java嵌套类
A class defined within another class is known as Nested class. The scope of the nested class is bounded by the scope of its enclosing class.
在另一个类中定义的一个类称为嵌套类。 嵌套类的范围受其封闭类的范围限制。
Syntax:
句法:
class Outer{
//class Outer members
class Inner{
//class Inner members
}
} //closing of class Outer
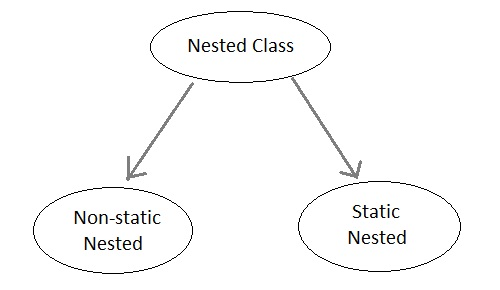
嵌套类的优点 (Advantages of Nested Class)
It is a way of logically grouping classes that are only used in one place.
这是一种对仅在一个地方使用的类进行逻辑分组的方法。
It increases encapsulation.
它增加了封装。
It can lead to more readable and maintainable code.
它可以导致更易读和可维护的代码。
If you want to create a class which is to be used only by the enclosing class, then it is not necessary to create a separate file for that. Instead, you can add it as "Inner Class"
如果要创建仅由封闭类使用的类,则无需为此创建单独的文件。 相反,您可以将其添加为“内部类”
静态嵌套类 (Static Nested Class)
If the nested class i.e the class defined within another class, has static modifier applied in it, then it is called as static nested class. Since it is, static nested classes can access only static members of its outer class i.e it cannot refer to non-static members of its enclosing class directly. Because of this restriction, static nested class is rarely used.
如果嵌套类(即在另一个类中定义的类)中应用了静态修饰符,则将其称为静态嵌套类 。 因此,静态嵌套类只能访问其外部类的静态成员,即它不能直接引用其封闭类的非静态成员。 由于此限制,很少使用静态嵌套类。
非静态嵌套类 (Non-static Nested class)
Non-static Nested class is the most important type of nested class. It is also known as Inner class. It has access to all variables and methods of Outer class including its private data members and methods and may refer to them directly. But the reverse is not true, that is, Outer class cannot directly access members of Inner class. Inner class can be declared private, public, protected, or with default access whereas an Outer class can have only public or default access.
非静态嵌套类是嵌套类最重要的类型。 也被称为内部类 。 它可以访问Outer类的所有变量和方法,包括其私有数据成员和方法,并且可以直接引用它们。 但是事实并非如此,也就是说,外部类不能直接访问内部类的成员。 内部类可以声明为私有,公共,受保护或具有默认访问权限,而外部类可以仅具有公共或默认访问权限。
One more important thing to notice about an Inner class is that it can be created only within the scope of Outer class. Java compiler generates an error if any code outside Outer class attempts to instantiate Inner class directly.
关于内部类,要注意的另一件事是,只能在外部类的范围内创建它。 如果Outer类之外的任何代码尝试直接实例化Inner类,则Java编译器将生成错误。
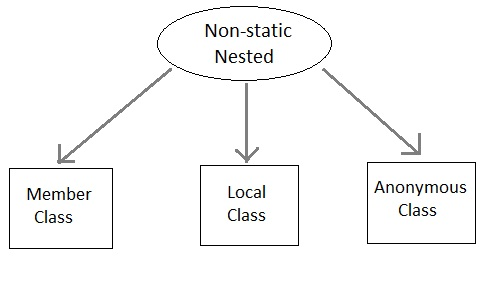
A non-static Nested class that is created outside a method is called Member inner class.
在方法外部创建的非静态嵌套类称为成员内部类 。
A non-static Nested class that is created inside a method is called local inner class. If you want to invoke the methods of local inner class, you must instantiate this class inside the method. We cannot use private, public or protected access modifiers with local inner class. Only abstract and final modifiers are allowed.
在方法内部创建的非静态嵌套类称为local internal class 。 如果要调用本地内部类的方法,则必须在方法内部实例化此类。 我们不能对本地内部类使用私有,公共或受保护的访问修饰符。 仅允许使用抽象和最终修饰符。
内部类(成员类)示例 (Example of Inner class(Member class))
class Outer
{
public void display()
{
Inner in=new Inner();
in.show();
}
class Inner
{
public void show()
{
System.out.println("Inside inner");
}
}
}
class Test
{
public static void main(String[] args)
{
Outer ot = new Outer();
ot.display();
}
}
Inside inner
内部内部
方法内部的内部类的示例(局部内部类) (Example of Inner class inside a method(local inner class))
class Outer
{
int count;
public void display()
{
for(int i=0;i<5;i++)
{
//Inner class defined inside for loop
class inner
{
public void show()
{
System.out.println("Inside inner "+(count++));
}
}
Inner in=new Inner();
in.show();
}
}
}
class Test
{
public static void main(String[] args)
{
Outer ot = new Outer();
ot.display();
}
}
Inside inner 0 Inside inner 1 Inside inner 2 Inside inner 3 Inside inner 4
内部内部0内部内部1内部内部2内部内部3内部内部4
在外部类外部实例化的内部类示例 (Example of Inner class instantiated outside Outer class)
class Outer
{
int count;
public void display()
{
Inner in = new Inner();
in.show();
}
class Inner
{
public void show()
{
System.out.println("Inside inner "+(++count));
}
}
}
class Test
{
public static void main(String[] args)
{
Outer ot = new Outer();
Outer.Inner in = ot.new Inner();
in.show();
}
}
Inside inner 1
内里1
匿名班 (Annonymous class)
A class without any name is called Annonymous class.
没有任何名称的类称为匿名类 。
interface Animal
{
void type();
}
public class ATest {
public static void main(String args[])
{
//Annonymous class created
Animal an = new Animal() {
public void type()
{
System.out.println("Annonymous animal");
}
};
an.type();
}
}
Annonymous animal
匿名动物
Here a class is created which implements Animal interace and its name will be decided by the compiler. This annonymous class will provide implementation of type() method.
在这里,将创建一个实现Animal interace的类,其名称将由编译器决定。 这个匿名类将提供type()方法的实现。
java嵌套类