In this tutorial we will cover basics of python while loop. In the previous tutorial, we learned about Python for loop.
在本教程中,我们将介绍python while循环的基础知识。 在上一教程中,我们了解了Python for loop 。
Python的while循环 (Python while loop)
Python while loop is used to repeatedly execute some statements until the condition is true. So the basic structure of python while loop is:
Python while循环用于重复执行某些语句,直到条件成立为止。 所以python while循环的基本结构是:
While condition :
#Start of the statements
Statement
. . . . . . .
Statement
#End of the Statements
else :
#this scope is optional
#This statements will be executed if the condition
#written to execute while loop is false
For example, the following code will give you some ideas about the while loop. In this example, we are printing numbers from 1 to 4 inside loop and 5 in outside of the loop
例如,以下代码将为您提供有关while循环的一些想法。 在此示例中,我们在循环内打印从1到4的数字,在循环外打印5的数字
cnt=1 #this is the initial variable
while cnt < 5 :
#inside of while loop
print (cnt,"This is inside of while loop")
cnt+=1
else :
#this statement will be printed if cnt is equals to 5
print (cnt, "This is outside of while loop")
Output

输出量
In the example of for loop tutorial, we print each letter from words. We can implement that code use while loop. The following code will show you that.
在for循环教程的示例中,我们从单词中打印每个字母。 我们可以使用while循环实现该代码。 以下代码将向您展示。
word="anaconda"
pos=0 #initial position is zero
while pos < len(word) :
print (word[pos])
#increment the position after printing the letter of that position
pos+=1
Output
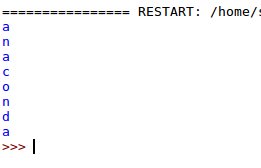
输出量
An interesting fact about the loop is if you implement something using for loop, you can implement that in a while loop too. Try to implement examples shown in for loop tutorial in a while loop.
关于循环的一个有趣的事实是,如果您使用for循环实现某些功能,则也可以在while循环中实现该功能。 尝试在while循环中实现for循环教程中显示的示例。
Python嵌套while循环 (Python Nested while loop)
You can write while loop inside another while loop. Suppose you need to print a pattern like this
您可以在另一个while循环内编写while循环。 假设您需要打印这样的图案
1 2
1 2
1 2 3
1 2 3
1 2 3 4
1 2 3 4
1 2 3 4 5
1 2 3 4 5
The following code will illustrate how to implement that using nested while loop.
以下代码将说明如何使用嵌套的while循环实现该功能。
line=1 #this is the initial variable
while line <= 5 :
pos = 1
while pos < line:
#This print will add space after printing the value
print pos,
#increment the value of pos by one
pos += 1
else:
#This print will add newline after printing the value
print pos
#increment the value of line by one
line += 1
Python while循环无限问题 (Python while loop infinite problem)
Since the while loop will continue to run until the condition becomes false, you should make sure it does otherwise program will never end. Sometimes it can come handy when you want your program to wait for some input and keep checking continuously.
由于while循环将继续运行直到条件变为false为止,因此您应确保它确实如此,否则程序将永远不会结束。 有时,当您希望程序等待一些输入并不断进行检查时,它可能会派上用场。
var = 100
while var == 100 : # an infinite loop
data = input("Enter something:")
print ("You entered : ", data)
print ("Good Bye Friend!")
If you run the above program, it will never end and you will have to kill it using the Ctrl+C keyboard command.
如果运行上述程序,它将永远不会结束,并且您将不得不使用Ctrl + C键盘命令将其杀死。
>>>
================= RESTART: /Users/pankaj/Desktop/infinite.py =================
Enter something:10
You entered : 10
Enter something:20
You entered : 20
Enter something:
Traceback (most recent call last):
File "/Users/pankaj/Desktop/infinite.py", line 3, in <module>
data = input("Enter something:")
KeyboardInterrupt
>>>
That’s all about python while loop example tutorial. For any queries please comment below.
这就是关于python while循环示例教程的全部内容。 如有任何疑问,请在下面评论。