Before we dive into variable Scope discussion, let me explain what a function is in JavaScript?
在深入讨论可变作用域之前,让我解释一下JavaScript中的函数是什么 ?
JavaScript中的函数 (Functions in JavaScript)
Basically, subprograms for a particular task is a function and calling of these functions is known as invoking. It’s convenient to have functions and it’s a good programming practice. They usually have a return value.
基本上,用于特定任务的子程序是一个函数,对这些函数的调用称为调用。 拥有函数很方便,并且是良好的编程习惯。 它们通常具有返回值。
To declare a function we need a name, function keyword, and it may or may not have parameter(s).
要声明一个函数,我们需要一个name , function关键字,并且它可能有也可能没有参数 。
Syntax: We can declare function with these ways:
语法:我们可以通过以下方式声明函数:
Type A)
类型A)
function functionName (param1, param2, param3, ...) {
//function body
}
Type B)
B型)
let funtionNameTwo = function (param1, param2, param3, ...) {
//function body
}
Type C)
C型)
var funtionNameThree = function (param1, param2, param3, ...) {
//function body
}
As here we have three ways to declare a function, my personal favorite is type B, so I will use it in this article, you can use whatever you like.
由于这里我们有三种方法来声明一个函数,我个人最喜欢的类型是B ,所以我将在本文中使用它,您可以随意使用。
Return value: It's a value that a function returns value to the system on completion.
返回值:函数在完成时将值返回给系统的值。
Let’s try some examples...
让我们尝试一些例子...
let tempConversion = function(celsius){
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
}
todayTemp = tempConversion(45)
yesterdayTemp = tempConversion(38)
tomorrowTemp = tempConversion(40)
console.log(`Temp for 3 consecutive days are ${yesterdayTemp}F, ${todayTemp}F, ${tomorrowTemp}F`)
Note:
注意:
If you noticed in the above code I used ' ' (you can find this symbol at the left of your number 1 key above tab button) symbol instead of inverted commas (" ") because we can use any variable's value in ${variableName} manner.
如果您在上面的代码中注意到我使用了'' (您可以在选项卡按钮上方的数字1键的左侧找到此符号),而不是使用反斜杠( “” ),因为我们可以在$ {variableName}中使用任何变量的值方式。
If you notice at some places I declared a variable with var keyword while some with let keyword, so why using different keywords for declaring a variable?
如果您在某些地方注意到我用var关键字声明了变量,而有些地方则用let关键字声明了,那么为什么要使用不同的关键字来声明变量呢?
Let’s see the code snippet that I run for you...
让我们看看我为您运行的代码片段...
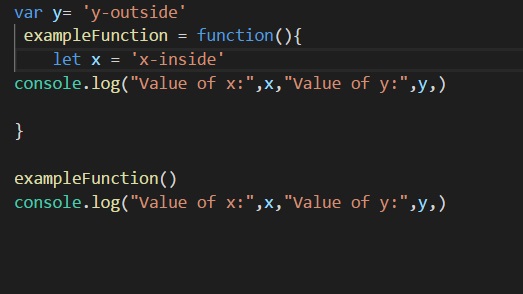
In the above sample, we have both var and let keywords used, one inside a function and another outside side of it, let's check its output.
在上面的示例中,我们同时使用了var和let关键字,一个在函数内,另一个在函数外,让我们检查其输出。
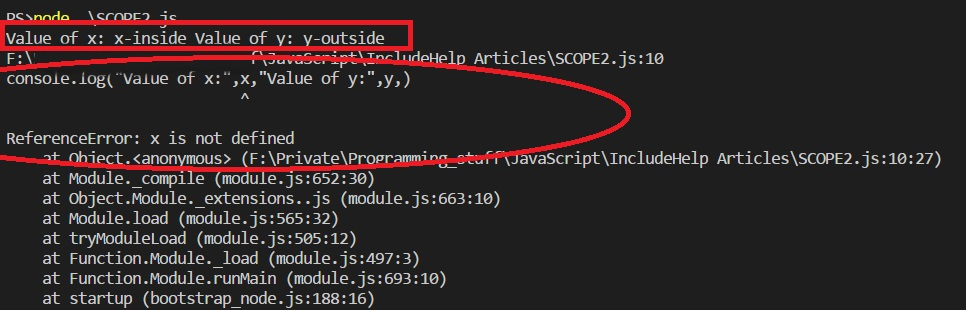
OOPS!!!! It’s some kind of error, now we have to see what it is. There is an output that is produced from the invoking of exampleFunction() so log function outside of our function.
糟糕!!! 这是某种错误,现在我们必须看看它是什么。 调用exampleFunction()会产生一个输出,因此将函数记录在函数之外。
In the first highlighted box, it looks like we got something as output, it says.
它说,在第一个突出显示的框中,看起来我们得到了一些输出。
Value of x: x-inside Value of y: y-outside.
x的值:x-内部 y的值:y-外部 。
In the second highlighted section, there is an error which says ReferenceError: x is not defined. Wait a minute we declared x, so happened to it?
在第二个突出显示的部分中,有一个错误,指出ReferenceError:x未定义 。 等一下我们声明了x ,发生了什么?
Well, the answer is simple when we use let keyword the variable is a local variable and if we use var keyword then the variable is the global scope.
好吧,当我们使用let关键字,变量是局部变量,而如果使用var关键字,则变量是全局范围,答案很简单。
Well, this is something new that I haven't told you about, so let discuss.
好吧,这是我还没有告诉您的新东西,所以让我们讨论一下。
JavaScript的范围 (Scope in JavaScript)
Scope simply refers to where a variable or a function is accessible.
范围只是指在哪里可以访问变量或函数。
JavaScript范围的类型 (Types of Scopes in JavaScript)
Global Scope: When a variable or a function is global then it can be accessed anywhere in our code.
全局范围:当变量或函数是全局变量时,可以在我们代码的任何位置访问它。
Local Scope: When an anything can only be accessed inside a block or a function.
Local Scope:当任何内容只能在块或函数内部访问时。
Variable also have a function scope that is those which are passed as an argument in function parameter and the variable can be accessed within the function.
变量还具有一个函数范围 ,该范围是作为函数参数中的参数传递的,并且可以在函数内访问该变量。
Let’s understand scopes better with a problem. The problem is the KINGS territory problem.
让我们更好地了解问题所在的范围。 问题是国王的领土问题 。
国王领土问题 (KINGS territory problem)
let king = 'foo' // line 1
if(true) {
let king = 'bar' //line 2
if(true){
let king = 'IncludeHelp' //line 3
console.log(king)
}
}
if(true){ //block 1
console.log("King at out side is:",king);
}
In the above code, we declared king in line 1 with let keyword and it will act as a global variable as it is declared in global domain hence in subsequent blocks it will be accessible.
在上面的代码中,我们在第1行中使用let关键字声明了king,它将在全局域中声明时用作全局变量,因此在后续块中将可以访问它。
Here is the task for you, in the above code try some variation like commenting line 1, 2, 3, 4 or block 1 and run it and see what happens.
这是您的任务,在上面的代码中尝试一些变体,例如注释第1、2、3、4或第1行,然后运行它,看看会发生什么。
翻译自: https://www.includehelp.com/code-snippets/understanding-functions-and-scopes-in-javascript.aspx