java中链式调用
Chained Exception was added to Java in JDK 1.4. This feature allows you to relate one exception with another exception, i.e one exception describes cause of another exception. For example, consider a situation in which a method throws an ArithmeticException because of an attempt to divide by zero but the actual cause of exception was an I/O error which caused the divisor to be zero. The method will throw only ArithmeticException to the caller. So the caller would not come to know about the actual cause of exception. Chained Exception is used in such type of situations.
JDK 1.4已将链式异常添加到Java。 此功能使您可以将一个异常与另一个异常相关联,即一个异常描述了另一个异常的原因。 例如,考虑一种情况,其中一种方法由于试图除以零而抛出ArithmeticException ,但实际的异常原因是导致除数为零的I / O错误。 该方法仅将ArithmeticException抛出给调用方。 因此,调用者不会知道异常的实际原因。 链式异常用于此类情况。
Two new constructors and two new methods were added to Throwable class to support chained exception.
向Throwable类添加了两个新的构造函数和两个新方法,以支持链式异常。
Throwable(Throwable cause)
可投掷 (可投掷原因)
Throwable(String str, Throwable cause)
Throwable (字符串str,Throwable原因)
In the first constructor, the paramter cause specifies the actual cause of exception. In the second form, it allows us to add an exception description in string form with the actual cause of exception.
在第一个构造函数中,参数原因指定异常的实际原因。 在第二种形式中,它允许我们以字符串形式添加具有异常实际原因的异常描述。
getCause() and initCause() are the two methods added to Throwable class.
getCause()和initCause()是添加到Throwable类的两个方法。
getCause() method returns the actual cause associated with current exception.
getCause()方法返回与当前异常关联的实际原因。
initCause() set an underlying cause(exception) with invoking exception.
initCause()设置具有异常的根本原因(异常)。
时间为例! (Time for an Example!)
Lets understand the chain exception with the help of an example, here, ArithmeticException was thrown by the program but the real cause of exception was IOException. We set the cause of exception using initCause() method.
让我们借助示例来了解链式异常,这里,程序抛出了ArithmeticException,但是异常的真正原因是IOException。 我们使用initCause()方法设置异常原因。
import java.io.IOException;
public class ChainedException
{
public static void divide(int a, int b)
{
if(b == 0)
{
ArithmeticException ae = new ArithmeticException("top layer");
ae.initCause(new IOException("cause"));
throw ae;
}
else
{
System.out.println(a/b);
}
}
public static void main(String[] args)
{
try
{
divide(5, 0);
}
catch(ArithmeticException ae) {
System.out.println( "caught : " +ae);
System.out.println("actual cause: "+ae.getCause());
}
}
}
caught:java.lang.ArithmeticException: top layer actual cause: java.io.IOException: cause
捕获:java.lang.ArithmeticException:顶层实际原因:java.io.IOException:原因
例 (Example)
lets see one more example to understand chain exception, here NumberFormatException was thrown but the actual cause of exception was a null pointer exception.
让我们再看一个例子来理解链式异常,这里抛出了NumberFormatException,但是异常的真正原因是空指针异常。
public class ChainedDemo1
{
public static void main(String[] args)
{
try
{
NumberFormatException a = new NumberFormatException("====> Exception");
a.initCause(new NullPointerException("====> Actual cause of the exception"));
throw a;
}
catch(NumberFormatException a)
{
System.out.println(a);
System.out.println(a.getCause());
}
}
}
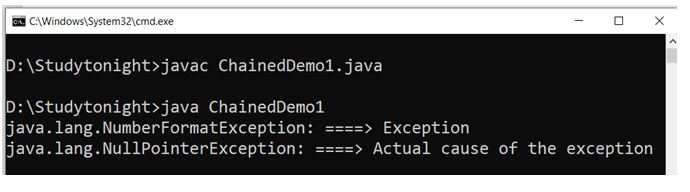
异常传播 (Exception propagation)
In Java, an exception is thrown from the top of the stack, if the exception is not caught it is put in the bottom of the stack, this process continues until it reaches to the bottom of the stack and caught. It is known as exception propagation. By default, an unchecked exception is forwarded in the called chain.
在Java中,从堆栈的顶部抛出一个异常,如果未捕获到该异常,则将其放入堆栈的底部,此过程将一直持续到到达堆栈的底部并被捕获为止。 这称为异常传播。 默认情况下,未检查的异常在被调用链中转发。
例 (Example)
In this example, an exception occurred in method a1 which is called by method a2 and a2 is called by method a3. Method a3() is enclosed in try block to provide the safe guard. We know exception will be thrown be method a1 but handled in method a3(). This is called exception propagation.
在此示例中,方法a1中发生了一个异常,该异常被方法a2调用,而a2被方法a3调用。 方法a3()包含在try块中以提供安全防护。 我们知道方法a1将引发异常,但方法a3()将处理异常。 这称为异常传播。
class ExpDemo1{
void a1()
{
int data = 30 / 0;
}
void a2()
{
a1();
}
void a3()
{
try {
a2();
}
catch (Exception e)
{
System.out.println(e);
}
}
public static void main(String args[])
{
ExpDemo1 obj1 = new ExpDemo1();
obj1.a3();
}
}
java.lang.ArithmeticException: / by zero
java.lang.ArithmeticException:/减零
例 (Example )
Lets take another example, here program throw an IOException from method m1() which is called inside the n1(). Exception thrown by method m1() is handled by method n1().
让我们再举一个例子,这里程序从方法m1()抛出IOException,该方法在n1()内部被调用。 方法m1()引发的异常由方法n1()处理。
import java.io.IOException;
class Demo{
void m1() throws IOException
{
throw new IOException("device error");
}
void n1() throws IOException
{
m1();
}
void p1()
{
try {
n1();
}
catch (Exception e)
{
System.out.println("Exception handled");
}
}
public static void main(String args[])
{
Demo obj = new Demo();
obj.p1();
}
}
翻译自: https://www.studytonight.com/java/chained-exception-in-java.php
java中链式调用