c语言中动态分配内存
Below is a basic memory architecture used by any C++ program:
以下是任何C ++程序使用的基本内存体系结构:
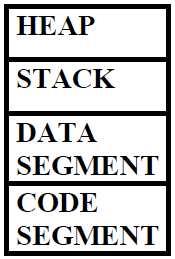
Code Segment: Compiled program with executive instructions are kept in code segment. It is read only. In order to avoid over writing of stack and heap, code segment is kept below stack and heap.
代码段 :带有执行指令的编译程序保存在代码段中。 它是只读的。 为了避免覆盖堆栈和堆,将代码段保留在堆栈和堆之下。
Data Segment: Global variables and static variables are kept in data segment. It is not read only.
数据段 :全局变量和静态变量保留在数据段中。 它不是只读的。
Stack: A stack is usually pre-allocated memory. The stack is a LIFO data structure. Each new variable is pushed onto the stack. Once variable goes out of scope, memory is freed. Once a stack variable is freed, that region of memory becomes available for other variables. The stack grows and shrinks as functions push and pop local variables. It stores local data, return addresses, arguments passed to functions and current status of memory.
堆栈 :堆栈通常是预先分配的内存。 堆栈是LIFO数据结构。 每个新变量都被压入堆栈。 一旦变量超出范围,就会释放内存。 释放堆栈变量后,该内存区域可用于其他变量。 随着函数推入和弹出局部变量,堆栈会增长和收缩。 它存储本地数据,返回地址,传递给函数的参数以及内存的当前状态。
Heap: Memory is allocated during program execution. Memory is allocated using new operator and deallocating memory using delete operator.
堆 :在程序执行期间分配内存。 使用new运算符分配内存,并使用delete运算符取消分配内存。
使用new
关键字分配堆内存 (Allocation of Heap Memory using new
Keyword)
Here we will learn how to allocate heap memory to a variable or class object using the new
keyword.
在这里,我们将学习如何使用new
关键字将堆内存分配给变量或类对象。
Syntax:
句法:
datatype pointername = new datatype
For example:
例如:
int *new_op = new int;
// allocating block of memory
int *new_op = new int[10];
If enough memory is not available in the heap it is indicated by throwing an exception of type std::bad_alloc
and a pointer is returned.
如果堆中没有足够的内存,则抛出std::bad_alloc
类型的异常来表示该异常,并返回一个指针。
使用delete
关键字重新分配内存 (Deallocation of memory using delete
Keyword)
Once heap memory is allocated to a variable or class object using the new
keyword, we can deallocate that memory space using the delete
keyword.
使用new
关键字将堆内存分配给变量或类对象后,我们可以使用delete
关键字释放该内存空间。
Syntax:
句法:
delete pointer variable
For example:
例如:
delete new_op;
The object's extent or the object's lifetime is the time for which the object remains in the memory during the program execution. Heap Memory allocation is slower than a stack. In heap there is no particular order in which you can allocate memory as in stack.
对象的范围或对象的生存期是在程序执行期间对象保留在内存中的时间。 堆内存分配比堆栈慢。 在堆中,没有像堆栈中那样分配内存的特定顺序。
了解C ++中的内存泄漏 (Understanding Memory Leak in C++)
Memory leak happens due to the mismanagement of memory allocations and deallocations. It mostly happens in case of dynamic memory allocation. There is no automatic garbage collection in C++ as in Java, so programmer is responsible for deallocating the memory used by pointers.
内存泄漏是由于内存分配和释放分配的管理不当造成的。 它主要发生在动态内存分配的情况下。 在C ++中没有像Java中那样自动进行垃圾回收 ,因此程序员负责释放指针使用的内存。
Misuse of an elevator in a building in real life is an example of memory leak. Suppose you stay in an apartment building which has 19 floors. You wanted to go to 10th floor so you pressed the button to call the lift. The status of elevator is displaying as basement for 20 minutes. Then you realize that something is wrong, and upon investigating you find out that kids were playing in basement and they had blocked the lift door.
现实生活中建筑物中电梯的滥用是内存泄漏的一个例子。 假设您住在19层的公寓楼中。 你还要去10 楼 ,所以你按下按钮呼叫电梯。 电梯状态显示为地下室20分钟。 然后,您意识到出了点问题,在进行调查时,您发现孩子们正在地下室玩耍,他们挡住了电梯门。
Similarly once a pointer is done with its operations it should free the memory used by it. So that other variables can use the memory and memory can be managed effectively.
类似地,一旦指针完成其操作,它将释放它所使用的内存。 这样其他变量就可以使用内存,并且可以有效地管理内存。
By using the delete
keyword we can delete the memory allocated:
通过使用delete
关键字,我们可以删除分配的内存:
For example:
例如:
*ex= new Example();
delete ex;
But in the above example dangling pointer issue can happen. Wait! what is a dangling pointer?
但是在上面的示例中, 悬挂指针问题可能发生。 等待! 什么是悬空指针?
什么是悬空指针? (What is a Dangling Pointer?)
A pointer pointing to a memory location of already deleted object is known as a dangling pointer.
指向已删除对象的存储位置的指针称为悬空指针。
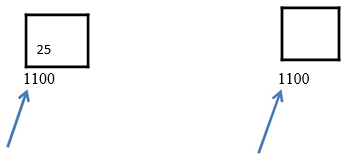
In the first figure pointer points to a memory location 1100 which contains a value 25.
在第一图中,指针指向包含值25的存储位置1100。
In the second figure pointer points to a memory location where object is deleted.
在第二个图中,指针指向删除对象的存储位置。
Dangling pointers arise due to object destruction, when an object reference is deleted or deallocated, without modifying the value of the pointer, so the pointer will keep on pointing to the same memory location. This problem can be avoided by initializing the pointer to NULL
.
当对象引用被删除或释放时,由于对象破坏而产生了悬空指针,而没有修改指针的值,因此指针将继续指向相同的内存位置。 通过初始化指向NULL
的指针可以避免此问题。
For example:
例如:
*ex = new Example();
Delete ex;
// assigning the pointer to NULL
ex = NULL;
什么是智能指针? (What is a Smart Pointer?)
Smart Pointer is used to manage the lifetimes of dynamically allocated objects. They ensure proper destruction of dynamically allocated objects. Smart pointers are defined in the memory header file.
智能指针用于管理动态分配对象的生存期。 它们确保正确销毁动态分配的对象。 智能指针在内存头文件中定义。
Smart pointers are built-in pointers, we don't have to worry about deleting them, they are automatically deleted.
智能指针是内置指针,我们不必担心删除它们,它们会被自动删除。
Here is an example of a smart pointer:
这是一个智能指针的示例:
S_ptr *ptr = new S_ptr();
ptr->action();
delete ptr;
翻译自: https://www.studytonight.com/cpp/memory-management-in-cpp.php
c语言中动态分配内存