python集合
Python collections module comes with with a number of container data types. These data types have different capabilities, as we will learn in this post. Let’s study about python collections module and it’s most important and widely used data types.
Python集合模块附带了许多容器数据类型。 这些数据类型具有不同的功能,我们将在本文中学习。 让我们研究python集合模块,它是最重要且使用最广泛的数据类型。
Python集合模块 (Python Collections Module)
The collections which we will study in python collections module are:
我们将在python集合模块中研究的集合是:
- OrderedDict OrderedDict
- defaultdict defaultdict
- counter 计数器
- namedtuple 元组
- deque 双端队列
Let’s get started.
让我们开始吧。
1. OrderedDict (1. OrderedDict)
With an OrderedDict
, the order of insertion is maintained when key and values are inserted into the dictionary. If we try to insert a key again, this will overwrite the previous value for that key.
使用OrderedDict
,当将键和值插入到字典中时,将保持插入顺序。 如果我们尝试再次插入密钥,这将覆盖该密钥的先前值。
Here is a sample program to demonstrate the usage of an OrderedDict
:
这是一个示例程序,用于演示OrderedDict
的用法:
from collections import OrderedDict
roll_no = OrderedDict([
(11, 'Shubham'),
(9, 'Pankaj'),
(17, 'JournalDev'),
])
for key, value in roll_no.items():
print(key, value)
Let’s see the output for this program:
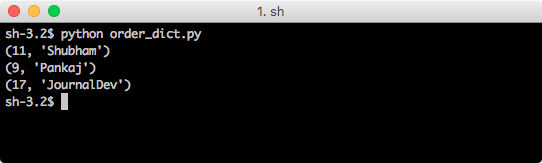
Notice that the output order was exactly the same as the order of insertion.
让我们看一下该程序的输出:
请注意,输出顺序与插入顺序完全相同。
2.默认字典 (2. Default Dict)
The default dictionary can contain duplicate keys. The advantage of using the default dictionary is that we can collect items which belong to the same key. Let’s look at a code snippet which demonstrates the same:
默认词典可以包含重复的键。 使用默认字典的优点是我们可以收集属于同一键的项目。 让我们看一下演示相同内容的代码片段:
from collections import defaultdict
marks = [
('Shubham', 89),
('Pankaj', 92),
('JournalDev', 99),
('JournalDev', 98)
]
dict_marks = defaultdict(list)
for key, value in marks:
dict_marks[key].append(value)
print(list(dict_marks.items()))
Let’s see the output for this program:
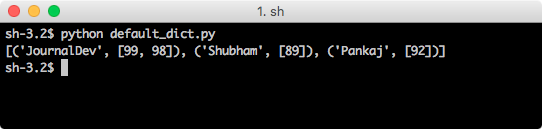
The key
JournalDev
was used two times and values for the same was collected once we printed the dictionary.
让我们看一下该程序的输出:
两次使用了键JournalDev
,并且在我们打印字典JournalDev
收集了相同的值。
3.柜台 (3. Counter)
The Counter collections allow us to keep a count of all the items which are inserted into the collection with the keys. Here is a sample program to show how it works:
Counter集合使我们可以保留所有使用键插入到集合中的项目的计数。 这是一个示例程序,说明其工作方式:
from collections import Counter
marks_list = [
('Shubham', 89),
('Pankaj', 92),
('JournalDev', 99),
('JournalDev', 98)
]
count = Counter(name for name, marks in marks_list)
print(count)
Let’s see the output for this program:
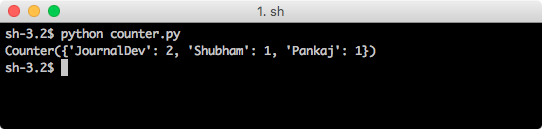
This way, we were able to count the number of times a key appeared in the list.
让我们看一下该程序的输出:
这样,我们就可以计算出密钥在列表中出现的次数。
4.命名元组 (4. Named Tuple)
As we already know, Python Tuples are immutable lists. This means that a value cannot be given to a key which aready exists in the tuple. First, let’s see how a Tuple can be made in Python:
众所周知, Python元组是不可变的列表。 这意味着不能将值赋给元组中存在区域的键。 首先,让我们看看如何用Python制作元组:
shubham = ('Shubham', 23, 'M')
print(shubham)
Let’s see the output for this program:
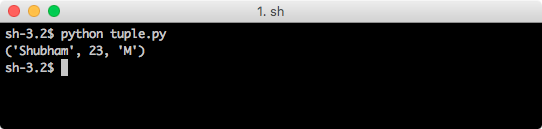
We can convert this Tuple to a Named tuple by assigning a name to all values present in this tuple. This will give a lot more context to the data present as well:
让我们看一下该程序的输出:
通过为该元组中存在的所有值分配名称,可以将该元组转换为命名元组。 这也将为当前数据提供更多上下文:
import collections
User = collections.namedtuple('User', 'name age gender')
shubham = User(name='Shubham', age=23, gender='M')
print(shubham)
print('Name of User: {0}'.format(shubham.name))
Output for this program will be:
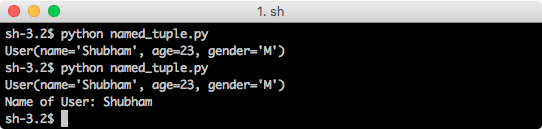
See how we can access properties of a named tuple with the name we provide. Also, remember that the key names cannot be Python keywords.
该程序的输出为:
了解如何使用我们提供的名称访问命名元组的属性。 另外,请记住, 键名不能是Python关键字 。
5.双端队列 (5. Deque)
A Deque is a double-ended queue which allows us to add and remove elements from both the ends. This enhances the capabilities of a stack or a queue. Here is a sample program:
双端队列是双端队列,它允许我们从两端添加和删除元素。 这增强了堆栈或队列的功能。 这是一个示例程序:
import collections
name = collections.deque('Shubham')
print('Deque :', name)
print('Queue Length:', len(name))
print('Left part :', name[0])
print('Right part :', name[-1])
name.remove('b')
print('remove(b):', name)
Let’s see the output for this program:

So, the dequeueing of the elements was done automatically. We can also insert elements in a Dequeue on a specific end. Let’s try it:
让我们看一下该程序的输出:
因此,元素的出队是自动完成的。 我们还可以在特定端的出队中插入元素。 让我们尝试一下:
import collections
name = collections.deque('Shubham')
print('Deque :', name)
name.extendleft('...')
name.append('-')
print('Deque :', name)
Let’s see the output for this program:

让我们看一下该程序的输出:
结论 (Conclusion)
In this post, we learned how we can manage data in Python and can use the collections module to make a lot of our operations easy.
在本文中,我们学习了如何在Python中管理数据以及如何使用collections模块使许多操作变得容易。
Reference: API Doc
参考: API文档
python集合