最大堆和最小堆和平衡二叉树
A Min Heap Binary Tree is a Binary Tree where the root node has the minimum key in the tree.
最小堆二叉树是二叉树,其中根节点在树中具有最小密钥。
The above definition holds true for all sub-trees in the tree. This is called the Min Heap property.
上面的定义对于树中的所有子树都适用。 这称为“ 最小堆”属性。
Almost every node other than the last two layers must have two children. That is, this is almost a complete binary tree, with the exception of the last 2 layers.
除了最后两层以外,几乎每个节点都必须有两个子节点。 也就是说,除了最后两层外,这几乎是完整的二叉树。
The below tree is an example of a min heap binary tree since the above two properties hold.
由于上面的两个属性成立,因此下面的树是最小堆二叉树的示例。
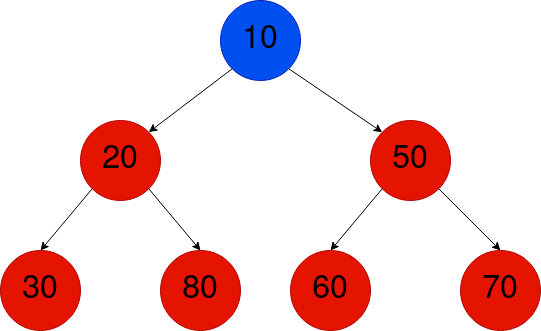
Now that we’ve covered what a min heap tree is, let’s look at how we can represent it.
现在我们已经了解了最小堆树是什么,让我们看看如何表示它。
最小堆树的表示 (Representation of a Min Heap Tree)
A Min Heap Binary Tree is commonly represented as an array, which is indexed according to the below format:
最小堆二叉树通常表示为数组,并根据以下格式进行索引:
Current Node | arr[i] |
Parent Node | arr[(i-1)/2] |
Left Child | arr[(2*i) + 1] |
Right Child | arr[(2*i )+ 2] |
当前节点 | arr[i] |
父节点 | arr[(i-1)/2] |
左子 | arr[(2*i) + 1] |
合适的孩子 | arr[(2*i )+ 2] |
The root of the whole tree is at arr[0]
.
整个树的根位于arr[0]
。
We will use the indexing as shown in the below figure. It’s not very hard to find the pattern here, which will match with the above table.
我们将使用下图所示的索引。 在此处找到与上表匹配的模式不是很困难。
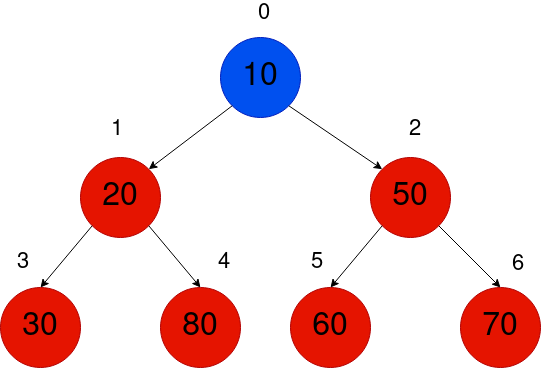
This indexing follows a Level Order Traversal of the Binary Tree, so a Binary Heap array is a Binary Tree using a level order traversal.
该索引遵循二进制树的级别顺序遍历 ,因此,二进制堆数组是使用级别顺序遍历的二进制树。
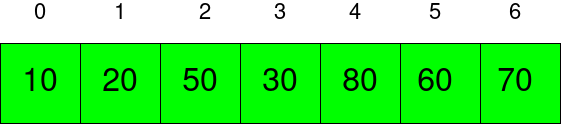
The above figure shows the array representation of the Min Heap Tree.
上图显示了最小堆树的数组表示形式。
Now that we’ve covered the concepts, let’s move onto implementing this in C!
现在我们已经涵盖了概念,让我们继续使用C来实现它!
实施最小堆树 (Implementing a Min Heap Tree)
We will use the array representation to build the tree. Let’s start writing the structure for the Min Heap.
我们将使用数组表示法来构建树。 让我们开始编写Min Heap的结构。
typedef struct MinHeap MinHeap;
struct MinHeap {
int* arr;
// Current Size of the Heap
int size;
// Maximum capacity of the heap
int capacity;
};
We’ll have an array of elements, and a size, which gets updated as elements are being inserted or deleted.
我们将有一个元素数组和一个大小,该大小会随着元素的插入或删除而更新。
The array also has a capacity, which indicates the maximum size of the array.
阵列还具有容量,该容量指示阵列的最大大小。
There are a few functions that we need to write to indicate that we are representing a Min Heap Tree, like finding the parent, and the children.
我们需要编写一些函数来表示我们正在代表最小堆树,例如查找父级和子级。
int parent(in