Java String replaceAll() replaces all the occurrences of a particular character, string or a regular expression in the string. The method returns a new string as its output. The method requires two parameters.
Java字符串replaceAll()替换所有出现的特定字符,字符串或字符串中的正则表达式。 该方法返回一个新字符串作为其输出。 该方法需要两个参数。
字符串replaceAll()语法 (String replaceAll() Syntax)
public String replaceAll(String regex, String replacement)
A regex that represents the pattern to look for while replacing and a replacement string to replace it with.
一个正则表达式 ,代表替换时要查找的模式以及替换 字符串 。
There are multiple types of substitutions that are possible with String replaceAll() method.
字符串replaceAll()方法可能有多种替换类型。
替换单个字符 (Replacing a single character )
To replace a single character, specify the character to be replaced as the regex.
要替换单个字符,请指定要替换的字符作为正则表达式。
public class Main {
public static void main(String[] args) {
String s = "Welcome to JournalDev";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceAll("o","@");
System.out.println("New String: "+rep);
}
}
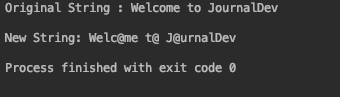
Original String: Welcome to JournalDev
New String: Welc@me t@ J@urnalDev
替换字符序列 (Replace sequence of characters )
To replace a sequence of characters, mention the sequence as the regex in the replaceAll() function.
要替换字符序列,请在replaceAll()函数中将该序列称为正则表达式。
public class Main {
public static void main(String[] args) {
String s = "Welcome to JournalDev";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceAll("to","this is");
System.out.println("New String: "+rep);
}
}
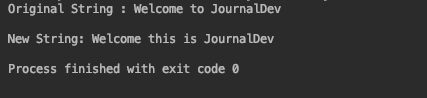
Original String : Welcome to JournalDev
New String: Welcome this is JournalDev
删除/替换空间 (Remove/Replace Spaces)
The replaceAll() method can remove or replace the whitespaces in your text string.
replaceAll()方法可以删除或替换文本字符串中的空格。
public class Main {
public static void main(String[] args) {
String s = "Welcome to JournalDev";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceAll(" ","");
System.out.println("New String: "+rep);
}
}
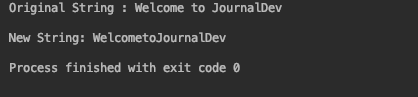
Original String : Welcome to JournalDev
New String: WelcometoJournalDev
Similarly, to replace the whitespaces:
同样,要替换空白:
public class Main {
public static void main(String[] args) {
String s = "Welcome to JournalDev";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceAll(" ","_");
System.out.println("New String: "+rep);
}
}
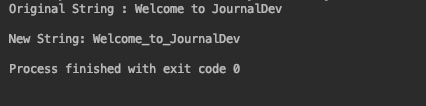
Original String : Welcome to JournalDev
New String: Welcome_to_JournalDev
隐藏文本中的数字 (Hiding numeric digits in a text )
The replaceAll() can hide numeric digits that appear in a string. The digits can be replaced by ‘*’.
replaceAll()可以隐藏出现在字符串中的数字。 这些数字可以用“ *”代替。
public class Main {
public static void main(String[] args) {
String s = "Hello! this is the number : 1234 ";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceAll("[0-9]","*");
System.out.println("New String: "+rep);
}
}
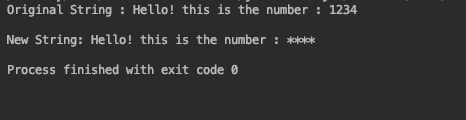
Original String : Hello! this is the number : 1234
New String: Hello! this is the number : ****
创建自定义消息 (Creating custom messages )
replaceAll() can replace the strings in a generic string to generate a custom message. Let’s take values from a map and generate a custom message.
replaceAll()可以替换通用字符串中的字符串以生成自定义消息。 让我们从地图中获取值并生成自定义消息。
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
String s = "dear #Employee#, your Current Salary is #Salary#.";
Map<String, String> vals = new HashMap<String, String>();
vals.put("#Employee#", "John");
vals.put("#Salary#", "12000");
for (String key : vals.keySet()) {
s = s.replaceAll(key, vals.get(key));
}
System.out.println(s);
}
}
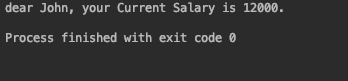
dear John, your Current Salary is 12000.
首先替换 (Replace First )
Unlike replceAll(), replaceFirst() only replaces the first instance of the pattern.
与replceAll()不同,replaceFirst()仅替换模式的第一个实例。
public class Main {
public static void main(String[] args) {
String s = "Welcome to JournalDev";
System.out.println("Original String : "+s);
System.out.println();
String rep = s.replaceFirst("o","@");
System.out.println("New String: "+rep);
}
}
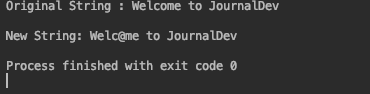
Original String: Welcome to JournalDev
New String: Welc@me to JournalDev
结论 (Conclusion)
The replaceAll() method replaces a regex with a string. This is different from the simple replace() method that only performs character substitutions. You can learn more about the repalceAll() function from its official documentation.
replaceAll()方法将正则表达式替换为字符串。 这与仅执行字符替换的简单replace()方法不同。 您可以从repalceAll()函数的正式文档中了解更多信息。
翻译自: https://www.journaldev.com/41936/java-string-replaceall