负数modulo运算
Java Modulo operator or modulus operator is used to getting the remainder when we divide an integer with another integer.
当我们将一个整数除以另一个整数时,使用Java Modulo运算符或模数运算符来获取余数。
Java Modulo运算符语法 (Java Modulo Operator Syntax)
The % character is the modulus operator in Java. Its syntax is:
%字符是Java中的模数运算符。 其语法为:
int remainder = int % int
The modulus operator always returns an integer. If we try to use the modulo operator with any other type of variable, we will get a compilation error.
模运算符始终返回整数。 如果我们尝试将模运算符与其他任何类型的变量一起使用,则会收到编译错误。
模运算符示例 (Modulus Operator Example)
Let’s look at a simple example of using the modulus operator to find the remainder when two integers are divided.
让我们看一个简单的示例,该示例使用模运算符查找两个整数相除后的余数。
package com.journaldev.java;
public class JavaModuloOperator {
public static void main(String[] args) {
int x = 10;
int y = 3;
int remainder = x % y;
System.out.println("10 % 3 = " + remainder);
}
}
使用Modulus Operator检查整数是偶数还是奇数 (Using Modulus Operator to check if an integer is even or odd)
One of the simple use cases of the modulus operator is to test if an integer is odd or even.
模运算符的一种简单使用情况是测试整数是否为奇数或偶数。
package com.journaldev.java;
import java.util.Scanner;
public class JavaModuloOperator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter an integer:");
int in = scanner.nextInt();
scanner.close();
if (in % 2 == 0) {
System.out.println(String.format("%d is even.", in));
}else {
System.out.println(String.format("%d is odd.", in));
}
}
}
Output:
输出:
Please enter an integer:
10
10 is even.
Please enter an integer:
9
9 is odd.
Here are the utility methods to check if the integer is even or odd.
这是检查整数是偶数还是奇数的实用方法。
public static boolean isEven(int x) {
return x % 2 == 0;
}
public static boolean isOdd(int x) {
return x % 2 != 0;
}
使用模量运算符检查一个整数是否被另一个整数除 (Using Modulus Operator to check if an integer is divided by another integer)
We can use the modulo operator to check if an integer is divided by another integer or not.
我们可以使用取模运算符来检查一个整数是否被另一个整数除。
package com.journaldev.java;
import java.util.Scanner;
public class JavaModuloOperator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter the first integer:");
int x = scanner.nextInt();
System.out.println("Please enter the second integer:");
int y = scanner.nextInt();
scanner.close();
if (x % y == 0) {
System.out.printf("%d is divided by %d", x, y);
} else {
System.out.printf("%d is not divided by %d", x, y);
}
}
}
Output:
输出:
Please enter the first integer:
10
Please enter the second integer:
5
10 is divided by 5
Here is the implementation of a utility method to check if an integer is divided by another integer or not.
这是检查一个整数是否被另一个整数除的实用程序方法的实现。
public static boolean isDivided(int x, int y) {
return x % y == 0;
}
具有负整数的模运算符 (Modulus Operator with negative integers)
When modulus operator is used with negative integers, the output retains the sign of the dividend.
当模运算符与负整数一起使用时,输出将保留被除数的符号。
jshell> 10 % 3
$66 ==> 1
jshell> -10 % 3
$67 ==> -1
jshell> 10 % -3
$68 ==> 1
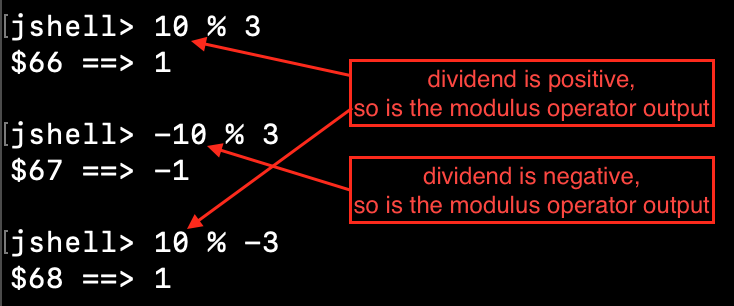
Java Modulo Operator Examples
Java Modulo运算符示例
翻译自: https://www.journaldev.com/31650/java-modulo-operator-modulus
负数modulo运算