javascript对话框
JavaScript对话框 (JavaScript Dialog Boxes)
Dialog boxes are a great way to provide feedback to the user when they submit a form. In JavaScript, there are three kinds of Dialog boxes,
对话框是向用户提交表单时提供反馈的好方法。 在JavaScript中,共有三种对话框,
Alert
警报
Confirm
确认
Prompt
提示
1) Alert
1)警报
An alert box acts as a warning popup for the user to indicate that something has been entered incorrectly. For example, if you had to enter your email and you didn't match the right email pattern like missed an '@' or something. They give an Ok button to proceed and logically the user is redirected to the same form so they can enter those fields again.
警报框充当用户的警告弹出窗口,指示输入的内容不正确。 例如,如果您必须输入电子邮件,但没有匹配正确的电子邮件模式,例如缺少“ @”之类的内容。 他们提供一个“确定”按钮以继续操作,并且在逻辑上将用户重定向到相同的表单,以便他们可以再次输入这些字段。
2) Confirm
2)确认
This box verifies if the field or fields entered by the user is accepted or not. When a confirm box opens up, the user will have two options 'Ok' and 'Cancel' to proceed further. The click events on these buttons are associated with a property of the window.confirm() method that returns true when the user clicks 'Ok' and false otherwise. Imagine that you are doing an online transaction through internet banking and you have entered some credential bank details. Confirm boxes are a way to let the user know that they have filled out a form with important details and can recheck them if they want.
此框验证用户输入的一个或多个字段是否被接受。 当打开确认框时,用户将具有两个选项“确定”和“取消”以继续进行操作。 这些按钮上的单击事件与window.confirm()方法的属性相关联,该方法在用户单击“确定”时返回true,否则返回false。 想象一下,您正在通过互联网银行进行在线交易,并且已经输入了一些凭证银行详细信息。 确认框是一种让用户知道他们已经填写了重要细节的表格的方式,并且可以根据需要重新检查。
3) Prompt
3)提示
Prompt boxes are very similar to confirm boxes with the only difference that they have an input value that the user can be asked to enter. For example, prompt boxes can be helpful when you're filling out a form where only on entering some basic details you are asked for more confidential details. The latter can be hooked up to the prompt's input field. The user is then given 'Ok' and 'Cancel' buttons which work the same way as they did for a Confirm box.
提示框与确认框非常相似,唯一的区别是提示框具有可以要求用户输入的输入值。 例如,当您填写表格时,仅在输入一些基本详细信息时才要求您提供更多机密详细信息,提示框可能会有所帮助。 后者可以连接到提示的输入字段。 然后,向用户提供“确定”和“取消”按钮,其作用与确认框相同 。
To demonstrate, let's create a simple sign-in form that utilizes all the three Dialog boxes.
为了演示,让我们创建一个使用所有三个对话框的简单登录表单。
Example: (index.html)
示例:(index.html)
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Dialog Boxes in Forms</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
</head>
<style>
body {
background: skyblue;
}
form {
position: relative;
top: 60px;
left: 40px;
padding: 40px;
border: 2px solid slategray;
background: slategray;
}
button {
position: relative;
left: 350px;
color: skyblue;
}
</style>
<body>
<div class="container">
<form>
<h3 class="white-text">Welcome to gingo! Let's sign you in :)</h2>
<p class="white-text">Lorem ipsum dolor sit amet consectetur adipisicing elit. Natus, eligendi corporis quam, similique ipsum expedita,
cum impedit culpa autem ea velit. Hic voluptas libero quasi neque, expedita saepe ex voluptate!</p>
<label for="name" class="white-text">
<input type="text" id="name">Enter your name
</label>
<label for="email" class="white-text">
<input type="text" id="email">Enter your email
</label>
<br><br><br>
<button class="btn submit">Proceed</button>
</form>
</div>
</body>
<script>
</script>
</html>
Output
输出量
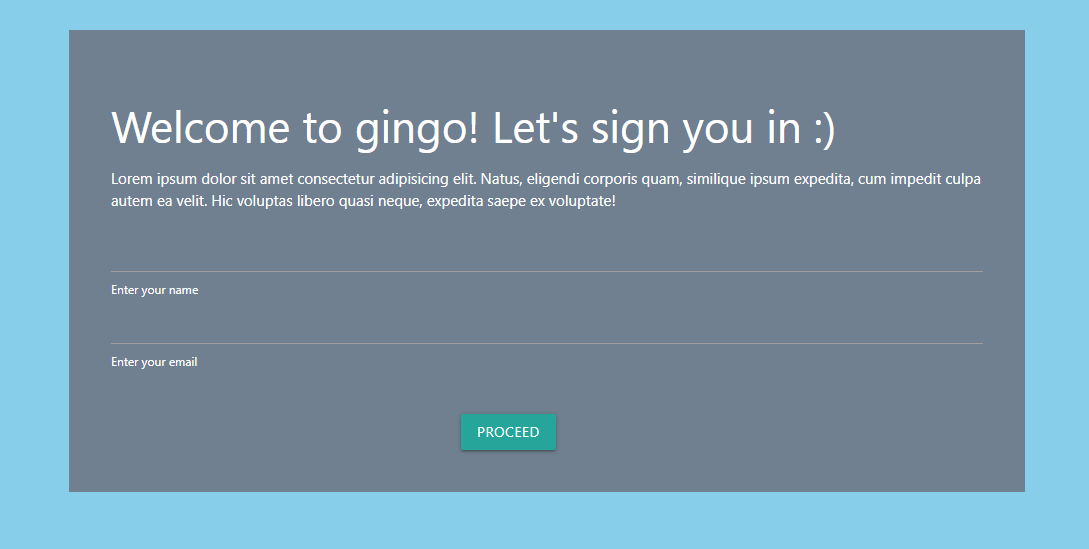
Now that we have a basic form setup, let's think about how we're going to use the three Dialog boxes to provide some feedback. We can implement alert to check if the user has filled the form so that they don't submit an empty form so let's do this first,
现在我们有了基本的表单设置,让我们考虑一下如何使用三个对话框提供一些反馈。 我们可以实施警报,以检查用户是否填写了表单,这样他们就不必提交空白表单,因此我们首先进行操作,
<script>
document.querySelector('.submit').addEventListener('click', e => {
e.preventDefault();
const name = document.querySelector('#name').value;
const email = document.querySelector('#email').value;
if (name == '' || email == '')
alert('Please fill all the fields!')
})
</script>
Output
输出量
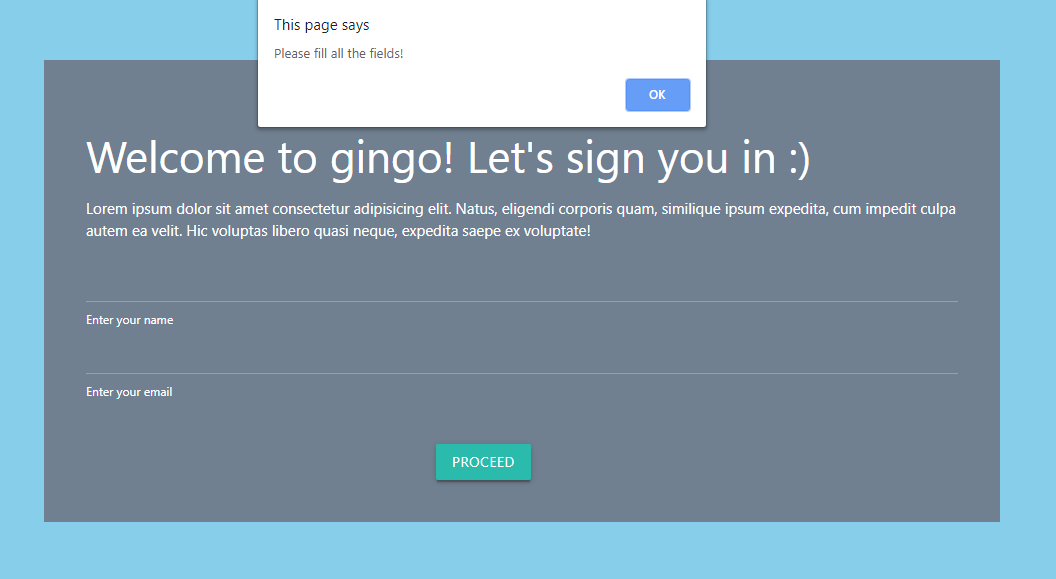
If the user did enter some value, let's give a prompt box to the user for entering a special security code and if you did enter it, we'll open a confirm Dialog box,
如果用户确实输入了一些值,我们将为用户提供一个输入特殊密码的提示框,如果您输入了它,我们将打开一个确认对话框,
<script>
document.querySelector('.submit').addEventListener('click', e => {
e.preventDefault();
const name = document.querySelector('#name').value;
const email = document.querySelector('#email').value;
if (name == '' || email == '')
alert('Please fill all the fields!')
else {
prompt('Enter your special security')
if (prompt() != null)
confirm('Are you sure you want to proceed?')
}
})
</script>
Output
输出量
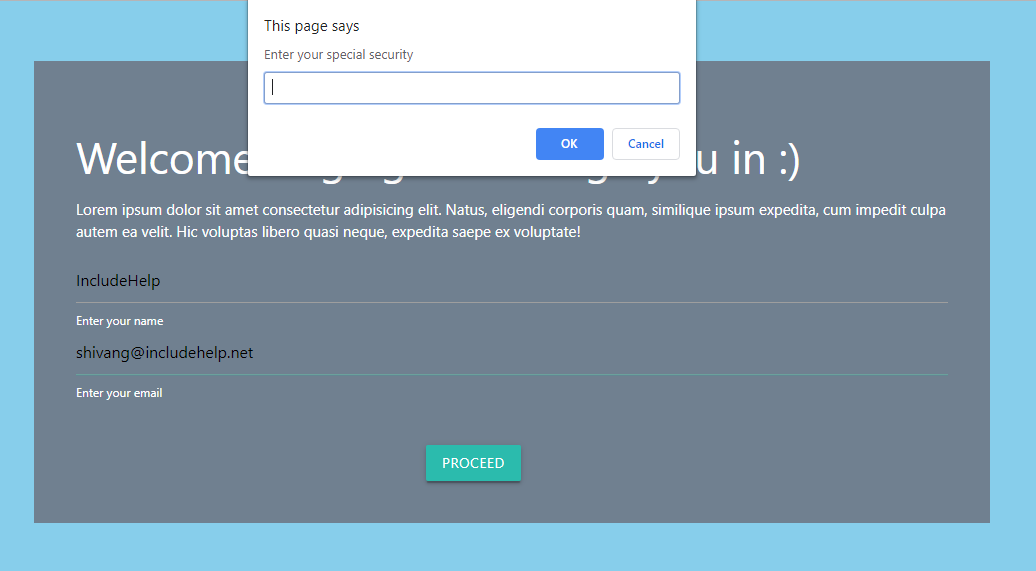
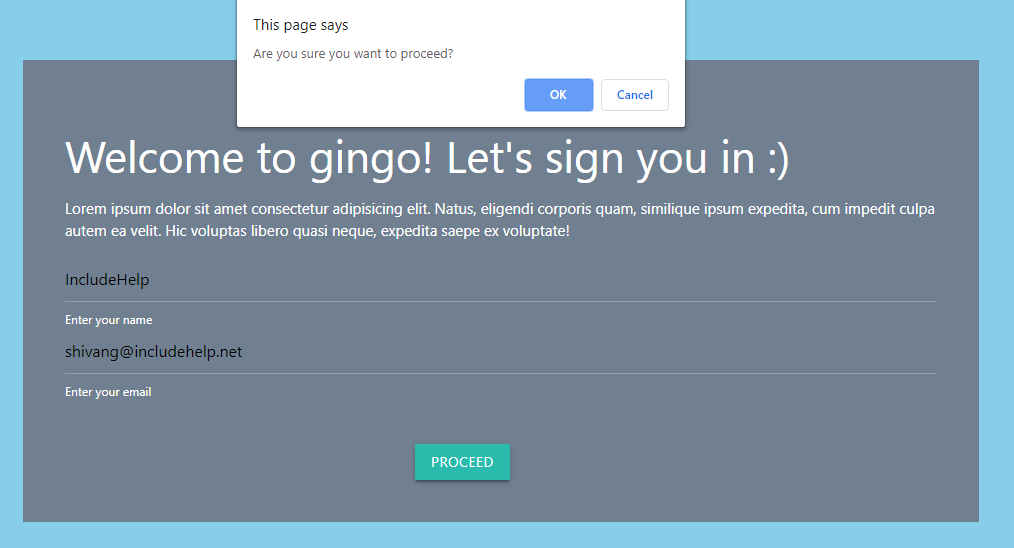
Thus through these Dialog boxes, you can provide feedback to the user that can improve the styles of your forms on your website.
因此,通过这些对话框,您可以向用户提供反馈,以改善您网站上表单的样式。
翻译自: https://www.includehelp.com/code-snippets/dialog-boxes-in-javascript.aspx
javascript对话框