kotlin数据类
In this tutorial, we’ll look at Kotlin Data Class. If you haven’t read the Kotlin Classes post, we recommend you to do so before proceeding.
在本教程中,我们将研究Kotlin数据类。 如果您还没有阅读Kotlin Classes帖子,我们建议您在继续之前阅读。
Kotlin数据类 (Kotlin Data Class)
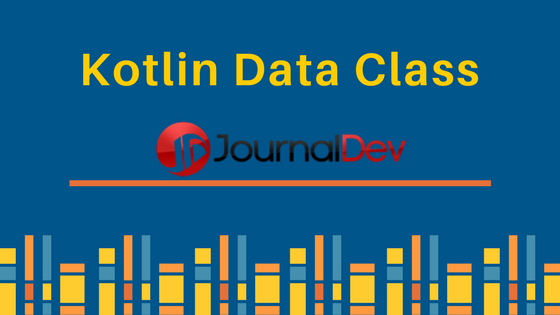
Do you get tired of writing thousands of lines of code for your POJO data classes in Java?
您是否厌倦了用Java为POJO数据类编写数千行代码?
Every Java Programmer at some stage must have taken a note of the number of lines of code they need to write for classes that just need to store some data. Let’s see how a Book.java
POJO class looks like:
每个Java程序员在某个阶段都必须记下他们需要为只需要存储一些数据的类编写的代码行数。 让我们看看Book.java
POJO类的样子:
public class Book {
private String name;
private String authorName;
private long lastModifiedTimeStamp;
private float rating;
private int downloads;
public Book(String name, String authorName, long lastModified, float rating, int downloads) {
this.name = name;
this.authorName = authorName;
this.lastModifiedTimeStamp = lastModified;
this.rating = rating;
this.downloads = downloads;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthorName() {
return authorName;
}
public void setAuthorName(String authorName) {
this.authorName = authorName;
}
public long getLastModifiedTimeStamp() {
return lastModifiedTimeStamp;
}
public void setLastModifiedTimeStamp(long lastModifiedTimeStamp) {
this.lastModifiedTimeStamp = lastModifiedTimeStamp;
}
public float getRating() {
return rating;
}
public void setRating(float rating) {
this.rating = rating;
}
public int getDownloads() {
return downloads;
}
public void setDownloads(int downloads) {
this.downloads = downloads;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Book that = (Book) o;
if (downloads != that.downloads)
return false;
if (name != null ? !name.equals(that.name) :
that.name != null) {
return false;
}
return authorName != null ?
authorName.equals(that.authorName) :
that.authorName == null;
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + (authorName != null ?
authorName.hashCode() : 0);
result = 31 * result + downloads;
return result;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + authorName + '\''