插入排序算法?
Consider you have 10 cards out of a deck of cards in your hand. And they are sorted, or arranged in the ascending order of their numbers.
假设您手中有10张纸牌。 然后按数字的升序对它们进行排序或排列。
If I give you another card, and ask you to insert the card in just the right position, so that the cards in your hand are still sorted. What will you do?
如果我再给您一张卡片,并要求您将卡片插入正确的位置,以便您手上的卡片仍然可以分类。 你会怎么做?
Well, you will have to go through each card from the starting or the back and find the right position for the new card, comparing it's value with each card. Once you find the right position, you will insert the card there.
好吧,您将不得不从头到尾浏览每张卡,找到新卡的正确位置,然后将其与每张卡的价值进行比较。 找到正确的位置后,您将在其中插入卡。
Similarly, if more new cards are provided to you, you can easily repeat the same process and insert the new cards and keep the cards sorted too.
同样,如果提供了更多新卡,则可以轻松地重复相同的过程并插入新卡,并使卡也保持排序。
This is exactly how insertion sort works. It starts from the index 1
(not 0
), and each index starting from index 1
is like a new card, that you have to place at the right position in the sorted subarray on the left.
这正是插入排序的工作方式。 它从索引1
开始(不是0
),并且每个从索引1
开始的索引都像一张新卡片,您必须将其放置在左侧排序子数组的右侧位置。
Following are some of the important characteristics of Insertion Sort:
以下是插入排序的一些重要特征 :
It is efficient for smaller data sets, but very inefficient for larger lists.
对于较小的数据集,它是有效的,但对于较大的列表,它的效率非常低。
Insertion Sort is adaptive, that means it reduces its total number of steps if a partially sorted array is provided as input, making it efficient.
插入排序是自适应的,这意味着如果提供部分排序的数组作为输入,它将减少步骤的总数,从而使其高效。
It is better than Selection Sort and Bubble Sort algorithms.
它比选择排序和冒泡排序算法更好。
Its space complexity is less. Like bubble Sort, insertion sort also requires a single additional memory space.
它的空间复杂度较小。 像冒泡排序一样,插入排序也需要单个额外的存储空间。
It is a stable sorting technique, as it does not change the relative order of elements which are equal.
这是一种稳定的排序技术,因为它不会更改相等元素的相对顺序。
插入排序如何工作? (How Insertion Sort Works?)
Following are the steps involved in insertion sort:
以下是插入排序涉及的步骤:
We start by making the second element of the given array, i.e. element at index
1
, thekey
. Thekey
element here is the new card that we need to add to our existing sorted set of cards(remember the example with cards above).我们首先制作给定数组的第二个元素,即索引
1
处的元素key
。 这里的key
元素是我们需要添加到现有已排序卡片组中的新卡片(请记住上面的卡片示例)。We compare the
key
element with the element(s) before it, in this case, element at index0
:我们将
key
元素与其之前的元素进行比较,在本例中为索引0
元素:key
element is less than the first element, we insert thekey
元素小于第一个元素,则将key
element before the first element.key
元素插入第一个元素之前。key
element is greater than the first element, then we insert it after the first element.key
元素大于第一个元素,则将其插入第一个元素之后。
Then, we make the third element of the array as
key
and will compare it with elements to it's left and insert it at the right position.然后,我们将数组的第三个元素作为
key
,并将其与左边的元素进行比较,并将其插入到正确的位置。And we go on repeating this, until the array is sorted.
我们继续重复此操作,直到对数组进行排序为止。
Let's consider an array with values {5, 1, 6, 2, 4, 3}
让我们考虑一个值为{5, 1, 6, 2, 4, 3}
5,1,6,2,4,4,3 {5, 1, 6, 2, 4, 3}
的数组
Below, we have a pictorial representation of how bubble sort will sort the given array.
下面,我们用图形表示了气泡排序如何对给定数组进行排序。
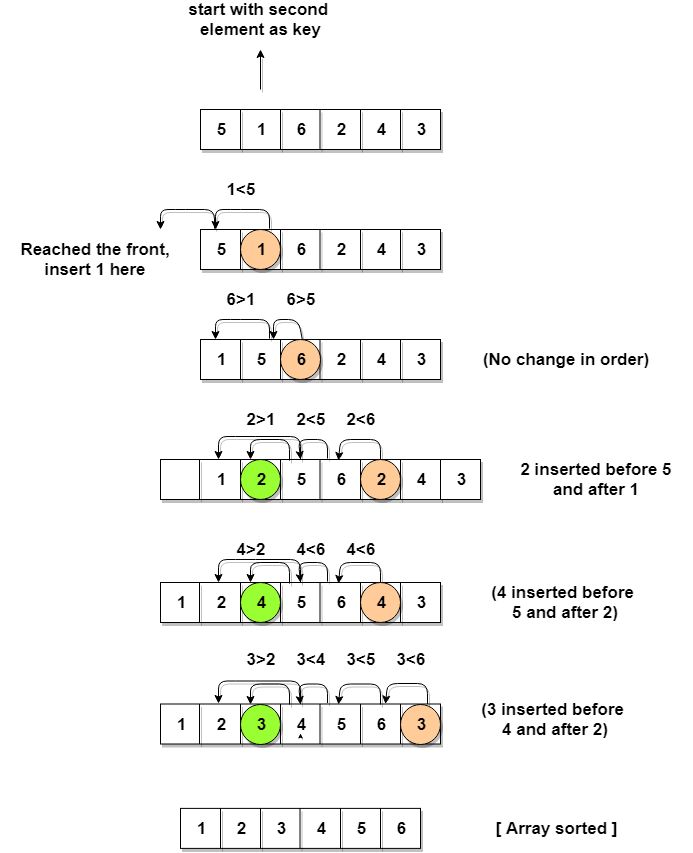
As you can see in the diagram above, after picking a key
, we start iterating over the elements to the left of the key
.
如您在上图中所看到的,选择一个key
,我们开始遍历key
左侧的元素。
We continue to move towards left if the elements are greater than the key
element and stop when we find the element which is less than the key
element.
如果元素大于key
元素,我们将继续向左移动;当发现小于key
元素的元素时,我们将停止。
And, insert the key
element after the element which is less than the key
element.
并且,在小于key
元素的元素之后插入key
元素。
实现插入排序算法 (Implementing Insertion Sort Algorithm)
Below we have a simple implementation of Insertion sort in C++ language.
下面,我们用C ++语言简单地实现了插入排序。
#include <stdlib.h>
#include <iostream>
using namespace std;
//member functions declaration
void insertionSort(int arr[], int length);
void printArray(int array[], int size);
// main function
int main()
{
int array[5] = {5, 1, 6, 2, 4, 3};
// calling insertion sort function to sort the array
insertionSort(array, 6);
return 0;
}
void insertionSort(int arr[], int length)
{
int i, j, key;
for (i = 1; i < length; i++)
{
j = i;
while (j > 0 && arr[j - 1] > arr[j])
{
key = arr[j];
arr[j] = arr[j - 1];
arr[j - 1] = key;
j--;
}
}
cout << "Sorted Array: ";
// print the sorted array
printArray(arr, length);
}
// function to print the given array
void printArray(int array[], int size)
{
int j;
for (j = 0; j < size; j++)
{
cout <
Sorted Array: 1 2 3 4 5 6
排序数组:1 2 3 4 5 6
Now let's try to understand the above simple insertion sort algorithm.
现在,让我们尝试了解上述简单的插入排序算法。
We took an array with 6 integers. We took a variable key
, in which we put each element of the array, during each pass, starting from the second element, that is a[1]
.
我们使用了一个包含6个整数的数组。 我们使用了一个可变key
,在每次通过期间,我们将从第二个元素a[1]
开始,将数组的每个元素放入其中。
Then using the while
loop, we iterate, until j
becomes equal to zero or we find an element which is greater than key
, and then we insert the key
at that position.
然后使用while
循环,进行迭代,直到j
等于零或找到一个大于key
的元素,然后将 key
插入该位置。
We keep on doing this, until j
becomes equal to zero, or we encounter an element which is smaller than the key
, and then we stop. The current key
is now at the right position.
我们继续这样做,直到j
等于零 ,或者遇到一个小于key
的元素,然后停止。 现在,当前key
位于正确的位置。
We then make the next element as key
and then repeat the same process.
然后,将下一个元素作为key
,然后重复相同的过程。
In the above array, first we pick 1 as key
, we compare it with 5(element before 1), 1 is smaller than 5, we insert 1 before 5. Then we pick 6 as key
, and compare it with 5 and 1, no shifting in position this time. Then 2 becomes the key
and is compared with 6 and 5, and then 2 is inserted after 1. And this goes on until the complete array gets sorted.
在上面的数组中,首先我们选择1作为key
,然后将其与5 (元素在1之前)进行比较, 1小于5 ,在5之前插入1 。 然后,我们选择6作为key
,并将其与5和1进行比较,这次没有位置偏移。 然后2成为key
,并与6和5相比较,然后2被1之后插入。 然后继续进行,直到对整个数组进行排序为止。
插入排序的复杂度分析 (Complexity Analysis of Insertion Sort)
As we mentioned above that insertion sort is an efficient sorting algorithm, as it does not run on preset conditions using for
loops, but instead it uses one while
loop, which avoids extra steps once the array gets sorted.
正如我们上面提到的,插入排序是一种有效的排序算法,因为它不使用for
循环在预设条件下运行,而是使用一个while
循环,这样可以避免对数组进行排序后的额外步骤。
Even though insertion sort is efficient, still, if we provide an already sorted array to the insertion sort algorithm, it will still execute the outer for
loop, thereby requiring n
steps to sort an already sorted array of n
elements, which makes its best case time complexity a linear function of n
.
即使插入排序很有效,但是,如果我们向插入排序算法提供一个已经排序的数组,它仍将执行外部for
循环,从而需要n
步骤来排序一个已经排序的n
元素的数组,这是最好的情况时间复杂度 n
的线性函数。
Worst Case Time Complexity [ Big-O ]: O(n2)
最坏情况下的时间复杂度[Big-O]: O(n 2 )
Best Case Time Complexity [Big-omega]: O(n)
最佳情况下的时间复杂度[大Ω]: O(n)
Average Time Complexity [Big-theta]: O(n2)
平均时间复杂度[Big-theta]: O(n 2 )
Space Complexity: O(1)
空间复杂度: O(1)
翻译自: https://www.studytonight.com/data-structures/insertion-sorting
插入排序算法?