python入门指南
This article comprehensively covers the different logic gates in Python. Logic gates are the most basic materials to implement digital components. The use of logic gates ranges from computer architecture to the field of electronics.
本文全面介绍了Python中的不同逻辑门。 逻辑门是实现数字组件的最基本材料。 逻辑门的使用范围从计算机体系结构到电子领域。
These gates deal with binary values, either 0 or 1. Different types of gates take different numbers of input, but all of them provide a single output. These logic gates when combined form complicated circuits.
这些门处理0或1的二进制值。不同类型的门采用不同数量的输入,但它们都提供单个输出。 这些逻辑门组合在一起会形成复杂的电路。
Let us try to implement logic gates in Python Language.
让我们尝试用Python语言实现逻辑门。
Python中的基本逻辑门 (Basic Logic Gates in Python)
There are three most basic logic gates in circuit development.
电路开发中有三个最基本的逻辑门。
或门 (OR Gate)
This gate provides the output as 1 if either of the inputs is 1. It is similar to an “addition” operation, with respect to binary numbers.
如果输入中的任意一个为1,则此门输出为1。就二进制数而言,此门类似于“加法”运算。
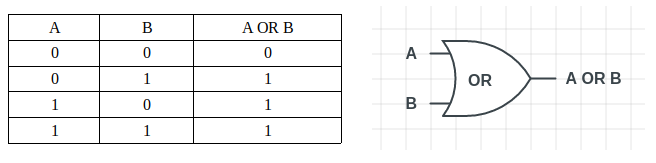
The table displayed above is the truth table. It is used to showcase all the combinations of values for inputs to a 'OR'
gate. The figure alongside the table denotes an 'OR'
gate.
上面显示的表是真值表。 它用于展示'OR'
门输入的所有值组合。 表格旁边的数字表示'OR'
门。
Implementation in Python:
用Python实现:
# Function to simulate OR Gate
def OR(A, B):
return A | B
print("Output of 0 OR 0 is", OR(0, 0))
print("Output of 0 OR 1 is", OR(0, 1))
print("Output of 1 OR 0 is", OR(1, 0))
print("Output of 1 OR 1 is", OR(1, 1))
We get the following output:
我们得到以下输出:
Output of 0 OR 0 is 0
Output of 0 OR 1 is 1
Output of 1 OR 0 is 1
Output of 1 OR 1 is 1
与门 (AND Gate)
This gate provides an output of 0 if either of the inputs are 0. This operation is considered as multiplication in binary numbers.
如果两个输入中的任何一个为0,则此门的输出为0。此操作被视为二进制数的乘法。
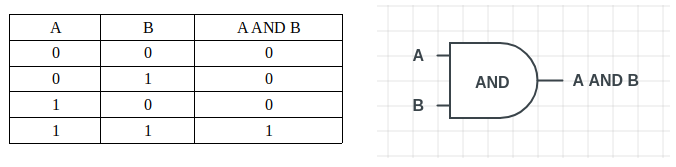
We can see in the truth table that whenever either of the two inputs is 0, the output is 0 too. The alongside figure denotes the 'AND'
gate.
我们可以在真值表中看到,只要两个输入中的任何一个为0,输出也为0。 旁边的数字表示'AND'
门。
Implementation in Python:
用Python实现:
# Function to simulate AND Gate
def AND(A, B):
return A & B
print("Output of 0 AND 0 is", AND(0, 0))
print("Output of 0 AND 1 is", AND(0, 1))
print("Output of 1 AND 0 is", AND(1, 0))
print("Output of 1 AND 1 is", AND(1, 1))
Output:
输出:
Output of 0 AND 0 is 0
Output of 0 AND 1 is 0
Output of 1 AND 0 is 0
Output of 1 AND 1 is 1
非门 (NOT Gate)
This gate provides the negation of the input given. This gate supports only a single input.
此门提供给定输入的求反。 该门仅支持单个输入。

The above table clearly displays the reversal of bits. The adjoining figure represents the 'NOT'
gate.
上表清楚地显示了位的反转。 相邻的图代表'NOT'
门。
Python implementation of binary NOT Gate:
二进制NOT Gate的Python实现:
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
print("Output of NOT 0 is", NOT(0))
print("Output of NOT 1 is", NOT(1))
Output:
输出:
Output of NOT 0 is 1
Output of NOT 1 is 0
Note: The
'NOT()'
function provides correct results for bit values 0 and 1.注意:
'NOT()'
函数为位值0和1提供正确的结果。
Python中的通用逻辑门 (Universal Logic Gates in Python)
There are two universal logic gates, 'NAND'
and 'NOR'
. They are named universal because any boolean circuit can be implemented using only these gates.
有两个通用逻辑门'NAND'
和'NOR'
。 之所以将它们命名为通用,是因为仅使用这些门就可以实现任何布尔电路。
与非门 (NAND Gate)
The 'NAND'
gate is a combination of 'AND'
gate followed by 'NOT'
gate. Opposite to 'AND'
gate, it provides an output of 0 only when both the bits are set, otherwise 1.
所述'NAND'
门是组合'AND'
接着栅极'NOT'
门。 与'AND'
门相对,仅当两个位置1时,它提供0输出,否则为1。
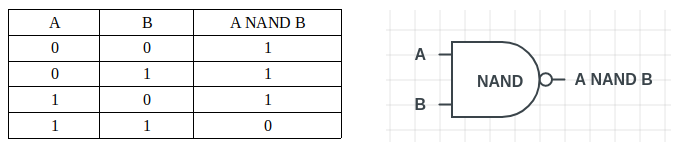
In Python, 'NAND()'
function can be implemented using the 'AND()'
and 'OR()'
functions created before.
在Python中,可以使用之前创建的'AND()'
和'OR()'
函数来实现'NAND()'
函数。
# Function to simulate AND Gate
def AND(A, B):
return A & B;
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate NAND Gate
def NAND(A, B):
return NOT(AND(A, B))
print("Output of 0 NAND 0 is", NAND(0, 0))
print("Output of 0 NAND 1 is", NAND(0, 1))
print("Output of 1 NAND 0 is", NAND(1, 0))
print("Output of 1 NAND 1 is", NAND(1, 1))
We get the following output:
我们得到以下输出:
Output of 0 NAND 0 is 1
Output of 0 NAND 1 is 1
Output of 1 NAND 0 is 1
Output of 1 NAND 1 is 0
或非门 (NOR Gate)
The 'NOR'
gate is a result of cascading of 'OR'
gate followed by 'NOT'
gate. Contrary to 'OR'
gate, it provides an output of 1, when all the inputs are 0.
'NOR'
门是'OR'
门与'NOT'
门级联的结果。 与'OR'
门相反,当所有输入均为0时,它提供的输出为1。
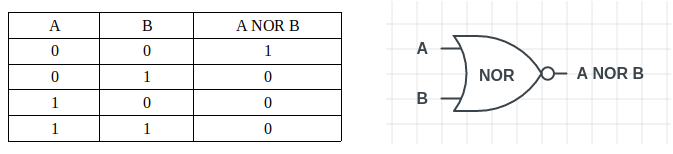
Similar to 'NAND()'
function, 'NOR()'
can be implemented using already created functions.
与'NAND()'
函数相似, 'NOR()'
可以使用已创建的函数实现。
# Function to calculate OR Gate
def OR(A, B):
return A | B;
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate NOR Gate
def NOR(A, B):
return NOT(OR(A, B))
print("Output of 0 NOR 0 is", NOR(0, 0))
print("Output of 0 NOR 1 is", NOR(0, 1))
print("Output of 1 NOR 0 is", NOR(1, 0))
print("Output of 1 NOR 1 is", NOR(1, 1))
Output:
输出:
Output of 0 NOR 0 is 1
Output of 0 NOR 1 is 0
Output of 1 NOR 0 is 0
Output of 1 NOR 1 is 0
Python中的独家逻辑门 (Exclusive Logic Gates in Python)
There are two special types of logic gates, XOR and XNOR, that focus on the number of inputs of 0 or 1, rather than individual values.
逻辑门有两种特殊类型,即XOR和XNOR,它们专注于0或1的输入数量,而不是单个值。
异或门 (XOR Gate)
An acronym for Exclusive-OR, 'XOR'
gate provides an output of 1 when the number of 1s in the input is odd.
当输入中的1的数量为奇数时, 'XOR'
门的缩写为'XOR'
。
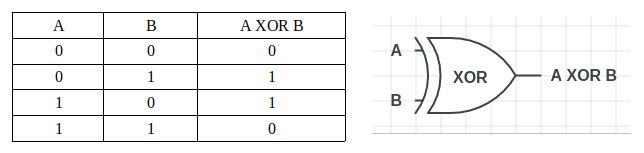
We can clearly see the output for the XOR Gate in the table above. It provides an output of 1 when the number of ones in the input is 1, that is odd.
我们可以在上表中清楚地看到XOR门的输出。 当输入中的数字为1时,它提供的输出为1,这是奇数。
We can implement 'XOR()'
function easily in Python by:
我们可以通过以下方式在Python中轻松实现'XOR()'
功能:
# Function to simulate XOR Gate
def XOR(A, B):
return A ^ B
print("Output of 0 XOR 0 is", XOR(0, 0))
print("Output of 0 XOR 1 is", XOR(0, 1))
print("Output of 1 XOR 0 is", XOR(1, 0))
print("Output of 1 XOR 1 is", XOR(1, 1))
We get the following output:
我们得到以下输出:
Output of 0 XOR 0 is 0
Output of 0 XOR 1 is 1
Output of 1 XOR 0 is 1
Output of 1 XOR 1 is 0
异或门 (XNOR Gate)
It is formed as a result of the combination of 'XOR'
and 'NOT'
gates. Opposite to 'XOR'
, it provides an output of 1, when the number of 1s in the input is even.
它是'XOR'
和'NOT'
门组合的结果。 与'XOR'
相反,当输入中1的数量为偶数时,它提供1的输出。
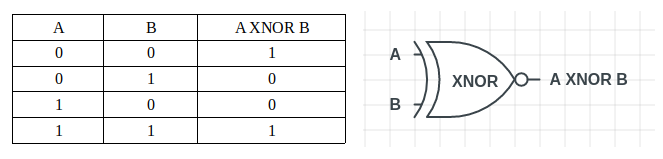
The 'XNOR()'
function can be implemented by using the 'XOR()'
and 'NOT()'
functions in Python.
可以通过使用Python中的'XOR()'
和'NOT()'
函数来实现'XNOR()'
函数。
# Function to simulate XOR Gate
def XOR(A, B):
return A ^ B
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate XNOR Gate
def XNOR(A, B):
return NOT(XOR(A, B))
print("Output of 0 XNOR 0 is", XNOR(0, 0))
print("Output of 0 XNOR 1 is", XNOR(0, 1))
print("Output of 1 XNOR 0 is", XNOR(1, 0))
print("Output of 1 XNOR 1 is", XNOR(1, 1))
Output:
输出:
Output of 0 XNOR 0 is 1
Output of 0 XNOR 1 is 0
Output of 1 XNOR 0 is 0
Output of 1 XNOR 1 is 1
结论 (Conclusion)
The implementation of logic gates in Python is very easy. As a programmer, you need to be aware of logic gates and operators in Python. We hope that this article enlightened the reader about the basics and execution of logic gates in Python.
用Python实现逻辑门非常容易。 作为程序员,您需要了解Python中的逻辑门和运算符。 我们希望本文能使读者对Python逻辑门的基础知识和执行有所启发。
For further reading, check out our other Python tutorials.
要进一步阅读,请查看我们的其他Python教程 。
python入门指南