java处理字符串
String is an object that represents sequence of characters. In Java, String is represented by String class which is located into java.lang
package
字符串是一个代表字符序列的对象 。 在Java中,String由位于java.lang
包中的String类表示。
It is probably the most commonly used class in java library. In java, every string that we create is actually an object of type String. One important thing to notice about string object is that string objects are immutable that means once a string object is created it cannot be changed.
它可能是Java库中最常用的类。 在Java中,我们创建的每个字符串实际上都是String类型的对象。 关于字符串对象要注意的一件事是字符串对象是不可变的 ,这意味着一旦创建了字符串对象,便无法更改它。
The Java String class implements Serializable, Comparable and CharSequence interface that we have represented using the below image.
Java String类实现了我们使用下图表示的Serializable,Comparable和CharSequence接口。
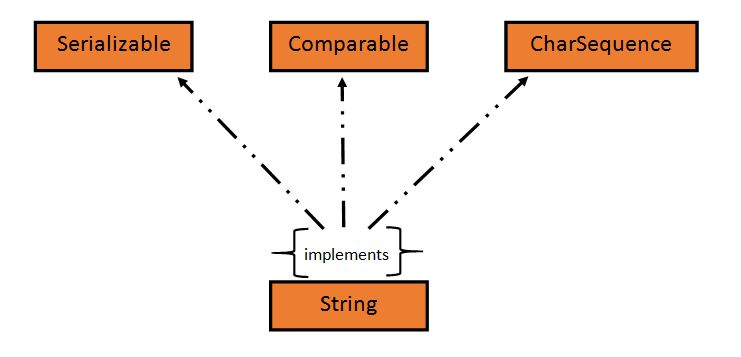
In Java, CharSequence Interface is used for representing a sequence of characters. CharSequence interface is implemented by String, StringBuffer and StringBuilder classes. This three classes can be used for creating strings in java.
在Java中, CharSequence接口用于表示字符序列。 CharSequence接口由String,StringBuffer和StringBuilder类实现。 这三个类可用于在Java中创建字符串。
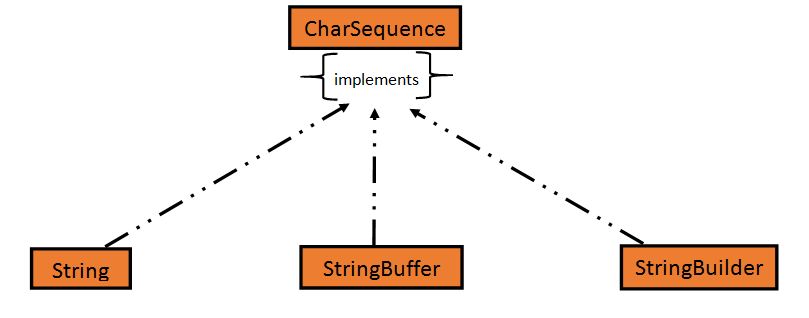
什么是不可变对象? (What is an Immutable object?)
An object whose state cannot be changed after it is created is known as an Immutable object. String, Integer, Byte, Short, Float, Double and all other wrapper classes objects are immutable.
创建后状态无法更改的对象称为不可变对象。 String,Integer,Byte,Short,Float,Double和所有其他包装器类对象都是不可变的。
创建一个字符串对象 (Creating a String object)
String can be created in number of ways, here are a few ways of creating string object.
可以通过多种方式创建字符串,以下是创建字符串对象的几种方法。
1)使用字符串文字 (1) Using a String literal)
String literal is a simple string enclosed in double quotes " "
. A string literal is treated as a String object.
字符串文字是用双引号" "
括住的简单字符串。 字符串文字被视为String对象。
public class Demo{
public static void main(String[] args) {
String s1 = "Hello Java";
System.out.println(s1);
}
}
Hello Java
你好Java
2)使用新的关键字 (2) Using new Keyword)
We can create a new string object by using new operator that allocates memory for the object.
我们可以使用为该对象分配内存的new运算符创建一个新的字符串对象。
public class Demo{
public static void main(String[] args) {
String s1 = new String("Hello Java");
System.out.println(s1);
}
}
Hello Java
你好Java
Each time we create a String literal, the JVM checks the string pool first. If the string literal already exists in the pool, a reference to the pool instance is returned. If string does not exist in the pool, a new string object is created, and is placed in the pool. String objects are stored in a special memory area known as string constant pool inside the heap memory.
每次我们创建String文字时,JVM都会首先检查字符串池。 如果字符串文字在池中已经存在,则返回对池实例的引用。 如果池中不存在字符串,则会创建一个新的字符串对象,并将其放置在池中。 字符串对象存储在堆内存中一个称为字符串常量池的特殊存储区域中。
字符串对象及其存储方式 (String object and How they are stored)
When we create a new string object using string literal, that string literal is added to the string pool, if it is not present there already.
当我们使用字符串文字创建一个新的字符串对象时,该字符串文字将被添加到字符串池中(如果尚未存在的话)。
String str= "Hello";
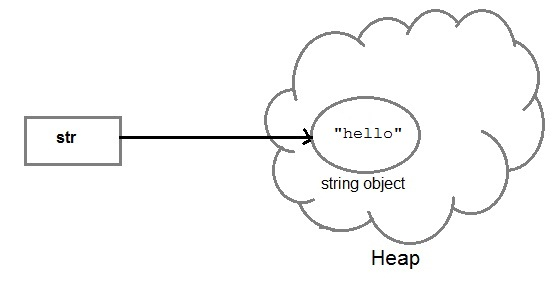
And, when we create another object with same string, then a reference of the string literal already present in string pool is returned.
并且,当我们使用相同的字符串创建另一个对象时,将返回字符串池中已经存在的字符串文字的引用。
String str2 = str;
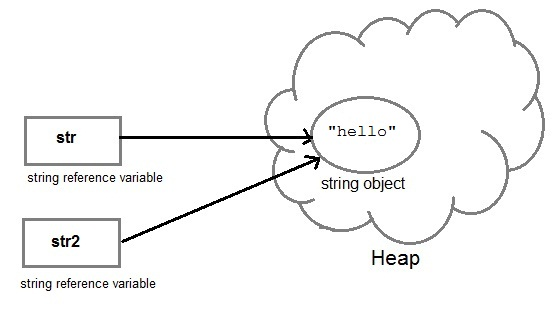
But if we change the new string, its reference gets modified.
但是,如果我们更改新字符串,则其引用将被修改。
str2=str2.concat("world");
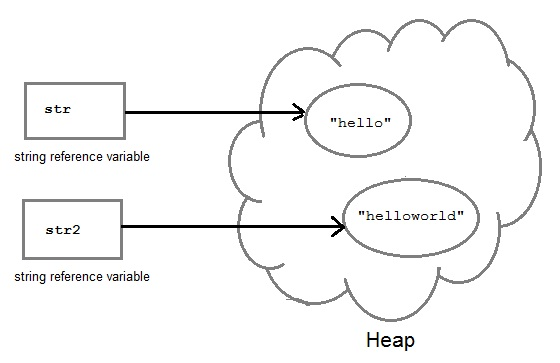
连接字符串 (Concatenating String)
There are 2 methods to concatenate two or more string.
有两种方法可以连接两个或多个字符串。
Using concat() method
使用concat()方法
Using
+
operator使用
+
运算符
1)使用concat()方法 (1) Using concat() method)
Concat()
method is used to add two or more string into a single string object. It is string class method and returns a string object.
Concat()
方法用于将两个或多个字符串添加到单个字符串对象中。 它是字符串类方法,并返回一个字符串对象。
public class Demo{
public static void main(String[] args) {
String s = "Hello";
String str = "Java";
String str1 = s.concat(str);
System.out.println(str1);
}
}
HelloJava
你好Java
2)使用+运算符 (2) Using + operator )
Java uses "+"
operator to concatenate two string objects into single one. It can also concatenate numeric value with string object. See the below example.
Java使用"+"
运算符将两个字符串对象连接为一个。 它还可以将数字值与字符串对象连接在一起。 请参见以下示例。
public class Demo{
public static void main(String[] args) {
String s = "Hello";
String str = "Java";
String str1 = s+str;
String str2 = "Java"+11;
System.out.println(str1);
System.out.println(str2);
}
}
HelloJava Java11
你好Java Java11
字符串比较 (String Comparison)
To compare string objects, Java provides methods and operators both. So we can compare string in following three ways.
为了比较字符串对象,Java提供了方法和运算符。 因此,我们可以通过以下三种方式比较字符串。
Using
equals()
method使用
equals()
方法Using
==
operator使用
==
运算符By
CompareTo()
method通过
CompareTo()
方法
使用equals()方法 (Using equals() method)
equals()
method compares two strings for equality. Its general syntax is,
equals()
方法比较两个字符串是否相等。 它的一般语法是
boolean equals (Object str)
例 (Example)
It compares the content of the strings. It will return true if string matches, else returns false.
它比较字符串的内容。 如果字符串匹配,它将返回true ,否则返回false 。
public class Demo{
public static void main(String[] args) {
String s = "Hell";
String s1 = "Hello";
String s2 = "Hello";
boolean b = s1.equals(s2); //true
System.out.println(b);
b = s.equals(s1) ; //false
System.out.println(b);
}
}
true false
真假
使用==运算符 (Using == operator)
The double equal (==)
operator compares two object references to check whether they refer to same instance. This also, will return true on successful match else returns false.
双重equal (==)
运算符比较两个对象引用以检查它们是否引用同一实例。 同样,如果匹配成功,则返回true ,否则返回false。
public class Demo{
public static void main(String[] args) {
String s1 = "Java";
String s2 = "Java";
String s3 = new String ("Java");
boolean b = (s1 == s2); //true
System.out.println(b);
b = (s1 == s3); //false
System.out.println(b);
}
}
true false
真假
说明 (Explanation)
We are creating a new object using new operator, and thus it gets created in a non-pool memory area of the heap. s1 is pointing to the String in string pool while s3 is pointing to the String in heap and hence, when we compare s1 and s3, the answer is false.
我们正在使用new运算符创建一个新对象,因此将在堆的非池内存区域中创建该对象。 s1指向字符串池中的字符串,而s3指向堆中的字符串,因此,当我们比较s1和s3时,答案为假。
The following image will explain it more clearly.
下图将更清楚地说明它。
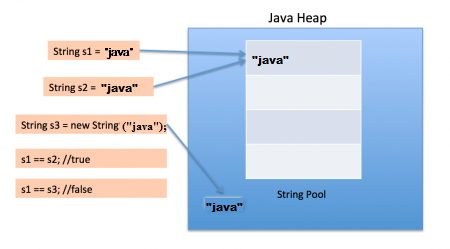
通过compareTo()
方法 (By compareTo()
method)
String compareTo()
method compares values and returns an integer value which tells if the string compared is less than, equal to or greater than the other string. It compares the String based on natural ordering i.e alphabetically. Its general syntax is.
字符串compareTo()
方法比较值并返回一个整数值,该值compareTo()
比较的字符串是否小于,等于或大于另一个字符串。 它根据自然顺序(即按字母顺序)比较字符串。 它的一般语法是。
句法: (Syntax:)
int compareTo(String str)
例: (Example:)
public class HelloWorld{
public static void main(String[] args) {
String s1 = "Abhi";
String s2 = "Viraaj";
String s3 = "Abhi";
int a = s1.compareTo(s2); //return -21 because s1 < s2
System.out.println(a);
a = s1.compareTo(s3); //return 0 because s1 == s3
System.out.println(a);
a = s2.compareTo(s1); //return 21 because s2 > s1
System.out.println(a);
}
}
-21 0 21
-21 0 21
翻译自: https://www.studytonight.com/java/string-handling-in-java.php
java处理字符串