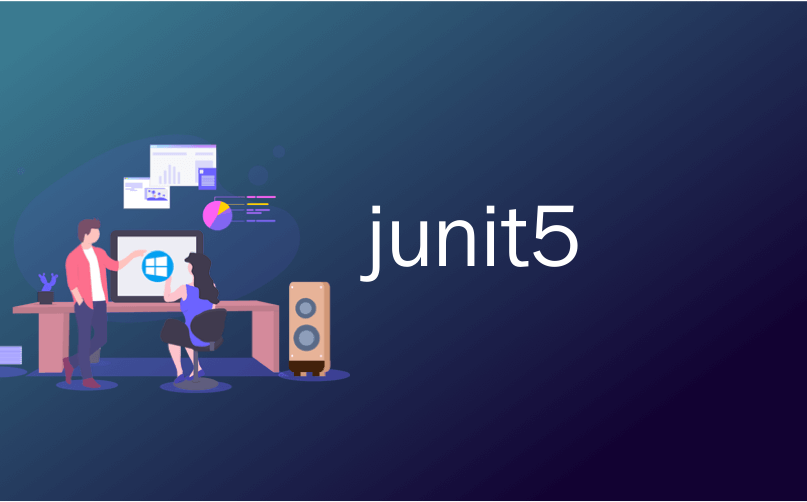
junit5
Java 8的AssertJ 3.0.0发行版比以前更容易测试异常。 在我以前的一篇博客文章中,我描述了如何利用纯Java 8实现此目的,但是使用AssertJ 3.0.0可能会删除我创建的许多代码。
警告:此博客文章主要包含代码示例。
SUT –被测系统
我们将测试以下2类抛出的异常。
第一个:
class DummyService {
public void someMethod() {
throw new RuntimeException("Runtime exception occurred");
}
public void someOtherMethod(boolean b) {
throw new RuntimeException("Runtime exception occurred",
new IllegalStateException("Illegal state"));
}
}
第二个:
class DummyService2 {
public DummyService2() throws Exception {
throw new Exception("Constructor exception occurred");
}
public DummyService2(boolean dummyParam) throws Exception {
throw new Exception("Constructor exception occurred");
}
}
assertThatThrownBy()示例
注意:为了使以下代码正常工作,需要静态导入org.assertj.core.api.Assertions.assertThatThrownBy
。
@Test
public void verifiesTypeAndMessage() {
assertThatThrownBy(new DummyService()::someMethod)
.isInstanceOf(RuntimeException.class)
.hasMessage("Runtime exception occurred")
.hasNoCause();
}
@Test
public void verifiesCauseType() {
assertThatThrownBy(() -> new DummyService().someOtherMethod(true))
.isInstanceOf(RuntimeException.class)
.hasMessage("Runtime exception occurred")
.hasCauseInstanceOf(IllegalStateException.class);
}
@Test
public void verifiesCheckedExceptionThrownByDefaultConstructor() {
assertThatThrownBy(DummyService2::new)
.isInstanceOf(Exception.class)
.hasMessage("Constructor exception occurred");
}
@Test
public void verifiesCheckedExceptionThrownConstructor() {
assertThatThrownBy(() -> new DummyService2(true))
.isInstanceOf(Exception.class)
.hasMessage("Constructor exception occurred");
}
提出的断言来自AbstractThrowableAssert
,您可以使用更多的断言!
没有抛出异常!
以下测试将失败,因为不会引发任何异常:
@Test
public void failsWhenNoExceptionIsThrown() {
assertThatThrownBy(() -> System.out.println());
}
消息是:
java.lang.AssertionError: Expecting code to raise a throwable.
AAA风格
如果您希望区分测试的行动阶段和断言阶段以提高可读性,则还可以:
@Test
public void aaaStyle() {
// arrange
DummyService dummyService = new DummyService();
// act
Throwable throwable = catchThrowable(dummyService::someMethod);
// assert
assertThat(throwable)
.isNotNull()
.hasMessage("Runtime exception occurred");
}
参考文献
- GitHub上提供了本文的源代码(请查看
com.github.kolorobot.assertj.exceptions
包) - AssertJ 3.0.0 for Java 8版本
翻译自: https://www.javacodegeeks.com/2015/04/junit-testing-exceptions-with-java-8-and-assertj-3-0-0.html
junit5