java运算符
Operator is a symbol which tells to the compiler to perform some operation. Java provides a rich set of operators do deal with various types of operations. Sometimes we need to perform arithmetic operations then we use plus (+) operator for addition, multiply(*) for multiplication etc.
运算符是告诉编译器执行某些操作的符号。 Java提供了一组丰富的运算符,可以处理各种类型的运算。 有时我们需要执行算术运算,然后使用加号(+)进行加法运算,使用乘法(*)进行乘法运算等。
Operators are always essential part of any programming language.
运算符始终是任何编程语言的重要组成部分。
Java operators can be divided into following categories:
Java运算符可分为以下几类:
Arithmetic operators
算术运算符
Relation operators
关系运算符
Logical operators
逻辑运算符
Bitwise operators
按位运算符
Assignment operators
赋值运算符
Conditional operators
条件运算符
Misc operators
杂项运算符
算术运算符 (Arithmetic operators)
Arithmetic operators are used to perform arithmetic operations like: addition, subtraction etc and helpful to solve mathematical expressions. The below table contains Arithmetic operators.
算术运算符用于执行算术运算,例如:加法,减法等,有助于求解数学表达式。 下表包含算术运算符。
Operator | Description |
---|---|
+ | adds two operands |
- | subtract second operands from first |
* | multiply two operand |
/ | divide numerator by denumerator |
% | remainder of division |
++ | Increment operator increases integer value by one |
-- | Decrement operator decreases integer value by one |
操作员 | 描述 |
---|---|
+ | 加两个操作数 |
- | 从第一个减去第二个操作数 |
* | 两个操作数相乘 |
/ | 用分子除以分子 |
% | 除法余数 |
++ | 增量运算符将整数值增加一 |
-- | 减法运算符将整数值减一 |
Example:
例:
Lets create an example to understand arithmetic operators and their operations.
让我们创建一个示例来了解算术运算符及其操作。
class Arithmetic_operators1{
public static void main(String as[])
{
int a, b, c;
a=10;
b=2;
c=a+b;
System.out.println("Addtion: "+c);
c=a-b;
System.out.println("Substraction: "+c);
c=a*b;
System.out.println("Multiplication: "+c);
c=a/b;
System.out.println("Division: "+c);
b=3;
c=a%b;
System.out.println("Remainder: "+c);
a=++a;
System.out.println("Increment Operator: "+a);
a=--a;
System.out.println("decrement Operator: "+a);
}
}
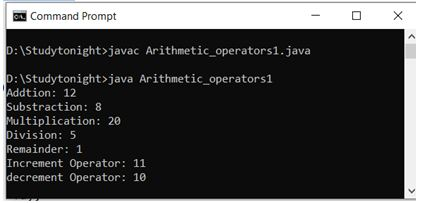
关系运算符 (Relation operators)
Relational operators are used to test comparison between operands or values. It can be use to test whether two values are equal or not equal or less than or greater than etc.
关系运算符用于测试操作数或值之间的比较。 可以用来测试两个值是否等于或小于等于或大于等。
The following table shows all relation operators supported by Java.
下表显示了Java支持的所有关系运算符。
Operator | Description |
---|---|
== | Check if two operand are equal |
!= | Check if two operand are not equal. |
> | Check if operand on the left is greater than operand on the right |
< | Check operand on the left is smaller than right operand |
>= | check left operand is greater than or equal to right operand |
<= | Check if operand on left is smaller than or equal to right operand |
操作员 | 描述 |
---|---|
== | 检查两个操作数是否相等 |
!= | 检查两个操作数是否不相等。 |
> | 检查左侧的操作数是否大于右侧的操作数 |
< | 左边的检查操作数小于右边的操作数 |
>= | 检查左操作数是否大于或等于右操作数 |
<= | 检查左侧的操作数是否小于或等于右侧的操作数 |
Example:
例:
In this example, we are using relational operators to test comparison like less than, greater than etc.
在此示例中,我们使用关系运算符来测试比较,例如小于,大于等。
class Relational_operators1{
public static void main(String as[])
{
int a, b;
a=40;
b=30;
System.out.println("a == b = " + (a == b) );
System.out.println("a != b = " + (a != b) );
System.out.println("a > b = " + (a > b) );
System.out.println("a < b = " + (a < b) );
System.out.println("b >= a = " + (b >= a) );
System.out.println("b <= a = " + (b <= a) );
}
}
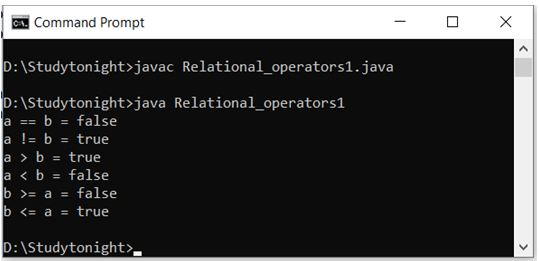
逻辑运算符 (Logical operators)
Logical Operators are used to check conditional expression. For example, we can use logical operators in if statement to evaluate conditional based expression. We can use them into loop as well to evaluate a condition.
逻辑运算符用于检查条件表达式。 例如,我们可以在if语句中使用逻辑运算符来评估基于条件的表达式。 我们也可以将它们用于循环以评估条件。
Java supports following 3 logical operator. Suppose we have two variables whose values are: a=true and b=false.
Java支持以下3个逻辑运算符。 假设我们有两个变量,其值为: a = true和b = false 。
Operator | Description | Example |
---|---|---|
&& | Logical AND | (a && b) is false |
|| | Logical OR | (a || b) is true |
! | Logical NOT | (!a) is false |
操作员 | 描述 | 例 |
---|---|---|
&& | 逻辑与 | (a && b)为假 |
|| | 逻辑或 | (a || b)是真的 |
! | 逻辑非 | (!a)是错误的 |
Example:
例:
In this example, we are using logical operators. There operators return either true or false value.
在此示例中,我们使用逻辑运算符。 那里的运算符返回true或false值。
class Logical_operators1{
public static void main(String as[])
{
boolean a = true;
boolean b = false;
System.out.println("a && b = " + (a&&b));
System.out.println("a || b = " + (a||b) );
System.out.println("!(a && b) = " + !(a && b));
}
}
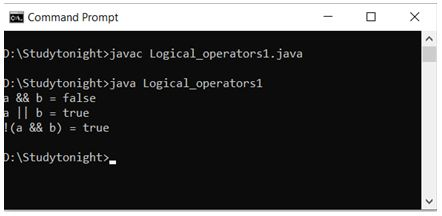
按位运算符 (Bitwise operators)
Bitwise operators are used to perform operations bit by bit.
按位运算符用于逐位执行操作。
Java defines several bitwise operators that can be applied to the integer types long, int, short, char and byte.
Java定义了几个按位运算符,可以将它们应用于整数long,int,short,char和byte。
The following table shows all bitwise operators supported by Java.
下表显示了Java支持的所有按位运算符。
Operator | Description |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise exclusive OR |
<< | left shift |
>> | right shift |
操作员 | 描述 |
---|---|
& | 按位与 |
| | 按位或 |
^ | 按位异或 |
<< | 左移 |
>> | 右移 |
Now lets see truth table for bitwise &
, |
and ^
现在让我们看一下真值表中的&
|
和^
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1个 | 0 | 1个 | 1个 |
1个 | 0 | 0 | 1个 | 1个 |
1个 | 1个 | 1个 | 1个 | 0 |
The bitwise shift operators shifts the bit value. The left operand specifies the value to be shifted and the right operand specifies the number of positions that the bits in the value are to be shifted. Both operands have the same precedence.
按位移位运算符对位值进行移位 。 左操作数指定要移位的值, 右操作 数指定值中要移位的位的数目 。 两个操作数具有相同的优先级。
Example: 例:Lets create an example that shows working of bitwise operators.
让我们创建一个示例,说明按位运算符的工作方式。
a = 0001000
b = 2
a << b = 0100000
a >> b = 0000010
class Bitwise_operators1{
public static void main(String as[])
{
int a = 50;
int b = 25;
int c = 0;
c = a & b;
System.out.println("a & b = " + c );
c = a | b;
System.out.println("a | b = " + c );
c = a ^ b;
System.out.println("a ^ b = " + c );
c = ~a;
System.out.println("~a = " + c );
c = a << 2;
System.out.println("a << 2 = " + c );
c = a >> 2;
System.out.println("a >>2 = " + c );
c = a >>> 2;
System.out.println("a >>> 2 = " + c );
}
}
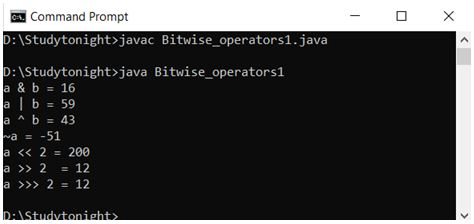
赋值运算符 (Assignment Operators)
Assignment operators are used to assign a value to a variable. It can also be used combine with arithmetic operators to perform arithmetic operations and then assign the result to the variable. Assignment operator supported by Java are as follows:
赋值运算符用于为变量赋值。 它也可以与算术运算符结合使用以执行算术运算,然后将结果分配给变量。 Java支持的赋值运算符如下:
Operator | Description | Example |
---|---|---|
= | assigns values from right side operands to left side operand | a = b |
+= | adds right operand to the left operand and assign the result to left | a+=b is same as a=a+b |
-= | subtracts right operand from the left operand and assign the result to left operand | a-=b is same as a=a-b |
*= | mutiply left operand with the right operand and assign the result to left operand | a*=b is same as a=a*b |
/= | divides left operand with the right operand and assign the result to left operand | a/=b is same as a=a/b |
%= | calculate modulus using two operands and assign the result to left operand | a%=b is same as a=a%b |
操作员 | 描述 | 例 |
---|---|---|
= | 将值从右侧操作数分配给左侧操作数 | a = b |
+= | 将右操作数添加到左操作数,并将结果分配给左 | a+=b 与a=a+b |
-= | 从左操作数中减去右操作数,并将结果分配给左操作数 | a-=b 与a =ab 相同 |
*= | 将多个左操作数与右操作数相加,并将结果分配给左操作数 | a*=b 与a=a*b |
/= | 将左操作数除以右操作数,并将结果分配给左操作数 | a/=b 与a=a/b |
%= | 使用两个操作数计算模数并将结果分配给左操作数 | a%=b 与a=a%b |
Example:
例:
Lets create an example to understand use of assignment operators. All assignment operators have right to left associativity.
让我们创建一个示例来了解赋值运算符的用法。 所有赋值运算符都具有从右到左的关联性。
class Assignment_operators1{
public static void main(String as[])
{
int a = 30;
int b = 10;
int c = 0;
c = a + b;
System.out.println("c = a + b = " + c );
c += a ;
System.out.println("c += a = " + c );
c -= a ;
System.out.println("c -= a = " + c );
c *= a ;
System.out.println("c *= a = " + c );
a = 20;
c = 25;
c /= a ;
System.out.println("c /= a = " + c );
a = 20;
c = 25;
c %= a ;
System.out.println("c %= a = " + c );
c <<= 2 ;
System.out.println("c <<= 2 = " + c );
c >>= 2 ;
System.out.println("c >>= 2 = " + c );
c >>= 2 ;
System.out.println("c >>= 2 = " + c );
c &= a ;
System.out.println("c &= a = " + c );
c ^= a ;
System.out.println("c ^= a = " + c );
c |= a ;
System.out.println("c |= a = " + c );
}
}
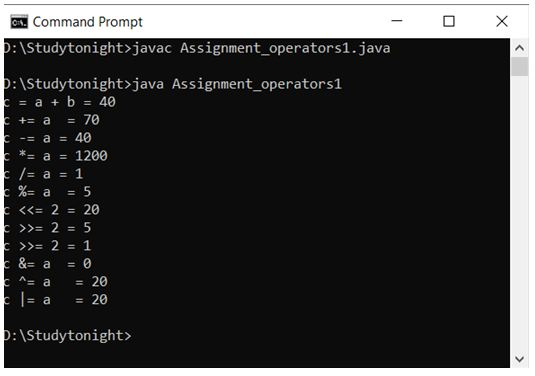
杂项 算子 (Misc. operator)
There are few other operator supported by java language.
java语言支持的其他运算符很少。
条件运算符 (Conditional operator)
It is also known as ternary operator because it works with three operands. It is short alternate of if-else statement. It can be used to evaluate Boolean expression and return either true or false value
它也被称为三元运算符,因为它可以处理三个操作数。 它是if-else语句的简短替代。 它可用于评估布尔表达式并返回true或false值
epr1 ? expr2 : expr3
Example:
例:
In ternary operator, if epr1 is true then expression evaluates after question mark (?) else evaluates after colon (:). See the below example.
在三元运算符中,如果epr1为true,则表达式在问号(?) 之后计算,否则在冒号(:)之后计算。 请参见以下示例。
class Conditional_operators1{
public static void main(String as[])
{
int a, b;
a = 20;
b = (a == 1) ? 30: 40;
System.out.println( "Value of b is : " + b );
b = (a == 20) ? 30: 40;
System.out.println( "Value of b is : " + b );
}
}
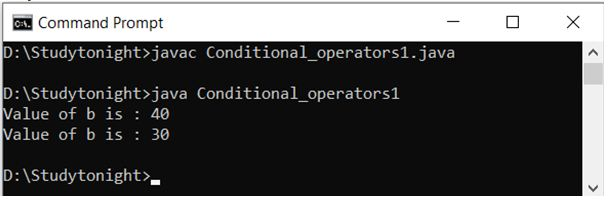
instanceOf
运算符 (instanceOf
operator)
It is a java keyword and used to test whether the given reference belongs to provided type or not. Type can be a class or interface. It returns either true or false.
它是一个Java 关键字 ,用于测试给定的引用是否属于提供的类型 。 类型可以是类或接口。 它返回true或false 。
Example:
例:
Here, we created a string reference variable that stores “studytonight”. Since it stores string value so we test it using isinstance operator to check whether it belongs to string class or not. See the below example.
在这里,我们创建了一个字符串参考变量,用于存储“ studytonight”。 由于它存储字符串值,因此我们使用isinstance运算符对其进行测试,以检查它是否属于字符串类。 请参见以下示例。
class instanceof_operators1{
public static void main(String as[])
{
String a = "Studytonight";
boolean b = a instanceof String;
System.out.println( b );
}
}
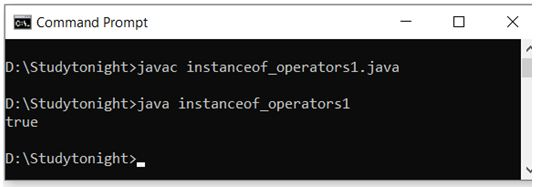
翻译自: https://www.studytonight.com/java/operators-in-java.php
java运算符