java中的垃圾收集器
Java garbage collection is the process of releasing unused memory occupied by unused objects. This process is done by the JVM automatically because it is essential for memory management.
Java垃圾回收是释放未使用对象占用的未使用内存的过程 。 该过程由JVM自动完成,因为它对于内存管理至关重要。
When a Java programs run on the JVM, objects are created on the heap, which is a portion of memory dedicated to the program. Eventually, some objects will no longer be needed.
当Java程序在JVM上运行时,将在堆上创建对象,这是专用于该程序的内存的一部分。 最终,将不再需要某些对象。
When there is no reference to an object, then that object is assumed to be no longer needed and the memory occupied by the object are released. This technique is called Garbage Collection.
如果没有引用某个对象,则假定不再需要该对象,并且释放该对象占用的内存。 此技术称为垃圾收集 。
垃圾收集如何运作? (How Garbage Collection Works?)
The garbage collection is a part of the JVM and is an automatic process done by JVM. We do not need to explicitly mark objects to be deleted. However, we can request to the JVM for garbage collection of an object but ultimately it depends on the JVM to call garbage collector.
垃圾收集是JVM的一部分,是JVM的自动过程。 我们不需要显式标记要删除的对象。 但是,我们可以请求JVM进行对象的垃圾回收,但是最终它取决于JVM来调用垃圾回收器。
Unlike C++ there is no explicit way to destroy object.
与C ++不同,没有明确的销毁对象的方法。
In the below image, you can understand that if an object does not have any reference than it will be dumped by the JVM.
在下图中,您可以理解,如果一个对象没有任何引用,那么它将被JVM转储。
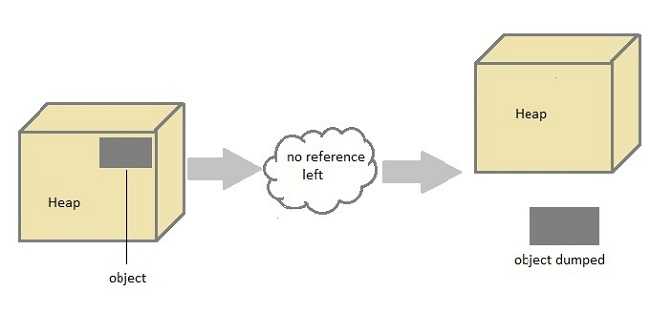
可以明确强制垃圾收集吗? (Can the Garbage Collection be forced explicitly ?)
No, the Garbage Collection can not be forced explicitly. We may request JVM for garbage collection by calling System.gc() method. But This does not guarantee that JVM will perform the garbage collection.
不可以,不能明确强制垃圾收集。 我们可以通过调用System.gc()方法来请求JVM进行垃圾回收 。 但这不能保证JVM将执行垃圾回收。
垃圾收集的优势 (Advantages of Garbage Collection)
Programmer doesn't need to worry about dereferencing an object.
程序员不必担心取消引用对象。
It is done automatically by JVM.
它是由JVM自动完成的。
Increases memory efficiency and decreases the chances for memory leak.
提高内存效率并减少内存泄漏的机会。
An object is able to get garbage collect if it is non-reference. We can make an object non-reference by using three ways.
如果对象是非引用对象,则可以进行垃圾回收。 我们可以通过三种方式使对象成为非引用对象。
1. set null to object reference which makes it able for garbage collection. For example:
1.将null设置为对象引用,使其能够进行垃圾回收。 例如:
Demo demo = new Demo();
demo = null; // ready for garbage collection
2. We can non-reference an object by setting new reference to it which makes it able for garbage collection. For example
2.我们可以通过设置对象的新引用来非引用该对象,从而使其能够进行垃圾回收。 例如
Demo demo = new Demo();
Demo demo2 = new Demo();
demo2 = demo // referring object
3. Anonymous object does not have any reference so if it is not in use, it is ready for the garbage collection.
3. 匿名对象没有任何引用,因此,如果不使用它,则可以进行垃圾回收。
finalize()
方法 (finalize()
method)
Sometime an object will need to perform some specific task before it is destroyed such as closing an open connection or releasing any resources held. To handle such situation finalize() method is used.
有时,某个对象在销毁之前需要执行某些特定任务,例如关闭打开的连接或释放所持有的任何资源。 为了处理这种情况,使用finalize()方法。
The finalize() method is called by garbage collection thread before collecting object. Its the last chance for any object to perform cleanup utility.
垃圾回收线程在收集object之前调用 finalize()方法。 它是任何对象执行清理实用程序的最后机会。
Signature of finalize() method
finalize()方法的签名
protected void finalize()
{
//finalize-code
}
需要记住的一些重要点 (Some Important Points to Remember)
It is defined in java.lang.Object class, therefore it is available to all the classes.
它在java.lang.Object类中定义,因此它可用于所有类。
It is declare as proctected inside Object class.
它在Object类中声明为受保护的。
It gets called only once by a Daemon thread named GC (Garbage Collector) thread.
名为GC(垃圾收集器)线程的守护程序线程仅调用一次。
要求垃圾收集 (Request for Garbage Collection)
We can request to JVM for garbage collection however, it is upto the JVM when to start the garbage collector.
我们可以请求JVM进行垃圾收集,但是,启动垃圾收集器的时间取决于JVM。
Java gc() method is used to call garbage collector explicitly. However gc() method does not guarantee that JVM will perform the garbage collection. It only request the JVM for garbage collection. This method is present in System and Runtime class.
Java gc()方法用于显式调用垃圾回收器。 但是,gc()方法不能保证JVM将执行垃圾回收。 它仅请求JVM进行垃圾收集。 System和Runtime类中提供了此方法。
gc()方法的示例 (Example of gc() Method)
Let's take an example and understand the functioning of the gc() method.
让我们举个例子,了解gc()方法的功能。
public class Test
{
public static void main(String[] args)
{
Test t = new Test();
t=null;
System.gc();
}
public void finalize()
{
System.out.println("Garbage Collected");
}
}
Garbage Collected
垃圾收集
翻译自: https://www.studytonight.com/java/garbage-collection.php
java中的垃圾收集器