Github Actions are a new feature from everyone's favorite code tool. While they take a little getting used to, they are very powerful tools for CI (continuous integration) and other checks on your pull requests.
Github Actions是每个人都喜欢的代码工具的新功能。 尽管它们已经习惯了一些,但它们是用于CI(连续集成)和对拉取请求进行其他检查的功能非常强大的工具。
Here, I'll talk about how to use Github Actions for calling webhooks. And as you know, once you can call a webhook, the internet is your oyster.
在这里,我将讨论如何使用Github Actions调用webhook。 如您所知,一旦您可以呼叫网络钩子,互联网就是您的牡蛎。
为什么不使用旧的网络钩子? (Why not the old webhooks?)
Now, you may be saying: "Github already has webhooks, why bother with actions?" The answer is simple: version control.
现在,您可能会说:“ Github已经存在Webhooks,为什么还要烦恼操作?” 答案很简单: 版本控制 。
If you work with anyone else on your code base, you want to be able to track how changes to configuration have come about, and who is responsible.
如果您与代码库中的其他任何人一起工作,则希望能够跟踪如何进行配置更改以及由谁负责。
With basic Github settings, you don't know these things - someone sets and configures a webhook. Maybe it fails one day, then what?
使用基本的Github设置,您将不知道这些事情-有人设置并配置了一个Webhook。 也许有一天失败了,那又如何呢?
With Github Actions, you don't have to leave your text editor to see what happens when you push your code.
使用Github Actions,您不必离开文本编辑器即可查看在推送代码时会发生什么。
The other reason is that there is a world of things you can do from Github Actions, and some ideas are below. I needed webhooks for a recent project, but there are features for running tests, gathering code coverage, linting, and more.
另一个原因是,您可以通过Github Actions做很多事情,下面是一些想法。 对于最近的项目,我需要使用webhooks,但是有一些功能可用于运行测试,收集代码覆盖范围,整理文件等。
Seeing as so many of us use Github every day, it can't hurt to get familiar with this new tool.
看到我们每天都有如此多的人使用Github,熟悉这个新工具不会有什么坏处。
你的第一个动作 (Your first action)
So, let's get started. The first thing you need to do is create a .github > workflows
folder. Inside of it, we'll put our actions. It doesn't matter what you call the file - GitHub will pick up all the actions you place in this special folder.
因此,让我们开始吧。 您需要做的第一件事是创建一个.github > workflows
文件夹。 在其中,我们将采取行动。 不管文件叫什么都无所谓-GitHub会选择您放置在此特殊文件夹中的所有操作。
Here are the contents of my webhook file. I have a "Test pedant" API endpoint that checks the files of my PR and leaves a pedantic comment if I haven't written any tests.
这是我的webhook文件的内容。 我有一个“ Test pedant” API端点,它检查我的PR文件并在没有编写任何测试的情况下留下书呆子注释。
# This is a basic workflow that is manually triggered
name: Test reminder
# Controls when the action will run. Workflow runs when manually triggered using the UI or API.
on:
# Trigger the workflow on push or pull request,
# but only for the master branch
pull_request:
branches: [ main ]
# A workflow run is made up of one or more jobs that can run sequentially or in parallel
jobs:
test_commentary:
# The type of runner that the job will run on
runs-on: ubuntu-latest
# Steps represent a sequence of tasks that will be executed as part of the job
steps:
# Runs a single command using the runners shell
- name: Webhook
uses: distributhor/workflow-webhook
with:
url: "http://amberisgreat.ngrok.io/api/test_pedant"
json: '{ "repository": "${{github.event.repository.full_name}}", "number": "${{github.event.number}}", "created_at": "${{github.event.pull_request.created_at}}", "updated_at": "${{github.event.pull_request.updated_at}}" }'
From top to bottom:
从上到下:
First we give a name to the action (
Test reminder
).首先,我们为操作命名(
Test reminder
)。Then we specify when we want it to run (
on
). You don't have to choose branches. If you want it for all PRs, just doon: [pull_request]
. Github has a huge list of events that can trigger an action.然后,我们指定我们希望它在何时运行(
on
)。 您不必选择分支。 如果您希望所有PR都使用它,只需执行on: [pull_request]
。 Github有大量事件可以触发一个动作 。jobs
is a list of the jobs. Where I havetest_commentary
you would put a key for your job (it can be anything). Your job probably runs onubuntu-latest
but check the job itself. A job might have multiple steps. Mine has one.jobs
是jobs
列表。 在我有test_commentary
您会为您的工作放置钥匙(可以是任何东西)。 您的作业可能在ubuntu-latest
上运行,但请检查作业本身。 一项工作可能有多个步骤。 我的有一个。Give your step a
name
(this can also be anything)给您的步骤
name
(也可以是任何东西)uses
refers to where the code is stored. (You can write your own actions if you want!)uses
是指代码的存储位置。 (如果需要,您可以编写自己的操作!)Different actions take different inputs - this one uses
with
and the input keys.不同的动作会采用不同的输入-这个
with
和键一起使用。
I'm using a webhook function from "distributhor". Github doesn't have an official webhook job yet, but they might by the time you read this.
我正在使用“ distributhor”的webhook函数 。 Github还没有正式的网络挂钩工作,但是当您阅读本文时,他们可能会正式。
This action takes at least one input: url
, the endpoint you want to hit with this action. json
refers to the data you are going to send.
该操作至少需要一个输入: url
,您要通过此操作命中的端点。 json
是指您要发送的数据。
Github Actions有效负载 (The Github Actions payload)
This information was a little tricky to hunt down in the Github Actions docs.
在Github Actions文档中寻找这些信息有些棘手。
The payload for a Github Action is nested under github.event
. You also need to know which action you are pushing data from - as there are various keys available on event
depending on which action you are referencing (listed as action
in the payload docs).
Github Action的有效载荷嵌套在github.event
下。 您还需要知道要从哪个操作推送数据-因为根据您引用的action
(在有效负载文档中列为action
), event
上有各种可用的键。
The relevant docs are here.
相关文档在这里 。
My library wouldn't let me just shove the whole event
into my webhook - that's why you see the keys specifically pulled out. Maybe the action you write will take the whole event
(and then you can tell me about it!)
我的图书馆不允许我将整个event
推送到我的Webhook中-这就是为什么您看到专门拉出的键的原因。 也许您编写的动作会占用整个event
(然后您可以告诉我!)
Github动作...动作 (Github Actions in... action)
And that is everything you need! As long as your webhook responds with a 200, your action will get a green check.
这就是您需要的一切! 只要您的Webhook响应为200,您的操作就会获得绿色对勾。
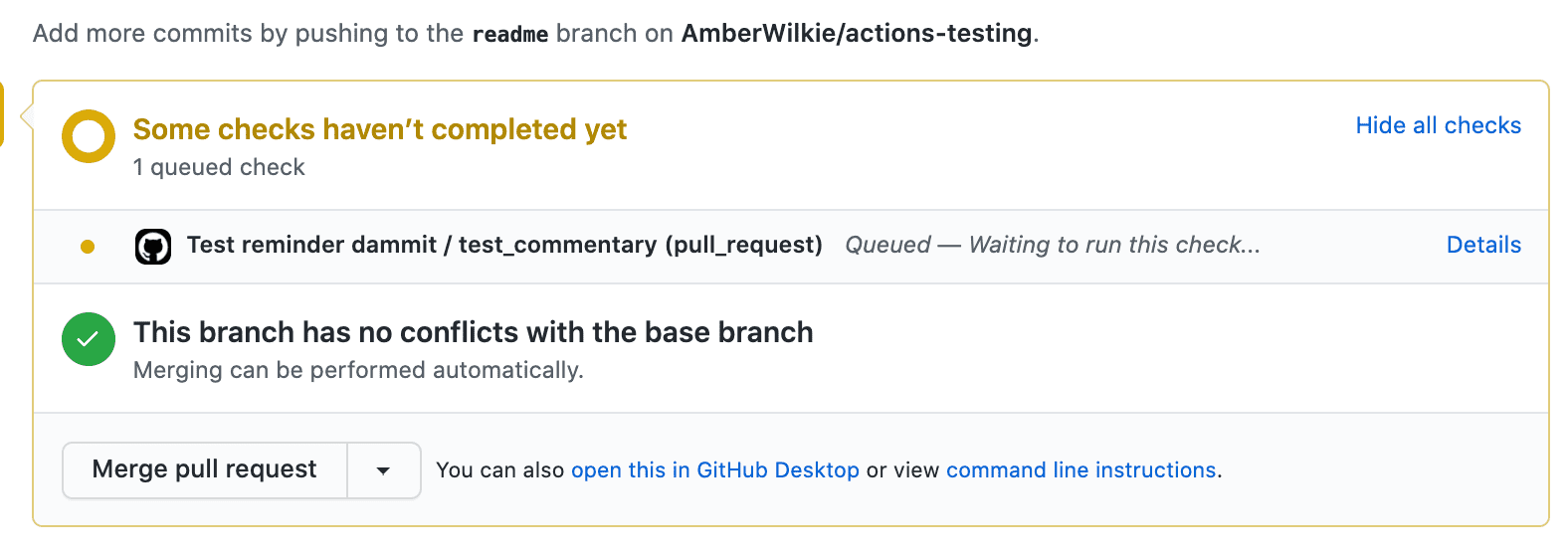
If your action fails, you can check out what went wrong in the Actions tab. Mine fails because I don't have my ngrok tunnel running anymore.
如果操作失败,则可以在“操作”选项卡中检查出了什么问题。 我的失败了,因为我的ngrok隧道不再运行了。
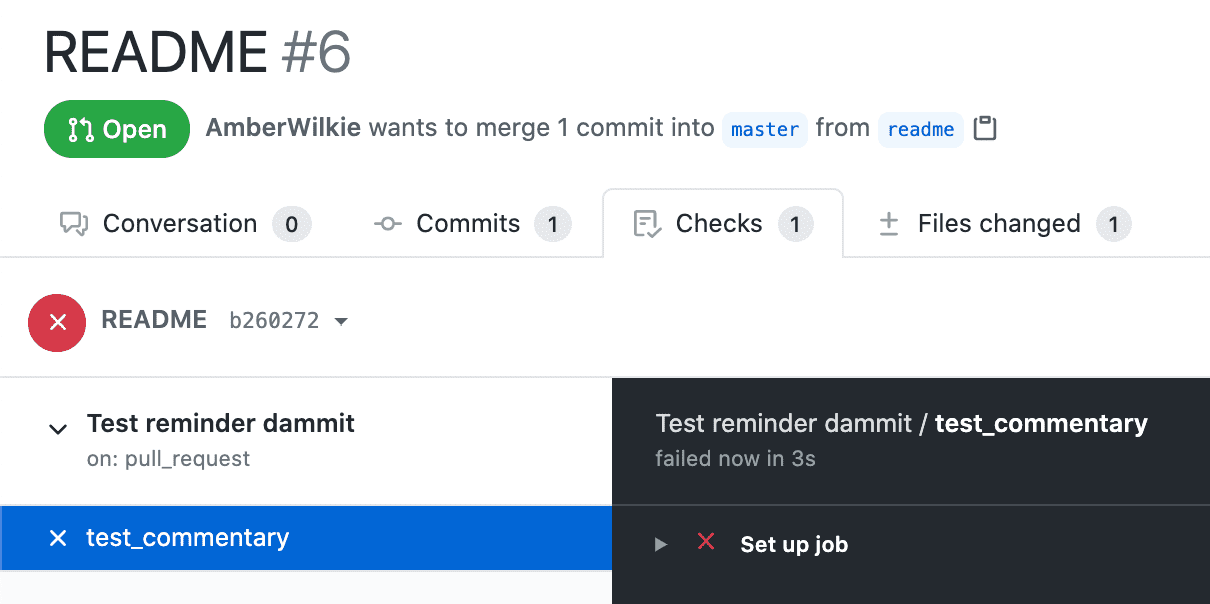
奖励:如何获取和使用ngrok (Bonus: how to get and use ngrok)
Unrelated to this feature, it's very useful to be able to open up a locally-hosted port for external services.
与此功能无关,能够为外部服务打开本地托管的端口非常有用。
In this case, I want my Github Action to trigger my localhost, so that I can test the payload and execution of my webhook. When I put this into production, I'll replace the url
with the production version of the code.
在这种情况下,我希望我的Github Action触发我的本地主机,以便可以测试有效负载和Webhook的执行。 将其投入生产后,我将用代码的生产版本替换url
。
Ngrok to the rescue! Ngrok is a service that creates a tunnel to your localhost. It's free, too.
Ngrok进行救援! Ngrok是一项服务,可创建到本地主机的隧道。 它也是免费的。
My company pays for a few of us to have reserved URLs (because web-to-mobile development things) but for your purposes here, free is great.
我公司为我们中的一些人支付了保留URL的费用(因为通过Web到移动设备进行开发),但是出于您在这里的目的,免费是很棒的。
Just brew install ngrok
(or whatever package manager you use), spin up your localhost serving the webhook code, and ngrok http <your-port>
.
只需brew install ngrok
(或您使用的任何软件包管理器),启动提供webhook代码的localhost,然后ngrok http <your-port>
。
Now you've created a public connection to your localhost. Github will be able to access it and you can test your execution. Just be aware, the free tunnels expire after a while and will require a new URL.
现在,您已经创建了到本地主机的公共连接。 Github将能够访问它,您可以测试执行情况。 请注意,免费隧道会在一段时间后过期,并且需要一个新的URL。
现在怎么办? (What now?)
If this gives you some cool ideas for what you can do with changes to your Github, you ought to check out the Actions Marketplace.
如果这为您提供了一些不错的想法,说明您可以对Github进行更改,那么您应该查看Actions Marketplace 。
In it, you'll find that some very clever folks have dreamed up all kinds of things to happen when events are triggered in your Github. Here you'll find auto-linters, Jira connections, deployment tools and much, much more.
在其中,您会发现一些非常聪明的人梦想着在Github中触发事件时发生的各种事情。 在这里,您会发现自动分类,Jira连接,部署工具以及更多其他内容。
Happy actioning!
行动愉快!
翻译自: https://www.freecodecamp.org/news/how-to-use-github-actions-to-call-webhooks/