排序算法python实现
Merge sort is one of the most efficient sorting algorithms. It works on the principle of Divide and Conquer based on the idea of breaking down a list into several sub-lists until each sublist consists of a single element and merging those sublists in a manner that results into a sorted list.
合并排序是最有效的排序算法之一。 它基于将一个列表分解为几个子列表,直到每个子列表由一个元素组成,然后将这些子列表合并为一个排序列表的思想,根据分而治之原理工作。
合并排序工作规则 (Merge Sort Working Rule)
The concept of Divide and Conquer involves three steps:
分而治之的概念涉及三个步骤:
- Divide the problem into multiple subproblems. 将问题分为多个子问题。
- Solve the Sub Problems. The idea is to break down the problem into atomic subproblems, where they are actually solved. 解决子问题。 想法是将问题分解为原子子问题,并在其中实际解决。
- Combine the solutions of the subproblems to find the solution of the actual problem. 结合子问题的解决方案以找到实际问题的解决方案。
So, the merge sort working rule involves the following steps:
因此,合并排序工作规则涉及以下步骤:
- Divide the unsorted array into subarray, each containing a single element. 将未排序的数组划分为子数组,每个子数组包含一个元素。
- Take adjacent pairs of two single-element array and merge them to form an array of 2 elements. 取相邻的两个单元素数组对,并将它们合并以形成2个元素的数组。
- Repeat the process till a single sorted array is obtained. 重复该过程,直到获得单个排序的数组。
An array of Size ‘N’ is divided into two parts ‘N/2’ size of each. then those arrays are further divided till we reach a single element.
大小为“ N”的数组分为两个部分,每个部分的大小为“ N / 2”。 然后将这些数组进一步划分,直到达到单个元素。
The base case here is reaching one single element. When the base case is hit, we start merging the left part and the right part and we get a sorted array at the end.
这里的基本情况是达到一个要素。 当遇到基本情况时,我们开始合并左部分和右部分,最后得到一个排序的数组。
Merge sort repeatedly breaks down an array into several subarrays until each subarray consists of a single element and merging those subarrays in a manner that results in a sorted array.
合并排序反复将一个数组分解为几个子数组,直到每个子数组由单个元素组成,然后以导致排序数组的方式合并这些子数组。
合并排序算法流程 (Merge Sort Algorithm Flow)
Array = {70,50,30,10,20,40,60}
数组= {70,50,30,10,20,40,60}
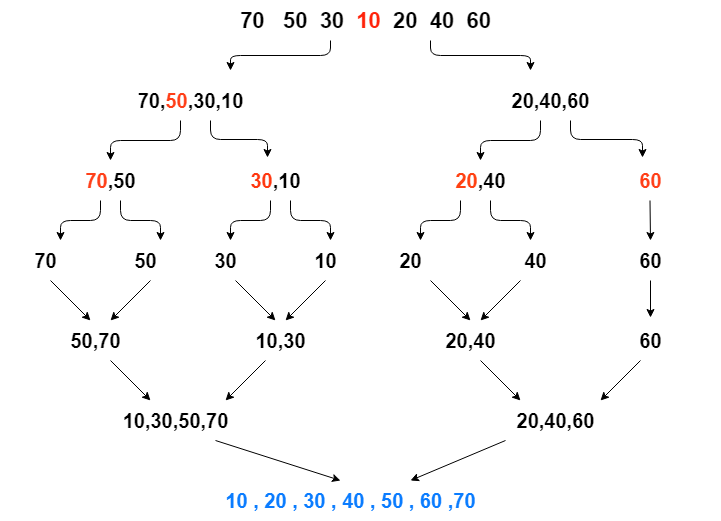
MergeSort
合并排序
We repeatedly break down the array in two parts, the left part, and the right part. the division takes place from the mid element.
我们反复将数组分为两部分,左部分和右部分。 划分从中间元素开始。
We divide until we reach a single element and then we start combining them to form a Sorted Array.
我们进行划分,直到达到单个元素,然后开始将它们组合以形成有序数组。
合并排序实现 (Merge Sort Implementations)
We will implement the merge sort algorithm in Java, C, and Python.
我们将在Java,C和Python中实现合并排序算法。
1. Java实现 (1. Java Implementation)
package com.journaldev.ds;
public class MergeSort {
public static void main(String[] args) {
int[] arr = { 70, 50, 30, 10, 20, 40, 60 };
int[] merged = mergeSort(arr, 0, arr.length - 1);
for (int val : merged) {
System.out.print(val + " ");
}
}
public static int[] mergeTwoSortedArrays(int[] one, int[] two) {
int[] sorted = new int[one.length + two.length];
int i = 0;
int j = 0;
int k = 0;
while (i < one.length && j < two.length) {
if (one[i] < two[j]) {
sorted[k] = one[i];
k++;
i++;
} else {
sorted[k] = two[j];
k++;
j++;
}
}
if (i == one.length) {
while (j < two.length) {
sorted[k] = two[j];
k++;
j++;
}
}
if (j == two.length) {
while (i < one.length) {
sorted[k] = one[i];
k++;
i++;
}
}
return sorted;
}
public static int[] mergeSort(int[] arr, int lo, int hi) {
if (lo == hi) {
int[] br = new int[1];
br[0] = arr[lo];
return br;
}
int mid = (lo + hi) / 2;
int[] fh = mergeSort(arr, lo, mid);
int[] sh = mergeSort(arr, mid + 1, hi);
int[] merged = mergeTwoSortedArrays(fh, sh);
return merged;
}
}
OUTPUT
输出值

Merge Sort Algorithm Java Implementation
合并排序算法Java实现
2. C实现 (2. C Implementation)
#include <stdio.h>
void merge(int arr[], int l, int m, int r)
{
int i, j, k;
int n1 = m - l + 1;
int n2 = r - m;
/* create temp arrays */
int L[n1], R[n2];
/* Copy data to temp arrays L[] and R[] */
for (i = 0; i < n1; i++)
L[i] = arr[l + i];
for (j = 0; j < n2; j++)
R[j] = arr[m + 1+ j];
/* Merge the temp arrays back into arr[l..r]*/
i = 0; // Initial index of first subarray
j = 0; // Initial index of second subarray
k = l; // Initial index of merged subarray
while (i < n1 && j < n2)
{
if (L[i] <= R[j])
{
arr[k] = L[i];
i++;
}
else
{
arr[k] = R[j];
j++;
}
k++;
}
/* Copy the remaining elements of L[], if there
are any */
while (i < n1)
{
arr[k] = L[i];
i++;
k++;
}
/* Copy the remaining elements of R[], if there
are any */
while (j < n2)
{
arr[k] = R[j];
j++;
k++;
}
}
/* l is for left index and r is the right index of the
sub-array of arr to be sorted */
void mergeSort(int arr[], int l, int r)
{
if (l < r)
{
// Same as (l+r)/2, but avoids overflow for
// large l and h
int m = l+(r-l)/2;
// Sort first and second halves
mergeSort(arr, l, m);
mergeSort(arr, m+1, r);
merge(arr, l, m, r);
}
}
void printArray(int A[], int size)
{
int i;
for (i=0; i < size; i++)
printf("%d ", A[i]);
printf("\n");
}
/* Driver program to test above functions */
int main()
{
int arr[] = {70, 50, 30, 10, 20, 40,60};
int arr_size = sizeof(arr)/sizeof(arr[0]);
printf("Given array is \n");
printArray(arr, arr_size);
mergeSort(arr, 0, arr_size - 1);
printf("\nSorted array is \n");
printArray(arr, arr_size);
return 0;
}
OUTPUT
输出值

Merge Sort C Implementation
合并Sort C实现
3. Python实现 (3. Python Implementation)
def merge_sort(unsorted_array):
if len(unsorted_array) > 1:
mid = len(unsorted_array) // 2 # Finding the mid of the array
left = unsorted_array[:mid] # Dividing the array elements
right = unsorted_array[mid:] # into 2 halves
merge_sort(left)
merge_sort(right)
i = j = k = 0
# data to temp arrays L[] and R[]
while i < len(left) and j < len(right):
if left[i] < right[j]:
unsorted_array[k] = left[i]
i += 1
else:
unsorted_array[k] = right[j]
j += 1
k += 1
# Checking if any element was left
while i < len(left):
unsorted_array[k] = left[i]
i += 1
k += 1
while j < len(right):
unsorted_array[k] = right[j]
j += 1
k += 1
# Code to print the list
def print_list(array1):
for i in range(len(array1)):
print(array1[i], end=" ")
print()
# driver code to test the above code
if __name__ == '__main__':
array = [12, 11, 13, 5, 6, 7]
print("Given array is", end="\n")
print_list(array)
merge_sort(array)
print("Sorted array is: ", end="\n")
print_list(array)
OUTPUT
输出值

Merge Sort Python Code
合并排序Python代码
合并排序时间和空间复杂性 (Merge Sort Time and Space Complexity)
1.空间复杂性 (1. Space Complexity)
Auxiliary Space: O(n)
Sorting In Place: No
Algorithm : Divide and Conquer
辅助空间: O(n)
到位排序:否
算法: 分而治之
2.时间复杂度 (2. Time Complexity)
Merge Sort is a recursive algorithm and time complexity can be expressed as following recurrence relation.
合并排序是一种递归算法,时间复杂度可以表示为以下递归关系。
T(n) = 2T(n/2) + O(n)
T(n)= 2T(n / 2)+ O(n)
The solution of the above recurrence is O(nLogn). The list of size N is divided into a max of Logn parts, and the merging of all sublists into a single list takes O(N) time, the worst-case run time of this algorithm is O(nLogn)
上述重复的解决方案是O(nLogn) 。 大小为N的列表被划分为最多Logn个部分,并且将所有子列表合并为一个列表需要O(N)时间,该算法的最坏运行时间是O(nLogn)
Best Case Time Complexity: O(n*log n)
最佳情况下时间复杂度: O(n * log n)
Worst Case Time Complexity: O(n*log n)
最坏情况下的时间复杂度: O(n * log n)
Average Time Complexity: O(n*log n)
平均时间复杂度: O(n * log n)
The time complexity of MergeSort is O(n*Log n) in all the 3 cases (worst, average and best) as the mergesort always divides the array into two halves and takes linear time to merge two halves.
在3种情况下(最差,平均和最佳),MergeSort的时间复杂度均为O(n * Log n),因为mergesort始终将数组分为两半,并花费线性时间来合并两半。
Further Readings:
进一步阅读:
- Merge Sort Wikipedia Page 合并排序维基百科页面
- Bubble Sort in Java Java中的冒泡排序
- Insertion Sort in Java Java中的插入排序
翻译自: https://www.journaldev.com/31541/merge-sort-algorithm-java-c-python
排序算法python实现