swagger flask
昂首阔步是什么? (What is Swagger?)
Swagger is a simple yet powerful representation of your RESTful API. With the largest ecosystem of API tooling on the planet, thousands of developers are supporting Swagger in almost every modern programming language and deployment environment. With a Swagger-enabled API, you get interactive documentation, client SDK generation and discoverability.
Swagger是RESTful API的简单但功能强大的表示形式。 凭借地球上最大的API工具生态系统,成千上万的开发人员正在几乎每种现代编程语言和部署环境中支持Swagger。 通过启用Swagger的API,您可以获得交互式文档,客户端SDK的生成和可发现性。
什么是Swagger UI? (What is Swagger UI?)
Swagger UI is a dependency-free collection of HTML, Javascript, and CSS assets that dynamically generate beautiful documentation and sandbox from a Swagger-compliant API. Because Swagger UI has no dependencies, you can host it in any server environment, or on your local machine. Head over to the online demo to see what it looks like for any publically accessible Swagger definition.
Swagger UI是HTML,Javascript和CSS资产的无依赖集合,可从兼容Swagger的API动态生成漂亮的文档和沙箱。 由于Swagger UI没有依赖关系,因此您可以将其托管在任何服务器环境或本地计算机上。 转至在线演示,以查看任何可公开访问的Swagger定义的外观。
什么是烧瓶? (du!) (What is Flask? (duhhhh!!))
Flask is a microframework for Python based on Werkzeug, Jinja 2 and good intentions. Why it is awesome? because it is simple yet powerful, talking is cheaper look at the code!
Flask是基于Werkzeug,Jinja 2和良好意图的Python微框架。 为什么很棒? 因为它既简单又强大,所以看代码更便宜!
from flask import Flask, jsonify, request app = Flask(__name__) @app.route('my_awesome_api', methods=['POST']) def my_awesome_endpoint(): data = request.json return jsonify(data=data, meta={"status": "ok"}) app.run()
from flask import Flask, jsonify, request app = Flask(__name__) @app.route('my_awesome_api', methods=['POST']) def my_awesome_endpoint(): data = request.json return jsonify(data=data, meta={"status": "ok"}) app.run()
Run the above script and then you can start posting to the API
运行上面的脚本,然后就可以开始发布到API
什么是弗拉斯格? (What is Flasgger?)
Flasgger is a Flask extension to help the creation of Flask APIs with documentation and live playground powered by SwaggerUI. You can define your API structure using YAML files and Flasgger creates all the specifications for you and you can use the same schema to validate the data.
Flasgger是Flask扩展,可通过SwaggerUI提供的文档和实时操场帮助创建Flask API。 您可以使用YAML文件定义API结构,Flasgger会为您创建所有规范,并且您可以使用相同的架构来验证数据。
GITHUB REPO: https://github.com/rochacbruno/flasgger
GITHUB REPO: https : //github.com/rochacbruno/flasgger
安装它 (Install it)
pip install flasgger
pip install flasgger
建立您的应用程式 (Create your app)
You can put API specs directly in docstrings
您可以将API规范直接放入文档字符串中
试试吧! (Try it!)
Now run your app and access http://localhost:5000/apidocs/index.html and you will play with Swagger UI!
现在运行您的应用程序并访问http:// localhost:5000 / apidocs / index.html ,您将可以使用Swagger UI!
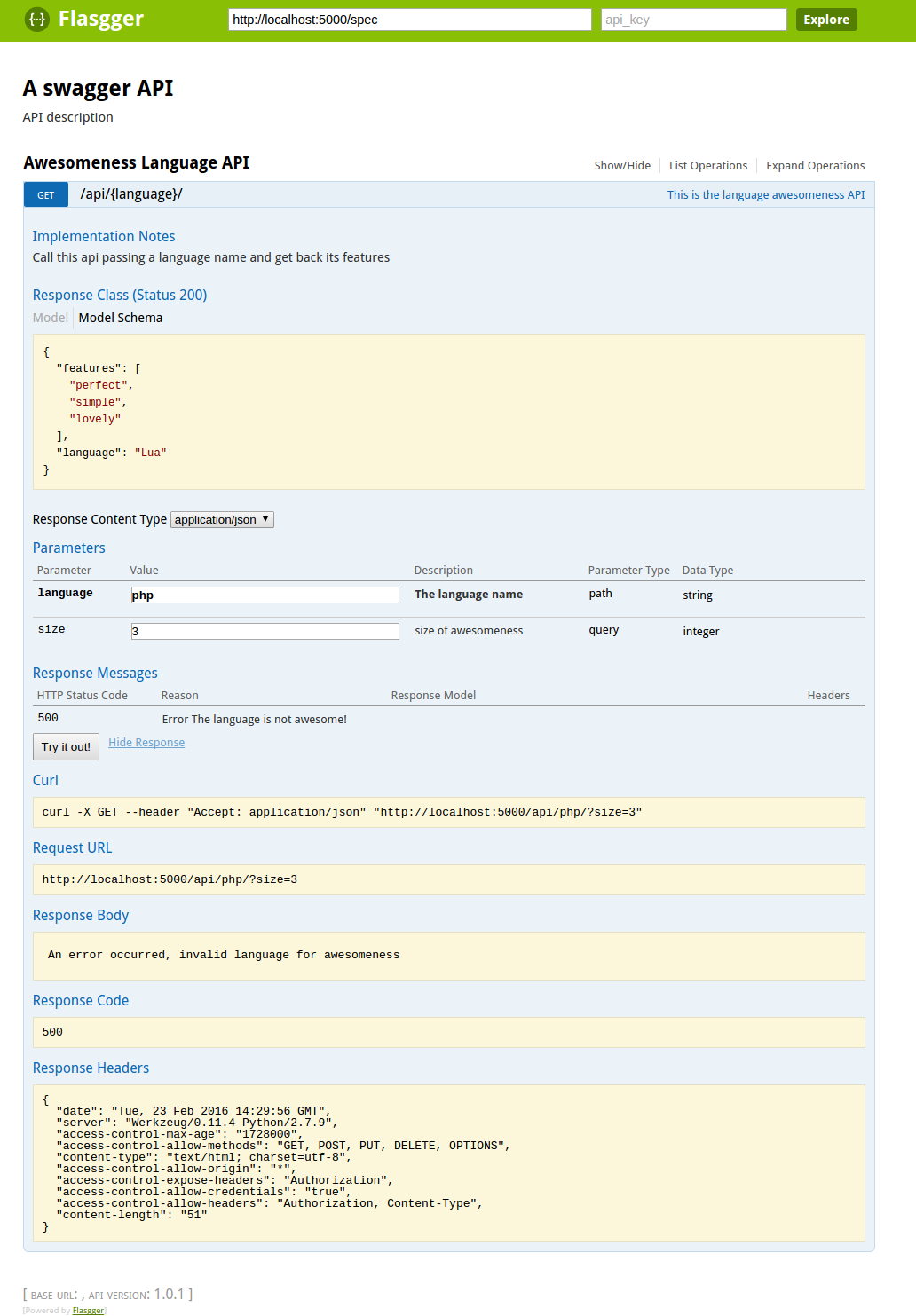
NOTE: All the default urls can be changed in configuration.
注意:可以在配置中更改所有默认URL。
It is also possible to use a separate file for specs
也可以将单独的文件用于规格
在单独的YML文件中创建您的api规范 (Create your api specification in a separated YML file)
In a file index.yml
put the specs definitions
在文件index.yml
放入规格定义
This is the language awesomeness API Call this api passing a language name and get back its features --- tags: - Awesomeness Language API parameters: - name: language in: path type: string required: true description: The language name - name: size in: query type: integer description: size of awesomeness responses: 500: description: Error The language is not awesome! 200: description: A language with its awesomeness schema: id: awesome properties: language: type: string description: The language name default: Lua features: type: array description: The awesomeness list items: type: string default: ["perfect", "simple", "lovely"]
This is the language awesomeness API Call this api passing a language name and get back its features --- tags: - Awesomeness Language API parameters: - name: language in: path type: string required: true description: The language name - name: size in: query type: integer description: size of awesomeness responses: 500: description: Error The language is not awesome! 200: description: A language with its awesomeness schema: id: awesome properties: language: type: string description: The language name default: Lua features: type: array description: The awesomeness list items: type: string default: ["perfect", "simple", "lovely"]
and then change the code to read from it using swag_from
decorator
然后更改代码以使用swag_from
装饰器从中读取
验证 (validation)
If you put the specs in a separate file it is also possible to use the same specs to validate the input
如果将规格放在单独的文件中,则也可以使用相同的规格来验证输入
from flasgger.utils import swag_from, validate, ValidationError @app.route('/api/<string:language>/', methods=['GET']) @swag_from('index.yml') def index(language): ... try: validate(data, 'awesome', 'index.yml', root=__file__) except ValidationError as e: return "Validation Error: %s" % e, 400 ...
from flasgger.utils import swag_from, validate, ValidationError @app.route('/api/<string:language>/', methods=['GET']) @swag_from('index.yml') def index(language): ... try: validate(data, 'awesome', 'index.yml', root=__file__) except ValidationError as e: return "Validation Error: %s" % e, 400 ...
更多信息 (More information)
You can find more information and some examples in the github repository
您可以在github存储库中找到更多信息和一些示例
有助于 (Contribute)
翻译自: https://www.pybloggers.com/2016/02/flasgger-api-playground-with-flask-and-swagger-ui/
swagger flask