编程实现strcpy函数
We earlier learned about strings in C programming in our Strings in C tutorial. Today, we will focus on strcpy() in C programming.
我们之前在“ C语言中的字符串教程”中了解了C编程中的字符串 。 今天,我们将专注于C编程中的 strcpy()。
C编程中的strcpy()函数 (The strcpy() function in C Programming)
The strcpy()
function copies the contents of one string into another. The base address of the target and the source(or the source string) should be supplied to this function in order to copy the source string into the target one. Let’s look at the syntax,
strcpy()
函数将一个字符串的内容复制到另一个字符串中。 应该将目标的基地址和源 (或源字符串)提供给此函数,以便将源字符串复制到目标字符串中。 让我们看一下语法,
strcpy( char *target, const char *source );
strcpy( char *target, const char *source );
strcpy()
goes on copying the characters from the source string into the target string until it encounters the Null character(‘\0’). Since the string gets copied in a character-by-character fashion. It should be kept in mind that the target string should have a dimension that can hold the copied string.
strcpy()
继续将字符从源字符串复制到目标字符串,直到遇到Null字符('\ 0')为止。 由于字符串是以逐字符的方式复制的。 请记住,目标字符串应具有可以容纳复制的字符串的维。
如何在C中使用strcpy()函数 (How to use the strcpy() function in C)
The given example below illustrates the use of strcpy()
function in C,
下面给出的示例说明了C中strcpy()
函数的用法,
#include<stdio.h>
#include<string.h>
int main()
{
char target[30],source[30];
printf("Enter the source string to be copied:");
fgets(source,30,stdin);
printf("\n\nsource---->target\n\n");
strcpy(target,source);
printf("The target after copying is:%s",target);
return 0;
}
Output:
输出:

- In the code above, we first declare the two target and source strings. 在上面的代码中,我们首先声明两个目标字符串和源字符串。
- After that, user input for the source string is taken. Which in this case was “JournalDev strcpy() example”. 之后,将使用用户输入的源字符串。 在本例中为“ JournalDev strcpy()示例” 。
- When we have the source string, we used the
strcpy()
function from the string.h header file. The function copied the contents of the source string into the target one. 当我们有了源字符串时,我们使用了string.h头文件中的strcpy()
函数。 该函数将源字符串的内容复制到目标字符串中。
Please note that the source string could also be passed directly to the strcpy()
function. But for the target string, we always need to specify the pointer pointing to it.
请注意 ,源字符串也可以直接传递给strcpy()
函数。 但是对于目标字符串,我们总是需要指定指向它的指针。
制作我们自己的字符串复制功能 (Making our own string copying function)
Let us attempt at making our own string copying function for a better understanding of how the strcpy()
function works.
让我们尝试创建自己的字符串复制函数,以更好地了解strcpy()
函数的工作方式。
#include<stdio.h>
char *My_strcpy(char *t, const char *s) //My_strcpy() definition
{
char *temp;
temp=t;
while(*s!='\0') //character-by-charaacter copying
{
*t=*s;
s++;
t++;
}
*t='\0'; //string termination
return temp;
}
int main()
{
char target[30],source[30];
printf("Enter the source string to be copied:");
fgets(source,30,stdin);
printf("\n\nsource---->target\n\n");
My_strcpy(target,source);
printf("The target after copying is:%s",target);
return 0;
}
Output:
输出:
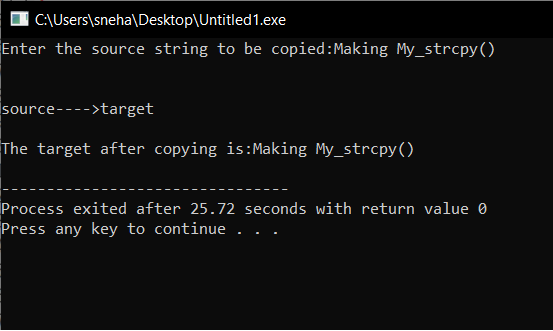
Above we declare as well as define My_strcpy()
function before the main()
function. And inside main()
we use it instead of the pre-defined strcpy()
function to get the above output.
上面我们在main()
函数之前声明并定义My_strcpy()
main()
函数。 在main()
内部,我们使用它代替预定义的strcpy()
函数来获取上述输出。
Did you notice while defining the My_strcpy()
function we initialized pointer *s
to be constant? This was done to ensure that by any means the function doesn’t alter the contents of the source string.
您在定义My_strcpy()
函数时是否注意到我们将指针*s
初始化为常量? 这样做是为了确保该函数无论如何都不会更改源字符串的内容。
This is how the function strcpy()
works in C programming.
这就是函数strcpy()
在C编程中的工作方式。
结论 (Conclusion)
In this tutorial, we first learned what is the strcpy()
function. We also learned how to implement our own strcpy() function through the implementation of My_strcpy()
.
在本教程中,我们首先学习了什么是strcpy()
函数。 我们还学习了如何通过My_strcpy()
的实现来实现自己的strcpy()函数。
For further reading – Check all the previous tutorials on C Programming on Journaldev.
要进一步阅读,请参阅Journaldev上有关C编程的所有先前教程。
参考资料 (References)
- https://en.wikibooks.org/wiki/C_Programming/String_manipulation https://zh.wikibooks.org/wiki/C_Programming/String_manipulation
- https://www.journaldev.com/35071/strings-in-c-programming https://www.journaldev.com/35071/strings-in-c-programming
- https://stackoverflow.com/questions/21759894/use-the-strcpy-c https://stackoverflow.com/questions/21759894/use-the-strcpy-c
翻译自: https://www.journaldev.com/35671/strcpy-in-c-programming
编程实现strcpy函数