Python argparse module is the preferred way to parse command line arguments. Parsing command-line arguments is a very common task, which Python scripts do and behave according to the passed values.
Python argparse模块是解析命令行参数的首选方法。 解析命令行参数是一个非常常见的任务,Python脚本根据传递的值来执行和操作。
Python的argparse (Python argparse)
Python argparse is the recommended command-line argument parsing module in Python. It is very common to the getopt
module but that is a little complicated and usually need more code for the same task.
Python argparse是Python中推荐的命令行参数解析模块。 这在getopt
模块中非常常见,但这有点复杂,并且通常需要更多代码来完成同一任务。
Let us go through various ways in which we can parse command-line arguments using this module.
让我们研究一下使用此模块可以解析命令行参数的各种方法。
为什么我们需要Python argparse模块? (Why we need Python argparse module?)
We will try to establish our need for argparse
module by looking at a simple example of using sys module:
通过查看使用sys模块的简单示例,我们将尝试建立对argparse
模块的需求:
import sys
if len(sys.argv) > 1:
print("Argument 1: {}".format(sys.argv[0]))
print("Argument 2: {}".format(sys.argv[1]))
else:
print("No arguments passed.")
With no arguments passed, the output will be:
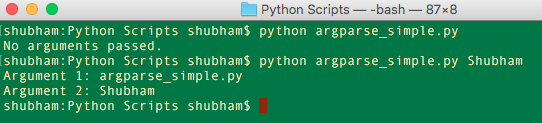
没有传递任何参数,输出将是:
Clearly, even the script name is caught a command-line parameter as well because, well, that’s what it is for python
.
显然,即使脚本名也被捕获为命令行参数,因为,这就是python
的含义。
Python argparse示例 (Python argparse example)
Now, you must be thinking that the above example was simple in terms of getting the arguments and using them. But thing is, when the script needs the parameters and they are not passed to it, that’s when the problems start.
现在,您必须考虑到上面的示例在获取参数和使用参数方面很简单。 但是事实是,当脚本需要参数而没有将参数传递给它时,问题就开始了。
With argparse, we can gracefully handle the absence and presence of parameters. Let’s study a simple example:
使用argparse,我们可以很好地处理参数的不存在和存在。 让我们研究一个简单的例子:
import argparse
parser = argparse.ArgumentParser()
parser.parse_args()
Let’s run the script various times with different options to see what it leads to:
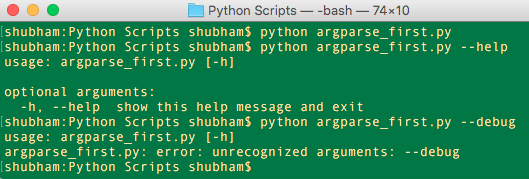
让我们使用不同的选项来多次运行脚本,以查看其结果:
Let’s understand what happened when we ran the script:
让我们了解一下运行脚本时发生的情况:
- Firstly, we ran the program with no arguments. In this case, the script remained silent. 首先,我们不带参数地运行程序。 在这种情况下,脚本保持沉默。
- The
--help
option is the only option which we need not specify with argparse as optional or required.--help
选项是我们不需要将argparse指定为optional或required的唯一选项。 - In last case, we used an unknown option which argparse,
claimed asunrecognized arguments
. 在最后一种情况下,我们使用了一个未知选项argparse,
声称是unrecognized arguments
。
So, with argparse, we can define which arguments to expect and which are optional.
因此,使用argparse,我们可以定义期望的参数和可选的参数。
Python argparse位置参数 (Python argparse positional arguments)
Now, in our scripts, it will be often when we need an argument which is mandatorily be passed to the script on execution. We will see an error if not passed. Let’s see an example:
现在,在脚本中,通常需要我们在执行时强制将其传递给脚本的参数。 如果未通过,我们将看到错误。 让我们来看一个例子:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("blog")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
else:
print("Didn't make it!")
When we run the script with different parameters, none, correct and something else:
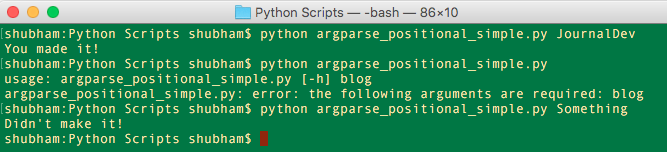
当我们使用不同的参数运行脚本时,无,更正等:
So, this way we can show an error when the argument is not passed and manage different values as well when it is passed.
因此,通过这种方式,我们可以在不传递参数时显示错误,并在传递参数时也管理不同的值。
Python argparse位置参数默认值 (Python argparse positional arguments default values)
In our last example, positional argument value is empty when not provided. But sometimes we want default values of a variable or argument, we can do that with argparse module.
在我们的最后一个示例中,未提供位置参数值是空的。 但是有时候我们想要变量或参数的默认值,我们可以使用argparse模块来实现。
See an example on how to pass default value for an argument:
请参阅有关如何为参数传递默认值的示例:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('blog', nargs='?', default="JournalDev")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
else:
print("Didn't make it!")
When we run the script with different parameters, none, correct and something else:

当我们使用不同的参数运行脚本时,无,更正等:
This time, absence of a parameter was gracefully managed as default values were passed.
这次,随着默认值的传递,可以很好地管理缺少参数的情况。
Python argparse参数帮助 (Python argparse argument help)
Whenever we make a new Python script, we know what arguments to pass and what is the fate of each argument. But what about a user who knows nothing about our script? How does he know what arguments to pass, in which order and what they do?
每当我们制作一个新的Python脚本时,我们就知道要传递什么参数,以及每个参数的命运。 但是,对于我们的脚本一无所知的用户呢? 他如何知道要传递哪些论据,按什么顺序执行什么工作?
This is where another attribute in add_argument
function comes to the rescue:
这是在add_argument
函数中的另一个属性可以解决的地方:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('blog', default="JournalDev", help="Best blog name here.")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
else:
print("Didn't make it!")
Now, when we run our script as (-h
is for help):
现在,当我们以( -h
寻求帮助)运行脚本时:
python argparse_positional_help.py -h
We get the following output:
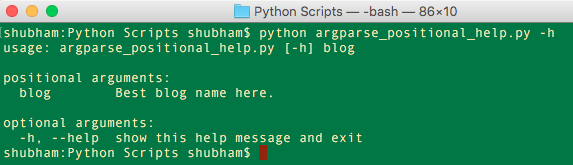
我们得到以下输出:
Isn’t that good? This way, the user of the script just needs to see what he/she should pass and in what order. Excellent!
这样不好吗 这样,脚本的用户只需要查看他/她应通过的内容和顺序即可。 优秀的!
位置参数数据类型 (Positional arguments Data Type)
Now, Positional arguments are always treated as Strings, unless you tell Python not to. Let’s see this with a sample code snippet:
现在,除非您告诉Python不这样做,否则位置参数始终被视为字符串。 让我们看一下示例代码片段:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('number', help="Enter number to triple it.")
args = parser.parse_args()
print(args.number*3)
In this script, we are just multiplying a number with three. When we run this with 3
as the input, we get the following output:

在此脚本中,我们只是将数字乘以三。 当我们以3
作为输入运行时,我们得到以下输出:
That happended because Python treated 3
as a String
, so it just appended the same String 3
time.
发生这种情况是因为Python将3
视为String
,所以它只是将相同的String 3
附加了一次。
This can be corrected if we inform Python of the datatype as with the help of type
attribute:
如果我们借助type
属性将数据类型告知Python,则可以更正此错误:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('number', help="Enter number to cube.", type=int)
args = parser.parse_args()
print(args.number*3)
This time when we run this script with 3
as the input, we get the following output:

这次,当我们以3
作为输入运行此脚本时,我们得到以下输出:
This way, we can even ensure that the passed data types are correct.
这样,我们甚至可以确保传递的数据类型正确。
Python argparse可选参数 (Python argparse optional arguments)
Now, in our scripts, it will be often when we need an argument which is optional be passed to the script. We will NOT see an error if not passed. Let’s see an example:
现在,在脚本中,通常需要将可选参数传递给脚本时。 如果未通过,我们将不会看到错误。 让我们来看一个例子:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--blog', help="Best blog name here.")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
This time, we used --
in the optional argument name. When we run the script:

这次,我们在可选参数名称中使用了--
。 当我们运行脚本时:
With the --
before the optional parameter name, we can define optional parameters in any order.
在可选参数名称之前使用--
,我们可以按任意顺序定义可选参数。
The method for passing default values, help messages and data types for optional parameters is same as in positional parameters. Just a point to be noted, if no value is passed to an optional argument, it is assigned a value of None
for the program.
传递默认值,帮助消息和可选参数的数据类型的方法与位置参数中的方法相同。 只是要注意一点,如果没有将值传递给可选参数,则该值将为程序分配为None
。
argparse可选参数的简称 (Short names for Optional arguments with argparse)
In our example above, we were very clear on what value we needed the user to be passed, optionally. That is nice but what if the descriptive name of the optional parameters in our scripts grows long. Fortunately, we can assign a short name to parameters as well. Let’s see an example snippet:
在上面的示例中,我们非常清楚需要可选地传递用户什么值。 很好,但是如果脚本中可选参数的描述性名称长了,该怎么办。 幸运的是,我们也可以为参数分配一个短名称。 让我们看一个示例片段:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-b', '--blog', help="Best blog name here.")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
Wasn’t that simple? Just use an extra parameter in add_argument function and it’s done. let’s run this script now:
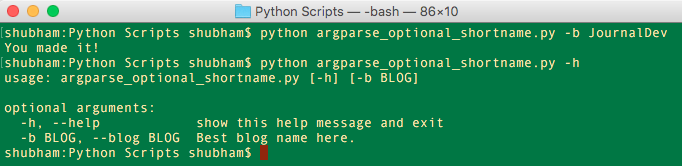
那不是那么简单吗? 只需在add_argument函数中使用一个额外的参数即可。 让我们现在运行此脚本:
将可选参数和位置参数与argparse结合 (Combining Optional and Positional parameters with argparse)
We can also combine the use of optional and positional command-line parameters in our script to be used. Let’s quickly see an example:
我们还可以在脚本中结合使用可选和位置命令行参数。 让我们快速看一个例子:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('blog', help="Best blog name here.")
parser.add_argument('-w', '--writer', help="Team Player.")
args = parser.parse_args()
if args.blog == 'JournalDev':
print('You made it!')
if args.writer == 'Shubham':
print('Technical Author.')
When we run the script:

当我们运行脚本时:
In this lesson, we learned about various ways through which we can manage the command-line parameters with Argbase module in Python.
在本课程中,我们学习了可以使用Python中的Argbase模块管理命令行参数的各种方法。
Reference: API Doc
参考: API文档