java线程池创建线程
To implement multithreading, Java defines two ways by which a thread can be created.
为了实现多线程,Java定义了两种创建线程的方式。
By implementing the Runnable interface.
通过实现Runnable接口。
By extending the Thread class.
通过扩展Thread类。
实施可运行接口 (Implementing the Runnable Interface)
The easiest way to create a thread is to create a class that implements the runnable interface. After implementing runnable interface, the class needs to implement the run()
method.
创建线程的最简单方法是创建一个实现可运行接口的类。 在实现可运行接口之后,该类需要实现run()
方法。
运行方法语法: (Run Method Syntax:)
public void run()
It introduces a concurrent thread into your program. This thread will end when
run()
method terminates.它将并发线程引入程序。 当
run()
方法终止时,该线程将终止。You must specify the code that your thread will execute inside
run()
method.您必须在
run()
方法中指定线程将执行的代码。run()
method can call other methods, can use other classes and declare variables just like any other normal method.run()
方法可以调用其他方法,可以使用其他类并声明变量,就像其他任何普通方法一样。
class MyThread implements Runnable
{
public void run()
{
System.out.println("concurrent thread started running..");
}
}
class MyThreadDemo
{
public static void main(String args[])
{
MyThread mt = new MyThread();
Thread t = new Thread(mt);
t.start();
}
}
concurrent thread started running..
并发线程开始运行。
To call the run()
method, start()
method is used. On calling start(), a new stack is provided to the thread and run() method is called to introduce the new thread into the program.
要调用run()
方法,请使用start()
方法。 调用start()时,将向线程提供一个新的堆栈,并调用run()方法将新线程引入程序中。
Note: If you are implementing Runnable interface in your class, then you need to explicitly create a Thread class object and need to pass the Runnable interface implemented class object as a parameter in its constructor.
注意:如果要在类中实现Runnable接口,则需要显式创建Thread类对象,并且需要将Runnable接口实现的类对象作为参数传递给其构造函数。
扩展线程类 (Extending Thread class)
This is another way to create a thread by a new class that extends Thread class and create an instance of that class. The extending class must override run()
method which is the entry point of new thread.
这是通过扩展Thread类的新类创建线程并创建该类的实例的另一种方法。 扩展类必须重写run()
方法,该方法是新线程的入口点。
class MyThread extends Thread
{
public void run()
{
System.out.println("concurrent thread started running..");
}
}
classMyThreadDemo
{
public static void main(String args[])
{
MyThread mt = new MyThread();
mt.start();
}
}
concurrent thread started running..
并发线程开始运行。
In this case also, we must override the run()
and then use the start()
method to run the thread. Also, when you create MyThread class object, Thread class constructor will also be invoked, as it is the super class, hence MyThread class object acts as Thread class object.
同样在这种情况下,我们必须重写run()
,然后使用start()
方法运行线程。 同样,当您创建MyThread类对象时,由于它是超类,因此也将调用Thread类构造函数,因此MyThread类对象充当Thread类对象。
如果不使用start()
方法直接调用run()
start()
方法怎么办? (What if we call run()
method directly without using start()
method?)
In above program if we directly call run()
method, without using start()
method,
在上面的程序中,如果我们直接调用run()
方法而不使用start()
方法,
public static void main(String args[])
{
MyThread mt = new MyThread();
mt.run();
}
Doing so, the thread won't be allocated a new call stack, and it will start running in the current call stack, that is the call stack of the main thread. Hence Multithreading won't be there.
这样做不会为线程分配新的调用堆栈,它将开始在当前调用堆栈(即主线程的调用堆栈)中运行。 因此,多线程将不存在。
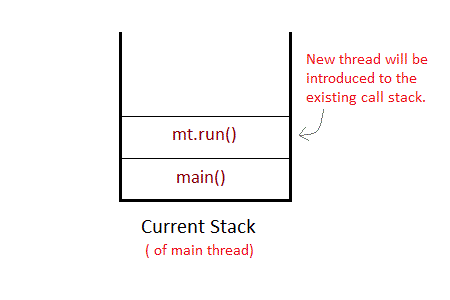
我们可以启动一个线程两次吗? (Can we Start a thread twice?)
No, a thread cannot be started twice. If you try to do so, IllegalThreadStateException will be thrown.
不,一个线程不能启动两次。 如果尝试这样做,将抛出IllegalThreadStateException 。
public static void main(String args[])
{
MyThread mt = new MyThread();
mt.start();
mt.start(); //Exception thrown
}
When a thread is in running state, and you try to start it again, or any method try to invoke that thread again using start() method, exception is thrown.
当线程处于运行状态时,您尝试再次启动它,或者任何方法尝试使用start()方法再次调用该线程,都会引发异常。
线程池 (Thread Pool)
In Java, is used for reusing the threads which were created previously for executing the current task. It also provides the solution if any problem occurs in the thread cycle or in resource thrashing. In Java Thread pool a group of threads are created, one thread is selected and assigned job and after completion of job, it is sent back in the group.
在Java中,用于重用先前创建的线程来执行当前任务。 如果在线程周期或资源颠簸中发生任何问题,它也提供了解决方案。 在Java线程池中,创建了一组线程,选择了一个线程并分配了作业,作业完成后,将其发送回该组中。
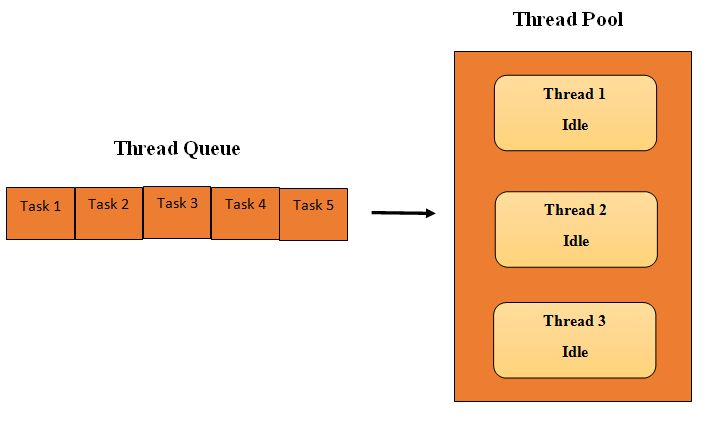
线程池有三种方法。 它们如下: (There are three methods of a Thread pool. They are as following:)
1. newFixedThreadPool(int)
1. newFixedThreadPool(int)
2. newCachedThreadPool()
2. newCachedThreadPool()
3. newSingleThreadExecutor()
3. newSingleThreadExecutor()
以下是创建线程池程序的步骤 (Following are the steps for creating a program of the thread pool)
1. create a runnable object to execute.
1.创建一个可运行的对象来执行。
2. using executors create an executor pool
2.使用执行程序创建执行程序池
3. Now Pass the object to the executor pool
3.现在将对象传递给执行者池
4. At last shutdown the executor pool.
4.最后关闭执行程序池。
例: (Example:)
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class WorkerThread implements Runnable
{
private String message;
public WorkerThread(String a)
{
this.message=a;
}
public void run()
{
System.out.println(Thread.currentThread().getName()+" (Start) message = "+message);
processmessage();
System.out.println(Thread.currentThread().getName()+" (End)");
}
private void processmessage()
{
try
{
Thread.sleep(5000);
}
catch (InterruptedException e)
{
System.out.println(e);
}
}
}
public class ThreadPoolDemo1
{
public static void main(String[] args)
{
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++)
{
Runnable obj = new WorkerThread("" + i);
executor.execute(obj);
}
executor.shutdown();
while (!executor.isTerminated())
{
}
System.out.println("********All threads are Finished********");
}
}
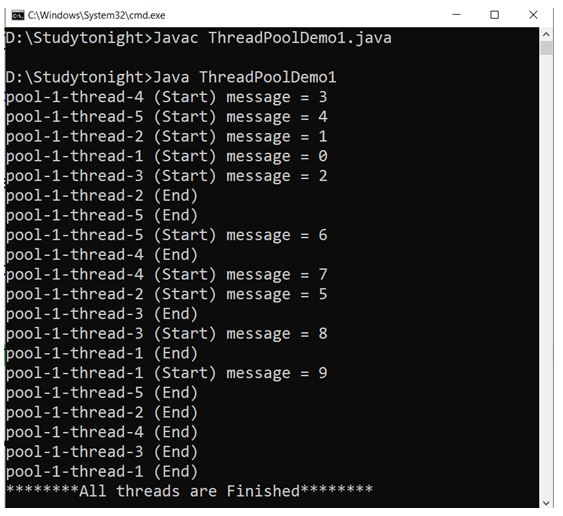
翻译自: https://www.studytonight.com/java/creating-a-thread.php
java线程池创建线程