If you have been a regular user of Web, you must have seen password strength functionality on many websites. Generally, when you go up to sign up or create your account, the website helps you to keep the secure password for your account by showing you your password strength.
如果您是Web的常规用户,则必须在许多网站上看到密码强度功能。 通常,当您上报注册或创建帐户时,该网站会通过显示密码强度来帮助您保留帐户的安全密码。
With JavaScript, we can also do that very well. All you should be aware of is that what strength metrics you are considering. If you are planning to implement a password strength checker for your website, then you should consider this article.
使用JavaScript,我们也可以做到这一点。 您只需要知道正在考虑的强度指标即可。 如果您打算为您的网站实施密码强度检查器,则应考虑本文。
JavaScript code:
JavaScript代码:
let strength = {
1: 'Very Weak',
2: 'Weak',
3: 'Medium',
4: 'Strong',
5: 'Very Strong'
};
First, we define strength values for our password strength checker. We have taken these values inside an object, so that we can directly check with the key the status, rather than using series of if-else.
首先,我们为密码强度检查器定义强度值。 我们将这些值放在对象内部,以便我们可以直接通过键检查状态,而不是使用一系列if-else。
JavaScript code:
JavaScript代码:
let strengthValue = {
'caps': false,
'length': false,
'special': false,
'numbers': false,
'small': false
};
Then we define strengthValue parameters for our tool. These are common metrics that you might have already seen, like if it includes capital letters, symbols, numbers etc. By default, the value is false since we won't count the false parameter in our strength value.
然后,为工具定义strengthValue参数。 这些是您可能已经看到的常见指标,例如是否包含大写字母,符号,数字等。默认情况下,该值为false,因为我们不会在强度值中计算false参数。
Now it's time to take input from the user and check the strength of their password.
现在是时候接受用户的输入并检查其密码的强度了。
JavaScript code:
JavaScript代码:
let password = prompt("Enter your password");
if(password.length >= 8) {
strengthValue.length = true;
}
We store this input in password variable and check it's length. Since many password checkers have length constraint, we take 8 here. If the length is greater than 8, then we make the length parameter true in strengthValue. Then we move on to check the string for strength metrics
我们将此输入存储在password变量中,并检查其长度。 由于许多密码检查器具有长度限制,因此这里取8。 如果长度大于8,然后我们作出strengthValue长度参数正确的。 然后我们继续检查强度指标字符串
JavaScript code:
JavaScript代码:
for(let index=0; index < password.length; index++) {
let char = password.charCodeAt(index);
if(!strengthValue.caps && char >= 65 && char <= 90) {
strengthValue.caps = true;
} else if(!strengthValue.numbers && char >=48 && char <= 57){
strengthValue.numbers = true;
} else if(!strengthValue.small && char >=97 && char <= 122){
strengthValue.small = true;
} else if(!strengthValue.numbers && char >=48 && char <= 57){
strengthValue.numbers = true;
} else if(!strengthValue.special && (char >=33 && char <= 47) || (char >=58 && char <= 64)) {
strengthValue.special = true;
}
}
Here first we loop into every character of the password. Inside it, we get the char code of each character in the password. We need character code so that we can compare with other character's char code.
在这里,首先我们进入密码的每个字符。 在其中,我们获取密码中每个字符的字符代码。 我们需要字符代码,以便我们可以与其他字符的字符代码进行比较。
In the first if condition we check if the value of strengthValue.caps is false, since, if this metric is already satisfied, we don't want to check it again. Along with that, we check if the character code falls in between character code of A to Z. Likewise, we do for other metrics as well.
在第一个if条件中,我们检查strengthValue.caps的值是否为false,因为如果已经满足该指标,我们就不想再次检查它。 与此同时,我们检查字符代码是否介于A到Z的字符代码之间。 同样,我们也会处理其他指标。
JavaScript code:
JavaScript代码:
let strengthIndicator = 0;
for(let metric in strengthValue) {
if(strengthValue[metric] === true) {
strengthIndicator++;
}
}
console.log("Your password: " + password + " ( " + strength[strengthIndicator] + " )");
Finally, we check in to get the value of all the metrics. For this, we loop through every metric in strengthValue using for..in loop, that s used to traverse all the keys of an object.
最后,我们检查以获取所有指标的值。 为此,我们使用for..in循环遍历 强度值中的每个度量 ,该遍历用于遍历对象的所有键。
Inside it, we simply check if the value of each metric is true. If it is, then increment the strengthIndicator value. And finally, we console out the value of the indicated value along with the password.
在其中,我们只需检查每个指标的值是否为true。 如果是,则增加strengthIndicator值。 最后,我们将指示值的值和密码一起调出。
This was just a simple example, how you can create common functionality that we see every day with Javascript. See the demo here,
这只是一个简单的示例,说明如何使用Javascript创建每天都会看到的常见功能。 在这里查看演示,
Output
输出量
1) It will prompt for password, enter the password and press okay.
1)提示输入密码,输入密码,然后按确定。
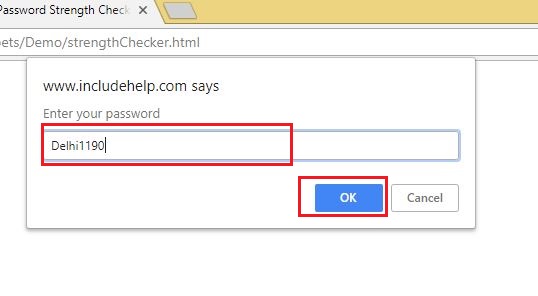
2) Press CTRL + SHIFT + J to open log.
2)按CTRL + SHIFT + J打开日志。
3) Here is your password and its strength.
3)这是您的密码及其强度。
Hope you like the article. Share your thoughts in the comments below.
希望你喜欢这篇文章。 在下面的评论中分享您的想法。
翻译自: https://www.includehelp.com/code-snippets/password-strength-checker-in-javascript.aspx