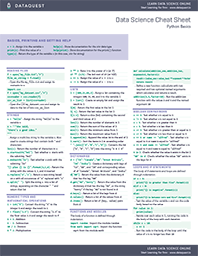
The printable version of this cheat sheet
该备忘单的可打印版本
It’s common when first learning Python for Data Science to have trouble remembering all the syntax that you need. While at Dataquest we advocate getting used to consulting the Python documentation, sometimes it’s nice to have a handy reference, so we’ve put together this cheat sheet to help you out!
第一次学习Python for Data Science时,通常很难记住您需要的所有语法。 在Dataquest时,我们提倡习惯于查阅Python文档 ,有时可以得到一些方便的参考,这很高兴,因此我们整理了这份备忘单,可以为您提供帮助!
If you’re interested in learning Python, we have a free Python Programming: Beginner course which can start you on your data science journey.
如果您对学习Python感兴趣,我们有一个免费的Python编程:入门课程,可以帮助您开始数据科学之旅。
Download a Printable PDF of this Cheat Sheet –>
关键基础知识,打印和获得帮助 (Key Basics, Printing and Getting Help)
x = 3 x = 3 | 3 to the variable 3 赋给变量x x | |
print(x) print(x) | x x 的值 | |
type(x) type(x) | Return the type of the variable x (in this case, int for integer) | 返回变量x 的类型(在这种情况下, int 为int ) |
help(x) help(x) | str data typestr 数据类型的文档 | |
help(print) help(print) | print() functionprint() 函数的文档 |
读取文件 (Reading Files)
| my_file.txt and assign its contents to my_file.txt 并将其内容分配给sing sing |
my_dataset.csv and assign its data to the list of lists my_dataset.csv 并将其数据分配给列表csv_as_list csv_as_list 的列表 |
弦乐 (Strings)
s = "hello" s = "hello" | "hello" to the variable "hello" 分配给变量s s |
| s . Also used to create strings that contain boths 。 也用于创建同时包含" and " 和' characters.' 字符的字符串。 |
len(s) len(s) | s s 的字符数 |
s.startswith("hel") s.startswith("hel") | s starts with the substring s 是否以子字符串"hel" "hel" 开头 |
s.endswith("lo") s.endswith("lo") | s ends with the substring s 是否以子字符串"lo" "lo" 结尾 |
"{} plus {} is {}".format(3,1,4) "{} plus {} is {}".format(3,1,4) | 3 , 3 , 1 , and 1 ,和4 inserted4 插入 |
s.replace("e","z") s.replace("e","z") | s with all occurances of s 返回一个新字符串,所有出现的"e" replaced with "e" 替换为"z" "z" |
s.strip() s.strip() | s with any whitespace at the start and end of the string removeds 的新字符串,并在字符串的开头和结尾删除了空格 |
s.split(" ") s.split(" ") | s into a list of strings, separating on the character s 拆分为字符串列表,以字符" " and return that list" " 分隔并返回该列表 |
数值类型和数学运算 (Numeric Types and Mathematical Operations)
i = int("5") i = int("5") | "5" to the integer "5" 转换为整数5 and assign the result to 5 并将结果分配给i i | |
f = float("2.5") f = float("2.5") | "2.5" to the float value "2.5" 转换为浮点值2.5 and assign the result to 2.5 ,并将结果分配给f f | |
5 + 5 5 + 5 | Addition | 加成 |
5 - 5 5 - 5 | Subtraction | 减法 |
10 / 2 10 / 2 | Division | 师 |
5 * 2 5 * 2 | Multiplication | 乘法 |
3 ** 2 3 ** 2 | 3 to the power of 3 提高到2 (or )2 (或)的幂 | |
27 ** (1/3) 27 ** (1/3) | 3 rd root of 27 (或)的第27 (or )3 根 | |
x += 1 x += 1 | x + 1 to x + 1 的值分配给x x | |
x -= 1 x -= 1 | x - 1 to x - 1 的值分配给x x |
清单 (Lists)
l = [100, 21, 88, 3] l = [100, 21, 88, 3] | 100 , 100 , 21 , 21 , 88 , and 88 ,和3 to the variable 3 至可变l l |
l = list() l = list() | l l |
l[0] l[0] | l l |
l[-1] l[-1] | l l |
l[1:3] l[1:3] | l l 的第二个和第三个值的切片(列表) |
len(l) len(l) | l l 的元素数 |
sum(l) sum(l) | l l 的值之和 |
min(l) min(l) | l l 返回最小值 |
max(l) max(l) | l l 返回最大值 |
l.append(16) l.append(16) | 16 to the end of 16 附加到l l 的末尾 |
l.sort() l.sort() | l in ascending orderl 中的项目进行排序 |
" ".join(["A", "B", "C", "D"]) " ".join(["A", "B", "C", "D"]) | ["A", "B", "C", "D"] into the string ["A", "B", "C", "D"] 转换为字符串"A B C D" "ABCD" |
辞典 (Dictionaries)
d = {"CA": "Canada", "GB": "Great Britain", "IN": "India"} d = {"CA": "Canada", "GB": "Great Britain", "IN": "India"} | "CA" , "CA" , "GB" , and "GB" 和"IN" and corresponding values of of "IN" 以及"Canada" , "Canada" , "Great Britain" , and "Great Britain" 和"India" "India" 对应值创建字典 |
d["GB"] d["GB"] | d that has the key d 中返回具有键"GB" "GB" |
d.get("AU","Sorry") d.get("AU","Sorry") | d that has the key d 具有关键"AU" , or the string "AU" 或字符串"Sorry" if the key "Sorry" 如果键"AU" is not found in "AU" 中找不到d d |
d.keys() d.keys() | d d 返回键列表 |
d.values() d.values() | d d 的值列表 |
d.items() d.items() | (key, value) pairs from d 返回d (key, value) 对的列表 |
模块和功能 (Modules and Functions)
The body of a function is defined through indentation
函数的主体通过缩进定义
import random import random | random random 导入模块 |
from random import random from random import random | random from the module random 从模块random random |
calculate with two required and two optional named arguments which calculates and returns a result.calculate ,该参数计算并返回结果。 | |
addition(3,5,factor=10) addition(3,5,factor=10) | addition function with the values 3 和3 and 5 以及命名参数5 and the named argument 10 运行10 addition 函数 |
布尔比较 (Boolean Comparisons)
x == 5 x == 5 | x is equal to x 是否等于5 5 |
x != 5 x != 5 | x is not equal to x 是否等于5 5 |
x > 5 x > 5 | x is greater than x 是否大于5 5 |
x < 5 x < 5 | x is less than x 是否小于5 5 |
x >= 5 x >= 5 | x is greater than or equal to x 是否大于或等于5 5 |
x <= 5 x <= 5 | x is less than or equal to x 是否小于或等于5 5 |
x == 5 or name == "alfred" x == 5 or name == "alfred" | x is equal to x 是否等于5 or 5 或name is equal to name 等于"alfred" "alfred" |
x == 5 and name == "alfred" x == 5 and name == "alfred" | x is equal to x 是否等于5 and 5 并且name is equal to name 是否等于"alfred" "alfred" |
5 in l 5 in l | 5 exists in the list l 是否存在值l 5 |
"GB" in d "GB" in d | "GB" exists in the keys for d 的键中是否存在值d "GB" |
语句和循环 (Statements and Loops)
The body of if statements and loops are defined through indentation
if语句和循环的主体是通过缩进定义的
| x and run the code body based on the valuex 的值并基于该值运行代码主体 |
l , running the code in the body of the loop with each iteration.l 每个值,并在每次迭代时在循环体内运行代码。 | |
| x is no longer less than x 的值不再小于10 10 |
下载此备忘单的可打印版本 (Download a printable version of this cheat sheet)
翻译自: https://www.pybloggers.com/2017/07/python-cheat-sheet-for-data-science/
python 桌面备忘录