java rmi
Remote method invocation(RMI) allow a java object to invoke method on an object running on another machine. RMI provide remote communication between java program. RMI is used for building distributed application.
远程方法调用(RMI)允许java对象在另一台计算机上运行的对象上调用方法。 RMI提供Java程序之间的远程通信。 RMI用于构建分布式应用程序。
RMI应用程序的概念 (Concept of RMI application)
A RMI application can be divided into two part,Client program and Server program. A Server program creates some remote object, make their references available for the client to invoke method on it. A Client program make request for remote objects on server and invoke method on them. Stub and Skeleton are two important object used for communication with remote object.
RMI应用程序可以分为两部分, 客户端程序和服务器程序。 Server程序创建一些远程对象,使它们的引用可供客户端在其上调用方法。 客户端程序在服务器上请求远程对象,并在它们上调用方法。 存根和骨架是用于与远程对象通信的两个重要对象。
存根 (Stub)
In RMI, a stub is an object that is used as a Gateway for the client-side. All the outgoing request are sent through it. When a client invokes the method on the stub object following things are performed internally:
在RMI中,存根是一个对象,用作客户端的网关。 所有外发请求都通过它发送。 当客户端在存根对象上调用该方法时,将在内部执行以下操作:
A connection is established using Remote Virtual Machine.
使用远程虚拟机建立连接。
It then transmits the parameters to the Remote Virtual Machine. This is also known as Marshals
然后,它将参数传输到远程虚拟机。 这也称为法警
After the 2nd step, it then waits for the output.
在第二步之后,然后等待输出。
Now it reads the value or exception which is come as an output.
现在,它读取作为输出来的值或异常。
At last, it returns the value to the client.
最后,它将值返回给客户端。
骨架 (Skeleton)
In RMI, a skeleton is an object that is used as a Gateway for the server-side.All the incoming request are sent through it. When a Server invokes the method on the skeleton object following things are performed internally:
在RMI中,框架是一个对象,用作服务器端的网关,所有传入的请求都通过它发送。 当服务器在骨架对象上调用该方法时,将在内部执行以下操作:
All the Parameters are read for the remote method.
读取所有参数以用于远程方法。
The method is invoked on the remote object.
该方法在远程对象上调用。
It then writes and transmits the parameters for the result. This is also known as Marshals.
然后,它为结果写入并传输参数。 这也称为法警。
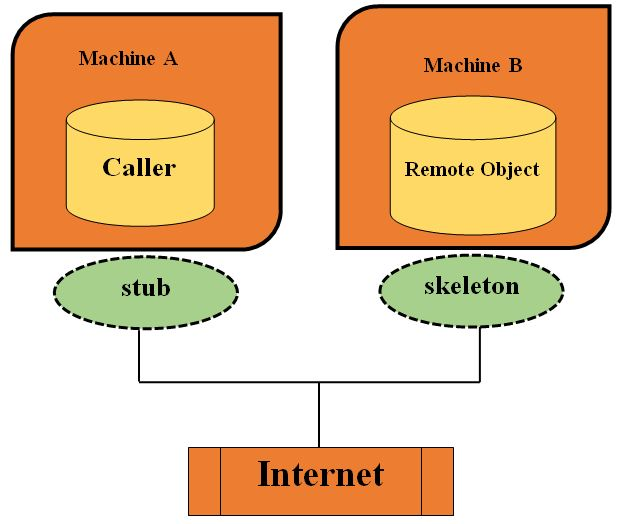
存根和骨架 (Stub and Skeleton)
Stub act as a gateway for Client program. It resides on Client side and communicate with Skeleton object. It establish the connection between remote object and transmit request to it.
存根充当客户端程序的网关。 它位于客户端,并与Skeleton对象通信。 它建立远程对象之间的连接并向其发送请求。
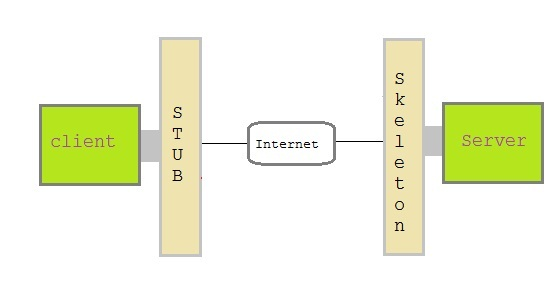
Skeleton object resides on server program. It is responsible for passing request from Stub to remote object.
骨架对象驻留在服务器程序上。 它负责将请求从存根传递到远程对象。
创建一个简单的RMI应用程序涉及以下步骤 (Creating a Simple RMI application involves following steps)
Define a remote interface.
定义一个远程接口。
Implementing remote interface.
实现远程接口。
create and start remote application
创建并启动远程应用程序
create and start client application
创建并启动客户端应用程序
定义一个远程接口 (Define a remote interface)
A remote interface specifies the methods that can be invoked remotely by a client. Clients program communicate to remote interfaces, not to classes implementing it. To be a remote interface, a interface must extend the Remote interface of java.rmi package.
远程接口指定客户端可以远程调用的方法。 客户端程序与远程接口通信,而不是与实现它的类通信。 要成为远程接口,接口必须扩展java.rmi包的Remote接口。
import java.rmi.*;
public interface AddServerInterface extends Remote
{
public int sum(int a,int b);
}
远程接口的实现 (Implementation of remote interface)
For implementation of remote interface, a class must either extend UnicastRemoteObject or use exportObject() method of UnicastRemoteObject class.
为了实现远程接口,类必须扩展UnicastRemoteObject或使用UnicastRemoteObject类的exportObject()方法。
import java.rmi.*;
import java.rmi.server.*;
public class Adder extends UnicastRemoteObject implements AddServerInterface
{
Adder()throws RemoteException{
super();
}
public int sum(int a,int b)
{
return a+b;
}
}
创建AddServer并托管rmi服务 (Create AddServer and host rmi service)
You need to create a server application and host rmi service Adder in it. This is done using rebind()
method of java.rmi.Naming class. rebind()
method take two arguments, first represent the name of the object reference and second argument is reference to instance of Adder
您需要创建一个服务器应用程序并在其中托管rmi服务Adder 。 这是使用java.rmi.Naming类的rebind()
方法完成的。 rebind()
方法rebind()
两个参数,第一个表示对象引用的名称,第二个参数是对Adder实例的引用
import java.rmi.*;
import java.rmi.registry.*;
public class AddServer {
public static void main(String args[]) {
try {
AddServerInterface addService=new Adder();
Naming.rebind("AddService",addService); //addService object is hosted with name AddService
}
catch(Exception e) {
System.out.println(e);
}
}
}
创建客户端应用程序 (Create client application)
Client application contains a java program that invokes the lookup()
method of the Naming class. This method accepts one argument, the rmi URL and returns a reference to an object of type AddServerInterface. All remote method invocation is done on this object.
客户端应用程序包含一个Java程序,该程序调用Naming类的lookup()
方法。 此方法接受一个参数rmi URL,并返回对类型AddServerInterface的对象的引用 。 所有远程方法调用都在此对象上完成。
import java.rmi.*;
public class Client {
public static void main(String args[]) {
try{
AddServerInterface st = (AddServerInterface)Naming.lookup("rmi://"+args[0]+"/AddService");
System.out.println(st.sum(25,8));
}
catch(Exception e) {
System.out.println(e);
}
}
}
运行此RMI应用程序的步骤 (Steps to run this RMI application)
Save all the above java file into a directory and name it as "rmi"
将上述所有Java文件保存到目录中,并将其命名为“ rmi”
compile all the java files
编译所有的java文件
javac *.java
Start RMI registry
启动RMI注册表
start rmiregistry
Run Server file
运行服务器文件
java AddServer
Run Client file in another command prompt abd pass local host port number at run time
在另一个命令提示符下运行客户端文件,在运行时Abd通过本地主机端口号
java Client 127.0.0.1
例: (Example:)
Program: Power.java
程序:Power.java
import java.rmi.*;
public interface Power extends Remote
{
public int power1()throwsRemoteException;
}
Program: PowerRemote.java
程序:PowerRemote.java
import java.rmi.*;
import java.rmi.server.*;
import java.util.Scanner;
public class PowerRemote extends UnicastRemoteObject implements Power
{
PowerRemote()throws RemoteException
{
super();
}
public int power1(int z)
{
int z;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the base number ::");
int x = sc.nextInt();
System.out.println("Enter the exponent number ::");
int y = sc.nextInt();
z=y^x;
System.out.println(z);
}
}
MyServer.java
MyServer.java
import java.rmi.*;
import java.rmi.registry.*;
public class MyServer
{
public static void main(String args[])
{
try
{
Power stub=new PowerRemote();
Naming.rebind("rmi://localhost:1995/shristee",stub);
}
catch(Exception e)
{
System.out.println(e);
}
}
}
MyClient.java
MyClient.java
import java.rmi.*;
public class MyClient
{
public static void main(String args[])
{
try
{
Power stub=(Power)Naming.lookup("rmi://localhost:1995/shristee");
System.out.println(stub.power1());
}
catch(Exception e){}
}
}
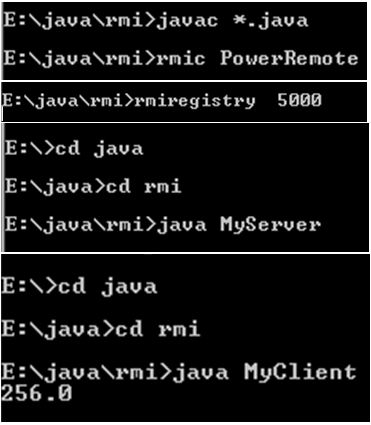
java rmi