创建第一个maven项目
In the earlier lessons, we have seen about the maven project architecture and the different configurations involved for a project. Now, we will understand how a sample Java project can be created using Maven. Maven can also be used to build and manage projects developed using C#, Ruby, Scala and few other languages. But, this is primarily used for java projects, so we will be using Java for examples. Also, we will see how the project is structured when created using maven.
在前面的课程中,我们了解了Maven项目体系结构以及项目涉及的不同配置。 现在,我们将了解如何使用Maven创建示例Java项目 。 Maven还可以用于构建和管理使用C#,Ruby,Scala和其他几种语言开发的项目。 但是,这主要用于Java项目,因此我们将以Java为例。 此外,我们还将看到使用maven创建项目时的结构。
Maven原型 (Maven Archetype)
Maven uses archetype to create any project. Archetype is nothing but the tool provided by the maven to create a project. It is also defined as 'an organizational pattern from which all other things of the similar kind are made'.
Maven使用原型创建任何项目。 原型不过是行家提供的用于创建项目的工具。 它也被定义为“一种组织模式,所有类似类型的其他事物都来自该组织模式” 。
Using archetypes provides an easy and instrumental way to create projects to the developers, where the best practices are employed as per the standards defined at the organization level. Also, archetypes enables the developers to quickly create a project and start running the same within a matter of commands.
使用原型为开发人员提供了一种简便且实用的方式来创建项目,该开发人员根据组织级别定义的标准采用了最佳实践。 同样,原型使开发人员能够快速创建项目并在命令范围内开始运行该项目。
In order to create a new simple maven java project just use the maven archetype plugin in the command line. Currently there are more than 850+ maven archetypes listed out in order to create projects under different frameworks like struts, spring, hibernate, web services (RESTful/SOAP) and many others.
为了创建一个新的简单的Maven Java项目,只需在命令行中使用Maven原型插件。 当前,列出了850多种Maven原型,以便在不同的框架(例如struts,spring,hibernate,Web服务(RESTful / SOAP)等)下创建项目。
So, let us create one simple project in maven. Open the command prompt and navigate to the workspace folder in your local machine (E.g. D:\Java\workspace). You can create the Workspace folder wherever you want on your PC or use your Workspace, if already created. Then type the below maven command(its a single line command) and press enter.
因此,让我们在maven中创建一个简单的项目。 打开命令提示符,然后导航到本地计算机上的工作空间文件夹(例如D:\ Java \ workspace) 。 您可以在PC上的任何位置创建Workspace文件夹,也可以使用Workspace(如果已创建)。 然后键入以下maven命令(单行命令)并按Enter。
mvn archetype:generate -DgroupId=com.java.samples -DartifactId=JavaSamples
-DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This maven command will create a Java project with the below details:
此maven命令将创建一个具有以下详细信息的Java项目:
groupId will be com.java.samples, representing the package.
groupId将是com.java.samples,代表程序包。
artifactId will be JavaSamples (on the build, JavaSamples.jar will be created)
artifactId将为JavaSamples(在构建中,将创建JavaSamples.jar)
archetypeArtifactId is nothing but the template used for creating this Java project.
archetypeArtifactId只是用于创建此Java项目的模板。
interactiveMode is used when the developer is aware of the actual spelling of the artifact id. In the above case maven-archetype-quickstart is the one which is used and it is the proper one. If developer is not aware of this then the interactiveMode is set to be
TRUE
so that it will scan the remote repositories for all available archetypes. This might take longer time.当开发人员知道工件ID的实际拼写时,将使用InteractiveMode 。 在上述情况下,使用的是maven-archetype-quickstart,它是正确的。 如果开发人员不知道这一点,则将InteractiveMode设置为
TRUE
以便它将在远程存储库中扫描所有可用的原型。 这可能需要更长的时间。
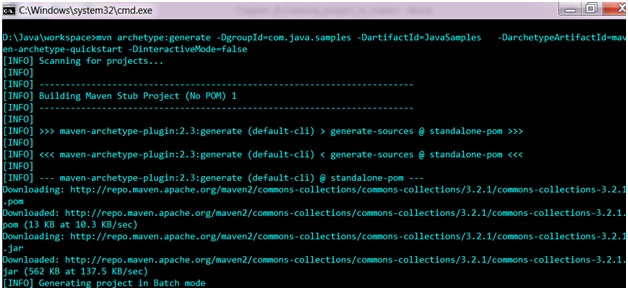
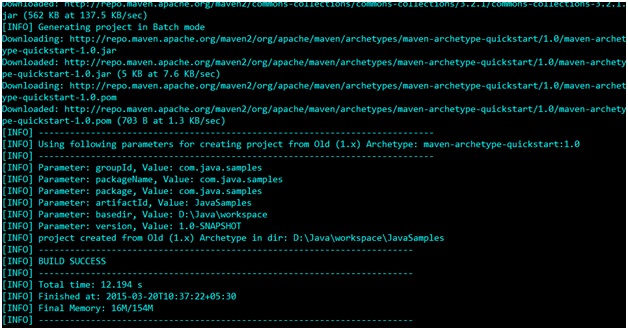
The above command instructs the maven to create a project with maven-archetype-quickstart template.
上面的命令指示maven使用maven-archetype-quickstart模板创建一个项目。
If you navigate to the folder D:\Java\workspace, a new project named JavaSamples will be created including all the base structure of a java project as well as shown below :
如果导航到文件夹D:\ Java \ workspace ,将创建一个名为JavaSamples的新项目,其中包括Java项目的所有基本结构,如下所示:
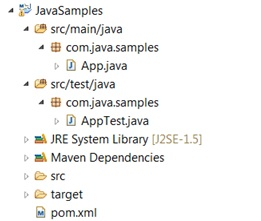
To see the following directory structure you need to Create a New Java Project on your Eclipse IDE, and import the following project there.
要查看以下目录结构,您需要在Eclipse IDE上创建一个New Java Project,然后在其中导入以下项目。
Table below will describe the main directories that are created as a part of the project.
下表将描述作为项目一部分创建的主要目录。
Plugin name | Description |
---|---|
JavaSamples | Root folder of the project containing source and pom.xml |
src\main\java | Contains all the java code under the package com.java.samples |
src\main\test | Contains all the java test code under the package com.java.samples |
src\main\resources | Contains all the resources like xml\properties file |
插件名称 | 描述 |
---|---|
Java样本 | 包含source和pom.xml的项目的根文件夹 |
src \ main \ java | 包含com.java.samples包下的所有Java代码 |
src \ main \ test | 包含com.java.samples包下的所有Java测试代码。 |
src \ main \ resources | 包含所有资源,例如xml \ properties文件 |
Under the folder D:\Java\workspace\JavaSamples\src\main\java\com\java\samples a sample java file called App.java will be created by default which will be having one print statement.
在文件夹D:\ Java \ workspace \ JavaSamples \ src \ main \ java \ com \ java \ samples下,默认情况下将创建一个名为App.java的示例Java文件,该文件将具有一个打印语句。
package com.java.samples;
/**
* Hello world!
*
*/
public class App
{
public static void main (String[] args)
{
System.out.println("Hello World!");
}
}
And under the folder D:\Java\workspace\JavaSamples\src\test\java\com\java\samples a sample java test class (JUnit) will be created AppTest.java
在文件夹D:\ Java \ workspace \ JavaSamples \ src \ test \ java \ com \ java \ samples下,将创建一个AppTest.java示例Java测试类(JUnit)。
package com.java.samples;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
/**
* Unit test for simple App.
*/
public class AppTest extends TestCase{
/**
* Create the test case
*
* @param testName name of the test case
*/
public AppTest(String testName)
{
super(testName);
}
/**
* @return the suite of tests being tested
*/
public static Test suite()
{
return new TestSuite(AppTest.class);
}
/**
* Rigourous Test :-)
*/
public void testApp()
{
assertTrue(true);
}
}
The test class code which is marked as Rigorous Test is the code which is automatically generated when a sample java project is created using maven. This method will just return TRUE and the test will be passed after the run. This method is just included as one sample test. You can modify this method or add your own test methods.
标记为“ 严格测试 ”的测试类代码是使用maven创建示例Java项目时自动生成的代码。 该方法将仅返回TRUE,并且运行后将通过测试。 此方法仅作为一个样本测试。 您可以修改此方法或添加自己的测试方法。
Also, the TestCase class is an abstract class from the Junit API which is used here in the sample project. This will also be included by default automatically when a sample maven java project is created (check the dependency entry for junit in the pom.xml). The TestCase class defines the fixtures to run mutilple tests in a class.
另外, TestCase类是Junit API的抽象类,在示例项目中在此使用。 创建示例maven java项目时,默认情况下也会自动包括该文件(请检查pom.xml中junit的依赖项)。 TestCase类定义了在类中运行多功能测试的灯具。
Developers are required to add their files as described in the above mentioned table. They are very much free to add any new packages as well as required during the project development. The above said folder structure is just created as per the archetype plugin defined.
开发人员需要按照上述表格中的说明添加文件。 他们非常自由地添加任何新软件包,以及在项目开发过程中所需的软件包。 上面所说的文件夹结构是根据定义的原型插件创建的。
Maven中的版本 (Versions in Maven)
There are 2 major versions made available for any maven project:
有2个主要版本可用于任何Maven项目:
SNAPSHOT: This is nothing but the development version. New version's availability will be verified during every checkout and downloaded if it is required. This version is not allowed for releases.
快照 :这只是开发版本。 新版本的可用性将在每次签出时进行验证,并在需要时进行下载。 此版本不允许发布。
FIXED: Downloaded only once to the local repository. This is obtained whenever a major version of the software release happens.
固定 :仅下载到本地存储库一次。 只要发生软件发行版的主要版本,就可以获取该文件。
翻译自: https://www.studytonight.com/maven/project-creation-in-maven
创建第一个maven项目