二叉树层级遍历
Level Order Traversal is one of the methods for traversing across a Binary Tree. In this article, we shall look at how we can implement this algorithm in C/C++.
级别顺序遍历是跨二叉树遍历的方法之一。 在本文中,我们将研究如何在C / C ++中实现此算法。
But before that, let us have our concepts covered.
但是在此之前,让我们涵盖一下我们的概念。
建立概念 (Building the concepts)
A Binary Tree is a data structure where every node has at-most two children. The topmost node is called the Root node.
二叉树是一种数据结构,其中每个节点最多具有两个子节点。 最顶层的节点称为“ 根”节点。

There are 4 common ways of traversing the nodes of a Binary Tree, namely:
遍历二叉树的节点有4种常见方法,即:
- In order Traversal 为了遍历
- Pre Order Traversal 预购遍历
- Post Order Traversal 订单遍历
- Level Order Traversal 级别顺序遍历
Let’s understand what a level in a Binary Tree means.
让我们了解二叉树中的级别的含义。
A level is the number of parent nodes corresponding to a given a node of the tree. It is basically the number of ancestors from that node until the root node.
级别是与树的给定节点相对应的父节点的数量。 它基本上是从该节点到根节点的祖先数。
So, for the root node (topmost node), it’s level is 0, since it has no parents. If it has children, both of them will have a level of 1, since it has only one ancestor until the root node, which is the root node itself.
因此,对于根节点(最高节点),其级别为0,因为它没有父级。 如果它有子节点,那么两个节点的级别都将为1,因为在根节点(即根节点本身)之前只有一个祖先。
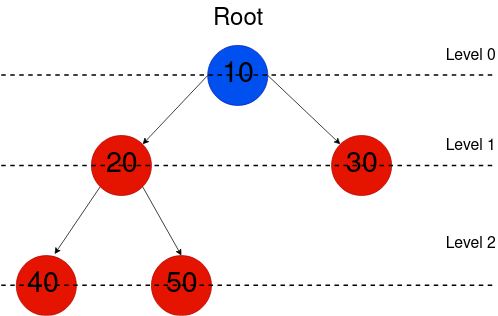
We also need to understand the notion of height in a Binary Tree. This is simply the length of the path from the root to the deepest node in the tree.
我们还需要了解二叉树中的高度概念。 这只是从根到树中最深节点的路径长度。
In this case, the height will be the length from the deepest node (40 or 50, since they have the maximum level) to the root. So the height of the tree is 2.
在这种情况下,高度将是从最深节点( 40或50 ,因为它们具有最大级别)到根的长度。 因此,树的高度为2 。
Now that we have our concepts covered, let’s understand how we can implement Level Order Traversal.
既然我们已经涵盖了概念,那么让我们了解如何实现级别顺序遍历。
级别顺序遍历 (Level Order Traversal )
A Level Order Traversal is a traversal which always traverses based on the level of the tree.
级别顺序遍历是始终根据树的级别进行遍历的遍历。
So, this traversal first traverses the nodes corresponding to Level 0, and then Level 1, and so on, from the root node.
因此,此遍历首先从根节点遍历与级别0,然后是级别1等的节点。
In the example Binary Tree above, the level order traversal will be:
在上面的示例二叉树中,级别顺序遍历为:
(Root) 10 -> 20 -> 30 -> 40 -> 50
(根) 10- > 20- > 30- > 40- > 50
To do this, we need to do 2 things.
为此,我们需要做两件事。
- We must first find the height of the tree 我们必须首先找到树的高度
- We need to find a way to print the nodes corresponding to every level. 我们需要找到一种方法来打印与每个级别相对应的节点。
找到树的高度 (Find the height of the Tree)
We will find the height of the tree first. To do this, the logic is simple.
我们将首先找到树的高度。 为此,逻辑很简单。
Since the height of the tree is defined as the largest path from the root to a leaf. So we can recursively compute the height of the left and right sub-trees, and find the maximum height of the sub-tree. The height of the tree will then simply be the height of the sub-tree + 1.
由于树的高度定义为从根到叶的最大路径。 因此,我们可以递归计算左右子树的高度,并找到子树的最大高度。 然后,树的高度将简单地是子树的高度+ 1。
C- style Pseudo Code:
C型伪代码 :
// Find height of a tree, defined by the root node
int tree_height(Node* root) {
if (root == NULL)
return 0;
else {
// Find the height of left, right subtrees
left_height = tree_height(root->left);
right_height = tree_height(root->right);
// Find max(subtree_height) + 1 to get the height of the tree
return max(left_height, right_height) + 1;
}
打印每个级别中的所有节点 (Print all nodes in every level)
Now that we have the height, we must print nodes for every level. To do this, we will use a for
loop to iterate all levels until the height, and print nodes at every level.
现在我们有了高度,我们必须为每个级别打印节点。 为此,我们将使用for
循环迭代所有级别直到高度,并在每个级别打印节点。
void print_tree_level_order(Node* root) {
int height = tree_height(root);
for (int i=0; i<height; i++) {
// Print the ith level
print_level(root, i);
}
}
Observe that we need another function to print the i
th level of the tree.
观察到我们需要另一个函数来打印树的第i
级。
Here again, we have a similar logic. But this time, after printing the root node, we change the root node to it’s left and right children and print both sub-trees.
同样,我们有类似的逻辑。 但是这一次,在打印根节点之后,我们将根节点更改为左右两个子节点,并打印两个子树。
This will continue until we reach a leaf node, that is when the auxiliary root will be NULL
at the next step. (Since leaf_node->left = NULL
and leaf_node->right = NULL
)
这将一直持续到到达叶节点为止,也就是说,在下一步中辅助根将为NULL
时。 (因为leaf_node-> left = NULL
和leaf_node-> right = NULL
)
void print_level(Node* root, int level_no) {
// Prints the nodes in the tree
// having a level = level_no
// We have a auxiliary root node
// for printing the root of every
// sub-tree
if (!root)
return;
if (level_no == 0) {
// We are at the top of a sub-tree
// So print the auxiliary root node
printf("%d -> ", root->value);
}
else {
// Make the auxiliary root node to
// be the left and right nodes for
// the sub-trees and decrease level by 1, since
// you are moving from top to bottom
print_level(root->left, level_no - 1);
print_level(root->right, level_no - 1);
}
}
Now, we have finally completed the Level Order Traversal!
现在,我们终于完成了等级顺序遍历!
I will provide the complete program below, which also has a section to construct the Binary Tree using insertion.
我将在下面提供完整的程序,其中还有一节使用插入来构造二叉树。
完整的C / C ++代码 (Complete C/C++ Code)
While this is originally a C program, the same can be compiled on C++ as well.
虽然这最初是C程序,但同样可以在C ++上编译。
/**
Code for https://journaldev.com
File Name: level_order.c
Purpose: Find the Level Order Traversal of a Binary Tree
@author Vijay Ramachandran
@date 28/01/2020
*/
#include <stdio.h>
#include <stdlib.h>
typedef struct Node Node;
// Define the Tree Node here
struct Node {
int value;
// Pointers to the left and right children
Node* left, *right;
};
Node* init_tree(int data) {
// Creates the tree and returns the
// root node
Node* root = (Node*) malloc (sizeof(Node));
root->left = root->right = NULL;
root->value = data;
return root;
}
Node* create_node(int data) {
// Creates a new node
Node* node = (Node*) malloc (sizeof(Node));
node->value = data;
node->left = node->right = NULL;
return node;
}
void free_tree(Node* root) {
// Deallocates memory corresponding
// to every node in the tree.
Node* temp = root;
if (!temp)
return;
free_tree(temp->left);
free_tree(temp->right);
if (!temp->left && !temp->right) {
free(temp);
return;
}
}
int tree_height(Node* root) {
// Get the height of the tree
if (!root)
return 0;
else {
// Find the height of both subtrees
// and use the larger one
int left_height = tree_height(root->left);
int right_height = tree_height(root->right);
if (left_height >= right_height)
return left_height + 1;
else
return right_height + 1;
}
}
void print_level(Node* root, int level_no) {
// Prints the nodes in the tree
// having a level = level_no
// We have a auxiliary root node
// for printing the root of every
// subtree
if (!root)
return;
if (level_no == 0) {
// We are at the top of a subtree
// So print the auxiliary root node
printf("%d -> ", root->value);
}
else {
// Make the auxiliary root node to
// be the left and right nodes for
// the subtrees and decrease level by 1, since
// you are moving from top to bottom
print_level(root->left, level_no - 1);
print_level(root->right, level_no - 1);
}
}
void print_tree_level_order(Node* root) {
if (!root)
return;
int height = tree_height(root);
for (int i=0; i<height; i++) {
printf("Level %d: ", i);
print_level(root, i);
printf("\n");
}
printf("\n\n-----Complete Level Order Traversal:-----\n");
for (int i=0; i<height; i++) {
print_level(root, i);
}
printf("\n");
}
int main() {
// Program to demonstrate Level Order Traversal
// Create the root node having a value of 10
Node* root = init_tree(10);
// Insert nodes onto the tree
root->left = create_node(20);
root->right = create_node(30);
root->left->left = create_node(40);
root->left->right = create_node(50);
// Level Order Traversal
print_tree_level_order(root);
// Free the tree!
free_tree(root);
return 0;
}
Output
输出量
Level 0: 10 ->
Level 1: 20 -> 30 ->
Level 2: 40 -> 50 ->
-----Complete Level Order Traversal:-----
10 -> 20 -> 30 -> 40 -> 50 ->
You can also download this through a Github gist that I created for this purpose. (Contains code for insertion as well)
您也可以通过我为此目的创建的Github要点下载它。 (还包含用于插入的代码)
结论 (Conclusion)
Hopefully you have a better understanding of how Level Order Traversal can be implemented in C/C++. If you have any questions, feel free to ask them in the comments section below!
希望您对如何在C / C ++中实现级别顺序遍历有更好的了解。 如果您有任何疑问,请随时在下面的评论部分中提问!
翻译自: https://www.journaldev.com/34929/level-order-traversal-in-a-binary-tree
二叉树层级遍历