java 静态成员 静态块
Static is a keyword in Java which is used to declare static stuffs. It can be used to create variable, method block or a class.
静态是Java中的一个关键字,用于声明静态内容。 它可以用来创建变量,方法块或类。
Static variable or method can be accessed without instance of a class because it belongs to class. Static members are common for all the instances of the class but non-static members are different for each instance of the class. Lets study how it works with variables and methods.
静态变量或方法可以在没有类实例的情况下进行访问,因为它属于类。 静态成员对于该类的所有实例都是通用的,但是非静态成员对于该类的每个实例都是不同的。 让我们研究它如何与变量和方法一起使用。
静态变量 (Static Variables)
Static variables defined as a class member can be accessed without object of that class. Static variable is initialized once and shared among different objects of the class. All the object of the class having static variable will have the same instance of static variable.
定义为类成员的静态变量可以在没有该类对象的情况下访问 。 静态变量被初始化一次,并在类的不同对象之间共享 。 具有静态变量的类的所有对象将具有相同的静态变量实例。
Note: Static variable is used to represent common property of a class. It saves memory.
注意:静态变量用于表示类的公共属性。 这样可以节省内存。
例: (Example:)
Suppose there are 100 employee in a company. All employee have its unique name and employee id but company name will be same all 100 employee. Here company name is the common property. So if you create a class to store employee detail, then declare company_name field as static
假设一家公司有100名员工。 所有员工都有其唯一的名称和员工ID,但公司名称将与所有100名员工相同。 在此,公司名称是共同财产。 因此,如果您创建一个用于存储员工详细信息的类,则将company_name字段声明为static
Below we have a simple class with one static variable, see the example.
下面我们有一个带有一个静态变量的简单类,请参见示例。
class Employee
{
int eid;
String name;
static String company = "Studytonight";
public void show()
{
System.out.println(eid + "-" + name + "-" + company);
}
public static void main( String[] args )
{
Employee se1 = new Employee();
se1.eid = 104;
se1.name = "Abhijit";
se1.show();
Employee se2 = new Employee();
se2.eid = 108;
se2.name = "ankit";
se2.show();
}
}
104-Abhijit-Studytonight 108-ankit-Studytonight
104-阿比吉特-研究之夜108-ankit-研究
We can understand it using the below image that shows different memory areas used by the program and how static variable is shared among the objects of the class.
我们可以使用下面的图像了解它,该图像显示了程序使用的不同存储区域,以及如何在类的对象之间共享静态变量。
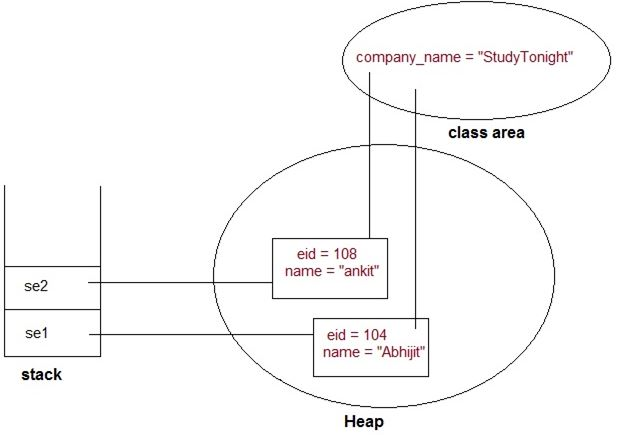
When we have a class variable like this, defined using the static keyword, then the variable is defined only once and is used by all the instances of the class. Hence if any of the class instance modifies it then it is changed for all the other instances of the class.
当我们有一个使用static关键字定义的类变量时,该变量仅定义一次,并由该类的所有实例使用。 因此,如果任何类实例对其进行了修改,则该类的所有其他实例都会对其进行更改。
静态变量与实例变量 (Static variable vs Instance variable)
Here are some differences between static variable and instance variables. Instance variables are non-static variables that store into heap and accessed through object only.
这是静态变量和实例变量之间的一些区别。 实例变量是存储在堆中并且只能通过对象访问的非静态变量。
Static variable | Instance Variable |
---|---|
Represent common property | Represent unique property |
Accessed using class name (can be accessed using object name as well) | Accessed using object |
Allocated memory only once | Allocated new memory each time a new object is created |
静态变量 | 实例变量 |
---|---|
代表共同财产 | 代表独特的财产 |
使用类名访问(也可以使用对象名访问) | 使用对象访问 |
仅分配一次内存 | 每次创建新对象时分配新内存 |
示例:静态变量与实例变量 (Example: static vs instance variables)
Let's take an example and understand the difference.
让我们举个例子,了解其中的区别。
public class Test
{
static int x = 100;
int y = 100;
public void increment()
{
x++; y++;
}
public static void main( String[] args )
{
Test t1 = new Test();
Test t2 = new Test();
t1.increment();
t2.increment();
System.out.println(t2.y);
System.out.println(Test.x); //accessed without any instance of class.
}
}
101 102
101102
See the difference in value of two variable. Static variable x shows the changes made to it by increment() method on the different object. While instance variable y show only the change made to it by increment() method on that particular instance.
看到两个变量的值之差。 静态变量x显示在不同对象上通过增量方法对它所做的更改。 而实例变量y仅显示该特定实例上的增量()方法对其所做的更改。
Java中的静态方法 (Static Method in Java)
A method can also be declared as static. Static methods do not need instance of its class for being accessed. main() method is the most common example of static method. main()
method is declared as static because it is called before any object of the class is created.
方法也可以声明为静态。 静态方法不需要访问其类的实例。 main()方法是静态方法的最常见示例。 main()
方法被声明为静态的,因为它是在创建该类的任何对象之前调用的。
Let's take an Example
让我们举个例子
class Test
{
public static void square(int x)
{
System.out.println(x*x);
}
public static void main (String[] arg)
{
square(8) //static method square () is called without any instance of class.
}
}
64
64
Java中的静态块 (Static block in Java)
Static block is used to initialize static data members. Static block executes even before main() method.
静态块用于初始化静态数据成员。 静态块甚至在main()方法之前执行。
It executes when the class is loaded in the memory. A class can have multiple Static blocks, which will execute in the same sequence in which they are programmed.
当将类加载到内存中时执行。 一个类可以有多个静态块,它们将按照编程时的相同顺序执行。
例 (Example)
Lets see an example in which we declared a static block to initialize the static variable.
让我们看一个示例,其中我们声明了一个静态块来初始化静态变量。
class ST_Employee
{
int eid;
String name;
static String company_name;
static {
company_name ="StudyTonight"; //static block invoked before main() method
}
public void show()
{
System.out.println(eid+" "+name+" "+company_name);
}
public static void main( String[] args )
{
ST_Employee se1 = new ST_Employee();
se1.eid = 104;
se1.name = "Abhijit";
se1.show();
}
}
104 Abhijit StudyTonight
104 Abhijit StudyTonight
不能从静态上下文引用非静态(实例)变量。 (Non-static (instance) variable cannot be referenced from a static context.)
When we try to access a non-static variable from a static context like main method, java compiler throws an error message a non-static variable cannot be referenced from a static context. This is because non-static variables are related with instance of class(object) and they get created when instance of a class is created by using new operator. So if we try to access a non-static variable without any instance, compiler will complain because those variables are not yet created and they don't have any existence until an instance is created and associated with it.
当我们尝试从像main方法之类的静态上下文中访问非静态变量时,java编译器会抛出错误消息, 指出无法从静态上下文中引用非静态变量 。 这是因为非静态变量与class(object)的实例相关,并且它们是在使用new运算符创建类的实例时创建的。 因此,如果我们尝试在没有任何实例的情况下访问非静态变量,则编译器会抱怨,因为这些变量尚未创建,并且在创建实例并将其关联之前它们不存在。
例 (Example)
Let's take an example of accessing non-static variable from a static context.
让我们以从静态上下文访问非静态变量为例。
class Test
{
int x;
public static void main(String[] args)
{
x = 10;
}
}
compiler error: non-static variable count cannot be referenced from a static context
编译器错误:无法从静态上下文引用非静态变量计数
为什么main()方法在Java中是静态的? (Why main() method is static in java?)
Because static methods can be called without any instance of a class and main()
is called before any instance of a class is created.
因为可以在没有类任何实例的情况下调用静态方法,并且在创建类的任何实例之前调用main()
。
java 静态成员 静态块