java关键字保留字
Java中的“ this”关键字是什么? (What is ‘this’ Keyword in Java?)
- Java this keyword returns a reference to the current Object. Java这个关键字返回对当前Object的引用。
- We can access object variable, call the method or constructor of the current object using this keyword. 我们可以访问对象变量,使用此关键字调用当前对象的方法或构造函数。
- Java ‘this’ keyword can be used to refer to any member of the current object from within an instance method or a constructor. Java'this'关键字可用于在实例方法或构造函数中引用当前对象的任何成员。
- this keyword is mostly used to avoid the confusion between a class attribute and a parameter. For example, if a parameter of a member function has the same name as that of an object variable, then the functionality may not work properly. 此关键字主要用于避免类属性和参数之间的混淆。 例如,如果成员函数的参数名称与对象变量的名称相同,则该功能可能无法正常工作。
Java这个关键字示例 (Java this Keyword Example)
Let’s say we have a class like below.
假设我们有一个类似下面的类。
public class Item{
String name;
// Constructor with a parameter and this keyword
public Item(String name) {
this.name = name;
}
// Call the constructor
public static void main(String[] args) {
Item Obj = new Item("car");
System.out.println(Obj.name);
}
}
以“ this”作为当前对象的参考输出 (Output with ‘this’ as referance to current object)

Java this Keyword Example
Java这个关键字示例
The code prints the name of the item as “car”. Now let’s see what happens when we remove ‘this’ keyword from the constructor.
代码将项目名称打印为“ car”。 现在让我们看看从构造函数中删除“ this”关键字时会发生什么。
public class Item{
String name;
// Constructor with a parameter and without this keyword
public Item(String name) {
name = name;
}
// Call the constructor
public static void main(String[] args) {
Item Obj = new Item("car");
System.out.println(Obj.name);
}
}
输出不带“ this”作为当前对象的参考 (Output without ‘this’ as referance to current object)
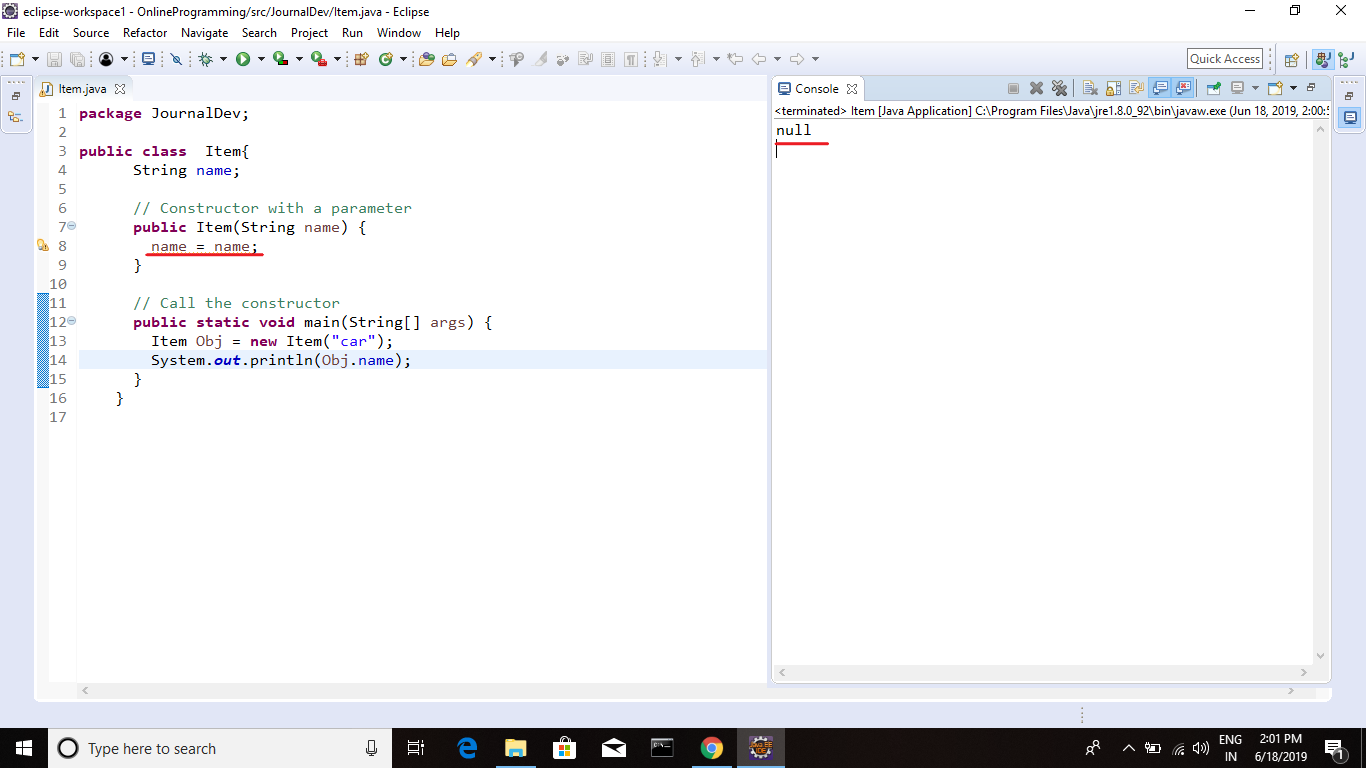
Output without ‘this’ Keyword
没有“ this”关键字的输出
The above example shows the application of this variable for accessing object’s attribute.
上面的示例显示了此变量在访问对象属性中的应用。
We can’t create two local variables with the same name.
我们不能创建两个具有相同名称的局部变量。
However, it is allowed to create one instance variable and one local variable or method parameter with the same name.
但是,允许创建一个具有相同名称的实例变量和一个局部变量或方法参数。
In this case, the local variable will hide the instance variable, which is called Variable Shadowing.
在这种情况下,局部变量将隐藏实例变量,称为变量阴影 。
To solve this problem, we use this keyword with a field to point to the instance variable instead of the local variable.
为解决此问题,我们将此关键字与字段一起使用以指向实例变量而不是局部变量。
“ this”关键字的一般用法 (General uses of ‘this’ keyword)
- Java ‘this’ keyword can be used to refer current class instance variable. Java'this'关键字可用于引用当前的类实例变量。
- We can use this() to invoke current class constructor. We can also pass parameters in this() statement. 我们可以使用this()来调用当前的类构造函数。 我们还可以在this()语句中传递参数。
- Java ‘this’ keyword can be used to invoke current class method (implicitly). Java'this'关键字可用于(隐式)调用当前的类方法。
- The ‘this’ keyword can be passed as an argument in method call. 'this'关键字可以在方法调用中作为参数传递。
- We can use ‘this’ keyword to return current class instance. 我们可以使用'this'关键字返回当前的类实例。
- We can use ‘this’ keyword to access object attributes in case of variable shadowing. 在可变阴影的情况下,我们可以使用'this'关键字访问对象属性。
There is also a special type of constructor called as Copy Constructor. Java does not have a default copy constructor but we can create it explicitly using this keyword.
还有一种特殊类型的构造函数,称为Copy Constructor 。 Java没有默认的副本构造函数,但是我们可以使用此关键字显式创建它。
复制构造函数和display()方法“ this”关键字 (Copy-Constructor & display() method ‘this’ keyword)
package com.journaldev.examples;
public class Blog {
String name;
int popularity;
// parameterized constructor
public Blog(String name, int popularity) {
this.name = name;
this.popularity = popularity;
}
// Copy-Constructor
public Blog(Blog b) {
this.popularity = b.popularity;
this.name = b.name;
}
public void display() {
System.out.println("name: " + this.name);
System.out.println("popularity: " + this.popularity + " %");
}
public static void main(String[] args) {
// parameterized constructor call
Blog obj1 = new Blog("JournalDev", 100);
obj1.display();
// Copy-Constructor call
Blog obj2 = new Blog(obj1);
obj2.display();
}
}
复制构造函数和display()方法的输出 (Output of Copy-Constructor & display() method)

Copy Constructor Output
复制构造函数输出
In the above code, we saw the application of ‘this’ keyword in a copy constructor and a method display().
在上面的代码中,我们看到了'this'关键字在复制构造函数中的应用以及方法display() 。
使用此关键字调用构造函数 (Calling a Constructor using this keyword)
Let’s look at an example to call a constructor using this keyword.
让我们看一个使用此关键字调用构造函数的示例。
package com.journaldev.examples;
public class Data {
Data() {
System.out.println("default constructor");
}
Data(int i) {
this();
System.out.println("int parameter constructor");
}
Data(String s) {
this(10);
System.out.println("string parameter constructor");
}
public static void main(String[] args) {
Data d = new Data(20);
System.out.println("------");
Data d1 = new Data("Hi");
}
}
Output:
输出 :
default constructor
int parameter constructor
------
default constructor
int parameter constructor
string parameter constructor
使用此关键字调用对象方法 (Using this keyword to call the object method)
Let’s look at an example of calling the object method using this keyword.
让我们看一个使用此关键字调用对象方法的示例。
package com.journaldev.examples;
public class Data {
Data() {
this.foo();
}
private void foo() {
System.out.println("foo method");
}
public static void main(String[] args) {
Data d = new Data();
}
}
在方法参数中使用此关键字 (Using this keyword in method argument)
package com.journaldev.examples;
public class Data {
private int id;
public Data(int id) {
this.id = id;
bar(this);
}
private void bar(Data data) {
System.out.println(data.id);
}
public static void main(String[] args) {
Data d = new Data(20);
}
}
Java这个关键字返回当前对象 (Java this Keyword to return Current Object)
Let’s look at a simple example where we will use this keyword to return the current object from a method.
让我们看一个简单的示例,在该示例中,我们将使用此关键字从方法中返回当前对象。
private Data bar(Data data) {
System.out.println(data.id);
return this;
}
结论 (Conclusion)
Java this keyword is helpful in getting the reference of the current object. It’s useful in accessing object attributes in case of variable shadowing. We can also use it to call the current class constructors.
Java这个关键字有助于获取当前对象的引用。 在变量阴影的情况下访问对象属性很有用。 我们还可以使用它来调用当前的类构造函数。
java关键字保留字