散列表平均查找长度
什么是链表? (What is a Linked List?)
- A linked list is a linear data structure used for storing collections of data 链表是一种线性数据结构,用于存储数据集合
- Successive elements are connected by pointers 连续元素通过指针连接
- The last element points to NULL 最后一个元素指向NULL
- Each element is a separate object and is called a Node 每个元素都是一个单独的对象,称为节点
- Each node in a linked list comprises of two parts
- Data
- Reference to Next Node
- 数据
- 参考下一个节点

LinkedList Node
LinkedList节点
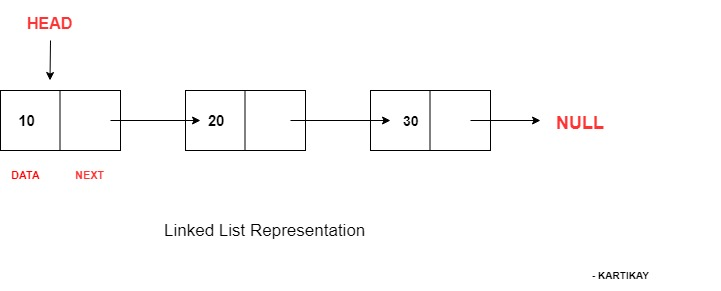
Linked List
链表
如何查找链接列表的长度? (How to Find the Length of a Linked List?)
There are two ways to find the length of a linked list:
有两种方法可以找到链表的长度:
- Iterative Approach 迭代法
- Recursive Approach 递归方法
使用迭代方法的链表长度 (Length of Linked List using Iterative Approach)
We will use the Linked list traversal to find the length of a linked list.
我们将使用“链表”遍历来查找链表的长度。
- Head Points to the First Node of The List. 头指向列表的第一个节点。
- Initialize the count variable with value 0 用值0初始化计数变量
- Initialize the temp variable with Head 用Head初始化temp变量
- As we access each Node, the value of count variable is increased by 1. 当我们访问每个节点时,count变量的值增加1。
- Stop The process when we reach null. 当我们达到空值时,停止该过程。
- Do not change the head reference. 请勿更改头部参考。
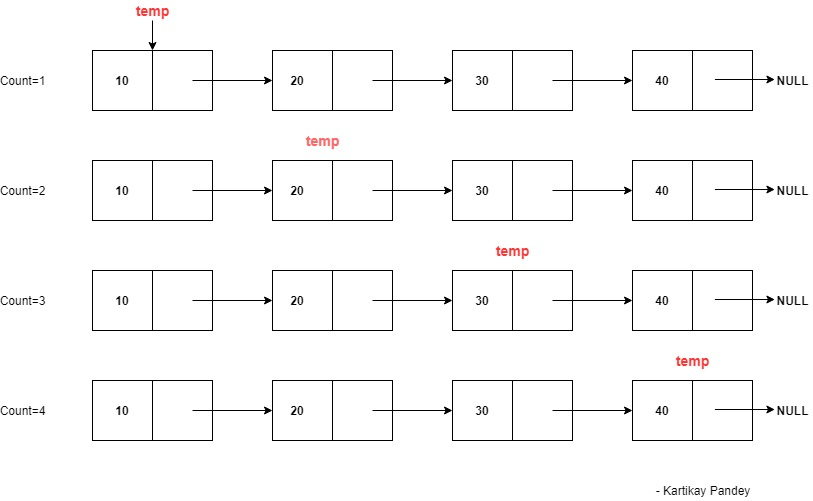
Iterative Approach for LinkedList Length
LinkedList长度的迭代方法
Java代码 (Code in Java)
package com.journaldev.ds;
public class MyLinkedList {
public class Node {
int data;
Node next;
}
public Node head;
public Node tail;
public int size;
public int getFirst() throws Exception {
if (this.size == 0) {
throw new Exception("linked list is empty");
}
return this.head.data;
}
public int getLast() throws Exception {
if (this.size == 0) {
throw new Exception("linked list is empty");
}
return this.tail.data;
}
public void display() {
Node temp = this.head;
while (temp != null) {
System.out.println(temp.data + " ");
temp = temp.next;
}
}
public void addFirst(int item) {
Node nn = new Node();
nn.data = item;
if (this.size == 0) {
this.head = nn;
this.tail = nn;
this.size = this.size + 1;
} else {
nn.next = this.head;
this.head = nn;
this.size = this.size + 1;
}
}
public int length() {
Node temp = this.head;
int count = 0;
while (temp != null) {
count++;
temp = temp.next;
}
return count;
}
public static void main(String[] args) {
MyLinkedList ll = new MyLinkedList();
ll.addFirst(10);
ll.addFirst(20);
ll.addFirst(30);
ll.addFirst(40);
ll.addFirst(50);
System.out.println("Length of Linked List is " + ll.length());
}
}
C语言代码 (Code in C)
#include <stdio.h>
#include <stdlib.h>
/* A structure of linked list node */
struct node {
int data;
struct node *next;
} *head;
void initialize(){
head = NULL;
}
/*
Inserts a node in front of a singly linked list.
*/
void insert(int num) {
/* Create a new Linked List node */
struct node* newNode = (struct node*) malloc(sizeof(struct node));
newNode->data = num;
/* Next pointer of new node will point to head node of linked list */
newNode->next = head;
/* make new node as the new head of linked list */
head = newNode;
printf("Inserted Element : %d\n", num);
}
int getLength(struct node *head){
int length =0;
while(head != NULL){
head = head->next;
length++;
}
return length;
}
/*
Prints a linked list from head node till the tail node
*/
void printLinkedList(struct node *nodePtr) {
while (nodePtr != NULL) {
printf("%d", nodePtr->data);
nodePtr = nodePtr->next;
if(nodePtr != NULL)
printf("-->");
}
}
int main() {
initialize();
/* Creating a linked List*/
insert(8);
insert(3);
insert(2);
insert(7);
insert(9);
printf("\nLinked List\n");
printLinkedList(head);
printf("\nLinked List Length : %d", getLength(head));
return 0;
}
Output
输出量

Iterative Solution Output
迭代解输出
使用递归解的链表长度 (Length of Linked List using Recursive Solution)
Base Case:
基本情况:
- Last Node points to Null value 最后一个节点指向空值
- Return 0 返回0
Recursive Case:
递归案例:
- At each step update the Value of Current Node to the Next Node 在每个步骤中,将当前节点的值更新到下一个节点
- Call= 1+fun(curr.next) 通话= 1+趣味(curr.next)
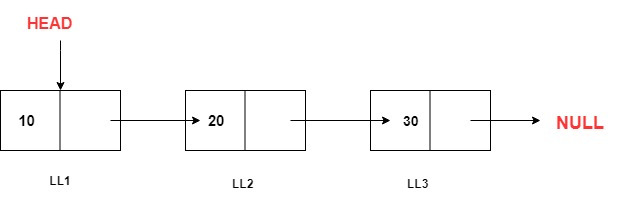
Recursive Solution
递归解
Example:
There are 3 elements in the linked list: LL1, LL2, and LL3.
例:
链接列表中有3个元素:LL1,LL2和LL3。
We will Observe What happens in the Memory Stack when the Recursive Call is made.
当进行递归调用时,我们将观察内存堆栈中会发生什么。
MEMORY STACK:
内存堆栈 :

Memory Stack
记忆体堆叠
The main function calls LL1, LL1 calls LL2, LL2 calls LL3, LL3 calls null value.
主函数调用LL1,LL1调用LL2,LL2调用LL3,LL3调用空值。
As Null value is reached, we return from here.
当达到Null值时,我们从这里返回。
0 is returned to LL3, LL3 returns 1 to LL2, LL2 returns 2 to LL1.
LL3返回0,LL3返回LL2,LL2返回2,LL1。
LL1 finally returns 3 to the main function.
LL1最后将3返回到主函数。
Java代码 (Code in Java)
package com.journaldev.ds;
public class MyLinkedList {
public class Node {
int data;
Node next;
}
public Node head;
public Node tail;
public int size;
public int getfirst() throws Exception {
if (this.size == 0) {
throw new Exception("linked list is empty");
}
return this.head.data;
}
public int RemoveFirst() throws Exception {
if (this.size == 0) {
throw new Exception("LL is empty");
}
Node temp = this.head;
if (this.size == 1) {
this.head = null;
this.tail = null;
size = 0;
} else {
this.head = this.head.next;
this.size--;
}
return temp.data;
}
public void addFirst(int item) {
Node nn = new Node();
nn.data = item;
if (this.size == 0) {
this.head = nn;
this.tail = nn;
this.size = this.size + 1;
} else {
nn.next = this.head;
this.head = nn;
this.size = this.size + 1;
}
}
public int lengthUsingRecursiveApproach (){
return lengthUsingRecursiveApproach(this.head);
}
private int lengthUsingRecursiveApproach(Node curr) {
// TODO Auto-generated method stub
if (curr == null) {
return 0;
}
return 1 + lengthUsingRecursiveApproach (curr.next);
}
public static void main(String[] args) {
MyLinkedList ll = new MyLinkedList();
// insert elements into the Linked List
ll.addFirst(10);
ll.addFirst(20);
ll.addFirst(30);
ll.addFirst(40);
ll.addFirst(50);
// Length of List
System.out.println("Recursive Approach length " + ll.lengthUsingRecursiveApproach(ll.head));
}
}
C语言代码 (Code in C)
#include <stdio.h>
struct Node
{
int data;
struct Node* next;
};
void push(struct Node** head_ref, int new_data)
{
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = new_data;
/* link the old list of the new node */
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
int getCount(struct Node* head)
{
// Base case
if (head == NULL)
return 0;
return 1 + getCount(head->next);
}
int main()
{
struct Node* head = NULL;
push(&head, 1);
push(&head, 3);
push(&head, 1);
push(&head, 2);
push(&head, 1);
printf("count of nodes is %d", getCount(head));
return 0;
}
Output
输出量
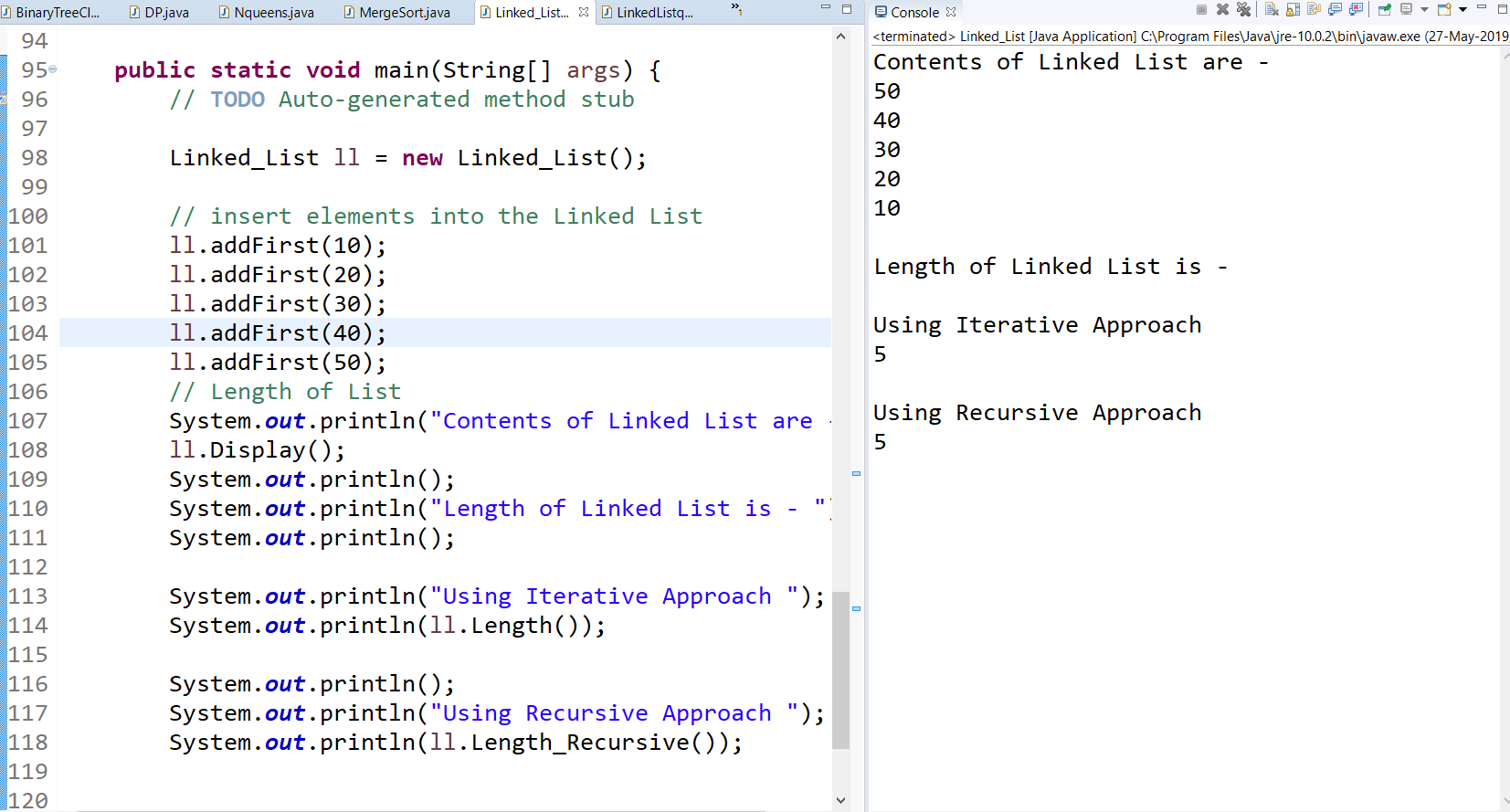
Recursive Solution Output
递归解决方案输出
时间复杂度 (Time Complexity)
O(N) in both the recursive and iterative solution, as all we need, is a single traversal to know the length.
正如我们所需要的,递归和迭代解决方案中的O(N)都是一次遍历以了解长度。
翻译自: https://www.journaldev.com/30153/find-length-of-a-linked-list
散列表平均查找长度