python中处理文件的包
Not in every case data is supposed to be taken directly from the user. There may arise cases when you have to take data from a stored raw file, in such cases, you will have to connect those files with a program such that it can read, write or modify the data.
并非在每种情况下都不应直接从用户那里获取数据。 在某些情况下,您必须从已存储的原始文件中获取数据,在这种情况下,您将必须将这些文件与程序连接,以便它可以读取,写入或修改数据。
In this section, we will see how to perform these tasks.
在本节中,我们将看到如何执行这些任务。
档案存取模式 (File Access Modes)
Since there is various purpose of using a file along with Python (i.e., read, write, append), it is important to specify each time for what operations Python should expect on the file. Thus, there are various access modes which we have to specify while opening a file in a program.
由于将文件与Python一起使用(即读取,写入,附加)有多种目的,因此每次指定Python对文件应执行的操作非常重要。 因此,在程序中打开文件时必须指定多种访问模式。
Python is capable of managing two type of files - Text(or normal characters) and Binary(Text only containing 1 and 0). Along with the access mode, it is also necessary to specify which type of file it is going to open.
Python能够管理两种类型的文件- 文本 (或普通字符)和二进制文件 (仅包含1和0的文本)。 除了访问模式外,还必须指定要打开的文件类型。
Python-打开文件 (Python - Opening a File)
In order to connect our program with the desired file, we need a stream that can fetch or write data in it. For this purpose, there are already some in-built classes/functions which we can utilize to solve the purpose. The first thing that we need to do is create a file object, i.e., Instance of File class which we can do using file()
or open()
function. In order to open a file -
为了将程序与所需的文件连接,我们需要一个可以在其中获取或写入数据的流。 为此,已经有一些内置的类/函数可以用来解决目的。 我们需要做的第一件事是创建一个文件对象,即File类的实例,我们可以使用file()
或open()
函数来完成。 为了打开文件-
>>> myFile = open([path of file], [access mode], [buffer size])
Here myFile will be the object of the file and open()
method will open the file specified in the [path of file]. Other two arguments, [access mode] will provide the access mode and [buffer size] will ask for how many chunks of data is to be retrieved from the file. Although, last two parameters [access mode] and [buffer size] are completely optional. By default, if there is no access mode specified then it is considered to be reading mode. [path of file] can be the complete path of the file or if it exists in the same file as a program then only providing the name is sufficient. More clearly-
此处myFile将是文件的对象, open()
方法将打开在[file path]中指定的文件。 其他两个参数,[访问模式]将提供访问模式,[缓冲区大小]将询问要从文件中检索多少数据块。 虽然,最后两个参数[访问模式]和[缓冲区大小]完全是可选的。 默认情况下,如果未指定访问模式,则将其视为读取模式。 [文件路径]可以是文件的完整路径,或者如果它与程序存在于同一文件中,则仅提供名称即可。 更清楚-

In above case, writing
在上述情况下,编写
>>> myFile = open("file.txt")
is sufficient. However, in situations like-
足够了。 但是,在类似情况下-
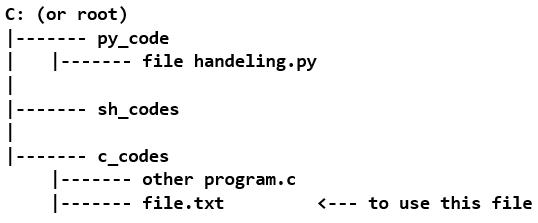
Note that this time your file.txt is not in the same folder as in above case, thus here you will have to specify the whole location.
请注意,这次,您的file.txt与上述情况不在同一文件夹中,因此,在此必须指定整个位置。
>>> myFile = open("C:/c_code/file.txt")
Once the file had been opened there is something called pointer that points to some position in the file. This pointer is used to read/write from that position. This pointer is pretty much like a text cursor which can be shifted freely (using arrow keys) or used to write or delete the text from a text, except that pointer can shift/read/write only through some functions.
打开文件后,将有一个称为指针的指针指向文件中的某个位置。 该指针用于从该位置读取/写入。 该指针非常类似于文本光标,可以自由移动(使用箭头键)或用于从文本中写入或删除文本,但该指针只能通过某些功能移动/读取/写入。
About access modes now, following are the available access modes that are to be specified in the argument-
现在,关于访问方式,以下是在参数中指定的可用访问方式:
read mode -
"r"
for text files and"rb"
for binary files. File pointer points at the beginning of the file.读取模式-
"r"
表示文本文件,"rb"
表示二进制文件。 文件指针指向文件的开头。>>> myFile = open("file.txt", "r")
write mode -
"w"
for text files and"wb"
for binary files. File pointer points at the beginning of the file.写模式-文本文件为
"w"
"wb"
,二进制文件为"wb"
。 文件指针指向文件的开头。>>> myFile = open("file.txt", "w")
append mode -
"a"
for text files and"ab"
for binary files. File pointer points at the end of the file.追加模式-
"a"
表示文本文件,"ab"
表示二进制文件。 文件指针指向文件的末尾。>>> myFile = open("file.txt", "a")
read/write mode -
"r+"
or"w+"
provide the option for doing both read and write operation on the same file object."rb+"
or"wb+"
for binary files. File pointer points at the beginning of the file.读/写模式-
"r+"
或"w+"
提供了对同一文件对象执行读和写操作的选项。 二进制文件为"rb+"
或"wb+"
。 文件指针指向文件的开头。>>> myFile = open("file.txt", "r+")
append/read -
"a+"
to enable read/append mode,"ab+"
for append/read mode on binary files. File pointer points at the end of the file.追加/读取-
"a+"
启用读取/追加模式,"ab+"
表示二进制文件的追加/读取模式。 文件指针指向文件的末尾。>>> myFile = open("file.txt", "a+")
As you can see opening files this way is pretty much similar to creating an object a file and doing some operations with/on it for read/write. Opening files in this way create a stream of the data buffer, after using the file it is always recommended to close this stream. This can be done using the close function. For instance, to close the myFile stream
如您所见,以这种方式打开文件非常类似于创建对象文件并对其进行一些操作以进行读/写。 以这种方式打开文件会创建一个数据缓冲区流,使用该文件后,始终建议关闭该流。 这可以使用关闭功能来完成。 例如,关闭myFile流
>>> myFile.close()
Another way of using a file is using with
keyword. Looking at syntax will make it clear how this works-
使用文件的另一种方法是with
关键字。 通过查看语法,可以清楚了解其工作原理-
with open("file.txt", "r+"):
// operations to perform on "file.txt"
// there can be many
// syntax is pretty much like that of methods
With this way, you are actually creating a scope for the file to be used. Once the program gets out of this scope the file gets closed itself.
通过这种方式,您实际上是在为要使用的文件创建作用域。 一旦程序脱离此范围,文件本身就会关闭。
More about reading and writing data into a file in next section.
下一节将详细介绍如何将数据读取和写入文件。
python中处理文件的包