java线程几种类型
Thread class is the main class on which Java's Multithreading system is based. Thread class, along with its companion interface Runnable will be used to create and run threads for utilizing Multithreading feature of Java.
线程类是Java多线程系统所基于的主要类。 线程类及其配套接口Runnable将用于创建和运行线程,以利用Java的多线程功能。
It provides constructors and methods to support multithreading. It extends object class and implements Runnable interface.
它提供了构造函数和方法来支持多线程。 它扩展了对象类并实现了Runnable接口。
线程类的签名 (Signature of Thread class)
public class Thread extends Object implements Runnable
线程类优先级常量 (Thread Class Priority Constants)
Field | Description |
---|---|
MAX_PRIORITY | It represents the maximum priority that a thread can have. |
MIN_PRIORITY | It represents the minimum priority that a thread can have. |
NORM_PRIORITY | It represents the default priority that a thread can have. |
领域 | 描述 |
---|---|
MAX_PRIORITY | 它表示线程可以具有的最大优先级。 |
MIN_PRIORITY | 它表示线程可以具有的最低优先级。 |
NORM_PRIORITY | 它表示线程可以具有的默认优先级。 |
线程类的构造函数 (Constructors of Thread class)
Thread()
线程 ()
Thread(String str)
线程 (字符串str)
Thread(Runnable r)
线程 (可运行r)
Thread(Runnable r, String str)
线程 (Runnable r,字符串str)
Thread(ThreadGroup group, Runnable target)
线程 ( ThreadGroup组, Runnable目标)
Thread(ThreadGroup group, Runnable target, String name)
线程 ( ThreadGroup组, Runnable目标,字符串名称)
Thread(ThreadGroup group, Runnable target, String name, long stackSize)
线程 ( ThreadGroup组, Runnable目标,字符串名称,长stackSize)
Thread(ThreadGroup group, String name)
线程 ( ThreadGroup组, 字符串名称)
线程类方法 (Thread Class Methods)
Thread class also defines many methods for managing threads. Some of them are,
线程类还定义了许多用于管理线程的方法。 他们之中有一些是,
Method | Description |
---|---|
setName() | to give thread a name |
getName() | return thread's name |
getPriority() | return thread's priority |
isAlive() | checks if thread is still running or not |
join() | Wait for a thread to end |
run() | Entry point for a thread |
sleep() | suspend thread for a specified time |
start() | start a thread by calling run() method |
activeCount() | Returns an estimate of the number of active threads in the current thread's thread group and its subgroups. |
checkAccess() | Determines if the currently running thread has permission to modify this thread. |
currentThread() | Returns a reference to the currently executing thread object. |
dumpStack() | Prints a stack trace of the current thread to the standard error stream. |
getId() | Returns the identifier of this Thread. |
getState() | Returns the state of this thread. |
getThreadGroup() | Returns the thread group to which this thread belongs. |
interrupt() | Interrupts this thread. |
interrupted() | Tests whether the current thread has been interrupted. |
isAlive() | Tests if this thread is alive. |
isDaemon() | Tests if this thread is a daemon thread. |
isInterrupted() | Tests whether this thread has been interrupted. |
setDaemon(boolean on) | Marks this thread as either a daemon thread or a user thread. |
setPriority(int newPriority) | Changes the priority of this thread. |
yield() | A hint to the scheduler that the current thread is willing to yield its current use of a processor. |
方法 | 描述 |
---|---|
setName() | 给线程起个名字 |
getName() | 返回线程的名称 |
getPriority() | 返回线程的优先级 |
活着() | 检查线程是否仍在运行 |
加入() | 等待线程结束 |
跑() | 线程的入口点 |
睡觉() | 将线程挂起指定的时间 |
开始() | 通过调用run()方法启动线程 |
activeCount() | 返回当前线程的线程组及其子组中活动线程的数量的估计值。 |
checkAccess() | 确定当前正在运行的线程是否有权修改此线程。 |
currentThread() | 返回对当前正在执行的线程对象的引用。 |
dumpStack() | 将当前线程的堆栈跟踪记录打印到标准错误流。 |
getId() | 返回此线程的标识符。 |
getState() | 返回此线程的状态。 |
getThreadGroup() | 返回该线程所属的线程组。 |
打断() | 中断此线程。 |
打断() | 测试当前线程是否已被中断。 |
活着() | 测试此线程是否仍然存在。 |
isDaemon() | 测试此线程是否是守护程序线程。 |
isInterrupted() | 测试此线程是否已被中断。 |
setDaemon(boolean on) | 将此线程标记为守护线程或用户线程。 |
setPriority(int newPriority) | 更改此线程的优先级。 |
让() | 给调度程序的提示是当前线程愿意放弃对处理器的当前使用。 |
注意事项 (Some Important points to Remember)
When we extend Thread class, we cannot override setName() and getName() functions, because they are declared final in Thread class.
扩展Thread类时,不能覆盖setName()和getName()函数,因为它们在Thread类中声明为final。
While using sleep(), always handle the exception it throws.
使用sleep()时 ,始终处理它引发的异常。
static void sleep(long milliseconds) throws InterruptedException
可运行的界面 (Runnable Interface)
It also used to create thread and should be used if you are only planning to override the run()
method and no other Thread methods.
它也用于创建线程,如果您仅打算覆盖run()
方法而没有其他Thread方法,则应使用它。
签名 (Signature)
@FunctionalInterface
public interface Runnable
可运行接口方法 (Runnable Interface Method)
It provides only single method that must be implemented by the class.
它仅提供必须由类实现的单个方法。
Method | Description |
---|---|
run() | It runs the implemented thread. |
方法 | 描述 |
---|---|
跑() | 它运行已实现的线程。 |
关机钩 ( Shutdown hook )
In Java, Shutdown hook is used to clean-up all the resource, it means closing all the files, sending alerts etc. We can also save the state when the JVM shuts down. Shutdown hook mostly used when any code is to be executed before any JVM shuts down. Following are some of the reasons when the JVM shut down:
在Java中,Shutdown hook用于清理所有资源,这意味着关闭所有文件,发送警报等。我们还可以在JVM关闭时保存状态。 关机钩子通常用于在任何JVM关闭之前要执行任何代码的情况。 以下是JVM关闭的一些原因:
Pressing ctrl+c on the command prompt
在命令提示符下按ctrl + c
When the System.exit(int) method is invoked.
调用System.exit(int)方法时。
When user logoff or shutdown etc
当用户注销或关闭等时
addShutdownHook(线程钩) (addShutdownHook(Thread hook))
The addShutdownHook(Thread hook) method is used to register the thread with the virtual machine. This method is of Runtime class.
addShutdownHook(Thread hook)方法用于在虚拟机中注册线程。 此方法属于Runtime类。
Example:
例:
class Demo6 extends Thread
{
public void run()
{
System.out.println("Shutdown hook task is Now completed...");
}
}
public class ShutdownDemo1
{
public static void main(String[] args)throws Exception
{
Runtime obj=Runtime.getRuntime();
obj.addShutdownHook(new Demo6());
System.out.println("Now main method is sleeping... For Exit press ctrl+c");
try
{
Thread.sleep(4000);
}
catch (Exception e) {}
}
}
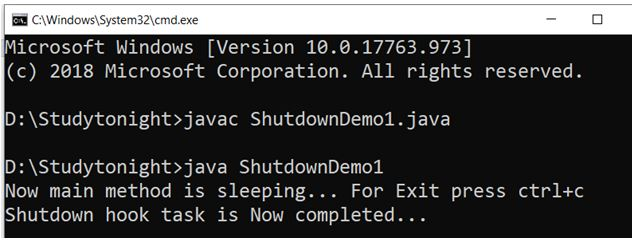
内存不足异常 (OutOfMemory Exception)
In Java, as we know that all objects are stored in the heap. The objects are created using the new keyword. The OutOfMemoryError occurs as follow:
在Java中,我们知道所有对象都存储在堆中。 使用new关键字创建对象。 OutOfMemoryError发生如下:

This error occurs when Java Virtual Machine is not able to allocate the object because it is out of memory and no memory can be available by the garbage collector.
当Java虚拟机无法分配对象,因为该对象内存不足并且垃圾收集器无法使用任何内存时,会发生此错误。
The meaning of OutOfMemoryError is that something wrong is in the program. Many times the problem can be out of control when the third party library caches strings.
OutOfMemoryError的含义是程序中有问题。 很多时候,第三方库缓存字符串时,问题可能会失控。
可能发生OutOfMemoryError的基本程序 (Basic program in which OutOfMemoryError can occur)
Example:
例:
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class OutOfMemoryDemo1 {
public static void main(String[] args) {
List
obj = new ArrayList<>();
Random obj1= new Random();
while (true)
obj.add(obj1.nextInt());
}
}
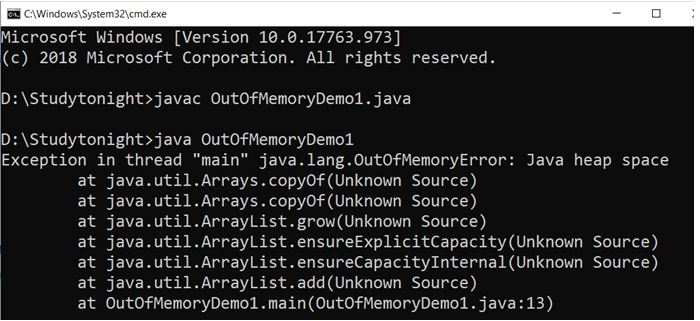
由于内存不足而可能发生OutOfMemoryError的程序 (Program in which OutOfMemoryError can occur because of low memory)
Example:
例:
public class OutOfMemoryErrorDemo2
{
public static void main(String[] args)
{
Integer[] a = new Integer[100000*10000*1000];
System.out.println("Done");
}
}
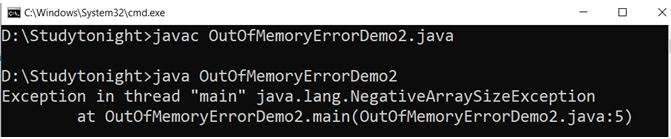
垃圾收集器超过限制时可能发生OutOfMemoryError的程序 (Program in which OutOfMemoryError can occur, when Garbage Collector exceed the limit)
Example:
例:
import java.util.*;
public class OutOfMemoryErrorDemo2{
public static void main(String args[]) throws Exception
{
Map a = new HashMap();
a = System.getProperties();
Random b = new Random();
while (true) {
a.put(b.nextInt(), "randomValue");
} }
}
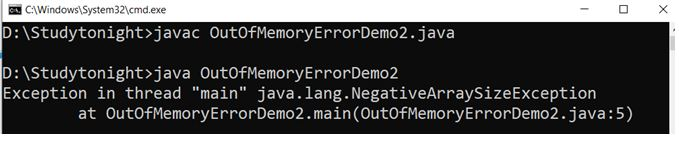
翻译自: https://www.studytonight.com/java/thread-class-and-functions.php
java线程几种类型