Here, we'll use the DOM to change CSS styles dynamically of a webpage. As a prerequisite, you must know what DOM is, what are DOM elements and how we get a reference to them? I suggest you go through HTML DOM and DOM Elements article in case you're unfamiliar with the topics.
在这里,我们将使用DOM来动态更改网页CSS样式 。 作为前提条件,您必须知道什么是DOM , 什么是DOM元素以及我们如何获得对它们的引用? 如果您不熟悉主题,建议您阅读HTML DOM和DOM Elements文章。
We know that DOM allows us to capture the DOM elements using CSS Selectors and we can change the content on the web page. However, interestingly we can also change the CSS styles of DOM elements dynamically using DOM manipulation.
我们知道DOM允许我们使用CSS选择器捕获DOM元素,并且我们可以更改网页上的内容。 但是,有趣的是,我们还可以使用DOM操作动态更改DOM元素CSS样式。
Create an "index.html" file and paste the following code. We'll be using this as our HTML template.
创建一个“ index.html”文件并粘贴以下代码。 我们将使用它作为我们HTML模板。
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>DOM CSS</title>
</head>
<body>
<h1>DOM CSS</h1>
<h3>Today we'll be using the DOM CSS to dynamically change styles</h3>
<div class="container">
<p>We'll be using the CSS Selectors for capturing DOM Elements</p>
<span>This is the starter template</span>
</div>
<div class="content">
<h3>Here is some lorem ipsum for you</h3>
<p>Lorem ipsum dolor, sit amet consectetur adipisicing elit. Dolor, porro. Explicabo necessitatibus neque illo provident consequatur. Cumque,earum delectus tempora neque illum pariatur autem dolorem nam doloribus ut ipsa expedita!</p>
</div>
<script>
</script>
</body>
</html>
Now, let's add some basic styles. Create a "styles.css" file and hook it up with your "index.html"
<link rel="stylesheet" href="styles.css">
Now add the following styles so that we have something to change,
HTML Output
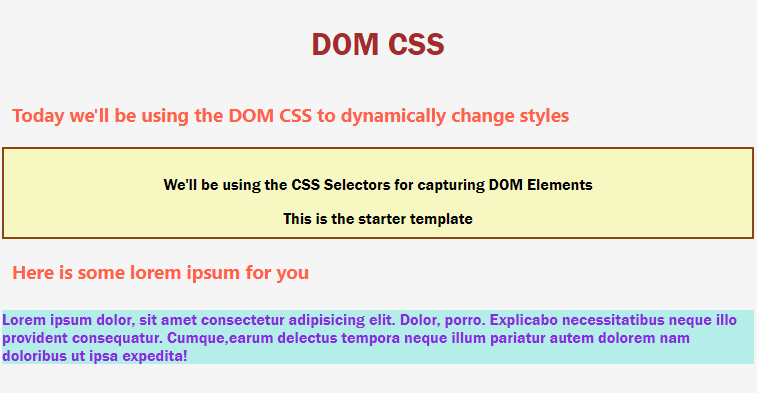
Alright. Now our page looks a bit colorful. Let's get a reference to all our DOM Elements using the querySelector() method.
Cool, now we have a reference to the title, our introduction text and our content div.
Let's first simply change the color of our title.
title.style.color = 'green';
HTML Output
Our title just changed color! You can also inspect it and see in the "styles.css" file on the developer tools that our h1 has a color of green assigned to it unlike earlier.
Let's try to increase the font-size of our "lorem ipsum".
content.style.fontSize = '44px';
HTML Output
Our lorem ipsum text just changed its size to 44px. You now have a basic idea of how to change CSS styles using DOM manipulation? We can also add and remove classes. Let's do one more example,
You can see that our intro text has a class container that we have styled differently. We can remove this class using the following,
introText.classList.remove('container');
HTML Output
Now our styles for the container class are removed from the introText. This way we can remove CSS classes to change CSS styles of DOM elements. Let's add it back.
introText.classList.add('container');
HTML Output
And our styles are back again! Thus we can also add classes to change CSS styles of our DOM elements.
Alternately, we can simply write
introText.classList.toggle('container');
This will add the container class if not present and remove it if it's present already.
现在,让我们添加一些基本样式。 创建一个“ styles.css”文件,并将其与“ index.html”挂钩
现在添加以下样式,以便我们进行一些更改,
body {
background : whitesmoke ;
}
h1 {
margin : 5 px auto ;
padding : 20 px ;
color : brown ;
font-family : ' Franklin Gothic Medium ' , ' Arial Narrow ' , Arial , sans-serif ;
text-align : center ;
}
h3 {
margin : 10 px auto ;
padding : 10 px ;
color : tomato ;
font-family : ' Segoe UI ' , Tahoma , Geneva , Verdana , sans-serif ;
}
. container {
text-align : center ;
padding : 10 px ;
background : rgba ( 255 , 255 , 0 , 0.212 ) ;
border : 2 px solid saddlebrown ;
font-family : ' Franklin Gothic Medium ' , ' Arial Narrow ' , Arial , sans-serif ;
}
. content p {
color : blueviolet ;
background : rgba ( 64 , 224 , 208 , 0.356 ) ;
font-family : ' Franklin Gothic Medium ' , ' Arial Narrow ' , Arial , sans-serif ;
}
HTML输出
好的。 现在我们的页面看起来有点丰富多彩。 让我们使用querySelector()方法获得对所有DOM元素的引用。
< script >
const title = document . querySelector ( ' h1 ' ) ;
const introText = document . querySelector ( ' .container ' ) ;
const content = document . querySelector ( ' .content p ' ) ;
< / script >
很酷,现在我们可以参考标题 ,介绍文字和内容div 。
首先让我们简单地更改标题的颜色。
HTML输出
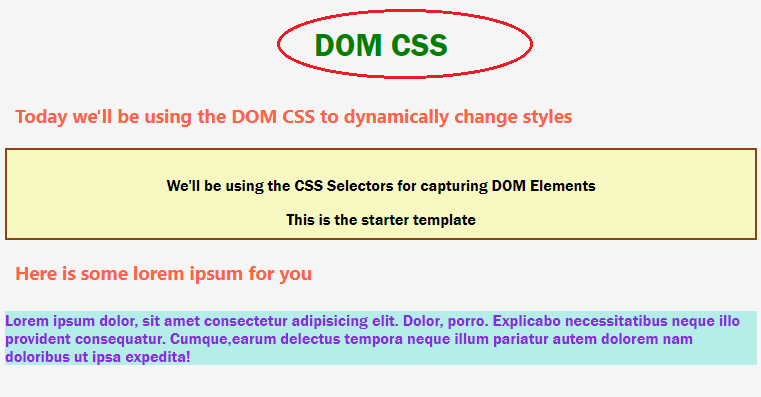
我们的标题刚刚变色! 您还可以对其进行检查,并在开发人员工具上的“ styles.css”文件中看到,我们的h1被分配了绿色,这与之前的情况不同。
让我们尝试增加“ lorem ipsum”的字体大小 。
HTML输出
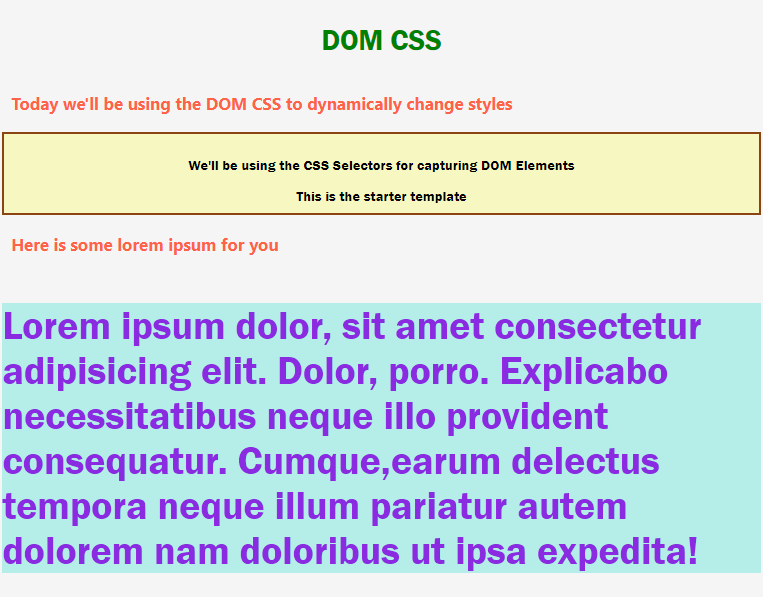
我们的lorem ipsum文本刚刚将其大小更改为44px 。 您现在对如何使用DOM操作更改CSS样式有一个基本的了解? 我们还可以添加和删除类。 让我们再举一个例子
您可以看到我们的介绍文本具有一个样式不同的类容器。 我们可以使用以下方法删除此类,
HTML输出
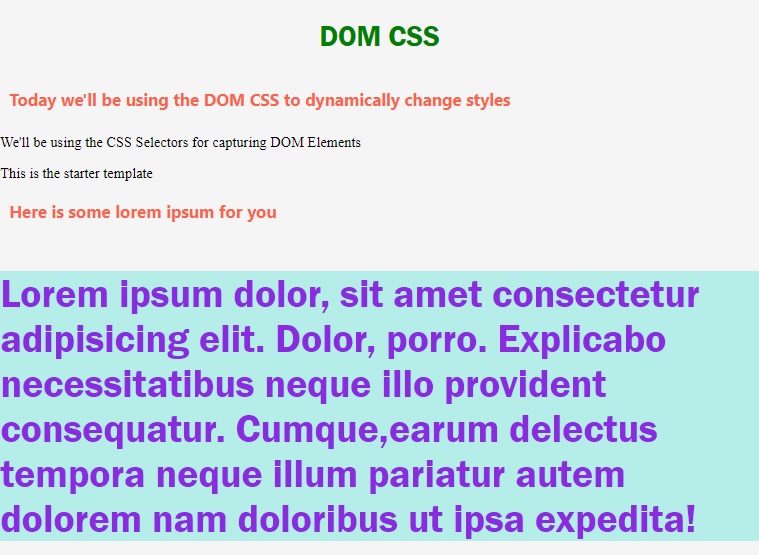
现在,我们从introText中删除了容器类的样式 。 这样,我们可以删除CSS类来更改DOM元素CSS样式。 让我们重新添加。
HTML输出
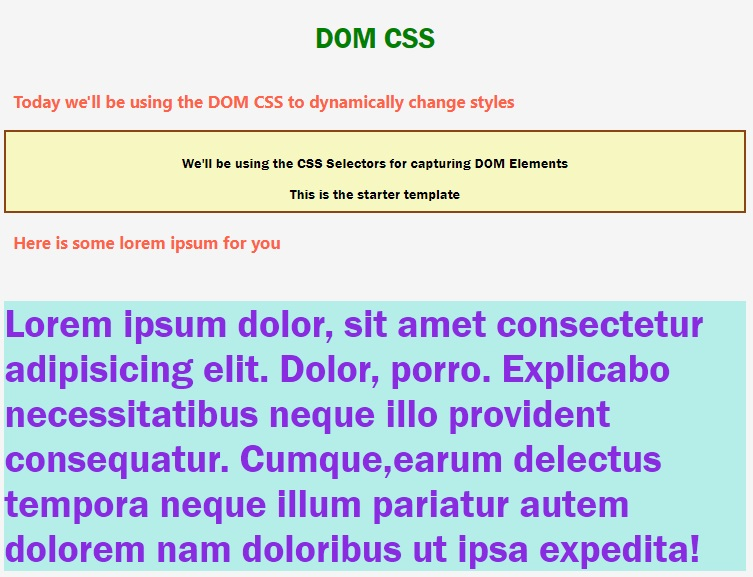
我们的风格又回来了! 因此,我们还可以添加类来更改DOM元素CSS样式。
或者,我们可以简单地写
如果不存在,它将添加容器类,如果已经存在,则将其删除。
最佳面试编码问题/挑战
翻译自: https://www.includehelp.com/code-snippets/javascript-dom-css.aspx