Lists in Java are ordered collection of data, whereas sets are an unordered collection of data. A list can have duplicate entries, a set can not. Both the data structures are useful in different scenarios.
Java中的列表是有序的数据集合,而集合是无序的数据集合。 列表可以有重复的条目,而集合则不能。 两种数据结构在不同的情况下都是有用的。
Knowing how to convert a set into a list is useful. It can convert unordered data into ordered data.
知道如何将集合转换为列表很有用。 它可以将无序数据转换为有序数据。
初始化集合 (Initializing a set)
Let’s initialize a set and add some elements to it.
让我们初始化一个集合并向其中添加一些元素。
import java.util.*;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
System.out.println(a);
}
}

The add() method of HashSet adds the elements to the set. Note that the elements are distinct. There is no way to get the elements according to their insertion order as the sets are unordered.
HashSet的add()方法将元素添加到集合中。 请注意,这些元素是不同的。 由于元素集是无序的,因此无法根据元素的插入顺序来获取元素。
用Java将集合转换为列表 (Converting Set to List in Java)
Let’s convert the set into a list. There are multiple ways of doing so. Each way is different from the other and these differences are subtle.
让我们将集合转换为列表。 这样做有多种方法。 每种方式都彼此不同,这些差异是细微的。
1.带有Set参数的列表构造函数 (1. List Constructor with Set argument)
The most straightforward way to convert a set to a list is by passing the set as an argument while creating the list. This calls the constructor and from there onwards the constructor takes care of the rest.
将集合转换为列表的最直接方法是在创建列表时将集合作为参数传递。 这将调用构造函数 ,从此以后构造函数将负责其余的工作。
import java.util.*;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr = new ArrayList<>(a);
System.out.println(arr);
System.out.println(arr.get(1));
}
}

Since the set has been converted to a list, the elements are now ordered. That means we can use the get() method to access elements by index.
由于已将集合转换为列表,因此现在对元素进行排序。 这意味着我们可以使用get()方法按索引访问元素。
2.使用常规的for循环 (2. Using conventional for loop )
We can use the good old for loop to explicitly copy the elements from the set to the list.
我们可以使用老式的for循环将元素从集合中显式复制到列表中。
import java.util.*;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr = new ArrayList<>(a);
for (int i : a)
arr.add(i);
System.out.println(arr);
System.out.println(arr.get(1));
}
}

For loop goes over the set element by element and adds the elements to the list.
For循环逐个遍历set元素并将这些元素添加到列表中。
3.列出addAll()方法 (3. List addAll() method)
Lists have a method called addAll() that adds multiple values to the list at once. You might recall this operation from its use in merging two lists. addAll() also works for adding the elements of a set to a list.
列表具有一个名为addAll()的方法,该方法可一次将多个值添加到列表中。 您可能会通过合并两个列表来回忆起该操作。 addAll()也可用于将集合的元素添加到列表中。
import java.util.*;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr = new ArrayList<>();
arr.addAll(a);
System.out.println(arr);
System.out.println(arr.get(1));
}
}

4.流API collect()方法 (4. Stream API collect() method)
Stream.collect() is available from Java 8 onwards. ToList collector collects all Stream elements into a List instance.
从Java 8开始可以使用Stream.collect() 。 ToList收集器将所有Stream元素收集到一个List实例中。
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr;
arr = a.stream().collect(Collectors.toList());
System.out.println(arr);
System.out.println(arr.get(1));
}
}

The documentation for stream.collect() mentions that there is no guarantee on the type, mutability, serializability, or thread-safety of the List returned. If more control over the returned List is required, use toCollection(Supplier).
stream.collect()的文档提到不能保证返回的List的类型,可变性,可序列化性或线程安全性。 如果需要对返回的List进行更多控制,请使用toCollection(Supplier)。
To specify the type of list use toCollection(ArrayList::new)
要指定列表的类型,请使用toCollection(ArrayList :: new)
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr;
arr = a.stream().collect(Collectors.toCollection(ArrayList::new));
System.out.println(arr);
System.out.println(arr.get(1));
}
}
Recommended Reading: Streams in Java
推荐读物 : Java流
5. List.copyOf()方法 (5. List.copyOf() method )
Java 10 onwards List has a copyOf() method. The method returns an unmodifiable List containing the elements of the given Collection, in its iteration order. The list can’t contain any null elements. In case the set contains ‘null’, the method returns a null pointer exception.
Java 10及更高版本的List具有copyOf()方法。 该方法以迭代顺序返回包含给定Collection元素的不可修改的List 。 该列表不能包含任何null元素。 如果集合包含“ null”,则该方法返回一个空指针异常。
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
List<Integer> arr;
arr = List.copyOf(a);
System.out.println(arr);
System.out.println(arr.get(1));
}
}

Adding a null in the set and trying to convert to a list :
在集合中添加null并尝试转换为列表:
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
a.add(null);
List<Integer> arr;
arr = List.copyOf(a);
System.out.println(arr);
System.out.println(arr.get(1));
}
}
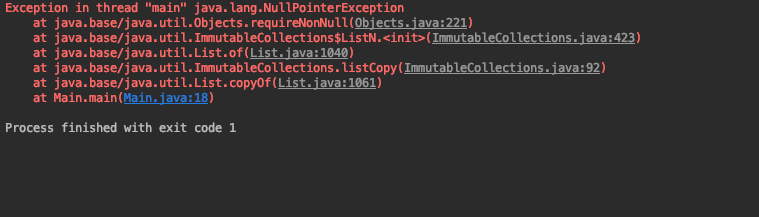
If you try to add an element to an immutable list you’ll get an error that looks like the following.
如果您尝试将元素添加到不可变列表中,则会收到类似于以下内容的错误。

Using the addAll() method to convert set with null element to a list :
使用addAll()方法将具有null元素的set转换为列表:
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args)
{
Set<Integer> a = new HashSet<>();
a.add(1);
a.add(2);
a.add(3);
a.add(1);
a.add(null);
List<Integer> arr = new ArrayList<>();
arr.addAll(a);
// arr = List.copyOf(a);
System.out.println(arr);
System.out.println(arr.get(1));
}
}

Note that the null appears at the beginning of the list.
请注意,空值出现在列表的开头。
结论 (Conclusion )
We saw some really interesting methods to convert a set into a list. It’s important to pay attention to the type of list that is created from each method. Like copyOf() method produces an immutable list and can’t handle null elements. Whereas, stream.collect() doesn’t guarantee anything. Constructor and addAll() are the most trustworthy among the batch.
我们看到了一些非常有趣的方法来将集合转换为列表。 重要的是要注意从每种方法创建的列表的类型。 像copyOf()方法一样, 它会生成不可变的列表 ,并且不能处理null元素。 而stream.collect()不能保证任何事情。 构造函数和addAll()是批处理中最可信赖的。